pygraphblas
Installation
pygraphblas requires the SuiteSparse:GraphBLAS library. The easiest way currently to install pygraphblas on Linux is to use pip:
pip install pygraphblas
This is currently only supported on Linux but Windows and MacOS binary packages are currently a work in progress.
To install from source, first install SuiteSparse, then run:
python setup.py install
There are two ways to download precompiled binaries of pygraphblas
with SuiteSparse included.
One way is to use pip install
pygraphblas
on an Intel Linux machine.
This will download a package
compatible with most modern linux distributions.
This also works in a
Docker container on Mac.
There are also pre-build docker images based on Ubuntu 20.04 that have
a pre-compiled SuiteSparse and pygraphblas installed.
These come in
two flavors minimal
which is the Ipython interpreter-only, and
notebook
which comes with a complete Jupyter Notebook server.
These
containers also work on Mac.
An installation script for Ubuntu 18.04 is provided in the
install-ubuntu.sh
file.
NOTE: DO NOT USE THESE PRE-COMPILED BINARIES FOR BENCHMARKING SUITESPARSE. These binaries are not guaranteed to be idealy compiled for your environment.
Docker
pygraphblas is distributed as two different docker images on Docker Hub . The "minimal" image, containing only the library and ipython and can be run with the command:
docker run --rm -it graphblas/pygraphblas-minimal ipython
You can run a "full" Jupyter notebook server with docker and try the example Notebooks use the command:
docker run --rm -it graphblas/pygraphblas-notebook
Open up the URL printed on your terminal screen, typically something
liker http://127.0.0.1:8888/tree/pygraphblas/demo
and see the
following Notebooks:
- Introduction to GraphBLAS with Python
- PageRank
- Betweeness Centrality
- K-Truss Subgraphs
- Triangle Counting
- Louvain Community Detection
- RadiX-Net Topologies
- User Defined Types
- Log Semiring Type
- Kronecker Power Graphs
Tests
To run the tests checkout pygraphblas and use:
$ ./test.sh
Summary
pygraphblas is a python extension that bridges The GraphBLAS API with the Python programming language. It uses the CFFI library to wrap the low level GraphBLAS API and provides high level Matrix and Vector Python types that make GraphBLAS simple and easy.
GraphBLAS is a sparse linear algebra API optimized for processing graphs encoded as sparse matrices and vectors. In addition to common real/integer matrix algebra operations, GraphBLAS supports over a thousand different Semiring algebra operations, that can be used as basic building blocks to implement a wide variety of graph algorithms. See Applications from Wikipedia for some specific examples.
pygraphblas leverages the expertise in the field of sparse matrix programming by The GraphBLAS Forum and uses the SuiteSparse:GraphBLAS API implementation. SuiteSparse:GraphBLAS is brought to us by the work of Dr. Tim Davis, professor in the Department of Computer Science and Engineering at Texas A&M University. News and information can provide you with a lot more background information, in addition to the references below.
While it is my goal to make it so that pygraphblas works with any GraphBLAS implementation, it currently only works with SuiteSparse. SuiteSparse is currently the only realistically usable GraphBLAS implementation, and additionally it provides several "extension" features and pre-packaged objects that are very useful for pygraphblas. If there is a GraphBLAS implementation you would like to see support for in pygraphblas, please consider creating an issue for it for discussion and/or sending me a pull request.
Introduction to Graphs and Matrices
GraphBLAS uses matrices and Linear Algebra to represent graphs, as described in this mathmatical introduction to GraphBLAS by Dr. Jeremy Kepner head and founder of MIT Lincoln Laboratory Supercomputing Center.
There are two useful matrix representations of graphs: Adjacency Matrices and Incidence Matrices. An Adjacency Matrix can hold a simple graph where a single edge joins one source node to one destination:
On the left is a graph, and on the right, the adjacency matrix that represents it. The matrix has a row and column for every node in the graph. If there is an edge going from node A to B, then there will be a value present in the intersection of As row with Bs column. How it differs from many other matrix representations is that the matrix is sparse, nothing is stored in computer memory where there are unused elements.
Two Incidence Matrices are used to store a relationship between two distinct sets of objects of nodes and edge elements.
Sparsity is important because one practical problem with matrix-encoding graphs is that most real-world graphs tend to be sparse, as above, only 7 of 36 possible elements have a value. Those that have values tend to be scattered randomly across the matrix (for "typical" graphs), so dense linear algebra libraries like BLAS or numpy do not encode or operate on them efficiently, as the relevant data is mostly empty memory with actual data elements spaced far apart. This wastes memory and CPU resources, and defeats CPU caching mechanisms.
For example, suppose a fictional social network has 1 billion users, and each user has about 100 friends, which means there are about 100 billion (1e+11) connections in the graph. A dense matrix large enough to hold this graph would need (1 billion)^2 or (1,000,000,000,000,000,000), a "quintillion" elements, but only 1e+11 of them would have meaningful values, leaving only 0.0000001th of the matrix being utilized.
By using a sparse matrix instead of dense, only the elements used are actually stored in memory. The parts of the matrix with no value are interpreted, but not necessarily stored, as an identity value, which may or may not be the actual number zero, but possibly other values like positive or negative infinity depending on the particular semiring operations applied to the matrix.
Semirings encapsulate different algebraic operations and identities that can be used to multiply matrices and vectors. Anyone who has multiplied matrices has used at least one Semiring before, typically referred to as "plus_times". This is the common operation of multiplying two matrices containing real numbers, the corresponding row and column entries are multipled and the results are summed for the final value.
Using the API
The core idea of the GraphBLAS is the mathematical duality between a
graph and a Matrix
.
There are a few ways to contstruct
matricies, but a simple approach is to provide three lists of data,
the first are are lists of the row and column positions that define
the begining and end of a graph edge, and the third list is the weight
for that edge:
>>> import pygraphblas as gb
>>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6]
>>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4]
>>> V = [True] * len(I)
>>> M = gb.Matrix.from_lists(I, J, V)
>>> print(M)
0 1 2 3 4 5 6
0| t t | 0
1| t t| 1
2| t | 2
3| t t | 3
4| t | 4
5| t | 5
6| t t t | 6
0 1 2 3 4 5 6
>>> from pygraphblas import gviz
>>> g = gviz.draw_graph(M, weights=False,
... filename='docs/imgs/Matrix_from_lists2')
>>> g = gviz.draw_matrix(M, scale=40,
... filename='docs/imgs/Matrix_from_lists_matrix')
GraphBLAS is a sparse Linear
Algebra API optimized
for processing graphs encoded as sparse matrices and vectors.
In
addition to common real/integer Matrix
algebra
operations, GraphBLAS supports over a thousand different
Semiring algebra operations,
that can be used as basic building blocks to implement a wide variety
of graph algorithms. See
Applications
from Wikipedia for some specific examples.
The core operation of Linear Algebra is Matrix Multiplication. In this GraphBLAS duality, this is an operation along the edges of a graph from nodes to their adjacenct neighbors, taking one step in a Breadth First Search across the graph:
>>> v = gb.Vector.from_lists([0], [True], M.nrows)
>>> y = v @ M
>>> g = gviz.draw_matrix_op(v, '@', M, y, scale=40, labels=True,
... column=False, filename='docs/imgs/bfs_step')
pygraphblas leverages the expertise in the field of sparse matrix programming by The GraphBLAS Forum and uses the SuiteSparse:GraphBLAS API implementation. SuiteSparse:GraphBLAS is brought to us by the work of Dr. Tim Davis, professor in the Department of Computer Science and Engineering at Texas A&M University. News and information can provide you with a lot more background information.
Expand source code
"""# Installation
pygraphblas requires the
[SuiteSparse:GraphBLAS](http://faculty.cse.tamu.edu/davis/GraphBLAS.html)
library. The easiest way currently to install pygraphblas on Linux is
to use pip:
pip install pygraphblas
This is currently only supported on Linux but Windows and MacOS binary
packages are currently a work in progress.
To install from source, first install SuiteSparse, then run:
python setup.py install
There are two ways to download precompiled binaries of pygraphblas
with SuiteSparse included. One way is to use `pip install
pygraphblas` on an Intel Linux machine. This will download a package
compatible with most modern linux distributions. This also works in a
Docker container on Mac.
There are also pre-build docker images based on Ubuntu 20.04 that have
a pre-compiled SuiteSparse and pygraphblas installed. These come in
two flavors `minimal` which is the Ipython interpreter-only, and
`notebook` which comes with a complete Jupyter Notebook server. These
containers also work on Mac.
An installation script for Ubuntu 18.04 is provided in the
`install-ubuntu.sh` file.
NOTE: DO NOT USE THESE PRE-COMPILED BINARIES FOR BENCHMARKING
SUITESPARSE. These binaries are not guaranteed to be idealy compiled
for your environment.
## Docker
pygraphblas is distributed as two different docker images on [Docker
Hub](https://cloud.docker.com/repository/docker/pygraphblas/pygraphblas/general)
. The "minimal" image, containing only the library and
[ipython](https://ipython.org/) and can be run with the command:
docker run --rm -it graphblas/pygraphblas-minimal ipython
You can run a "full" [Jupyter notebook](https://jupyter.org/) server
with docker and try the example Notebooks use the command:
docker run --rm -it graphblas/pygraphblas-notebook
Open up the URL printed on your terminal screen, typically something
liker `http://127.0.0.1:8888/tree/pygraphblas/demo` and see the
following Notebooks:
- [Introduction to GraphBLAS with Python](https://github.com/Graphegon/pygraphblas/blob/main/demo/Introduction-to-GraphBLAS-with-Python.ipynb)
- [PageRank](https://github.com/Graphegon/pygraphblas/blob/main/demo/PageRank.ipynb)
- [Betweeness Centrality](https://github.com/Graphegon/pygraphblas/blob/main/demo/BetweenessCentrality.ipynb)
- [K-Truss Subgraphs](https://github.com/Graphegon/pygraphblas/blob/main/demo/K-Truss.ipynb)
- [Triangle Counting](https://github.com/Graphegon/pygraphblas/blob/main/demo/Triangle-Counting.ipynb)
- [Louvain Community Detection](https://github.com/Graphegon/pygraphblas/blob/main/demo/Louvain.ipynb)
- [RadiX-Net Topologies](https://github.com/Graphegon/pygraphblas/blob/main/demo/RadiX-Net-with-pygraphblas.ipynb)
- [User Defined Types](https://github.com/Graphegon/pygraphblas/blob/main/demo/User-Defined-Types.ipynb)
- [Log Semiring Type](https://github.com/Graphegon/pygraphblas/blob/main/demo/Log-Semiring.ipynb)
- [Kronecker Power Graphs](https://github.com/Graphegon/pygraphblas/blob/main/demo/Sierpinski-Graph.ipynb)
# Tests
To run the tests checkout pygraphblas and use:
$ ./test.sh
# Summary
pygraphblas is a python extension that bridges [The GraphBLAS
API](http://graphblas.org) with the [Python](https://python.org)
programming language. It uses the
[CFFI](https://cffi.readthedocs.io/en/latest/) library to wrap the low
level GraphBLAS API and provides high level Matrix and Vector Python
types that make GraphBLAS simple and easy.
GraphBLAS is a sparse linear algebra API optimized for processing
graphs encoded as sparse matrices and vectors. In addition to common
real/integer matrix algebra operations, GraphBLAS supports over a
thousand different [Semiring](https://en.wikipedia.org/wiki/Semiring)
algebra operations, that can be used as basic building blocks to
implement a wide variety of graph algorithms. See
[Applications](https://en.wikipedia.org/wiki/Semiring#Applications)
from Wikipedia for some specific examples.
pygraphblas leverages the expertise in the field of sparse matrix
programming by [The GraphBLAS Forum](http://graphblas.org) and uses
the
[SuiteSparse:GraphBLAS](http://faculty.cse.tamu.edu/davis/GraphBLAS.html)
API implementation. SuiteSparse:GraphBLAS is brought to us by the work
of [Dr. Tim Davis](http://faculty.cse.tamu.edu/davis/welcome.html),
professor in the Department of Computer Science and Engineering at
Texas A&M University. [News and
information](http://faculty.cse.tamu.edu/davis/news.html) can provide
you with a lot more background information, in addition to the
references below.
While it is my goal to make it so that pygraphblas works with any
GraphBLAS implementation, it currently only works with SuiteSparse.
SuiteSparse is currently the only realistically usable GraphBLAS
implementation, and additionally it provides several "extension"
features and pre-packaged objects that are very useful for
pygraphblas. If there is a GraphBLAS implementation you would like to
see support for in pygraphblas, please consider creating an issue for
it for discussion and/or sending me a pull request.
# Introduction to Graphs and Matrices
GraphBLAS uses matrices and Linear Algebra to represent graphs, as
described [in this mathmatical introduction to
GraphBLAS](http://www.mit.edu/~kepner/GraphBLAS/GraphBLAS-Math-release.pdf)
by [Dr. Jeremy Kepner](http://www.mit.edu/~kepner/) head and founder
of [MIT Lincoln Laboratory Supercomputing
Center](http://news.mit.edu/2016/lincoln-laboratory-establishes-supercomputing-center-0511).
There are two useful matrix representations of graphs: [Adjacency
Matrices](https://en.wikipedia.org/wiki/Adjacency_matrix) and
[Incidence Matrices](https://en.wikipedia.org/wiki/Incidence_matrix).
An Adjacency Matrix can hold a simple graph where a single edge joins
one source node to one destination:
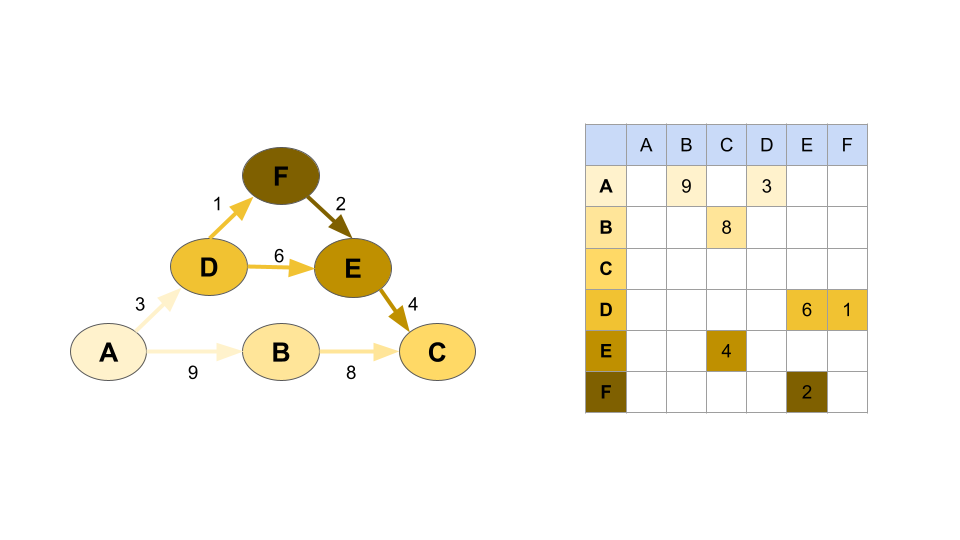
On the left is a graph, and on the right, the adjacency matrix that
represents it. The matrix has a row and column for every node in the
graph. If there is an edge going from node A to B, then there will be
a value present in the intersection of As row with Bs column. How it
differs from many other matrix representations is that the matrix is
sparse, nothing is stored in computer memory where there are unused
elements.
Two Incidence Matrices are used to store a relationship between two
distinct sets of objects of nodes and edge elements.
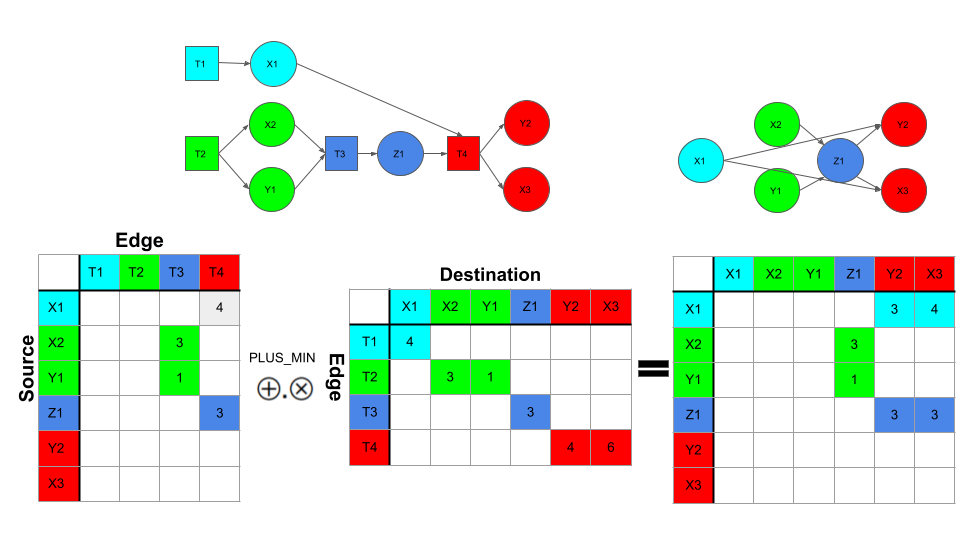
Sparsity is important because one practical problem with
matrix-encoding graphs is that most real-world graphs tend to be
sparse, as above, only 7 of 36 possible elements have a value. Those
that have values tend to be scattered randomly across the matrix
(for "typical" graphs), so dense linear algebra libraries like BLAS or
numpy do not encode or operate on them efficiently, as the relevant
data is mostly empty memory with actual data elements spaced far
apart. This wastes memory and CPU resources, and defeats CPU caching
mechanisms.
For example, suppose a fictional social network has 1 billion users,
and each user has about 100 friends, which means there are about 100
billion (1e+11) connections in the graph. A dense matrix large enough
to hold this graph would need (1 billion)^2 or
(1,000,000,000,000,000,000), a "quintillion" elements, but only 1e+11
of them would have meaningful values, leaving only 0.0000001th of the
matrix being utilized.
By using a sparse matrix instead of dense, only the elements used are
actually stored in memory. The parts of the matrix with no value are
*interpreted*, but not necessarily stored, as an identity value, which
may or may not be the actual number zero, but possibly other values
like positive or negative infinity depending on the particular
semiring operations applied to the matrix.
Semirings encapsulate different algebraic operations and identities
that can be used to multiply matrices and vectors. Anyone who has
multiplied matrices has used at least one Semiring before, typically
referred to as "plus_times". This is the common operation of
multiplying two matrices containing real numbers, the corresponding row
and column entries are multipled and the results are summed for the
final value.
# Using the API
The core idea of the GraphBLAS is the mathematical duality between a
graph and a `pygraphblas.Matrix`. There are a few ways to contstruct
matricies, but a simple approach is to provide three lists of data,
the first are are lists of the row and column positions that define
the begining and end of a graph edge, and the third list is the weight
for that edge:
>>> import pygraphblas as gb
>>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6]
>>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4]
>>> V = [True] * len(I)
>>> M = gb.Matrix.from_lists(I, J, V)
>>> print(M)
0 1 2 3 4 5 6
0| t t | 0
1| t t| 1
2| t | 2
3| t t | 3
4| t | 4
5| t | 5
6| t t t | 6
0 1 2 3 4 5 6
>>> from pygraphblas import gviz
>>> g = gviz.draw_graph(M, weights=False,
... filename='docs/imgs/Matrix_from_lists2')
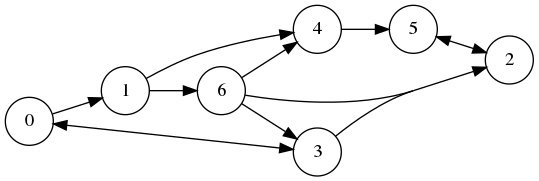
>>> g = gviz.draw_matrix(M, scale=40,
... filename='docs/imgs/Matrix_from_lists_matrix')
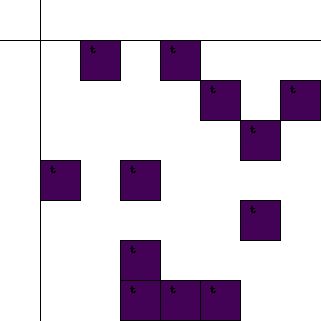
GraphBLAS is a sparse [Linear
Algebra](https://en.wikipedia.org/wiki/Linear_algebra) API optimized
for processing graphs encoded as sparse matrices and vectors. In
addition to common real/integer `pygraphblas.Matrix` algebra
operations, GraphBLAS supports over a thousand different
[Semiring](https://en.wikipedia.org/wiki/Semiring) algebra operations,
that can be used as basic building blocks to implement a wide variety
of graph algorithms. See
[Applications](https://en.wikipedia.org/wiki/Semiring#Applications)
from Wikipedia for some specific examples.
The core operation of Linear Algebra is [Matrix
Multiplication](https://en.wikipedia.org/wiki/Matrix_multiplication).
In this GraphBLAS duality, this is an operation along the edges of a
graph from nodes to their adjacenct neighbors, taking one step in a
[Breadth First
Search](https://en.wikipedia.org/wiki/Breadth-first_search) across the
graph:
>>> v = gb.Vector.from_lists([0], [True], M.nrows)
>>> y = v @ M
>>> g = gviz.draw_matrix_op(v, '@', M, y, scale=40, labels=True,
... column=False, filename='docs/imgs/bfs_step')
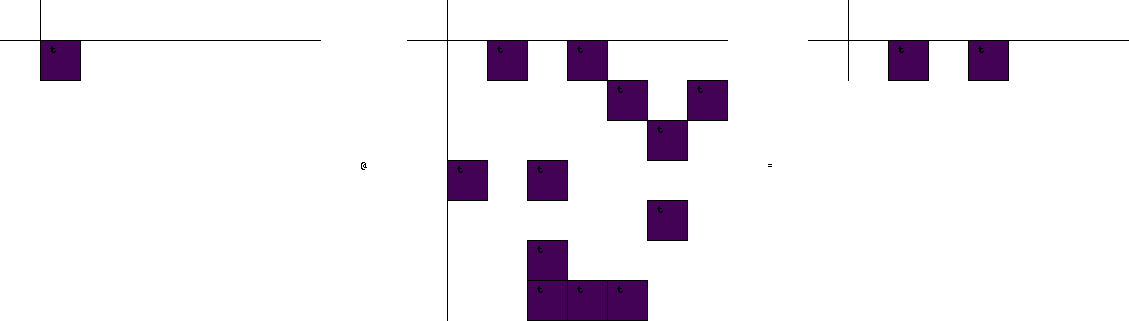
pygraphblas leverages the expertise in the field of sparse matrix
programming by [The GraphBLAS Forum](http://graphblas.org) and uses
the
[SuiteSparse:GraphBLAS](http://faculty.cse.tamu.edu/davis/GraphBLAS.html)
API implementation. SuiteSparse:GraphBLAS is brought to us by the work
of [Dr. Tim Davis](http://faculty.cse.tamu.edu/davis/welcome.html),
professor in the Department of Computer Science and Engineering at
Texas A&M University. [News and
information](http://faculty.cse.tamu.edu/davis/news.html) can provide
you with a lot more background information.
"""
from suitesparse_graphblas import lib, ffi, initialize, is_initialized
def init(blocking=False):
initialize(blocking=blocking, memory_manager="c")
if not is_initialized(): # pragma: nocover
init()
from .base import (
lib,
ffi,
GxB_INDEX_MAX,
GxB_IMPLEMENTATION,
GxB_SPEC,
options_get,
options_set,
)
IMPLEMENTATION_MAJOR = lib.GxB_IMPLEMENTATION_MAJOR
IMPLEMENTATION_MINOR = lib.GxB_IMPLEMENTATION_MINOR
IMPLEMENTATION_SUB = lib.GxB_IMPLEMENTATION_SUB
IMPLEMENTATION_VERSION = (
IMPLEMENTATION_MAJOR,
IMPLEMENTATION_MINOR,
IMPLEMENTATION_SUB,
)
from . import _version
__version__ = _version.get_versions()["version"]
def get_version(): # pragma: nocover
"""Return the pygraphblas version."""
return __version__
from .semiring import build_semirings
from .binaryop import build_binaryops, Accum, binary_op
from .unaryop import build_unaryops, unary_op
from .selectop import build_selectops, select_op
from .monoid import build_monoids
from .matrix import Matrix
from .vector import Vector
from .scalar import Scalar
from . import descriptor
__pdoc__ = {
"base": False,
"build": False,
"unaryop": False,
"binaryop": False,
"monoid": False,
"semiring": False,
"matrix": False,
"vector": False,
"scalar": False,
"types": False,
"run_doctests": False,
"descriptor": True,
"selectop": True,
}
build_semirings(__pdoc__)
build_binaryops(__pdoc__)
build_unaryops(__pdoc__)
build_monoids(__pdoc__)
build_selectops(__pdoc__)
from .types import (
BOOL,
FP64,
FP32,
FC64,
FC32,
INT64,
INT32,
INT16,
INT8,
UINT64,
UINT32,
UINT16,
UINT8,
)
__all__ = [
"GxB_INDEX_MAX",
"GxB_IMPLEMENTATION",
"GxB_SPEC",
"Matrix",
"Vector",
"Scalar",
"Accum",
"BOOL",
"FP64",
"FP32",
"FC64",
"FC32",
"INT64",
"INT32",
"INT16",
"INT8",
"UINT64",
"UINT32",
"UINT16",
"UINT8",
"descriptor",
"selectop",
"binary_op",
"unary_op",
"select_op",
"options_set",
"options_get",
]
GxB_INDEX_MAX = GxB_INDEX_MAX
"""Maximum key size for SuiteSparse, defaults to `2**60`."""
GxB_IMPLEMENTATION = GxB_IMPLEMENTATION
""" Tuple containing GxB_IMPLEMENTATION (MAJOR, MINOR, SUB) """
GxB_SPEC = GxB_SPEC
""" Tuple containing GxB_SPEC (MAJOR, MINOR, SUB) """
def run_doctests(raise_on_error=False):
from . import matrix
from . import vector
from . import descriptor
from . import base
from . import unaryop
from . import binaryop
import sys, doctest
this = sys.modules[__name__]
for mod in (
this,
selectop,
unaryop,
binaryop,
matrix,
vector,
descriptor,
base,
):
doctest.testmod(
mod, optionflags=doctest.ELLIPSIS, raise_on_error=raise_on_error
)
from . import _version
__version__ = _version.get_versions()["version"]
Types
class Matrix (matrix, typ=None)
-
GraphBLAS Sparse Matrix
This is a high-level wrapper around the GrB_Matrix C type using the cffi library.
A Matrix supports many possible operations according to the GraphBLAS API. Many of those operations have overloaded operators.
Operator Description Default A @ B Matrix Matrix Multiplication type default PLUS_TIMES semiring v @ A Vector Matrix Multiplication type default PLUS_TIMES semiring A @ v Matrix Vector Multiplication type default PLUS_TIMES semiring A @= B In-place Matrix Matrix Multiplication type default PLUS_TIMES semiring v @= A In-place Vector Matrix Multiplication type default PLUS_TIMES semiring A @= v In-place Matrix Vector Multiplication type default PLUS_TIMES semiring A | B Matrix Union type default SECOND combiner A |= B In-place Matrix Union type default SECOND combiner A & B Matrix Intersection type default SECOND combiner A &= B In-place Matrix Intersection type default SECOND combiner A + B Matrix Element-Wise Union type default PLUS combiner A += B In-place Matrix Element-Wise Union type default PLUS combiner A - B Matrix Element-Wise Union type default MINUS combiner A -= B In-place Matrix Element-Wise Union type default MINUS combiner A * B Matrix Element-Wise Intersection type default TIMES combiner A *= B In-place Matrix Element-Wise Intersection type default TIMES combiner A / B Matrix Element-Wise Intersection type default DIV combiner A /= B In-place Matrix Element-Wise Intersection type default DIV combiner A == B Compare Element-Wise Union type default EQ operator A != B Compare Element-Wise Union type default NE operator A < B Compare Element-Wise Union type default LT operator A > B Compare Element-Wise Union type default GT operator A <= B Compare Element-Wise Union type default LE operator A >= B Compare Element-Wise Union type default GE operator Note that all the above operator syntax is mearly sugar over various combinations of calling
Matrix.mxm()
,Matrix.mxv()
,Vector.vxm()
,Matrix.eadd()
, andMatrix.emult()
.Expand source code
class Matrix: """GraphBLAS Sparse Matrix This is a high-level wrapper around the GrB_Matrix C type using the [cffi](https://cffi.readthedocs.io/en/latest/) library. A Matrix supports many possible operations according to the GraphBLAS API. Many of those operations have overloaded operators. Operator | Description | Default --- | --- | --- A @ B | Matrix Matrix Multiplication | type default PLUS_TIMES semiring v @ A | Vector Matrix Multiplication | type default PLUS_TIMES semiring A @ v | Matrix Vector Multiplication | type default PLUS_TIMES semiring A @= B | In-place Matrix Matrix Multiplication | type default PLUS_TIMES semiring v @= A | In-place Vector Matrix Multiplication | type default PLUS_TIMES semiring A @= v | In-place Matrix Vector Multiplication | type default PLUS_TIMES semiring A \\| B | Matrix Union | type default SECOND combiner A \\|= B | In-place Matrix Union | type default SECOND combiner A & B | Matrix Intersection | type default SECOND combiner A &= B | In-place Matrix Intersection | type default SECOND combiner A + B | Matrix Element-Wise Union | type default PLUS combiner A += B | In-place Matrix Element-Wise Union | type default PLUS combiner A - B | Matrix Element-Wise Union | type default MINUS combiner A -= B | In-place Matrix Element-Wise Union | type default MINUS combiner A * B | Matrix Element-Wise Intersection | type default TIMES combiner A *= B | In-place Matrix Element-Wise Intersection | type default TIMES combiner A / B | Matrix Element-Wise Intersection | type default DIV combiner A /= B | In-place Matrix Element-Wise Intersection | type default DIV combiner A == B | Compare Element-Wise Union | type default EQ operator A != B | Compare Element-Wise Union | type default NE operator A < B | Compare Element-Wise Union | type default LT operator A > B | Compare Element-Wise Union | type default GT operator A <= B | Compare Element-Wise Union | type default LE operator A >= B | Compare Element-Wise Union | type default GE operator Note that all the above operator syntax is mearly sugar over various combinations of calling `Matrix.mxm`, `Matrix.mxv`, `pygraphblas.Vector.vxm`, `Matrix.eadd`, and `Matrix.emult`. """ __slots__ = ("_matrix", "type", "_funcs", "_keep_alives") def __init__(self, matrix, typ=None): if typ is None: new_type = ffi.new("GrB_Type*") _check(self, lib.GxB_Matrix_type(new_type, matrix[0])) typ = types._gb_type_to_type(new_type[0]) self._matrix = matrix self.type = typ """The type of the Matrix. >>> M = Matrix.sparse(types.INT8) >>> M.type == types.INT8 True """ self._keep_alives = weakref.WeakKeyDictionary() def __del__(self): _check(self, lib.GrB_Matrix_free(self._matrix)) @classmethod def sparse(cls, typ, nrows=None, ncols=None, fill=None, mask=None): """Create an empty sparse Matrix from the given type. The dimensions can be specified with `nrows` and `ncols`. If no dimensions are specified, they default to `GxB_INDEX_MAX`. >>> m = Matrix.sparse(types.UINT8) >>> m.nrows == lib.GxB_INDEX_MAX True >>> m.ncols == lib.GxB_INDEX_MAX True >>> m.nvals == 0 True Optional row and column dimension bounds can be provided to the method: >>> m = Matrix.sparse(types.UINT8, 10, 10) >>> m.nrows == 10 True >>> m.ncols == 10 True >>> m.nvals == 0 True One of the Python types `(bool, int, float, complex)` can be passed instead. They are turned into `(BOOL, INT64, FP64, FC64)` respectively: >>> Matrix.sparse(int) <Matrix(INT64, nvals: 0)> A sparse matrix can be "filled" with a starting value using the `fill` parameter and the `mask` parameter. The `mask` is required otherwise `fill` is ignored. >>> mask = Matrix.sparse(types.BOOL) >>> mask[1,1] = True >>> list(Matrix.sparse(float, fill=3.14, mask=mask)) [(1, 1, 3.14)] If `mask` is provided but no `fill`, the `type.default_zero` value is used: >>> list(Matrix.sparse(float, mask=mask)) [(1, 1, 0.0)] """ if nrows is None: nrows = lib.GxB_INDEX_MAX if ncols is None: ncols = lib.GxB_INDEX_MAX new_mat = ffi.new("GrB_Matrix*") if not issubclass(typ, types.Type): typ = types._gb_from_type(typ) _base_check(lib.GrB_Matrix_new(new_mat, typ._gb_type, nrows, ncols)) m = cls(new_mat, typ) if mask is not None: if fill is None: fill = m.type.default_zero m.assign_scalar(fill, mask=mask) return m @classmethod def dense(cls, typ, nrows=None, ncols=None, fill=None, sparsity=None): """Return a dense Matrix nrows by ncols. If `sparsity` is provided it is used for the sparsity of the new matrix See the [SuiteSparse User Guide](https://raw.githubusercontent.com/DrTimothyAldenDavis/GraphBLAS/stable/Doc/GraphBLAS_UserGuide.pdf) for details. >>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M) 0 1 2 0| 0 0 0| 0 1| 0 0 0| 1 2| 0 0 0| 2 0 1 2 If a `fill` value is present, use that, otherwise use the `self.type.default_zero` attribute of the given type. >>> M = Matrix.dense(types.UINT8, 3, 3, fill=1) >>> print(M) 0 1 2 0| 1 1 1| 0 1| 1 1 1| 1 2| 1 1 1| 2 0 1 2 A dense matrix can be the maximum possible dimension, in which case it is an "iso" valued matrix. >>> M = Matrix.dense(types.UINT8) >>> M.nrows == lib.GxB_INDEX_MAX True >>> M[42,42] 0 """ if nrows is None: nrows = lib.GxB_INDEX_MAX if ncols is None: ncols = lib.GxB_INDEX_MAX assert nrows > 0 and ncols > 0, "dense matrix must be at least 1x1" m = cls.sparse(typ, nrows, ncols) if sparsity is not None: m.sparsity = sparsity if fill is None: fill = m.type.default_zero m[:, :] = fill return m @classmethod def iso(cls, value, nrows=None, ncols=None): """Build a dense "iso" matrix from a scalar value. This is similar to `Matrix.dense` but infers the type of the new Matrix from the provided vbalue. >>> M = Matrix.iso(3) >>> assert M[42,42] == 3 >>> M = Matrix.iso(3, 2, 2) >>> print(M) 0 1 0| 3 3| 0 1| 3 3| 1 0 1 If you change an iso matrix, it is no longer stored as an iso object, and your matrix will grow in size. >>> M[1,1] = 2 >>> print(M) 0 1 0| 3 3| 0 1| 3 2| 1 0 1 """ if nrows is None: nrows = lib.GxB_INDEX_MAX if ncols is None: ncols = lib.GxB_INDEX_MAX typ = types._gb_from_type(type(value)) return cls.dense(typ, nrows, ncols, value) @classmethod def from_lists(cls, I, J, V=None, nrows=None, ncols=None, typ=None): """Create a new matrix from the given lists of row indices, column indices, and values. If nrows or ncols are not provided, they are computed from the max values of the provides row and column indices lists. >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> M = Matrix.from_lists(I, J) >>> print(M) 0 1 2 3 4 5 6 0| t t | 0 1| t t| 1 2| t | 2 3| t t | 3 4| t | 4 5| t | 5 6| t t t | 6 0 1 2 3 4 5 6 >>> from pygraphblas.gviz import draw_graph >>> draw_graph(M, filename='docs/imgs/Matrix_from_lists') <graphviz.dot.Digraph object at ...> 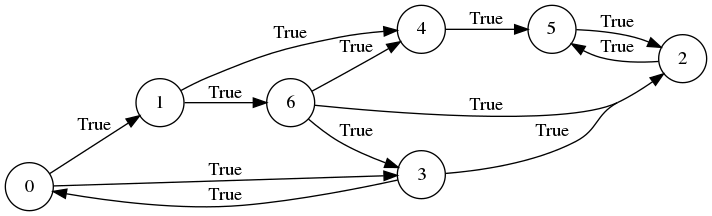 If the third argument is a scalar value instead of a list, it is used to construct an "iso" Matrix where all values equal that scalar. >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = True >>> M = Matrix.from_lists(I, J, V) >>> print(M) 0 1 2 3 4 5 6 0| t t | 0 1| t t| 1 2| t | 2 3| t t | 3 4| t | 4 5| t | 5 6| t t t | 6 0 1 2 3 4 5 6 """ assert len(I) == len(J) if V is None: V = True if isinstance(V, (bool, int, float)): V_func = lambda i: V else: V_func = lambda i: V[i] if not nrows: nrows = max(I) + 1 if not ncols: ncols = max(J) + 1 # TODO use ffi and GrB_Matrix_build if typ is None: typ = types._gb_from_type(type(V_func(0))) m = cls.sparse(typ, nrows, ncols) for v_i, (i, j) in enumerate(zip(I, J)): m[i, j] = V_func(v_i) return m @classmethod def from_diag(cls, v, k=0, desc=None): """ GxB_Matrix_diag constructs a matrix from a vector. Let n be the length of the v vector, from GrB_Vector_size (&n, v). If k = 0, then C is an n-by-n diagonal matrix with the entries from v along the main diagonal of C, with C(i,i) = v(i). If k is nonzero, C is square with dimension n+abs(k). If k is positive, it denotes diagonals above the main diagonal, with C(i,i+k) = v(i). If k is negative, it denotes diagonals below the main diagonal of C, with C(i-k,i) = v(i). This behavior is identical to the MATLAB statement C = diag(v,k), where v is a vector, except that GxB_Matrix_diag can also do typecasting. >>> v = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> print(Matrix.from_diag(v)) 0 1 2 0| 1 | 0 1| 2 | 1 2| 3| 2 0 1 2 >>> print(Matrix.from_diag(v, 1)) 0 1 2 3 0| 1 | 0 1| 2 | 1 2| 3| 2 3| | 3 0 1 2 3 >>> print(Matrix.from_diag(v, -1)) 0 1 2 3 0| | 0 1| 1 | 1 2| 2 | 2 3| 3 | 3 0 1 2 3 """ l = v.size + abs(k) C = cls.sparse(v.type, l, l) if desc is None: # pragma: nocover desc = current_desc.get(NULL) if desc is not NULL: # pragma: nocover desc = desc.get_desc() _check(C, lib.GxB_Matrix_diag(C._matrix[0], v._vector[0], k, desc)) return C @classmethod def from_mm(cls, mm_file): """Create a new matrix by reading a Matrix Market file. >>> from pathlib import Path >>> M = Matrix.from_mm(Path('docs/test_mm.mm')) >>> print(M) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 7 | 4 5| 8 | 5 6| 9 10 11 | 6 0 1 2 3 4 5 6 """ from mmparse import mmread, get_mm_type_converter with mmread(mm_file) as f: header, row_iter = f mm_type = header["mm_type"] nrows = header["nrows"] ncols = header["ncols"] symmetric = header["mm_storage"] == "symmetric" typ = get_mm_type_converter(mm_type) m = cls.sparse(typ, nrows, ncols) for l, i, j, v in row_iter: m[i, j] = v if symmetric: m[j, i] = v return m @classmethod def from_tsv(cls, tsv_file, typ, nrows, ncols, **kwargs): """Create a new matrix by reading a tab separated value file. >>> M = Matrix.from_tsv(Path('docs/test_tsvfile.tsv'), types.INT32, 7, 7) >>> print(M) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 7 | 4 5| 8 | 5 6| 9 10 11 | 6 0 1 2 3 4 5 6 """ kwargs["delimiter"] = "\t" return cls.from_csv(tsv_file, typ, nrows, ncols, **kwargs) @classmethod def from_csv( cls, csv_file, typ, nrows, ncols, one_based=True, **reader_kwargs ): # pragma: nocover """Create a new matrix by reading a comma separated value file. kwargs to this function are passed to the underlying `csv.Reader` object, so you can control various options like quoting and alternate delimiters that way. >>> M = Matrix.from_csv(Path('docs/test_tsvfile.tsv'), types.INT32, 7, 7, delimiter='\\t') >>> print(M) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 7 | 4 5| 8 | 5 6| 9 10 11 | 6 0 1 2 3 4 5 6 """ import csv if typ is types.BOOL: convert = bool elif typ in ( types.INT8, types.INT16, types.INT32, types.INT64, types.UINT8, types.UINT16, types.UINT32, types.UINT64, ): convert = int elif typ in (types.FP32, types.FP64): convert = float elif typ in (types.FC32, types.FC64): convert = complex M = cls.sparse(typ, nrows, ncols) with open(csv_file, newline="") as f: reader = csv.reader(f, **reader_kwargs) for row in reader: if len(row) > 3: raise TypeError("File can contain only 3 columns: row, col and val") i, j, v = row i = int(i) j = int(j) if one_based: i = i - 1 j = j - 1 M[i, j] = convert(v) return M @classmethod def binread(cls, bin_file, opener=Path.open): # pragma: nocover """Create a new matrix by reading a SuiteSparse specific binary file.""" from suitesparse_graphblas.io import binary matrix = binary.binread(bin_file, opener) return cls(matrix) from_binfile = binread @classmethod def random( cls, typ, nvals, nrows=lib.GxB_INDEX_MAX, ncols=lib.GxB_INDEX_MAX, make_pattern=False, make_symmetric=False, make_skew_symmetric=False, make_hermitian=True, no_diagonal=False, seed=None, ): # pragma: nocover """Create a new random Matrix of the given type, number of rows, columns and values. Other flags set additional properties the matrix will hold. >>> from .gviz import draw_graph >>> M = Matrix.random(types.UINT8, 20, 5, 5, ... make_symmetric=True, no_diagonal=True, seed=42) >>> draw_graph(M, filename='../docs/imgs/Matrix_random') <graphviz.dot.Digraph object at ...> 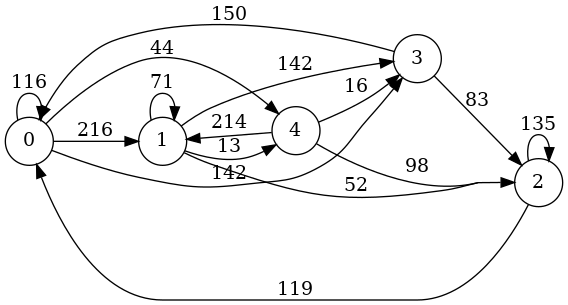 """ M = Matrix.sparse(typ, nrows, ncols) if seed is not None: random.seed(seed) if typ in (types.BOOL, types.UINT8, types.UINT16, types.UINT32, types.UINT64): make_skew_symmetric = False if M.nrows == 0 or M.ncols == 0: nvals = 0 if M.nrows != M.ncols: make_symmetric = False make_skew_symmetric = False make_hermitian = False if make_pattern or make_symmetric: make_skew_symmetric = False make_hermitian = False if make_skew_symmetric: make_hermitian = False no_diagonal = true if typ not in (types.FC32, types.FC64): make_hermitian = False if typ is types.BOOL: f = partial(random.randint, 0, 1) if typ is types.UINT8: f = partial(random.randint, 0, (2 ** 8) - 1) if typ is types.UINT16: f = partial(random.randint, 0, (2 ** 16) - 1) if typ is types.UINT32: f = partial(random.randint, 0, (2 ** 32) - 1) if typ is types.UINT64: f = partial(random.randint, 0, (2 ** 64) - 1) if typ is types.INT8: f = partial(random.randint, (-(2 ** 7)) + 1, (2 ** 7) - 1) if typ is types.INT16: f = partial(random.randint, (-(2 ** 15)) + 1, (2 ** 15) - 1) if typ is types.INT32: f = partial(random.randint, (-(2 ** 31)) + 1, (2 ** 31) - 1) if typ is types.INT64: f = partial(random.randint, (-(2 ** 63)) + 1, (2 ** 63) - 1) if typ in (types.FP32, types.FP64): f = random.random if typ in (types.FC32, types.FC64): f = lambda: complex(random.random(), random.random()) for i in range(nvals): i = random.randint(0, M.nrows - 1) j = random.randint(0, M.ncols - 1) M[i, j] = f() return M @classmethod def identity(cls, typ, nrows, value=None): """Return a new square identity Matrix of nrows with diagonal set to one. If one is None, use the default `Type.default_one` value. >>> M = Matrix.identity(types.UINT8, 3, value=42) >>> print(M) 0 1 2 0| 42 | 0 1| 42 | 1 2| 42| 2 0 1 2 """ result = cls.sparse(typ, nrows, nrows) if value is None: value = result.type.default_one for i in range(nrows): result[i, i] = value return result @classmethod def ssget(cls, name_or_id=None, binary_cache_dir=None): # pragma: nocover """Load a matrix from the [SuiteSparse Matrix Market](https://sparse.tamu.edu/). See [the ssgetpy library](https://github.com/drdarshan/ssgetpy) for search argument: >>> from pprint import pprint >>> from operator import itemgetter >>> pprint(sorted(list(Matrix.ssget('Newman/karate')), key=itemgetter(0))) [('karate.mtx', <Matrix(BOOL, shape: (34, 34), nvals: 156)>)] """ import ssgetpy results = [] result = ssgetpy.search(name_or_id)[0] mm_path, _ = result.download(extract=True) mm_path = Path(mm_path) for m in mm_path.glob("*.mtx"): Mbin = mm_path / (m.name + ".grb") if binary_cache_dir and Mbin.exists(): M = cls.from_binfile(Mbin) else: M = cls.from_mm(mm_path / m) if binary_cache_dir: M.to_binfile(Mbin) M.wait() yield m.name, M @property def gb_type(self): """Return the GraphBLAS low-level type object of the Matrix. This is only used if interacting with the low level API. >>> M = Matrix.sparse(types.INT8) >>> M.gb_type == lib.GrB_INT8 True """ new_type = ffi.new("GrB_Type*") _check(self, lib.GxB_Matrix_type(new_type, self._matrix[0])) return new_type[0] @property def nrows(self): """Return the number of Matrix rows. >>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.nrows 3 """ n = ffi.new("GrB_Index*") _check(self, lib.GrB_Matrix_nrows(n, self._matrix[0])) return n[0] @property def ncols(self): """Return the number of Matrix columns. >>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.ncols 3 """ n = ffi.new("GrB_Index*") _check(self, lib.GrB_Matrix_ncols(n, self._matrix[0])) return n[0] @property def shape(self): """Numpy-like description of matrix shape as 2-tuple (nrows, ncols). >>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.shape (3, 3) """ return (self.nrows, self.ncols) @property def square(self): """True if Matrix is square, else False. >>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.square True >>> M = Matrix.sparse(types.UINT8, 3, 4) >>> M.square False """ return self.nrows == self.ncols @property def nvals(self): """Return the number of values stored in the Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.nvals 3 """ n = ffi.new("GrB_Index*") _check(self, lib.GrB_Matrix_nvals(n, self._matrix[0])) return n[0] @property def memory_usage(self): """Returns the memory usage of the Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> assert M.memory_usage > 0 """ n = ffi.new("size_t*") _check(self, lib.GxB_Matrix_memoryUsage(n, self._matrix[0])) return n[0] @property def T(self): """Compute transpose of the Matrix. See `Matrix.transpose`. Note: This property can be expensive, if you need the transpose more than once, consider storing this in a local variable. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> MT = M.T >>> MT.iseq(M.transpose()) True """ return self.transpose() @property def M(self): """Return the structural "mask" pattern of this matrix. See `pattern()`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 142]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|142 | 2 0 1 2 >>> print(M.M) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2 """ return self.pattern() def dup(self, clear=False): """Create an duplicate Matrix. If `clear` is true return an empty duplicate. >>> A = Matrix.sparse(types.UINT8) >>> A[1,1] = 42 >>> B = A.dup() >>> B[1,1] 42 >>> B is not A True >>> C = A.dup(True) >>> assert not C """ if clear: return self.__class__.sparse(self.type, self.nrows, self.ncols) new_mat = ffi.new("GrB_Matrix*") _check(self, lib.GrB_Matrix_dup(new_mat, self._matrix[0])) return self.__class__(new_mat, self.type) @property def hyper_switch(self): """Get the hyper_switch threshold. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> hs = A.hyper_switch >>> 0 < hs < 1 True """ switch = ffi.new("double*") _check( self, lib.GxB_Matrix_Option_get(self._matrix[0], lib.GxB_HYPER_SWITCH, switch), ) return switch[0] @hyper_switch.setter def hyper_switch(self, switch): """Set the hyper_switch threshold. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> A.hyper_switch = 0.5 >>> hs = A.hyper_switch >>> hs == 0.5 True """ switch = ffi.cast("double", switch) _check( self, lib.GxB_Matrix_Option_set(self._matrix[0], lib.GxB_HYPER_SWITCH, switch), ) @property def format(self): """Get Matrix format. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> A.format == lib.GxB_BY_ROW True """ format = ffi.new("GxB_Format_Value*") _check(self, lib.GxB_Matrix_Option_get(self._matrix[0], lib.GxB_FORMAT, format)) return format[0] @format.setter def format(self, format): """Set Matrix format. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> A.format = lib.GxB_BY_COL >>> A.format == lib.GxB_BY_COL True """ format = ffi.cast("GxB_Format_Value", format) _check(self, lib.GxB_Matrix_Option_set(self._matrix[0], lib.GxB_FORMAT, format)) @property def sparsity(self): """Get Matrix sparsity control. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> A.sparsity == lib.GxB_AUTO_SPARSITY True """ sparsity = ffi.new("int*") _check( self, lib.GxB_Matrix_Option_get( self._matrix[0], lib.GxB_SPARSITY_CONTROL, sparsity ), ) return sparsity[0] @sparsity.setter def sparsity(self, sparsity): """Set Matrix sparsity control. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> A.sparsity = lib.GxB_FULL + lib.GxB_BITMAP >>> A.sparsity == lib.GxB_FULL + lib.GxB_BITMAP """ sparsity = ffi.cast("int", sparsity) _check( self, lib.GxB_Matrix_Option_set( self._matrix[0], lib.GxB_SPARSITY_CONTROL, sparsity ), ) @property def sparsity_status(self): """Set Matrix sparsity status. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> A.sparsity_status in [1,2,4,8] True """ status = ffi.new("int*") _check( self, lib.GxB_Matrix_Option_get(self._matrix[0], lib.GxB_SPARSITY_STATUS, status), ) return status[0] def pattern(self, typ=types.BOOL, out=None): """Return the pattern of the matrix where every present value in this matrix is set to identity value for the provided type which defaults to BOOL. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 142]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|142 | 2 0 1 2 >>> P = M.pattern() >>> print(P) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2 Pre-constructed matrix can be passed as the `out` parameter: >>> C = Matrix.dense(types.BOOL, 3, 3) >>> P = M.pattern(out=C) >>> print(C) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2 """ if out is None: out = Matrix.sparse(typ, self.nrows, self.ncols) return self.apply(typ.ONE, out=out) @property def S(self): """Return the vector "structure". This is the same as calling `Matrix.pattern()` with no arguments. >>> M = Matrix.from_lists([0, 1, 2], [0, 1, 2], [1, 2, 3]) >>> assert M.S == M.pattern() """ return self.pattern() def binwrite(self, filename, comments="", opener=Path.open): # pragma: nocover """Write this matrix using custom SuiteSparse binary format.""" from suitesparse_graphblas.io import binary binary.binwrite(self._matrix, filename, comments, opener) return to_binfile = binwrite def to_lists(self): """Extract the rows, columns and values of the Matrix as 3 lists. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.to_lists() [[0, 1, 2], [1, 2, 0], [42, 314, 4224]] """ I = ffi.new("GrB_Index[%s]" % self.nvals) J = ffi.new("GrB_Index[%s]" % self.nvals) V = self.type._ffi.new(self.type._c_type + "[%s]" % self.nvals) n = ffi.new("GrB_Index*") n[0] = self.nvals _check(self, self.type._Matrix_extractTuples(I, J, V, n, self._matrix[0])) return [list(I), list(J), list(map(self.type._to_value, V))] def clear(self): """Clear the matrix. This does not change the size but removes all values. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.nvals == 3 True >>> M.clear() >>> print(M) 0 1 2 0| | 0 1| | 1 2| | 2 0 1 2 """ _check(self, lib.GrB_Matrix_clear(self._matrix[0])) def resize(self, nrows=GxB_INDEX_MAX, ncols=GxB_INDEX_MAX): """Resize the matrix. If the dimensions decrease, entries that fall outside the resized matrix are deleted. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 149]) >>> M.shape (3, 3) >>> M.resize(10, 10) >>> print(M) 0 1 2 3 4 5 6 7 8 9 0| 42 | 0 1| 314 | 1 2|149 | 2 3| | 3 4| | 4 5| | 5 6| | 6 7| | 7 8| | 8 9| | 9 0 1 2 3 4 5 6 7 8 9 """ _check(self, lib.GrB_Matrix_resize(self._matrix[0], nrows, ncols)) def transpose(self, cast=None, out=None, mask=None, accum=None, desc=None): """Return Transpose of this matrix. This function can serve multiple interesting purposes including typecasting. See the [SuiteSparse User Guide](https://raw.githubusercontent.com/DrTimothyAldenDavis/GraphBLAS/stable/Doc/GraphBLAS_UserGuide.pdf) >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|149 | 2 0 1 2 >>> MT = M.transpose() >>> print(MT) 0 1 2 0| 149| 0 1| 42 | 1 2| 314 | 2 0 1 2 >>> MT = M.transpose(cast=types.BOOL, desc=descriptor.T0) >>> print(MT) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2 >>> N = M.dup(True) >>> MT = M.transpose(desc=descriptor.T0, out=N) >>> print(MT) 0 1 2 0| 42 | 0 1| 314| 1 2|149 | 2 0 1 2 """ if out is None: new_dimensions = ( (self.nrows, self.ncols) if T0 in (desc or ()) else (self.ncols, self.nrows) ) _out = ffi.new("GrB_Matrix*") if cast is not None: typ = cast else: typ = self.type _check(self, lib.GrB_Matrix_new(_out, typ._gb_type, *new_dimensions)) out = self.__class__(_out, typ) mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_transpose(out._matrix[0], mask, accum, self._matrix[0], desc) ) return out def cast(self, cast, out=None): """Cast this matrix to the provided type. If out is not provided, a new matrix is of the cast type is created. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|149 | 2 0 1 2 >>> N = M.cast(types.FP32) >>> print(N.to_string(width=5, prec=4)) 0 1 2 0| 42.0 | 0 1| 314.0| 1 2|149.0 | 2 0 1 2 >>> N = M.cast(types.FP64) >>> print(N.to_string(width=5, prec=4)) 0 1 2 0| 42.0 | 0 1| 314.0| 1 2|149.0 | 2 0 1 2 >>> N = M.cast(types.INT64) >>> print(N.to_string(width=5, prec=4)) 0 1 2 0| 42 | 0 1| 314| 1 2| 149 | 2 0 1 2 """ if out is None and self.type == cast: return self return self.transpose(cast, out, desc=T0) def eadd( self, other, add_op=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Element-wise addition with other matrix Element-wise addition takes the set union of the patterns of A and B and applies a binary operator for all entries that appear in the set intersection of the patterns of A and B. The default operators is the `PLUS` binary operator of the output type. The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation. >>> from .gviz import draw_graph >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = list(range(len(I))) >>> A = Matrix.from_lists(I, J, V, 7, 7) >>> draw_graph(A, filename='docs/imgs/Matrix_eadd_A') <graphviz.dot.Digraph object at ...> 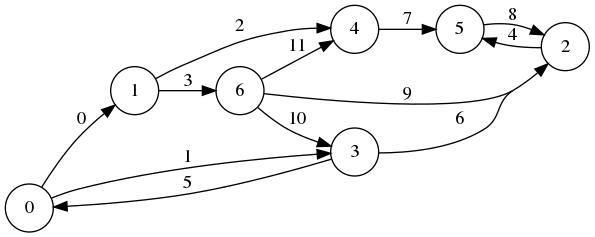 >>> B = Matrix.from_lists( ... [0, 1, 4, 6], ... [1, 3, 5, 5], ... [9, 1, 4, 7], 7, 7) >>> draw_graph(B, filename='docs/imgs/Matrix_eadd_B') <graphviz.dot.Digraph object at ...> 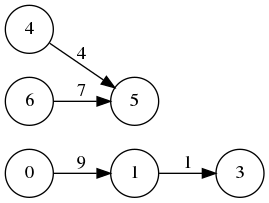 >>> draw_graph(A.eadd(B), filename='docs/imgs/Matrix_eadd_C') <graphviz.dot.Digraph object at ...> >>> print(A.eadd(B)) 0 1 2 3 4 5 6 0| 9 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 11 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 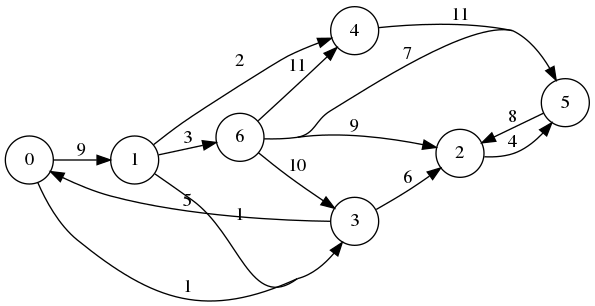 This can also be accomplished with the `+` operators: >>> print(A + B) 0 1 2 3 4 5 6 0| 9 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 11 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 The combining operator used can be provided either as a context manager or passed to `mxv` as the `add_op` argument. >>> with types.INT64.MIN: ... print(A + B) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 `eadd` is also called when a monoid is used by name as an attribute of an object: >>> print(A.min_monoid(B)) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 >>> print(A.eadd(B, A.type.min_plus)) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 The following operators default to use `eadd`: Operator | Description | Default --- | --- | --- A \\| B | Matrix Union | type default SECOND combiner A \\|= B | In-place Matrix Union | type default SECOND combiner A + B | Matrix Element-Wise Union | type default PLUS combiner A += B | In-place Matrix Element-Wise Union | type default PLUS combiner A - B | Matrix Element-Wise Union | type default MINUS combiner A -= B | In-place Matrix Element-Wise Union | type default MINUS combiner """ func = lib.GrB_Matrix_eWiseAdd_BinaryOp if add_op is None: add_op = current_binop.get(NULL) elif isinstance(add_op, Monoid): func = lib.GrB_Matrix_eWiseAdd_Monoid elif isinstance(add_op, Semiring): func = lib.GrB_Matrix_eWiseAdd_Semiring mask, accum, desc = self._get_args(mask, accum, desc) if out is None: typ = cast or types.promote(self.type, other.type) _out = ffi.new("GrB_Matrix*") _check(self, lib.GrB_Matrix_new(_out, typ._gb_type, self.nrows, self.ncols)) out = Matrix(_out, typ) if add_op is NULL: add_op = out.type._default_addop() _check( self, func( out._matrix[0], mask, accum, add_op.get_op(), self._matrix[0], other._matrix[0], desc, ), ) return out union = eadd def emult( self, other, mult_op=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Element-wise multiplication with other matrix. Element-wise multiplication applies a binary operator element-wise on two matrices A and B, for all entries that appear in the set intersection of the patterns of A and B. Other operators other than addition can be used. The pattern of the result of the element-wise multiplication is exactly this set intersection. Entries in A but not B, or visa versa, do not appear in the result. The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation. >>> from .gviz import draw_graph >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = list(range(len(I))) >>> A = Matrix.from_lists(I, J, V, 7, 7) >>> draw_graph(A, filename='docs/imgs/Matrix_emult_A') <graphviz.dot.Digraph object at ...> 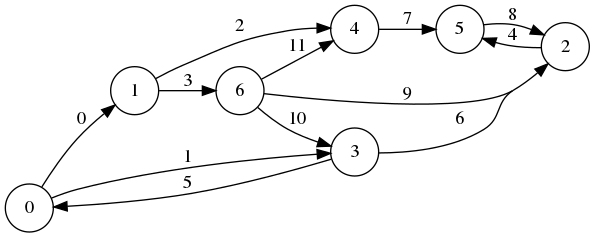 >>> B = Matrix.from_lists( ... [0, 1, 1, 6, 6], ... [1, 4, 6, 3, 5], ... [9, 1, 4, 7, 11], 7, 7) >>> draw_graph(B, filename='docs/imgs/Matrix_emult_B') <graphviz.dot.Digraph object at ...> 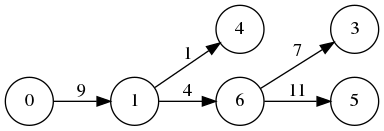 >>> draw_graph(A.emult(B), filename='docs/imgs/Matrix_emult_C') <graphviz.dot.Digraph object at ...> >>> print(A.emult(B)) 0 1 2 3 4 5 6 0| 0 | 0 1| 2 12| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 70 | 6 0 1 2 3 4 5 6 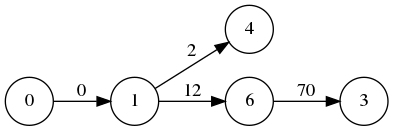 This can also be accomplished with the `+` operators: >>> print(A * B) 0 1 2 3 4 5 6 0| 0 | 0 1| 2 12| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 70 | 6 0 1 2 3 4 5 6 The combining operator used can be provided either as a context manager or passed to `mxv` as the `add_op` argument. >>> with types.INT64.MIN: ... print(A * B) 0 1 2 3 4 5 6 0| 0 | 0 1| 1 3| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 7 | 6 0 1 2 3 4 5 6 `emult` is also called when a binary operator is accessed by name as an attribute of an object: >>> print(A.min(B)) 0 1 2 3 4 5 6 0| 0 | 0 1| 1 3| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 7 | 6 0 1 2 3 4 5 6 The following operators default to using `emult`: Operator | Description | Default --- | --- | --- A & B | Matrix Intersection | type default SECOND combiner A &= B | In-place Matrix Intersection | type default SECOND combiner A * B | Matrix Element-Wise Intersection | type default TIMES combiner A *= B | In-place Matrix Element-Wise Intersection | type default TIMES combiner A / B | Matrix Element-Wise Intersection | type default DIV combiner A /= B | In-place Matrix Element-Wise Intersection | type default DIV combiner """ if mult_op is None: mult_op = current_binop.get(NULL) elif isinstance(mult_op, str): mult_op = _get_bin_op(mult_op, self.type) mask, accum, desc = self._get_args(mask, accum, desc) if out is None: typ = cast or types.promote(self.type, other.type) _out = ffi.new("GrB_Matrix*") _check(self, lib.GrB_Matrix_new(_out, typ._gb_type, self.nrows, self.ncols)) out = Matrix(_out, typ) if mult_op is NULL: mult_op = out.type._default_multop() mult_op = mult_op.get_op() _check( self, lib.GrB_Matrix_eWiseMult_BinaryOp( out._matrix[0], mask, accum, mult_op, self._matrix[0], other._matrix[0], desc, ), ) return out intersection = emult def all(self, other, op): """Do all elements in self compare True with op to other? >>> from . import INT64 >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> N = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> assert M.all(N, INT64.EQ) >>> assert not M.all(N, INT64.GT) """ if self.shape != other.shape: return False if self.nvals != other.nvals: return False C = self.emult(other, op, cast=types.BOOL) if C.nvals != self.nvals: return False return C.reduce_bool(types.BOOL.land_monoid) def iseq(self, other): """Compare two matrices for equality returning True or False. Not to be confused with `==` which will return a matrix of BOOL values comparing *elements* for equality. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> N = M.dup() >>> M.iseq(N) True >>> del N[0, 1] >>> M.iseq(N) False """ if self.type != other.type: return False return self.all(other, self.type.EQ) def isne(self, other): """Compare two matrices for inequality. See `Matrix.iseq`.""" return not self.iseq(other) def __iter__(self): """Iterate over the (row, col, value) triples of the Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> sorted(list(iter(M))) [(0, 1, 42), (1, 2, 314), (2, 0, 4224)] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = ffi.new("GrB_Index[%s]" % nvals) J = ffi.new("GrB_Index[%s]" % nvals) X = self.type._ffi.new("%s[%s]" % (self.type._c_type, nvals)) _check(self, self.type._Matrix_extractTuples(I, J, X, _nvals, self._matrix[0])) return zip(I, J, map(self.type._to_value, X)) def to_arrays(self): """Convert Matrix to tuple of three dense [array](https:/docs.python.org/3/library/array.html) objects. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.to_arrays() (array('L', [0, 1, 2]), array('L', [1, 2, 0]), array('q', [42, 314, 4224])) """ if self.type._typecode is None: raise TypeError("This matrix has no array typecode.") nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = ffi.new("GrB_Index[%s]" % nvals) J = ffi.new("GrB_Index[%s]" % nvals) X = self.type._ffi.new("%s[%s]" % (self.type._c_type, nvals)) _check(self, self.type._Matrix_extractTuples(I, J, X, _nvals, self._matrix[0])) return array("L", I), array("L", J), array(self.type._typecode, X) @property def rows(self): """An cffi cdata array of row indexes present in the matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.rows) [0, 1, 2] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = ffi.new("GrB_Index[%s]" % nvals) J = NULL X = NULL _check(self, self.type._Matrix_extractTuples(I, J, X, _nvals, self._matrix[0])) return I @property def I(self): """Iterator over `Matrix.rows`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.I) [0, 1, 2] """ return iter(self.rows) @property def npI(self): """numpy array from `Matrix.rows`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.npI array([0, 1, 2], dtype=uint64) """ return np.frombuffer(ffi.buffer(self.rows), dtype=np.uint64) @property def cols(self): """An cdata array of column indexes present in the matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.cols) [1, 2, 0] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = NULL J = ffi.new("GrB_Index[%s]" % nvals) X = NULL _check(self, self.type._Matrix_extractTuples(I, J, X, _nvals, self._matrix[0])) return J @property def J(self): """Iterator over `Matrix.cols`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.J) [1, 2, 0] """ return iter(self.cols) @property def npJ(self): """numpy array from `Matrix.cols`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.npJ array([1, 2, 0], dtype=uint64) """ return np.frombuffer(ffi.buffer(self.cols), dtype=np.uint64) @property def vals(self): """An cdata array of values present in the matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.vals) [42, 314, 4224] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = NULL J = NULL V = self.type._ffi.new("%s[%s]" % (self.type._c_type, nvals)) _check(self, self.type._Matrix_extractTuples(I, J, V, _nvals, self._matrix[0])) return V @property def V(self): """Iterator over `Matrix.vals`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.V) [42, 314, 4224] """ return iter(self.vals) @property def npV(self): """numpy array from `Matrix.vals`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.npV array([ 42, 314, 4224]) """ return np.frombuffer(ffi.buffer(self.vals), dtype=self.type._numpy_t) def __getattr__(self, name): """Look up operators as attributes for the given object.""" try: attr = getattr(self.type, name) except AttributeError: # pragma: nocover raise AttributeError(f"Matrix has no attribute or type operator {name}") return partial(attr, self) def __len__(self): """Return the number of elements in the Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> len(M) 3 """ return self.nvals def __and__(self, other): op = current_binop.get(self.type.SECOND) return self.emult(other, op) def __iand__(self, other): op = current_binop.get(self.type.SECOND) return self.emult(other, op, out=self) def __or__(self, other): op = current_binop.get(self.type.SECOND) return self.eadd(other, op) def __ior__(self, other): op = current_binop.get(self.type.SECOND) return self.eadd(other, op, out=self) def __add__(self, other): op = current_binop.get(self.type.PLUS) if not isinstance(other, Matrix): return self.apply_second(op, other) return self.eadd(other, op) def __radd__(self, other): op = current_binop.get(self.type.PLUS) if not isinstance(other, Matrix): return self.apply_first(other, op) return other.eadd(self, op) # pragma: nocover def __iadd__(self, other): op = current_binop.get(self.type.PLUS) if not isinstance(other, Matrix): return self.apply_second(op, other, out=self) return self.eadd(other, op, out=self) def __sub__(self, other): op = current_binop.get(self.type.MINUS) if not isinstance(other, Matrix): return self.apply_second(op, other) return self.eadd(other, op) def __rsub__(self, other): op = current_binop.get(self.type.MINUS) if not isinstance(other, Matrix): return self.apply_first(other, op) return other.eadd(self, op) # pragma: nocover def __isub__(self, other): op = current_binop.get(self.type.MINUS) if not isinstance(other, Matrix): return self.apply_second(op, other, out=self) return other.eadd(self, op, out=self) def __mul__(self, other): op = current_binop.get(self.type.TIMES) if not isinstance(other, Matrix): return self.apply_second(op, other) return self.emult(other, op) def __rmul__(self, other): op = current_binop.get(self.type.TIMES) if not isinstance(other, Matrix): return self.apply_first(other, op) return other.emult(self, op) # pragma: nocover def __imul__(self, other): op = current_binop.get(self.type.TIMES) if not isinstance(other, Matrix): return self.apply_second(op, other) return other.emult(self, op, out=self) def __truediv__(self, other): op = current_binop.get(self.type.DIV) if not isinstance(other, Matrix): return self.apply_second(op, other) return self.emult(other, op) def __rtruediv__(self, other): op = current_binop.get(self.type.DIV) if not isinstance(other, Matrix): return self.apply_first(other, op) return other.emult(self, op) # pragma: nocover def __itruediv__(self, other): op = current_binop.get(self.type.DIV) if not isinstance(other, Matrix): return self.apply_second(op, other) return other.emult(self, op, out=self) def __invert__(self): return self.apply(self.type.MINV) def __neg__(self): return self.apply(self.type.AINV) def __abs__(self): return self.apply(self.type.ABS) def __pow__(self, exponent): if exponent == 0: return self.__class__.identity(self.type, self.nrows) if exponent == 1: return self result = self.dup() for i in range(1, exponent): result.mxm(self, out=result) return result def kronpow(self, exponent): """Do "Kronecker Power" expansion. This is useful for graph generation through expanding patterns. And it draws pretty pictures. >>> from .gviz import draw_matrix >>> initiator = Matrix.from_lists([0, 0, 1], [0, 1, 1], [0.77, 0.88, 0.99]) >>> initiator.kronpow(0).iseq(Matrix.identity(types.FP64, 2)) True >>> initiator.kronpow(1).iseq(initiator) True >>> M = initiator.kronpow(3) >>> g = draw_matrix(M, scale=40, ... filename='docs/imgs/Matrix_kronpow') 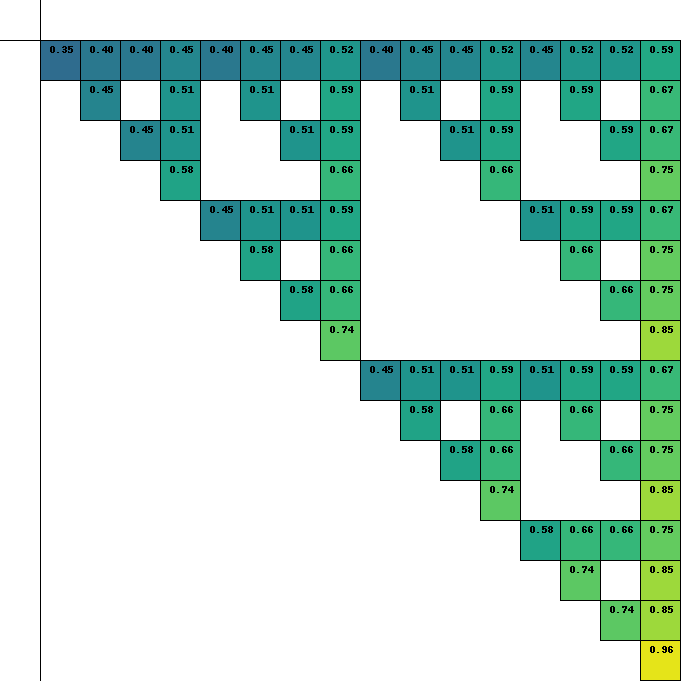 """ if exponent == 0: return self.__class__.identity(self.type, self.nrows) if exponent == 1: return self result = self.dup() for i in range(1, exponent): result = result.kronecker(result) return result def reduce_bool(self, mon=None, mask=None, accum=None, desc=None): """Reduce matrix to a boolean. >>> M = Matrix.sparse(types.INT8) >>> M.reduce_bool() False >>> M[0,1] = True >>> M.reduce_bool() True >>> M.reduce_bool(types.BOOL.LOR_MONOID) True """ if mon is None: mon = current_monoid.get(types.BOOL.LOR_MONOID) mon = mon.get_op() result = ffi.new("_Bool*") mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_reduce_BOOL(result, accum, mon, self._matrix[0], desc) ) return result[0] def reduce_int(self, mon=None, mask=None, accum=None, desc=None): """Reduce matrix to an integer. >>> M = Matrix.sparse(types.INT8) >>> M.reduce_int() 0 >>> M[0,1] = 42 >>> M[0,2] = 42 >>> M.reduce_int() 84 >>> M.reduce_int(types.INT8.MIN_MONOID) 42 """ if mon is None: mon = current_monoid.get(types.INT64.PLUS_MONOID) mon = mon.get_op() result = ffi.new("int64_t*") mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_reduce_INT64(result, accum, mon, self._matrix[0], desc) ) return result[0] def reduce_float(self, mon=None, mask=None, accum=None, desc=None): """Reduce matrix to an float. >>> M = Matrix.sparse(types.FP32) >>> M.reduce_float() 0.0 >>> M[0,1] = 42.0 >>> M[0,2] = 42.0 >>> M.reduce_float() 84.0 """ if mon is None: mon = current_monoid.get(self.type.PLUS_MONOID) mon = mon.get_op() mask, accum, desc = self._get_args(mask, accum, desc) result = ffi.new("double*") _check( self, lib.GrB_Matrix_reduce_FP64(result, accum, mon, self._matrix[0], desc) ) return result[0] def reduce(self, mon=None, accum=None, desc=None): """Do a scalar reduce based on this object's type: >>> M = Matrix.random(types.UINT8, 10, 3, 3, seed=42) >>> M.reduce() 133 >>> M = Matrix.random(types.FP32, 10, 3, 3, seed=42) >>> M.reduce() 2.937098979949951 >>> M = Matrix.random(types.UINT8, 10, 3, 3, seed=42) >>> M.reduce(M.type.min_monoid) 13 >>> M = Matrix.random(types.BOOL, 10, 3, 3, seed=42) >>> M.reduce() True """ if mon is None: if self.type is types.BOOL: mon = current_monoid.get(getattr(self.type, "lor_monoid")) else: mon = current_monoid.get(getattr(self.type, "plus_monoid")) mon = mon.get_op() mask, accum, desc = self._get_args(None, accum, desc) result = ffi.new(self.type._c_type + "*") _check( self, self.type._Matrix_reduce(result, accum, mon, self._matrix[0], desc) ) return result[0] def reduce_vector( self, mon=None, out=None, cast=None, mask=None, accum=None, desc=None ): """Reduce matrix to a vector. >>> M = Matrix.sparse(types.FP32, 3, 3) >>> print(M.reduce_vector()) 0| 1| 2| >>> M[0,1] = 42.0 >>> M[0,2] = 42.0 >>> M[2,0] = -42.0 >>> print(M.reduce_vector()) 0|84.0 1| 2|-42.0 >>> print(M.reduce_vector(types.FP32.MIN_MONOID)) 0|42.0 1| 2|-42.0 >>> v = Vector.sparse(types.FP32, M.nrows) >>> print(M.reduce_vector(out=v)) 0|84.0 1| 2|-42.0 >>> M = Matrix.sparse(types.BOOL, 3, 3) >>> print(M.reduce_vector()) 0| 1| 2| >>> M[0,1] = True >>> M[0,2] = True >>> M[2,0] = True >>> print(M.reduce_vector()) 0| t 1| 2| t If there is no `out` parameter, the newly created result vector can also be cast to a different type: >>> print(M.reduce_vector(cast=types.UINT8)) 0| 2 1| 2| 1 """ if out is None: if cast is None: T = self.type else: T = cast out = Vector.sparse(T, self.nrows) if mon is None: if out.type is types.BOOL: mon = current_monoid.get(getattr(out.type, "lor_monoid")) else: mon = current_monoid.get(getattr(out.type, "plus_monoid")) mon = mon.get_op() mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_reduce_Monoid( out._vector[0], mask, accum, mon, self._matrix[0], desc ), ) return out def apply(self, op, out=None, mask=None, accum=None, desc=None): """Apply Unary op to matrix elements. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.apply(types.INT64.ABS)) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.apply(types.INT64.ABS)) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 """ if out is None: out = self.__class__.sparse(self.type, self.nrows, self.ncols) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_apply( out._matrix[0], mask, accum, op, self._matrix[0], desc ), ) return out def apply_first(self, first, op, out=None, mask=None, accum=None, desc=None): """Apply a binary operator to the entries in a matrix, binding the first input to a scalar first. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.apply_first(1, types.INT64.PLUS)) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 >>> N = Matrix.sparse(M.type, M.nrows, M.ncols) >>> print(M.apply_first(1, types.INT64.PLUS, out=N)) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 `apply_first` is also used when a `Matrix` is used "on the right" for math operations like `+-*.` with a scalar: >>> print(1 + M) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 """ if out is None: out = self.__class__.sparse(self.type, self.nrows, self.ncols) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) if isinstance(first, Scalar): f = lib.GxB_Matrix_apply_BinaryOp1st first = first._scalar[0] else: f = self.type._Matrix_apply_BinaryOp1st _check(self, f(out._matrix[0], mask, accum, op, first, self._matrix[0], desc)) return out def apply_second(self, op, second, out=None, mask=None, accum=None, desc=None): """Apply a binary operator to the entries in a matrix, binding the second input to a scalar second. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.apply_second(types.INT64.PLUS, 1)) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 `apply_second` is also used when a `Matrix` is used "on the left" for math operations like `+-*.` with a scalar: >>> print(M + 1) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 """ if out is None: out = self.__class__.sparse(self.type, self.nrows, self.ncols) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) if isinstance(second, Scalar): f = lib.GxB_Matrix_apply_BinaryOp2nd second = second._scalar[0] else: f = self.type._Matrix_apply_BinaryOp2nd _check(self, f(out._matrix[0], mask, accum, op, self._matrix[0], second, desc)) return out def select(self, op, thunk=None, out=None, mask=None, accum=None, desc=None): """Select elements that match the given select operation condition. Can be a string mapping to following operators: Operator | Library Operation | Definition --- | --- | --- `>` | lib.GxB_GT_THUNK | Select greater than 'thunk'. `<` | lib.GxB_LT_THUNK | Select less than 'thunk'. `>=` | lib.GxB_GE_THUNK | Select greater than or equal to 'thunk'. `<=` | lib.GxB_LE_THUNK | Select less than or equal to 'thunk'. `!=` | lib.GxB_NE_THUNK | Select not equal to 'thunk'. `==` | lib.GxB_EQ_THUNK | Select equal to 'thunk'. `>0` | lib.GxB_GT_ZERO | Select greater than zero. `<0` | lib.GxB_LT_ZERO | Select less than zero. `>=0` | lib.GxB_GE_ZERO | Select greater than or equal to zero. `<=0` | lib.GxB_LE_ZERO | Select less than or equal to zero. `!=0` | lib.GxB_NONZERO | Select nonzero value. `==0` | lib.GxB_EQ_ZERO | Select equal to zero. `max` | no equivalent | Select max values. `min` | no equivalent | Select min values. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.select('>', 0)) 0 1 2 0| | 0 1| | 1 2|149 | 2 0 1 2 >>> print(M.select('>=', 0)) 0 1 2 0| | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.select('<', 0)) 0 1 2 0| -42 | 0 1| | 1 2| | 2 0 1 2 >>> N = M.dup(clear=True) >>> M.select('<', 0, out=N) is N True >>> print(N) 0 1 2 0| -42 | 0 1| | 1 2| | 2 0 1 2 >>> N = M.dup(clear=True) >>> M.select('min', out=N) is N True >>> print(N) 0 1 2 0| -42 | 0 1| | 1 2| | 2 0 1 2 >>> N = M.dup(clear=True) >>> M.select('max', out=N) is N True >>> print(N) 0 1 2 0| | 0 1| | 1 2|149 | 2 0 1 2 """ if out is None: out = self.__class__.sparse(self.type, self.nrows, self.ncols) if isinstance(op, str): if op == "min": op = lib.GxB_EQ_THUNK thunk = self.reduce_float(self.type.min_monoid) elif op == "max": op = lib.GxB_EQ_THUNK thunk = self.reduce_float(self.type.max_monoid) else: op = _get_select_op(op) elif isinstance(op, SelectOp): op = op.get_op() if thunk is None: thunk = NULL if isinstance(thunk, (bool, int, float, complex)): thunk = Scalar.from_value(thunk) if isinstance(thunk, Scalar): self._keep_alives[self._matrix] = thunk thunk = thunk._scalar[0] mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GxB_Matrix_select( out._matrix[0], mask, accum, op, self._matrix[0], thunk, desc ), ) return out def tril(self, offset=None): """Select the lower triangular Matrix. The diagonal `offset` can be used to select all below any diagonal rank, positive towars the upper right coner and negative toward the lower left. >>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.tril()) 0 1 2 0| 0 | 0 1| 0 0 | 1 2| 0 0 0| 2 0 1 2 >>> print(M.tril(1)) 0 1 2 0| 0 0 | 0 1| 0 0 0| 1 2| 0 0 0| 2 0 1 2 >>> print(M.tril(-1)) 0 1 2 0| | 0 1| 0 | 1 2| 0 0 | 2 0 1 2 """ return self.select(lib.GxB_TRIL, thunk=offset) def triu(self, offset=None): """Select the upper triangular Matrix. The diagonal `offset` can be used to select all above any diagonal rank, positive towars the upper right coner and negative toward the lower left. >>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.triu()) 0 1 2 0| 0 0 0| 0 1| 0 0| 1 2| 0| 2 0 1 2 >>> print(M.triu(1)) 0 1 2 0| 0 0| 0 1| 0| 1 2| | 2 0 1 2 >>> print(M.triu(-1)) 0 1 2 0| 0 0 0| 0 1| 0 0 0| 1 2| 0 0| 2 0 1 2 """ return self.select(lib.GxB_TRIU, thunk=offset) def diag(self, offset=None): """Select the diagonal Matrix. The diagonal `offset` can be used to select any diagonal rank, positive towars the upper right coner and negative toward the lower left. >>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.diag()) 0 1 2 0| 0 | 0 1| 0 | 1 2| 0| 2 0 1 2 >>> print(M.diag(1)) 0 1 2 0| 0 | 0 1| 0| 1 2| | 2 0 1 2 >>> print(M.diag(-1)) 0 1 2 0| | 0 1| 0 | 1 2| 0 | 2 0 1 2 """ return self.select(lib.GxB_DIAG, thunk=offset) def vector_diag(self, k=0, desc=None): """ GxB_Vector_diag extracts a vector v from an input matrix A, which may be rectangular. If k = 0, the main diagonal of A is extracted; k > 0 denotes diagonals above the main diagonal of A, and k < 0 denotes diagonals below the main diagonal of A. Let A have dimension m-by-n. If k is in the range 0 to n-1, then v has length min(m,n-k). If k is negative and in the range -1 to -m+1, then v has length min(m+k,n). If k is outside these ranges, v has length 0 (this is not an error). This function computes the same thing as the MATLAB statement v = diag(A,k) when A is a matrix, except that GxB_Vector_diag can also do typecasting. >>> from pygraphblas import UINT8 >>> A = Matrix.dense(UINT8, 2, 2, fill=1) >>> print(A) 0 1 0| 1 1| 0 1| 1 1| 1 0 1 >>> print(A.vector_diag()) 0| 1 1| 1 >>> print(A.vector_diag(1)) 0| 1 >>> A.vector_diag(2) <Vector(UINT8 size: 0, nvals: 0)> >>> print(A.vector_diag(-1)) 0| 1 >>> A.vector_diag(-2) <Vector(UINT8 size: 0, nvals: 0)> """ n, m = self.shape if k in range(0, n): l = min(m, n - k) elif k in range(-1, -m, -1): l = min(m + k, n) else: l = 0 v = Vector.sparse(self.type, l) _, _, desc = self._get_args(desc=desc) _check(self, lib.GxB_Vector_diag(v._vector[0], self._matrix[0], k, desc)) return v def offdiag(self, offset=None): """Select the off-diagonal Matrix. The diagonal `offset` can be used to select off any diagonal rank, positive towars the upper right coner and negative toward the lower left. >>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.offdiag()) 0 1 2 0| 0 0| 0 1| 0 0| 1 2| 0 0 | 2 0 1 2 >>> print(M.offdiag(1)) 0 1 2 0| 0 0| 0 1| 0 0 | 1 2| 0 0 0| 2 0 1 2 >>> print(M.offdiag(-1)) 0 1 2 0| 0 0 0| 0 1| 0 0| 1 2| 0 0| 2 0 1 2 """ return self.select(lib.GxB_OFFDIAG, thunk=offset) def nonzero(self): """Select the non-zero Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M.nonzero()) 0 1 2 0| 42 | 0 1| | 1 2|149 | 2 0 1 2 """ return self.select(lib.GxB_NONZERO) def _full(self): """""" B = self.__class__.sparse(self.type, self.nrows, self.ncols) _check( self, self.type._Matrix_assignScalar( B._matrix[0], NULL, NULL, self.type.default_one, lib.GrB_ALL, 0, lib.GrB_ALL, 0, NULL, ), ) return self.eadd(B, self.type.FIRST) def _compare(self, other, op, strop): C = self.__class__.sparse(types.BOOL, self.nrows, self.ncols) if isinstance(other, (bool, int, float, complex)): if op(other, 0): B = self.__class__.dup(self) B[:, :] = other self.emult(B, strop, out=C) return C else: self.select(strop, other).apply(types.BOOL.ONE, out=C) return C elif isinstance(other, Matrix): A = self._full() B = other._full() A.emult(B, strop, out=C) return C else: raise TypeError("Unknown matrix comparison type.") def __gt__(self, other): return self._compare(other, operator.gt, ">") def __lt__(self, other): return self._compare(other, operator.lt, "<") def __ge__(self, other): return self._compare(other, operator.ge, ">=") def __le__(self, other): return self._compare(other, operator.le, "<=") def __eq__(self, other): return self._compare(other, operator.eq, "==") def __ne__(self, other): return self._compare(other, operator.ne, "!=") def _get_args(self, mask=None, accum=None, desc=None): # if mask is not None and desc is None: # desc = S if isinstance(mask, Matrix): mask = mask._matrix[0] elif isinstance(mask, Vector): mask = mask._vector[0] else: mask = NULL if accum is None: accum = current_accum.get(NULL) if accum is not NULL: accum = accum.get_op() if desc is None: desc = current_desc.get(NULL) if desc is not NULL: desc = desc.get_desc() return mask, accum, desc def mxm( self, other, semiring=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Matrix-matrix multiply. Multiply this matrix by `other` matrix. See Section 9.6 in the [SuiteSparse User Guide](https://raw.githubusercontent.com/DrTimothyAldenDavis/GraphBLAS/stable/Doc/GraphBLAS_UserGuide.pdf) for details. `mxm` can be called directly or with the `@` operator: >>> m = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> n = Matrix.from_lists([0, 1, 2], [1, 2, 0], [2, 3, 4]) >>> print(m) 0 1 2 0| 1 | 0 1| 2| 1 2| 3 | 2 0 1 2 >>> print(n) 0 1 2 0| 2 | 0 1| 3| 1 2| 4 | 2 0 1 2 Matrix multiply `m` by `n`: >>> o = m.mxm(n) >>> print(o) 0 1 2 0| 3| 0 1| 8 | 1 2| 6 | 2 0 1 2 Matrix matrix with the `@` operator: >>> o = m @ n >>> print(o) 0 1 2 0| 3| 0 1| 8 | 1 2| 6 | 2 0 1 2 By default, `mxm` and `@` create a new result matrix of the correct type and dimensions if one is not provided. If you want to provide your own matrix to put the result in, you can pass it in the `out` parameter. This is useful for accumulating results into a single matrix with minimal copying. This is also supported by the `@=` syntax: >>> o = m.dup() >>> o.mxm(n, accum=types.INT64.min, out=o) is o True >>> print(o) 0 1 2 0| 1 3| 0 1| 8 2| 1 2| 3 6 | 2 0 1 2 >>> o = m.dup() >>> with Accum(types.INT64.min): ... o @= n >>> print(o) 0 1 2 0| 1 3| 0 1| 8 2| 1 2| 3 6 | 2 0 1 2 The default semiring depends on the infered result type. In the case of numbers, the default semiring is `PLUS_TIMES`. In the case of type `BOOL`, it is `BOOL.LOR_LAND`. >>> from pygraphblas import INT64 >>> o = m.mxm(n, semiring=INT64.min_plus) >>> print(o) 0 1 2 0| 4| 0 1| 6 | 1 2| 5 | 2 0 1 2 An explicit semiring can be passed to the method or provided with a context manager: >>> with INT64.min_plus: ... o = m @ n >>> print(o) 0 1 2 0| 4| 0 1| 6 | 1 2| 5 | 2 0 1 2 Or the semiring can be accessed via an attribute on the matrix: >>> o = m.min_plus(n) >>> print(o) 0 1 2 0| 4| 0 1| 6 | 1 2| 5 | 2 0 1 2 Descriptors and accumulators can also be provided as an argument or a context manager: >>> descriptor.T0 <Descriptor T0> >>> o = m.mxm(n, desc=descriptor.T0) >>> print(o) 0 1 2 0| 12 | 0 1| 2 | 1 2| 6| 2 0 1 2 >>> with descriptor.T0: ... o = m @ n >>> print(o) 0 1 2 0| 12 | 0 1| 2 | 1 2| 6| 2 0 1 2 The accumulator context manager requires an extra `Accum` helper class to distinguish it from binary ops used in `eadd` and `emult`. Output can be cast to a specific type by passing the cast parameter: >>> o = m.mxm(n, cast=types.FP32) >>> print(o) 0 1 2 0| 3.0| 0 1|8.0 | 1 2| 6.0 | 2 0 1 2 """ if semiring is None: semiring = current_semiring.get(NULL) if out is None: if cast is not None: typ = cast elif semiring is not NULL: typ = semiring.ztype else: typ = types.promote(self.type, other.type) out = self.__class__.sparse(typ, self.nrows, other.ncols) else: typ = out.type if semiring is NULL: semiring = out.type._default_semiring() semiring = semiring.get_op() mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_mxm( out._matrix[0], mask, accum, semiring, self._matrix[0], other._matrix[0], desc, ), ) return out def mxv( self, other, semiring=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Matrix-vector multiply. Multiply this matrix by `other` column vector "on the right". For row vector multiplication "on the left" see `Vector.vxm`. See Section 9.6 in the [SuiteSparse User Guide](https://raw.githubusercontent.com/DrTimothyAldenDavis/GraphBLAS/stable/Doc/GraphBLAS_UserGuide.pdf) for details. `mxv` can also be called directly or with the `@` operator: >>> from pygraphblas import INT64 >>> m = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> v = Vector.from_lists([0, 1, 2], [2, 3, 4]) >>> o = m.mxv(v) >>> print(o) 0| 3 1| 8 2| 6 >>> o = m @ v >>> print(o) 0| 3 1| 8 2| 6 By default, `mxv` and `@` create a new result matrix of the correct type and dimensions if one is not provided. If you want to provide your own matrix to put the result in, you can pass it in the `out` parameter. This is useful for accumulating results into a single matrix with minimal copying. >>> o = v.dup() >>> m.mxv(v, accum=INT64.plus, out=o) is o True >>> print(o) 0| 5 1|11 2|10 The default semiring depends on the infered result type. In the case of numbers, the default semiring is `PLUS_TIMES`. In the case of type `BOOL`, it is `BOOL.LOR_LAND`. An explicit semiring can be passed to the method or provided with a context manager: >>> o = m.mxv(v, semiring=INT64.min_plus) >>> print(o) 0| 4 1| 6 2| 5 >>> with INT64.min_plus: ... o = m @ v >>> print(o) 0| 4 1| 6 2| 5 >>> o = m.min_plus(v) >>> print(o) 0| 4 1| 6 2| 5 Descriptors and accumulators can also be provided as an argument or a context manager: >>> o = m.mxv(v, desc=descriptor.T0) >>> print(o) 0|12 1| 2 2| 6 >>> with descriptor.T0: ... o = m @ v >>> print(o) 0|12 1| 2 2| 6 >>> del o[1] >>> o = m.mxv(v, mask=o) >>> print(o) 0| 3 1| 2| 6 >>> o = m.mxv(v, cast=types.FP32) >>> print(o) 0|3.0 1|8.0 2|6.0 """ if semiring is None: semiring = current_semiring.get(NULL) if out is None: new_dimension = self.ncols if T0 in (desc or ()) else self.nrows if cast is not None: typ = cast elif semiring is not NULL: typ = semiring.ztype else: typ = types.promote(self.type, other.type) out = Vector.sparse(typ, new_dimension) else: typ = out.type if semiring is NULL: semiring = out.type._default_semiring() semiring = semiring.get_op() mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_mxv( out._vector[0], mask, accum, semiring, self._matrix[0], other._vector[0], desc, ), ) return out def __matmul__(self, other): if isinstance(other, Matrix): return self.mxm(other) elif isinstance(other, Vector): return self.mxv(other) else: raise TypeError("Right argument to @ must be Matrix or Vector.") def __imatmul__(self, other): return self.mxm(other, out=self) def kronecker( self, other, op=None, cast=None, out=None, mask=None, accum=None, desc=None ): """[Kronecker product](https://en.wikipedia.org/wiki/Kronecker_product). >>> n = Matrix.from_lists([0, 1, 2], [1, 2, 0], [2, 3, 4]) >>> m = Matrix.dense(types.UINT64, 3, 3, fill=1) >>> print(n.kronecker(m)) 0 1 2 3 4 5 6 7 8 0| 2 2 2 | 0 1| 2 2 2 | 1 2| 2 2 2 | 2 3| 3 3 3| 3 4| 3 3 3| 4 5| 3 3 3| 5 6| 4 4 4 | 6 7| 4 4 4 | 7 8| 4 4 4 | 8 0 1 2 3 4 5 6 7 8 >>> o = Matrix.sparse(types.UINT64, 9, 9) >>> m.kronecker(n, out=o) is o True >>> print(o) 0 1 2 3 4 5 6 7 8 0| 2 2 2 | 0 1| 3 3 3| 1 2| 4 4 4 | 2 3| 2 2 2 | 3 4| 3 3 3| 4 5| 4 4 4 | 5 6| 2 2 2 | 6 7| 3 3 3| 7 8| 4 4 4 | 8 0 1 2 3 4 5 6 7 8 >>> print(m.kronecker(n, op=types.UINT64.MIN)) 0 1 2 3 4 5 6 7 8 0| 1 1 1 | 0 1| 1 1 1| 1 2| 1 1 1 | 2 3| 1 1 1 | 3 4| 1 1 1| 4 5| 1 1 1 | 5 6| 1 1 1 | 6 7| 1 1 1| 7 8| 1 1 1 | 8 0 1 2 3 4 5 6 7 8 """ mask, accum, desc = self._get_args(mask, accum, desc) typ = cast or types.promote(self.type, other.type) if out is None: out = self.__class__.sparse( typ, self.nrows * other.nrows, self.ncols * other.ncols ) if op is None: op = current_binop.get(self.type.TIMES) op = op.get_op() _check( self, lib.GrB_Matrix_kronecker_BinaryOp( out._matrix[0], mask, accum, op, self._matrix[0], other._matrix[0], desc ), ) return out def extract_matrix( self, row_index=None, col_index=None, out=None, mask=None, accum=None, desc=None, ): """Extract a submatrix. `GrB_Matrix_extract` extracts a submatrix from another matrix. The input matrix may be transposed first, via the descriptor. The result type remains the same. `row_index` and `col_index` can be slice objects that default to the equivalent of GrB_ALL. Python slice objects support the SuiteSparse extensions for `GxB_RANGE`, `GxB_BACKWARDS` and `GxB_STRIDE`. See the User Guide for details. The size of `C` is `|row_index|`-by-`|col_index|`. Entries outside that sub-range are not accessed and do not take part in the computation. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M.extract_matrix()) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.extract_matrix(0, 1)) 0 0| 42| 0 0 >>> O = Matrix.sparse(types.UINT64, 1, 1) >>> M.extract_matrix(0, 1, out=O) is O True >>> print(O) 0 0| 42| 0 0 >>> print(M.extract_matrix(slice(1,2), 2)) 0 0| 0| 0 1| | 1 0 >>> print(M.extract_matrix(0, slice(0,1))) 0 1 0| 42| 0 0 1 >>> N = Matrix.from_lists([1, 2], [2, 0], [True, True]) >>> print(M[N]) 0 1 2 0| | 0 1| 0| 1 2|149 | 2 0 1 2 """ ta = T0 in (desc or ()) mask, accum, desc = self._get_args(mask, accum, desc) result_nrows = self.ncols if ta else self.nrows result_ncols = self.nrows if ta else self.ncols if isinstance(row_index, int): I, ni, isize = _build_range(slice(row_index, row_index), result_nrows - 1) else: I, ni, isize = _build_range(row_index, result_nrows - 1) if isinstance(col_index, int): J, nj, jsize = _build_range(slice(col_index, col_index), result_ncols - 1) else: J, nj, jsize = _build_range(col_index, result_ncols - 1) if isize is None: isize = result_nrows if jsize is None: jsize = result_ncols if out is None: out = self.__class__.sparse(self.type, isize, jsize) _check( self, lib.GrB_Matrix_extract( out._matrix[0], mask, accum, self._matrix[0], I, ni, J, nj, desc ), ) return out def extract_col( self, col_index, row_slice=None, out=None, mask=None, accum=None, desc=None ): """Extract a column Vector. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.extract_col(0)) 0| 1| 2|149 >>> v = Vector.sparse(types.UINT64, M.ncols) >>> M.extract_col(0, out=v) is v True >>> print(v) 0| 1| 2|149 """ stop_val = self.ncols if T0 in (desc or ()) else self.nrows if out is None: out = Vector.sparse(self.type, stop_val) mask, accum, desc = self._get_args(mask, accum, desc) I, ni, size = _build_range(row_slice, stop_val) _check( self, lib.GrB_Col_extract( out._vector[0], mask, accum, self._matrix[0], I, ni, col_index, desc ), ) return out def extract_row( self, row_index, col_slice=None, out=None, mask=None, accum=None, desc=None ): """Extract a row Vector. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.extract_row(0)) 0| 1|42 2| """ desc = desc & T0 if desc else T0 return self.extract_col( row_index, col_slice, out, desc=desc, mask=None, accum=None ) def __getitem__(self, index): if isinstance(index, int): # a[3] extract single row return self.extract_row(index, None) if isinstance(index, slice): # a[3:] extract submatrix of rows return self.extract_matrix(index, None) if isinstance(index, Matrix): return self.extract_matrix(mask=index) if not isinstance(index, (tuple, list)): raise TypeError i0 = index[0] i1 = index[1] if isinstance(i0, int) and isinstance(i1, int): # a[3,3] extract single element result = self.type._ffi.new(self.type._ptr) _check( self, self.type._Matrix_extractElement( result, self._matrix[0], index[0], index[1] ), ) return self.type._to_value(result[0]) if isinstance(i0, int) and isinstance(i1, slice): # a[3,:] extract slice of row vector return self.extract_row(i0, i1) if isinstance(i0, slice) and isinstance(i1, int): # a[:,3] extract slice of col vector return self.extract_col(i1, i0) # a[:,:] or a[[0,1,2], [3,4,5]] extract submatrix with slice or row/col indices return self.extract_matrix(i0, i1) def assign_col( self, col_index, value, row_slice=None, mask=None, accum=None, desc=None ): """Assign a vector to a column. >>> M = Matrix.sparse(types.BOOL, 3, 3) >>> M.assign_col(1, Vector.from_lists([1, 2], [True, True], 3)) >>> print(M) 0 1 2 0| | 0 1| t | 1 2| t | 2 0 1 2 """ stop_val = self.ncols if T0 in (desc or ()) else self.nrows I, ni, size = _build_range(row_slice, stop_val) mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Col_assign( self._matrix[0], mask, accum, value._vector[0], I, ni, col_index, desc ), ) def assign_row( self, row_index, value, col_slice=None, mask=None, accum=None, desc=None ): """Assign a vector to a row. >>> M = Matrix.sparse(types.BOOL, 3, 3) >>> M.assign_row(1, Vector.from_lists([1, 2], [True, True], 3)) >>> print(M) 0 1 2 0| | 0 1| t t| 1 2| | 2 0 1 2 """ stop_val = self.nrows if T0 in (desc or ()) else self.ncols I, ni, size = _build_range(col_slice, stop_val) mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Row_assign( self._matrix[0], mask, accum, value._vector[0], row_index, I, ni, desc ), ) def assign_matrix( self, value, rindex=None, cindex=None, mask=None, accum=None, desc=None ): """Assign a submatrix. Note: The name for this method `Matrix.assign_matrix()` is deprecated, use the name `Matrix.assign()` instead. >>> M = Matrix.sparse(types.BOOL, 3, 3) >>> S = Matrix.sparse(types.BOOL, 3, 3) >>> S[1,1] = True >>> M.assign_matrix(S) >>> print(M) 0 1 2 0| | 0 1| t | 1 2| | 2 0 1 2 >>> M.clear() Masked assignment with `M[key] = value` syntax can be done with if the index and value arguments are type `Matrix`: >>> M[S] = S >>> print(M) 0 1 2 0| | 0 1| t | 1 2| | 2 0 1 2 """ I, ni, isize = _build_range(rindex, self.nrows - 1) J, nj, jsize = _build_range(cindex, self.ncols - 1) isize = self.nrows jsize = self.ncols mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_assign( self._matrix[0], mask, accum, value._matrix[0], I, ni, J, nj, desc ), ) assign = assign_matrix def assign_scalar( self, value, row_slice=None, col_slice=None, mask=None, accum=None, desc=None ): """Assign a scalar `value` to the Matrix. >>> M = Matrix.sparse(types.BOOL, 3, 3) The values of `row_slice` and `col_slice` determine what elements are assigned to the Matrix. The value `None` maps to the GraphBLAS symbol `lib.GrB_ALL`, so the default behavior, with no other arguments, assigns the scalar to all elements: >>> M.assign_scalar(True) >>> print(M) 0 1 2 0| t t t| 0 1| t t t| 1 2| t t t| 2 0 1 2 >>> M.clear() This is the same as the slice syntax with a bare colon: >>> M[:,:] = True >>> print(M) 0 1 2 0| t t t| 0 1| t t t| 1 2| t t t| 2 0 1 2 >>> M.clear() If `row_slice` or `col_slice` is an integer, use it as an index to one row or column: >>> M.assign_scalar(True, 1) >>> print(M) 0 1 2 0| | 0 1| t t t| 1 2| | 2 0 1 2 >>> M.clear() An integer index and a scalar does row assignment: >>> M[1] = True >>> print(M) 0 1 2 0| | 0 1| t t t| 1 2| | 2 0 1 2 >>> M.clear() this is the same as the syntax: >>> M[1,:] = True >>> print(M) 0 1 2 0| | 0 1| t t t| 1 2| | 2 0 1 2 >>> M.clear() If `col_slice` is an integer, it does column assignment: >>> M.assign_scalar(True, None, 1) >>> print(M) 0 1 2 0| t | 0 1| t | 1 2| t | 2 0 1 2 >>> M.clear() Which is the same as the syntax: >>> M[:,1] = True >>> print(M) 0 1 2 0| t | 0 1| t | 1 2| t | 2 0 1 2 >>> M.clear() Just an integer index does a row assignment: >>> M.clear() >>> M[1] = Vector.from_lists([0,1], [True, True],3) >>> print(M) 0 1 2 0| | 0 1| t t | 1 2| | 2 0 1 2 >>> M.clear() >>> M[0:1,0:1] = True >>> print(M) 0 1 2 0| t t | 0 1| t t | 1 2| | 2 0 1 2 >>> M.clear() """ mask, accum, desc = self._get_args(mask, accum, desc) if row_slice is not None: if isinstance(row_slice, int): I, ni, isize = _build_range(slice(row_slice, row_slice), self.nrows - 1) else: I, ni, isize = _build_range(row_slice, self.nrows - 1) else: I = lib.GrB_ALL ni = 0 if col_slice is not None: if isinstance(col_slice, int): J, nj, jsize = _build_range(slice(col_slice, col_slice), self.ncols - 1) else: J, nj, jsize = _build_range(col_slice, self.ncols - 1) else: J = lib.GrB_ALL nj = 0 scalar_type = types._gb_from_type(type(value)) _check( self, scalar_type._Matrix_assignScalar( self._matrix[0], mask, accum, value, I, ni, J, nj, desc ), ) def __setitem__(self, index, value): if isinstance(index, int): # A[3] if isinstance(value, Vector): # A[3] = Vector return self.assign_row(index, value) if isinstance(value, (bool, int, float, complex)): # A[3] = scalar return self.assign_scalar(value, index) raise TypeError elif isinstance(index, slice): if isinstance(value, Matrix): # A[3:] = assign submatrix to rows self.assign_matrix(value, index, None) return if isinstance(value, (bool, int, float, complex)): # A[3:] = 3 assign scalar to rows self.assign_scalar(value, index, None) return raise TypeError elif isinstance(index, Matrix): if isinstance(value, Matrix): # A[M] = B masked matrix assignment self.assign_matrix(value, mask=index) return if not isinstance(value, (bool, int, float, complex)): raise TypeError # A[M] = s masked scalar assignment self.assign_scalar(value, mask=index) return elif not isinstance(index, (tuple, list)): raise TypeError i0 = index[0] i1 = index[1] if isinstance(i0, int) and isinstance(i1, int): val = self.type._from_value(value) _check(self, self.type._Matrix_setElement(self._matrix[0], val, i0, i1)) return if isinstance(i0, int) and isinstance(i1, slice): # a[3,:] assign slice of row vector or scalar if isinstance(value, Vector): self.assign_row(i0, value, i1) else: self.assign_scalar(value, i0, i1) return if isinstance(i0, slice) and isinstance(i1, int): # a[:,3] extract slice of col vector or scalar if isinstance(value, Vector): self.assign_col(i1, value, i0) else: self.assign_scalar(value, i0, i1) return if isinstance(i0, slice) and isinstance(i1, slice): if isinstance(value, (bool, int, float, complex)): self.assign_scalar(value, i0, i1) return else: # a[:,:] assign submatrix self.assign_matrix(value, i0, i1) return raise TypeError def __delitem__(self, index): if ( not isinstance(index, tuple) or not isinstance(index[0], int) or not isinstance(index[1], int) ): raise TypeError( "__delitem__ currently only supports single element removal" "assign an empty vector like `A[i] = Vector.sparse(A.type, A.nrows)`" "to remove rows or columns. " ) _check(self, lib.GrB_Matrix_removeElement(self._matrix[0], index[0], index[1])) def __contains__(self, index): try: v = self[index] return True except NoValue: return False def get(self, i, j, default=None): """Get the element at row `i` col `j` or return the default value if the element is not present. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.get(1, 2) 0 >>> M.get(0, 0) is None True >>> M.get(0, 0, 'foo') 'foo' """ try: return self[i, j] except NoValue: return default def wait(self): """Wait for this Matrix to complete before allowing another thread to change it. """ _check(self, lib.GrB_Matrix_wait(self._matrix)) def to_markdown_table(self, title="A", width=2): """Return a string markdown table representation of the Matrix. >>> M = Matrix.from_lists([0, 0, 1, 2], [1, 2, 2, 0], [42, 2, 0, 149]) >>> print(M.to_markdown_table()) A|0|1|2 ---|---|---|--- 0| |42| 2 1| | | 0 2| 149| | """ rows = set(self.rows) cols = set(self.cols) result = f"""\ {title}|{'|'.join(map(str, cols))} ---|{"|".join(['---'] * len(cols))} """ for i, row in enumerate(rows): result += f"{row}| " + "|".join( self.type.format_value(self.get(row, col, ""), width) for col in cols ) if i != len(rows) - 1: result += "\n" return result.rstrip() def to_html_table(self, title="A", width=2): """Return a string markdown table representation of the Matrix. >>> M = Matrix.from_lists([0, 0, 1, 2], [1, 2, 2, 0], [42, 2, 0, 149]) >>> print(M.to_html_table()) <table> <th>A</th> <th>0</th> <th>1</th> <th>2</th> <BLANKLINE> <tr> <th>0</th> <td> </td> <td>42</td> <td> 2</td> </tr> <BLANKLINE> <tr> <th>1</th> <td> </td> <td> </td> <td> 0</td> </tr> <BLANKLINE> <tr> <th>2</th> <td>149</td> <td> </td> <td> </td> </tr> </table> """ from mako.template import Template t = Template( """\ <% rows = set(A.rows) cols = set(A.cols) %><table> <th>${title}</th> % for col in cols: <th>${col}</th> % endfor % for row in rows: ${makerow(row)} % endfor </table><%def name="makerow(row)"> <tr> <th>${row}</th> % for col in cols: <td>${A.type.format_value(A.get(row, col, ''))}</td> % endfor </tr></%def>""" ) return t.render(A=self, title=title) def _repr_html_(self): # pragma: nocover """jupyter notebook magic render method.""" return self.to_html_table() def print(self, level=2, name="A", f=sys.stdout): # pragma: nocover """Print the matrix using `GxB_Matrix_fprint()`, by default to `sys.stdout`.. Level 1: Short description Level 2: Short list, short numbers Level 3: Long list, short number Level 4: Short list, long numbers Level 5: Long list, long numbers """ _check( self, lib.GxB_Matrix_fprint(self._matrix[0], bytes(name, "utf8"), level, f) ) def to_string( self, format_string="{:>%s}", width=3, prec=5, empty_char="", cell_sep="" ): """Return a string representation of the Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.to_string() ' 0 1 2\\n 0| 42 | 0\\n 1| 0| 1\\n 2|149 | 2\\n 0 1 2' """ format_string = format_string % width header = ( format_string.format("") + " " + "".join(format_string.format(i) for i in range(self.ncols)) ) result = header + "\n" for row in range(self.nrows): result += format_string.format(row) + "|" for col in range(self.ncols): value = self.get(row, col, empty_char) result += cell_sep + self.type.format_value(value, width, prec) result += "| " + str(row) + "\n" result += header return result def __str__(self): return self.to_string() def __repr__(self): tname = self.type.__name__ if self.nrows == lib.GxB_INDEX_MAX and self.ncols == lib.GxB_INDEX_MAX: return f"<Matrix({tname}, nvals: {self.nvals})>" return f"<Matrix({tname}, shape: {self.shape}, nvals: {self.nvals})>" @classmethod def from_scipy_sparse(cls, m): """ GrB_Type is inferred from m.dtype. >>> A = Matrix.from_lists([0, 1, 2], [1, 1, 2], [1, 2, 3]) >>> s = A.to_scipy_sparse() >>> B = Matrix.from_scipy_sparse(s) >>> assert A.iseq(B) """ ss = m.tocoo() nrows, ncols = ss.shape typ = types.Type._dtype_gb_map[m.dtype.type] return cls.from_lists( [i.item() for i in ss.row], [j.item() for j in ss.col], [v.item() for v in ss.data], typ=typ, nrows=nrows, ncols=ncols, ) def to_scipy_sparse(self, format="csr"): """Return a scipy sparse matrix of this Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.to_scipy_sparse() <3x3 sparse matrix of type '<class 'numpy.int64'>'... """ from scipy import sparse rows, cols, vals = self.to_arrays() s = sparse.coo_matrix( (vals, (rows, cols)), shape=self.shape, dtype=self.type._numpy_t ) if format == "coo": return s if format not in {"bsr", "csr", "csc", "coo", "lil", "dia", "dok"}: raise TypeError(f"Invalid format: {format}") return s.asformat(format) def to_numpy(self): """Return a dense numpy matrix of this Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.to_numpy() array([[ 0, 42, 0], [ 0, 0, 0], [149, 0, 0]], dtype=int64) """ s = self.to_scipy_sparse("coo") return s.toarray() def out_degree(self, typ=types.UINT64, out=None): """Return a UINT64 vector of the out-degree of this graph: >>> M = Matrix.from_lists([0, 1, 0, 2], [1, 2, 2, 0], [42, 0, 3, 149]) >>> print(M.out_degree()) 0| 2 1| 1 2| 1 """ return self.cast(typ).plus_pair(Vector.iso(1, self.nrows), out=out) def gini(self, typ=types.FP64): """Calculate the Gini coefficient of the graph. >>> M = Matrix.random(types.UINT8, 10, 10, 10, seed=42) >>> M.gini() 0.23333333333333334 >>> M = Matrix.random(types.UINT8, 100, 10, 10, seed=42) >>> M.gini() 0.0967741935483871 >>> M = Matrix.random(types.UINT8, 10000, 100, 100, seed=42) >>> M.gini() 0.0483808618504436 >>> M = Matrix.dense(types.UINT8, 100, 100) >>> M.gini() 0.0 """ array = self.out_degree(typ).npV array.sort() n = array.shape[0] index = np.arange(1, n + 1) return (np.sum((2 * index - n - 1) * array)) / (n * np.sum(array))
Static methods
def sparse(typ, nrows=None, ncols=None, fill=None, mask=None)
-
Create an empty sparse Matrix from the given type. The dimensions can be specified with
nrows
andncols
. If no dimensions are specified, they default toGxB_INDEX_MAX
.>>> m = Matrix.sparse(types.UINT8) >>> m.nrows == lib.GxB_INDEX_MAX True >>> m.ncols == lib.GxB_INDEX_MAX True >>> m.nvals == 0 True
Optional row and column dimension bounds can be provided to the method:
>>> m = Matrix.sparse(types.UINT8, 10, 10) >>> m.nrows == 10 True >>> m.ncols == 10 True >>> m.nvals == 0 True
One of the Python types
(bool, int, float, complex)
can be passed instead. They are turned into(BOOL, INT64, FP64, FC64)
respectively:>>> Matrix.sparse(int) <Matrix(INT64, nvals: 0)>
A sparse matrix can be "filled" with a starting value using the
fill
parameter and themask
parameter. Themask
is required otherwisefill
is ignored.>>> mask = Matrix.sparse(types.BOOL) >>> mask[1,1] = True >>> list(Matrix.sparse(float, fill=3.14, mask=mask)) [(1, 1, 3.14)]
If
mask
is provided but nofill
, thetype.default_zero
value is used:>>> list(Matrix.sparse(float, mask=mask)) [(1, 1, 0.0)]
Expand source code
@classmethod def sparse(cls, typ, nrows=None, ncols=None, fill=None, mask=None): """Create an empty sparse Matrix from the given type. The dimensions can be specified with `nrows` and `ncols`. If no dimensions are specified, they default to `GxB_INDEX_MAX`. >>> m = Matrix.sparse(types.UINT8) >>> m.nrows == lib.GxB_INDEX_MAX True >>> m.ncols == lib.GxB_INDEX_MAX True >>> m.nvals == 0 True Optional row and column dimension bounds can be provided to the method: >>> m = Matrix.sparse(types.UINT8, 10, 10) >>> m.nrows == 10 True >>> m.ncols == 10 True >>> m.nvals == 0 True One of the Python types `(bool, int, float, complex)` can be passed instead. They are turned into `(BOOL, INT64, FP64, FC64)` respectively: >>> Matrix.sparse(int) <Matrix(INT64, nvals: 0)> A sparse matrix can be "filled" with a starting value using the `fill` parameter and the `mask` parameter. The `mask` is required otherwise `fill` is ignored. >>> mask = Matrix.sparse(types.BOOL) >>> mask[1,1] = True >>> list(Matrix.sparse(float, fill=3.14, mask=mask)) [(1, 1, 3.14)] If `mask` is provided but no `fill`, the `type.default_zero` value is used: >>> list(Matrix.sparse(float, mask=mask)) [(1, 1, 0.0)] """ if nrows is None: nrows = lib.GxB_INDEX_MAX if ncols is None: ncols = lib.GxB_INDEX_MAX new_mat = ffi.new("GrB_Matrix*") if not issubclass(typ, types.Type): typ = types._gb_from_type(typ) _base_check(lib.GrB_Matrix_new(new_mat, typ._gb_type, nrows, ncols)) m = cls(new_mat, typ) if mask is not None: if fill is None: fill = m.type.default_zero m.assign_scalar(fill, mask=mask) return m
def dense(typ, nrows=None, ncols=None, fill=None, sparsity=None)
-
Return a dense Matrix nrows by ncols.
If
sparsity
is provided it is used for the sparsity of the new matrix See the SuiteSparse User Guide for details.>>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M) 0 1 2 0| 0 0 0| 0 1| 0 0 0| 1 2| 0 0 0| 2 0 1 2
If a
fill
value is present, use that, otherwise use theself.type.default_zero
attribute of the given type.>>> M = Matrix.dense(types.UINT8, 3, 3, fill=1) >>> print(M) 0 1 2 0| 1 1 1| 0 1| 1 1 1| 1 2| 1 1 1| 2 0 1 2
A dense matrix can be the maximum possible dimension, in which case it is an "iso" valued matrix.
>>> M = Matrix.dense(types.UINT8) >>> M.nrows == lib.GxB_INDEX_MAX True >>> M[42,42] 0
Expand source code
@classmethod def dense(cls, typ, nrows=None, ncols=None, fill=None, sparsity=None): """Return a dense Matrix nrows by ncols. If `sparsity` is provided it is used for the sparsity of the new matrix See the [SuiteSparse User Guide](https://raw.githubusercontent.com/DrTimothyAldenDavis/GraphBLAS/stable/Doc/GraphBLAS_UserGuide.pdf) for details. >>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M) 0 1 2 0| 0 0 0| 0 1| 0 0 0| 1 2| 0 0 0| 2 0 1 2 If a `fill` value is present, use that, otherwise use the `self.type.default_zero` attribute of the given type. >>> M = Matrix.dense(types.UINT8, 3, 3, fill=1) >>> print(M) 0 1 2 0| 1 1 1| 0 1| 1 1 1| 1 2| 1 1 1| 2 0 1 2 A dense matrix can be the maximum possible dimension, in which case it is an "iso" valued matrix. >>> M = Matrix.dense(types.UINT8) >>> M.nrows == lib.GxB_INDEX_MAX True >>> M[42,42] 0 """ if nrows is None: nrows = lib.GxB_INDEX_MAX if ncols is None: ncols = lib.GxB_INDEX_MAX assert nrows > 0 and ncols > 0, "dense matrix must be at least 1x1" m = cls.sparse(typ, nrows, ncols) if sparsity is not None: m.sparsity = sparsity if fill is None: fill = m.type.default_zero m[:, :] = fill return m
def iso(value, nrows=None, ncols=None)
-
Build a dense "iso" matrix from a scalar value.
This is similar to
Matrix.dense()
but infers the type of the new Matrix from the provided vbalue.>>> M = Matrix.iso(3) >>> assert M[42,42] == 3
>>> M = Matrix.iso(3, 2, 2) >>> print(M) 0 1 0| 3 3| 0 1| 3 3| 1 0 1
If you change an iso matrix, it is no longer stored as an iso object, and your matrix will grow in size.
>>> M[1,1] = 2 >>> print(M) 0 1 0| 3 3| 0 1| 3 2| 1 0 1
Expand source code
@classmethod def iso(cls, value, nrows=None, ncols=None): """Build a dense "iso" matrix from a scalar value. This is similar to `Matrix.dense` but infers the type of the new Matrix from the provided vbalue. >>> M = Matrix.iso(3) >>> assert M[42,42] == 3 >>> M = Matrix.iso(3, 2, 2) >>> print(M) 0 1 0| 3 3| 0 1| 3 3| 1 0 1 If you change an iso matrix, it is no longer stored as an iso object, and your matrix will grow in size. >>> M[1,1] = 2 >>> print(M) 0 1 0| 3 3| 0 1| 3 2| 1 0 1 """ if nrows is None: nrows = lib.GxB_INDEX_MAX if ncols is None: ncols = lib.GxB_INDEX_MAX typ = types._gb_from_type(type(value)) return cls.dense(typ, nrows, ncols, value)
def from_lists(I, J, V=None, nrows=None, ncols=None, typ=None)
-
Create a new matrix from the given lists of row indices, column indices, and values. If nrows or ncols are not provided, they are computed from the max values of the provides row and column indices lists.
>>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> M = Matrix.from_lists(I, J) >>> print(M) 0 1 2 3 4 5 6 0| t t | 0 1| t t| 1 2| t | 2 3| t t | 3 4| t | 4 5| t | 5 6| t t t | 6 0 1 2 3 4 5 6 >>> from pygraphblas.gviz import draw_graph >>> draw_graph(M, filename='docs/imgs/Matrix_from_lists') <graphviz.dot.Digraph object at ...>
If the third argument is a scalar value instead of a list, it is used to construct an "iso" Matrix where all values equal that scalar.
>>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = True >>> M = Matrix.from_lists(I, J, V) >>> print(M) 0 1 2 3 4 5 6 0| t t | 0 1| t t| 1 2| t | 2 3| t t | 3 4| t | 4 5| t | 5 6| t t t | 6 0 1 2 3 4 5 6
Expand source code
@classmethod def from_lists(cls, I, J, V=None, nrows=None, ncols=None, typ=None): """Create a new matrix from the given lists of row indices, column indices, and values. If nrows or ncols are not provided, they are computed from the max values of the provides row and column indices lists. >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> M = Matrix.from_lists(I, J) >>> print(M) 0 1 2 3 4 5 6 0| t t | 0 1| t t| 1 2| t | 2 3| t t | 3 4| t | 4 5| t | 5 6| t t t | 6 0 1 2 3 4 5 6 >>> from pygraphblas.gviz import draw_graph >>> draw_graph(M, filename='docs/imgs/Matrix_from_lists') <graphviz.dot.Digraph object at ...> 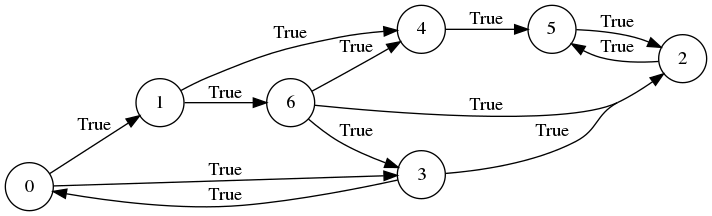 If the third argument is a scalar value instead of a list, it is used to construct an "iso" Matrix where all values equal that scalar. >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = True >>> M = Matrix.from_lists(I, J, V) >>> print(M) 0 1 2 3 4 5 6 0| t t | 0 1| t t| 1 2| t | 2 3| t t | 3 4| t | 4 5| t | 5 6| t t t | 6 0 1 2 3 4 5 6 """ assert len(I) == len(J) if V is None: V = True if isinstance(V, (bool, int, float)): V_func = lambda i: V else: V_func = lambda i: V[i] if not nrows: nrows = max(I) + 1 if not ncols: ncols = max(J) + 1 # TODO use ffi and GrB_Matrix_build if typ is None: typ = types._gb_from_type(type(V_func(0))) m = cls.sparse(typ, nrows, ncols) for v_i, (i, j) in enumerate(zip(I, J)): m[i, j] = V_func(v_i) return m
def from_diag(v, k=0, desc=None)
-
GxB_Matrix_diag constructs a matrix from a vector. Let n be the length of the v vector, from GrB_Vector_size (&n, v). If k = 0, then C is an n-by-n diagonal matrix with the entries from v along the main diagonal of C, with C(i,i) = v(i). If k is nonzero, C is square with dimension n+abs(k). If k is positive, it denotes diagonals above the main diagonal, with C(i,i+k) = v(i). If k is negative, it denotes diagonals below the main diagonal of C, with C(i-k,i) = v(i). This behavior is identical to the MATLAB statement C = diag(v,k), where v is a vector, except that GxB_Matrix_diag can also do typecasting.
>>> v = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> print(Matrix.from_diag(v)) 0 1 2 0| 1 | 0 1| 2 | 1 2| 3| 2 0 1 2 >>> print(Matrix.from_diag(v, 1)) 0 1 2 3 0| 1 | 0 1| 2 | 1 2| 3| 2 3| | 3 0 1 2 3 >>> print(Matrix.from_diag(v, -1)) 0 1 2 3 0| | 0 1| 1 | 1 2| 2 | 2 3| 3 | 3 0 1 2 3
Expand source code
@classmethod def from_diag(cls, v, k=0, desc=None): """ GxB_Matrix_diag constructs a matrix from a vector. Let n be the length of the v vector, from GrB_Vector_size (&n, v). If k = 0, then C is an n-by-n diagonal matrix with the entries from v along the main diagonal of C, with C(i,i) = v(i). If k is nonzero, C is square with dimension n+abs(k). If k is positive, it denotes diagonals above the main diagonal, with C(i,i+k) = v(i). If k is negative, it denotes diagonals below the main diagonal of C, with C(i-k,i) = v(i). This behavior is identical to the MATLAB statement C = diag(v,k), where v is a vector, except that GxB_Matrix_diag can also do typecasting. >>> v = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> print(Matrix.from_diag(v)) 0 1 2 0| 1 | 0 1| 2 | 1 2| 3| 2 0 1 2 >>> print(Matrix.from_diag(v, 1)) 0 1 2 3 0| 1 | 0 1| 2 | 1 2| 3| 2 3| | 3 0 1 2 3 >>> print(Matrix.from_diag(v, -1)) 0 1 2 3 0| | 0 1| 1 | 1 2| 2 | 2 3| 3 | 3 0 1 2 3 """ l = v.size + abs(k) C = cls.sparse(v.type, l, l) if desc is None: # pragma: nocover desc = current_desc.get(NULL) if desc is not NULL: # pragma: nocover desc = desc.get_desc() _check(C, lib.GxB_Matrix_diag(C._matrix[0], v._vector[0], k, desc)) return C
def from_mm(mm_file)
-
Create a new matrix by reading a Matrix Market file.
>>> from pathlib import Path >>> M = Matrix.from_mm(Path('docs/test_mm.mm')) >>> print(M) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 7 | 4 5| 8 | 5 6| 9 10 11 | 6 0 1 2 3 4 5 6
Expand source code
@classmethod def from_mm(cls, mm_file): """Create a new matrix by reading a Matrix Market file. >>> from pathlib import Path >>> M = Matrix.from_mm(Path('docs/test_mm.mm')) >>> print(M) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 7 | 4 5| 8 | 5 6| 9 10 11 | 6 0 1 2 3 4 5 6 """ from mmparse import mmread, get_mm_type_converter with mmread(mm_file) as f: header, row_iter = f mm_type = header["mm_type"] nrows = header["nrows"] ncols = header["ncols"] symmetric = header["mm_storage"] == "symmetric" typ = get_mm_type_converter(mm_type) m = cls.sparse(typ, nrows, ncols) for l, i, j, v in row_iter: m[i, j] = v if symmetric: m[j, i] = v return m
def from_tsv(tsv_file, typ, nrows, ncols, **kwargs)
-
Create a new matrix by reading a tab separated value file.
>>> M = Matrix.from_tsv(Path('docs/test_tsvfile.tsv'), types.INT32, 7, 7) >>> print(M) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 7 | 4 5| 8 | 5 6| 9 10 11 | 6 0 1 2 3 4 5 6
Expand source code
@classmethod def from_tsv(cls, tsv_file, typ, nrows, ncols, **kwargs): """Create a new matrix by reading a tab separated value file. >>> M = Matrix.from_tsv(Path('docs/test_tsvfile.tsv'), types.INT32, 7, 7) >>> print(M) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 7 | 4 5| 8 | 5 6| 9 10 11 | 6 0 1 2 3 4 5 6 """ kwargs["delimiter"] = "\t" return cls.from_csv(tsv_file, typ, nrows, ncols, **kwargs)
def from_csv(csv_file, typ, nrows, ncols, one_based=True, **reader_kwargs)
-
Create a new matrix by reading a comma separated value file.
kwargs to this function are passed to the underlying
csv.Reader
object, so you can control various options like quoting and alternate delimiters that way.>>> M = Matrix.from_csv(Path('docs/test_tsvfile.tsv'), types.INT32, 7, 7, delimiter='\t') >>> print(M) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 7 | 4 5| 8 | 5 6| 9 10 11 | 6 0 1 2 3 4 5 6
Expand source code
@classmethod def from_csv( cls, csv_file, typ, nrows, ncols, one_based=True, **reader_kwargs ): # pragma: nocover """Create a new matrix by reading a comma separated value file. kwargs to this function are passed to the underlying `csv.Reader` object, so you can control various options like quoting and alternate delimiters that way. >>> M = Matrix.from_csv(Path('docs/test_tsvfile.tsv'), types.INT32, 7, 7, delimiter='\\t') >>> print(M) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 7 | 4 5| 8 | 5 6| 9 10 11 | 6 0 1 2 3 4 5 6 """ import csv if typ is types.BOOL: convert = bool elif typ in ( types.INT8, types.INT16, types.INT32, types.INT64, types.UINT8, types.UINT16, types.UINT32, types.UINT64, ): convert = int elif typ in (types.FP32, types.FP64): convert = float elif typ in (types.FC32, types.FC64): convert = complex M = cls.sparse(typ, nrows, ncols) with open(csv_file, newline="") as f: reader = csv.reader(f, **reader_kwargs) for row in reader: if len(row) > 3: raise TypeError("File can contain only 3 columns: row, col and val") i, j, v = row i = int(i) j = int(j) if one_based: i = i - 1 j = j - 1 M[i, j] = convert(v) return M
def binread(bin_file, opener=<function Path.open>)
-
Create a new matrix by reading a SuiteSparse specific binary file.
Expand source code
@classmethod def binread(cls, bin_file, opener=Path.open): # pragma: nocover """Create a new matrix by reading a SuiteSparse specific binary file.""" from suitesparse_graphblas.io import binary matrix = binary.binread(bin_file, opener) return cls(matrix)
def from_binfile(bin_file, opener=<function Path.open>)
-
Create a new matrix by reading a SuiteSparse specific binary file.
Expand source code
@classmethod def binread(cls, bin_file, opener=Path.open): # pragma: nocover """Create a new matrix by reading a SuiteSparse specific binary file.""" from suitesparse_graphblas.io import binary matrix = binary.binread(bin_file, opener) return cls(matrix)
def random(typ, nvals, nrows=1152921504606846976, ncols=1152921504606846976, make_pattern=False, make_symmetric=False, make_skew_symmetric=False, make_hermitian=True, no_diagonal=False, seed=None)
-
Create a new random Matrix of the given type, number of rows, columns and values. Other flags set additional properties the matrix will hold.
>>> from .gviz import draw_graph >>> M = Matrix.random(types.UINT8, 20, 5, 5, ... make_symmetric=True, no_diagonal=True, seed=42) >>> draw_graph(M, filename='../docs/imgs/Matrix_random') <graphviz.dot.Digraph object at ...>
Expand source code
@classmethod def random( cls, typ, nvals, nrows=lib.GxB_INDEX_MAX, ncols=lib.GxB_INDEX_MAX, make_pattern=False, make_symmetric=False, make_skew_symmetric=False, make_hermitian=True, no_diagonal=False, seed=None, ): # pragma: nocover """Create a new random Matrix of the given type, number of rows, columns and values. Other flags set additional properties the matrix will hold. >>> from .gviz import draw_graph >>> M = Matrix.random(types.UINT8, 20, 5, 5, ... make_symmetric=True, no_diagonal=True, seed=42) >>> draw_graph(M, filename='../docs/imgs/Matrix_random') <graphviz.dot.Digraph object at ...> 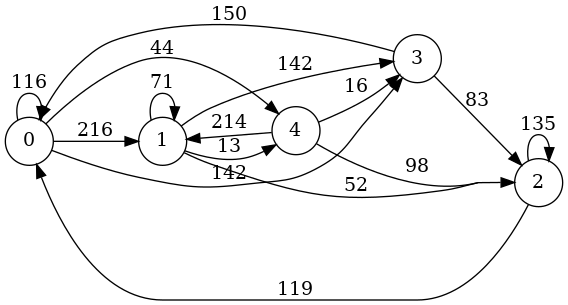 """ M = Matrix.sparse(typ, nrows, ncols) if seed is not None: random.seed(seed) if typ in (types.BOOL, types.UINT8, types.UINT16, types.UINT32, types.UINT64): make_skew_symmetric = False if M.nrows == 0 or M.ncols == 0: nvals = 0 if M.nrows != M.ncols: make_symmetric = False make_skew_symmetric = False make_hermitian = False if make_pattern or make_symmetric: make_skew_symmetric = False make_hermitian = False if make_skew_symmetric: make_hermitian = False no_diagonal = true if typ not in (types.FC32, types.FC64): make_hermitian = False if typ is types.BOOL: f = partial(random.randint, 0, 1) if typ is types.UINT8: f = partial(random.randint, 0, (2 ** 8) - 1) if typ is types.UINT16: f = partial(random.randint, 0, (2 ** 16) - 1) if typ is types.UINT32: f = partial(random.randint, 0, (2 ** 32) - 1) if typ is types.UINT64: f = partial(random.randint, 0, (2 ** 64) - 1) if typ is types.INT8: f = partial(random.randint, (-(2 ** 7)) + 1, (2 ** 7) - 1) if typ is types.INT16: f = partial(random.randint, (-(2 ** 15)) + 1, (2 ** 15) - 1) if typ is types.INT32: f = partial(random.randint, (-(2 ** 31)) + 1, (2 ** 31) - 1) if typ is types.INT64: f = partial(random.randint, (-(2 ** 63)) + 1, (2 ** 63) - 1) if typ in (types.FP32, types.FP64): f = random.random if typ in (types.FC32, types.FC64): f = lambda: complex(random.random(), random.random()) for i in range(nvals): i = random.randint(0, M.nrows - 1) j = random.randint(0, M.ncols - 1) M[i, j] = f() return M
def identity(typ, nrows, value=None)
-
Return a new square identity Matrix of nrows with diagonal set to one.
If one is None, use the default
Type.default_one
value.>>> M = Matrix.identity(types.UINT8, 3, value=42) >>> print(M) 0 1 2 0| 42 | 0 1| 42 | 1 2| 42| 2 0 1 2
Expand source code
@classmethod def identity(cls, typ, nrows, value=None): """Return a new square identity Matrix of nrows with diagonal set to one. If one is None, use the default `Type.default_one` value. >>> M = Matrix.identity(types.UINT8, 3, value=42) >>> print(M) 0 1 2 0| 42 | 0 1| 42 | 1 2| 42| 2 0 1 2 """ result = cls.sparse(typ, nrows, nrows) if value is None: value = result.type.default_one for i in range(nrows): result[i, i] = value return result
def ssget(name_or_id=None, binary_cache_dir=None)
-
Load a matrix from the SuiteSparse Matrix Market.
See the ssgetpy library for search argument:
>>> from pprint import pprint >>> from operator import itemgetter >>> pprint(sorted(list(Matrix.ssget('Newman/karate')), key=itemgetter(0))) [('karate.mtx', <Matrix(BOOL, shape: (34, 34), nvals: 156)>)]
Expand source code
@classmethod def ssget(cls, name_or_id=None, binary_cache_dir=None): # pragma: nocover """Load a matrix from the [SuiteSparse Matrix Market](https://sparse.tamu.edu/). See [the ssgetpy library](https://github.com/drdarshan/ssgetpy) for search argument: >>> from pprint import pprint >>> from operator import itemgetter >>> pprint(sorted(list(Matrix.ssget('Newman/karate')), key=itemgetter(0))) [('karate.mtx', <Matrix(BOOL, shape: (34, 34), nvals: 156)>)] """ import ssgetpy results = [] result = ssgetpy.search(name_or_id)[0] mm_path, _ = result.download(extract=True) mm_path = Path(mm_path) for m in mm_path.glob("*.mtx"): Mbin = mm_path / (m.name + ".grb") if binary_cache_dir and Mbin.exists(): M = cls.from_binfile(Mbin) else: M = cls.from_mm(mm_path / m) if binary_cache_dir: M.to_binfile(Mbin) M.wait() yield m.name, M
def from_scipy_sparse(m)
-
GrB_Type is inferred from m.dtype.
>>> A = Matrix.from_lists([0, 1, 2], [1, 1, 2], [1, 2, 3]) >>> s = A.to_scipy_sparse() >>> B = Matrix.from_scipy_sparse(s) >>> assert A.iseq(B)
Expand source code
@classmethod def from_scipy_sparse(cls, m): """ GrB_Type is inferred from m.dtype. >>> A = Matrix.from_lists([0, 1, 2], [1, 1, 2], [1, 2, 3]) >>> s = A.to_scipy_sparse() >>> B = Matrix.from_scipy_sparse(s) >>> assert A.iseq(B) """ ss = m.tocoo() nrows, ncols = ss.shape typ = types.Type._dtype_gb_map[m.dtype.type] return cls.from_lists( [i.item() for i in ss.row], [j.item() for j in ss.col], [v.item() for v in ss.data], typ=typ, nrows=nrows, ncols=ncols, )
Instance Attributes
var gb_type
-
Return the GraphBLAS low-level type object of the Matrix. This is only used if interacting with the low level API.
>>> M = Matrix.sparse(types.INT8) >>> M.gb_type == lib.GrB_INT8 True
Expand source code
@property def gb_type(self): """Return the GraphBLAS low-level type object of the Matrix. This is only used if interacting with the low level API. >>> M = Matrix.sparse(types.INT8) >>> M.gb_type == lib.GrB_INT8 True """ new_type = ffi.new("GrB_Type*") _check(self, lib.GxB_Matrix_type(new_type, self._matrix[0])) return new_type[0]
var nrows
-
Return the number of Matrix rows.
>>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.nrows 3
Expand source code
@property def nrows(self): """Return the number of Matrix rows. >>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.nrows 3 """ n = ffi.new("GrB_Index*") _check(self, lib.GrB_Matrix_nrows(n, self._matrix[0])) return n[0]
var ncols
-
Return the number of Matrix columns.
>>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.ncols 3
Expand source code
@property def ncols(self): """Return the number of Matrix columns. >>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.ncols 3 """ n = ffi.new("GrB_Index*") _check(self, lib.GrB_Matrix_ncols(n, self._matrix[0])) return n[0]
var shape
-
Numpy-like description of matrix shape as 2-tuple (nrows, ncols).
>>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.shape (3, 3)
Expand source code
@property def shape(self): """Numpy-like description of matrix shape as 2-tuple (nrows, ncols). >>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.shape (3, 3) """ return (self.nrows, self.ncols)
var square
-
True if Matrix is square, else False.
>>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.square True >>> M = Matrix.sparse(types.UINT8, 3, 4) >>> M.square False
Expand source code
@property def square(self): """True if Matrix is square, else False. >>> M = Matrix.sparse(types.UINT8, 3, 3) >>> M.square True >>> M = Matrix.sparse(types.UINT8, 3, 4) >>> M.square False """ return self.nrows == self.ncols
var nvals
-
Return the number of values stored in the Matrix.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.nvals 3
Expand source code
@property def nvals(self): """Return the number of values stored in the Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.nvals 3 """ n = ffi.new("GrB_Index*") _check(self, lib.GrB_Matrix_nvals(n, self._matrix[0])) return n[0]
var memory_usage
-
Returns the memory usage of the Matrix.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> assert M.memory_usage > 0
Expand source code
@property def memory_usage(self): """Returns the memory usage of the Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> assert M.memory_usage > 0 """ n = ffi.new("size_t*") _check(self, lib.GxB_Matrix_memoryUsage(n, self._matrix[0])) return n[0]
var T
-
Compute transpose of the Matrix. See
Matrix.transpose()
.Note: This property can be expensive, if you need the transpose more than once, consider storing this in a local variable.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> MT = M.T >>> MT.iseq(M.transpose()) True
Expand source code
@property def T(self): """Compute transpose of the Matrix. See `Matrix.transpose`. Note: This property can be expensive, if you need the transpose more than once, consider storing this in a local variable. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> MT = M.T >>> MT.iseq(M.transpose()) True """ return self.transpose()
var M
-
Return the structural "mask" pattern of this matrix. See
pattern()
.>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 142]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|142 | 2 0 1 2 >>> print(M.M) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2
Expand source code
@property def M(self): """Return the structural "mask" pattern of this matrix. See `pattern()`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 142]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|142 | 2 0 1 2 >>> print(M.M) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2 """ return self.pattern()
var hyper_switch
-
Get the hyper_switch threshold. (See SuiteSparse User Guide)
>>> A = Matrix.sparse(types.UINT8) >>> hs = A.hyper_switch >>> 0 < hs < 1 True
Expand source code
@property def hyper_switch(self): """Get the hyper_switch threshold. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> hs = A.hyper_switch >>> 0 < hs < 1 True """ switch = ffi.new("double*") _check( self, lib.GxB_Matrix_Option_get(self._matrix[0], lib.GxB_HYPER_SWITCH, switch), ) return switch[0]
var format
-
Get Matrix format. (See SuiteSparse User Guide)
>>> A = Matrix.sparse(types.UINT8) >>> A.format == lib.GxB_BY_ROW True
Expand source code
@property def format(self): """Get Matrix format. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> A.format == lib.GxB_BY_ROW True """ format = ffi.new("GxB_Format_Value*") _check(self, lib.GxB_Matrix_Option_get(self._matrix[0], lib.GxB_FORMAT, format)) return format[0]
var sparsity
-
Get Matrix sparsity control. (See SuiteSparse User Guide)
>>> A = Matrix.sparse(types.UINT8) >>> A.sparsity == lib.GxB_AUTO_SPARSITY True
Expand source code
@property def sparsity(self): """Get Matrix sparsity control. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> A.sparsity == lib.GxB_AUTO_SPARSITY True """ sparsity = ffi.new("int*") _check( self, lib.GxB_Matrix_Option_get( self._matrix[0], lib.GxB_SPARSITY_CONTROL, sparsity ), ) return sparsity[0]
var sparsity_status
-
Set Matrix sparsity status. (See SuiteSparse User Guide)
>>> A = Matrix.sparse(types.UINT8) >>> A.sparsity_status in [1,2,4,8] True
Expand source code
@property def sparsity_status(self): """Set Matrix sparsity status. (See SuiteSparse User Guide) >>> A = Matrix.sparse(types.UINT8) >>> A.sparsity_status in [1,2,4,8] True """ status = ffi.new("int*") _check( self, lib.GxB_Matrix_Option_get(self._matrix[0], lib.GxB_SPARSITY_STATUS, status), ) return status[0]
var S
-
Return the vector "structure". This is the same as calling
Matrix.pattern()
with no arguments.>>> M = Matrix.from_lists([0, 1, 2], [0, 1, 2], [1, 2, 3]) >>> assert M.S == M.pattern()
Expand source code
@property def S(self): """Return the vector "structure". This is the same as calling `Matrix.pattern()` with no arguments. >>> M = Matrix.from_lists([0, 1, 2], [0, 1, 2], [1, 2, 3]) >>> assert M.S == M.pattern() """ return self.pattern()
var rows
-
An cffi cdata array of row indexes present in the matrix.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.rows) [0, 1, 2]
Expand source code
@property def rows(self): """An cffi cdata array of row indexes present in the matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.rows) [0, 1, 2] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = ffi.new("GrB_Index[%s]" % nvals) J = NULL X = NULL _check(self, self.type._Matrix_extractTuples(I, J, X, _nvals, self._matrix[0])) return I
var I
-
Iterator over
Matrix.rows
.>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.I) [0, 1, 2]
Expand source code
@property def I(self): """Iterator over `Matrix.rows`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.I) [0, 1, 2] """ return iter(self.rows)
var npI
-
numpy array from
Matrix.rows
.>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.npI array([0, 1, 2], dtype=uint64)
Expand source code
@property def npI(self): """numpy array from `Matrix.rows`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.npI array([0, 1, 2], dtype=uint64) """ return np.frombuffer(ffi.buffer(self.rows), dtype=np.uint64)
var cols
-
An cdata array of column indexes present in the matrix.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.cols) [1, 2, 0]
Expand source code
@property def cols(self): """An cdata array of column indexes present in the matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.cols) [1, 2, 0] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = NULL J = ffi.new("GrB_Index[%s]" % nvals) X = NULL _check(self, self.type._Matrix_extractTuples(I, J, X, _nvals, self._matrix[0])) return J
var J
-
Iterator over
Matrix.cols
.>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.J) [1, 2, 0]
Expand source code
@property def J(self): """Iterator over `Matrix.cols`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.J) [1, 2, 0] """ return iter(self.cols)
var npJ
-
numpy array from
Matrix.cols
.>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.npJ array([1, 2, 0], dtype=uint64)
Expand source code
@property def npJ(self): """numpy array from `Matrix.cols`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.npJ array([1, 2, 0], dtype=uint64) """ return np.frombuffer(ffi.buffer(self.cols), dtype=np.uint64)
var vals
-
An cdata array of values present in the matrix.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.vals) [42, 314, 4224]
Expand source code
@property def vals(self): """An cdata array of values present in the matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.vals) [42, 314, 4224] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = NULL J = NULL V = self.type._ffi.new("%s[%s]" % (self.type._c_type, nvals)) _check(self, self.type._Matrix_extractTuples(I, J, V, _nvals, self._matrix[0])) return V
var V
-
Iterator over
Matrix.vals
.>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.V) [42, 314, 4224]
Expand source code
@property def V(self): """Iterator over `Matrix.vals`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> list(M.V) [42, 314, 4224] """ return iter(self.vals)
var npV
-
numpy array from
Matrix.vals
.>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.npV array([ 42, 314, 4224])
Expand source code
@property def npV(self): """numpy array from `Matrix.vals`. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.npV array([ 42, 314, 4224]) """ return np.frombuffer(ffi.buffer(self.vals), dtype=self.type._numpy_t)
var type
-
The type of the Matrix.
>>> M = Matrix.sparse(types.INT8) >>> M.type == types.INT8 True
Methods
def dup(self, clear=False)
-
Create an duplicate Matrix.
If
clear
is true return an empty duplicate.>>> A = Matrix.sparse(types.UINT8) >>> A[1,1] = 42 >>> B = A.dup() >>> B[1,1] 42 >>> B is not A True >>> C = A.dup(True) >>> assert not C
Expand source code
def dup(self, clear=False): """Create an duplicate Matrix. If `clear` is true return an empty duplicate. >>> A = Matrix.sparse(types.UINT8) >>> A[1,1] = 42 >>> B = A.dup() >>> B[1,1] 42 >>> B is not A True >>> C = A.dup(True) >>> assert not C """ if clear: return self.__class__.sparse(self.type, self.nrows, self.ncols) new_mat = ffi.new("GrB_Matrix*") _check(self, lib.GrB_Matrix_dup(new_mat, self._matrix[0])) return self.__class__(new_mat, self.type)
def pattern(self, typ=pygraphblas.types.BOOL, out=None)
-
Return the pattern of the matrix where every present value in this matrix is set to identity value for the provided type which defaults to BOOL.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 142]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|142 | 2 0 1 2 >>> P = M.pattern() >>> print(P) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2
Pre-constructed matrix can be passed as the
out
parameter:>>> C = Matrix.dense(types.BOOL, 3, 3) >>> P = M.pattern(out=C) >>> print(C) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2
Expand source code
def pattern(self, typ=types.BOOL, out=None): """Return the pattern of the matrix where every present value in this matrix is set to identity value for the provided type which defaults to BOOL. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 142]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|142 | 2 0 1 2 >>> P = M.pattern() >>> print(P) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2 Pre-constructed matrix can be passed as the `out` parameter: >>> C = Matrix.dense(types.BOOL, 3, 3) >>> P = M.pattern(out=C) >>> print(C) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2 """ if out is None: out = Matrix.sparse(typ, self.nrows, self.ncols) return self.apply(typ.ONE, out=out)
def binwrite(self, filename, comments='', opener=<function Path.open>)
-
Write this matrix using custom SuiteSparse binary format.
Expand source code
def binwrite(self, filename, comments="", opener=Path.open): # pragma: nocover """Write this matrix using custom SuiteSparse binary format.""" from suitesparse_graphblas.io import binary binary.binwrite(self._matrix, filename, comments, opener) return
def to_binfile(self, filename, comments='', opener=<function Path.open>)
-
Write this matrix using custom SuiteSparse binary format.
Expand source code
def binwrite(self, filename, comments="", opener=Path.open): # pragma: nocover """Write this matrix using custom SuiteSparse binary format.""" from suitesparse_graphblas.io import binary binary.binwrite(self._matrix, filename, comments, opener) return
def to_lists(self)
-
Extract the rows, columns and values of the Matrix as 3 lists.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.to_lists() [[0, 1, 2], [1, 2, 0], [42, 314, 4224]]
Expand source code
def to_lists(self): """Extract the rows, columns and values of the Matrix as 3 lists. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.to_lists() [[0, 1, 2], [1, 2, 0], [42, 314, 4224]] """ I = ffi.new("GrB_Index[%s]" % self.nvals) J = ffi.new("GrB_Index[%s]" % self.nvals) V = self.type._ffi.new(self.type._c_type + "[%s]" % self.nvals) n = ffi.new("GrB_Index*") n[0] = self.nvals _check(self, self.type._Matrix_extractTuples(I, J, V, n, self._matrix[0])) return [list(I), list(J), list(map(self.type._to_value, V))]
def clear(self)
-
Clear the matrix. This does not change the size but removes all values.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.nvals == 3 True >>> M.clear() >>> print(M) 0 1 2 0| | 0 1| | 1 2| | 2 0 1 2
Expand source code
def clear(self): """Clear the matrix. This does not change the size but removes all values. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.nvals == 3 True >>> M.clear() >>> print(M) 0 1 2 0| | 0 1| | 1 2| | 2 0 1 2 """ _check(self, lib.GrB_Matrix_clear(self._matrix[0]))
def resize(self, nrows=1152921504606846976, ncols=1152921504606846976)
-
Resize the matrix. If the dimensions decrease, entries that fall outside the resized matrix are deleted.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 149]) >>> M.shape (3, 3) >>> M.resize(10, 10) >>> print(M) 0 1 2 3 4 5 6 7 8 9 0| 42 | 0 1| 314 | 1 2|149 | 2 3| | 3 4| | 4 5| | 5 6| | 6 7| | 7 8| | 8 9| | 9 0 1 2 3 4 5 6 7 8 9
Expand source code
def resize(self, nrows=GxB_INDEX_MAX, ncols=GxB_INDEX_MAX): """Resize the matrix. If the dimensions decrease, entries that fall outside the resized matrix are deleted. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 149]) >>> M.shape (3, 3) >>> M.resize(10, 10) >>> print(M) 0 1 2 3 4 5 6 7 8 9 0| 42 | 0 1| 314 | 1 2|149 | 2 3| | 3 4| | 4 5| | 5 6| | 6 7| | 7 8| | 8 9| | 9 0 1 2 3 4 5 6 7 8 9 """ _check(self, lib.GrB_Matrix_resize(self._matrix[0], nrows, ncols))
def transpose(self, cast=None, out=None, mask=None, accum=None, desc=None)
-
Return Transpose of this matrix.
This function can serve multiple interesting purposes including typecasting. See the SuiteSparse User Guide
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|149 | 2 0 1 2
>>> MT = M.transpose() >>> print(MT) 0 1 2 0| 149| 0 1| 42 | 1 2| 314 | 2 0 1 2
>>> MT = M.transpose(cast=types.BOOL, desc=descriptor.T0) >>> print(MT) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2
>>> N = M.dup(True) >>> MT = M.transpose(desc=descriptor.T0, out=N) >>> print(MT) 0 1 2 0| 42 | 0 1| 314| 1 2|149 | 2 0 1 2
Expand source code
def transpose(self, cast=None, out=None, mask=None, accum=None, desc=None): """Return Transpose of this matrix. This function can serve multiple interesting purposes including typecasting. See the [SuiteSparse User Guide](https://raw.githubusercontent.com/DrTimothyAldenDavis/GraphBLAS/stable/Doc/GraphBLAS_UserGuide.pdf) >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|149 | 2 0 1 2 >>> MT = M.transpose() >>> print(MT) 0 1 2 0| 149| 0 1| 42 | 1 2| 314 | 2 0 1 2 >>> MT = M.transpose(cast=types.BOOL, desc=descriptor.T0) >>> print(MT) 0 1 2 0| t | 0 1| t| 1 2| t | 2 0 1 2 >>> N = M.dup(True) >>> MT = M.transpose(desc=descriptor.T0, out=N) >>> print(MT) 0 1 2 0| 42 | 0 1| 314| 1 2|149 | 2 0 1 2 """ if out is None: new_dimensions = ( (self.nrows, self.ncols) if T0 in (desc or ()) else (self.ncols, self.nrows) ) _out = ffi.new("GrB_Matrix*") if cast is not None: typ = cast else: typ = self.type _check(self, lib.GrB_Matrix_new(_out, typ._gb_type, *new_dimensions)) out = self.__class__(_out, typ) mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_transpose(out._matrix[0], mask, accum, self._matrix[0], desc) ) return out
def cast(self, cast, out=None)
-
Cast this matrix to the provided type. If out is not provided, a new matrix is of the cast type is created.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|149 | 2 0 1 2 >>> N = M.cast(types.FP32) >>> print(N.to_string(width=5, prec=4)) 0 1 2 0| 42.0 | 0 1| 314.0| 1 2|149.0 | 2 0 1 2
>>> N = M.cast(types.FP64) >>> print(N.to_string(width=5, prec=4)) 0 1 2 0| 42.0 | 0 1| 314.0| 1 2|149.0 | 2 0 1 2
>>> N = M.cast(types.INT64) >>> print(N.to_string(width=5, prec=4)) 0 1 2 0| 42 | 0 1| 314| 1 2| 149 | 2 0 1 2
Expand source code
def cast(self, cast, out=None): """Cast this matrix to the provided type. If out is not provided, a new matrix is of the cast type is created. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 314| 1 2|149 | 2 0 1 2 >>> N = M.cast(types.FP32) >>> print(N.to_string(width=5, prec=4)) 0 1 2 0| 42.0 | 0 1| 314.0| 1 2|149.0 | 2 0 1 2 >>> N = M.cast(types.FP64) >>> print(N.to_string(width=5, prec=4)) 0 1 2 0| 42.0 | 0 1| 314.0| 1 2|149.0 | 2 0 1 2 >>> N = M.cast(types.INT64) >>> print(N.to_string(width=5, prec=4)) 0 1 2 0| 42 | 0 1| 314| 1 2| 149 | 2 0 1 2 """ if out is None and self.type == cast: return self return self.transpose(cast, out, desc=T0)
def eadd(self, other, add_op=None, cast=None, out=None, mask=None, accum=None, desc=None)
-
Element-wise addition with other matrix
Element-wise addition takes the set union of the patterns of A and B and applies a binary operator for all entries that appear in the set intersection of the patterns of A and B. The default operators is the
PLUS
binary operator of the output type.The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation.
>>> from .gviz import draw_graph >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = list(range(len(I))) >>> A = Matrix.from_lists(I, J, V, 7, 7) >>> draw_graph(A, filename='docs/imgs/Matrix_eadd_A') <graphviz.dot.Digraph object at ...>
>>> B = Matrix.from_lists( ... [0, 1, 4, 6], ... [1, 3, 5, 5], ... [9, 1, 4, 7], 7, 7) >>> draw_graph(B, filename='docs/imgs/Matrix_eadd_B') <graphviz.dot.Digraph object at ...>
>>> draw_graph(A.eadd(B), filename='docs/imgs/Matrix_eadd_C') <graphviz.dot.Digraph object at ...> >>> print(A.eadd(B)) 0 1 2 3 4 5 6 0| 9 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 11 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6
This can also be accomplished with the
+
operators:>>> print(A + B) 0 1 2 3 4 5 6 0| 9 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 11 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6
The combining operator used can be provided either as a context manager or passed to
mxv
as theadd_op
argument.>>> with types.INT64.MIN: ... print(A + B) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6
eadd
is also called when a monoid is used by name as an attribute of an object:>>> print(A.min_monoid(B)) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6
>>> print(A.eadd(B, A.type.min_plus)) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6
The following operators default to use
eadd
:Operator Description Default A | B Matrix Union type default SECOND combiner A |= B In-place Matrix Union type default SECOND combiner A + B Matrix Element-Wise Union type default PLUS combiner A += B In-place Matrix Element-Wise Union type default PLUS combiner A - B Matrix Element-Wise Union type default MINUS combiner A -= B In-place Matrix Element-Wise Union type default MINUS combiner Expand source code
def eadd( self, other, add_op=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Element-wise addition with other matrix Element-wise addition takes the set union of the patterns of A and B and applies a binary operator for all entries that appear in the set intersection of the patterns of A and B. The default operators is the `PLUS` binary operator of the output type. The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation. >>> from .gviz import draw_graph >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = list(range(len(I))) >>> A = Matrix.from_lists(I, J, V, 7, 7) >>> draw_graph(A, filename='docs/imgs/Matrix_eadd_A') <graphviz.dot.Digraph object at ...> 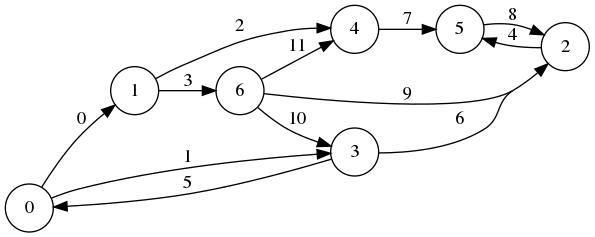 >>> B = Matrix.from_lists( ... [0, 1, 4, 6], ... [1, 3, 5, 5], ... [9, 1, 4, 7], 7, 7) >>> draw_graph(B, filename='docs/imgs/Matrix_eadd_B') <graphviz.dot.Digraph object at ...> 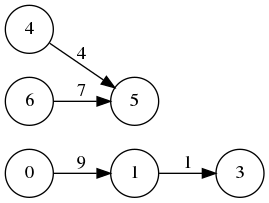 >>> draw_graph(A.eadd(B), filename='docs/imgs/Matrix_eadd_C') <graphviz.dot.Digraph object at ...> >>> print(A.eadd(B)) 0 1 2 3 4 5 6 0| 9 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 11 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 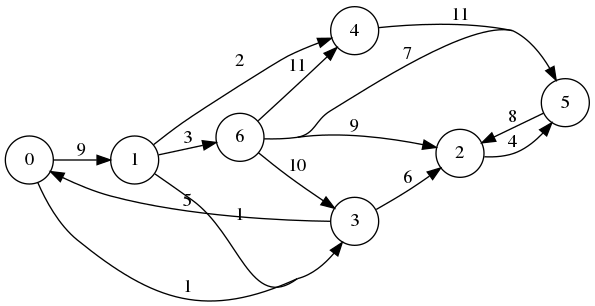 This can also be accomplished with the `+` operators: >>> print(A + B) 0 1 2 3 4 5 6 0| 9 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 11 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 The combining operator used can be provided either as a context manager or passed to `mxv` as the `add_op` argument. >>> with types.INT64.MIN: ... print(A + B) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 `eadd` is also called when a monoid is used by name as an attribute of an object: >>> print(A.min_monoid(B)) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 >>> print(A.eadd(B, A.type.min_plus)) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 The following operators default to use `eadd`: Operator | Description | Default --- | --- | --- A \\| B | Matrix Union | type default SECOND combiner A \\|= B | In-place Matrix Union | type default SECOND combiner A + B | Matrix Element-Wise Union | type default PLUS combiner A += B | In-place Matrix Element-Wise Union | type default PLUS combiner A - B | Matrix Element-Wise Union | type default MINUS combiner A -= B | In-place Matrix Element-Wise Union | type default MINUS combiner """ func = lib.GrB_Matrix_eWiseAdd_BinaryOp if add_op is None: add_op = current_binop.get(NULL) elif isinstance(add_op, Monoid): func = lib.GrB_Matrix_eWiseAdd_Monoid elif isinstance(add_op, Semiring): func = lib.GrB_Matrix_eWiseAdd_Semiring mask, accum, desc = self._get_args(mask, accum, desc) if out is None: typ = cast or types.promote(self.type, other.type) _out = ffi.new("GrB_Matrix*") _check(self, lib.GrB_Matrix_new(_out, typ._gb_type, self.nrows, self.ncols)) out = Matrix(_out, typ) if add_op is NULL: add_op = out.type._default_addop() _check( self, func( out._matrix[0], mask, accum, add_op.get_op(), self._matrix[0], other._matrix[0], desc, ), ) return out
def union(self, other, add_op=None, cast=None, out=None, mask=None, accum=None, desc=None)
-
Element-wise addition with other matrix
Element-wise addition takes the set union of the patterns of A and B and applies a binary operator for all entries that appear in the set intersection of the patterns of A and B. The default operators is the
PLUS
binary operator of the output type.The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation.
>>> from .gviz import draw_graph >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = list(range(len(I))) >>> A = Matrix.from_lists(I, J, V, 7, 7) >>> draw_graph(A, filename='docs/imgs/Matrix_eadd_A') <graphviz.dot.Digraph object at ...>
>>> B = Matrix.from_lists( ... [0, 1, 4, 6], ... [1, 3, 5, 5], ... [9, 1, 4, 7], 7, 7) >>> draw_graph(B, filename='docs/imgs/Matrix_eadd_B') <graphviz.dot.Digraph object at ...>
>>> draw_graph(A.eadd(B), filename='docs/imgs/Matrix_eadd_C') <graphviz.dot.Digraph object at ...> >>> print(A.eadd(B)) 0 1 2 3 4 5 6 0| 9 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 11 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6
This can also be accomplished with the
+
operators:>>> print(A + B) 0 1 2 3 4 5 6 0| 9 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 11 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6
The combining operator used can be provided either as a context manager or passed to
mxv
as theadd_op
argument.>>> with types.INT64.MIN: ... print(A + B) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6
eadd
is also called when a monoid is used by name as an attribute of an object:>>> print(A.min_monoid(B)) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6
>>> print(A.eadd(B, A.type.min_plus)) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6
The following operators default to use
eadd
:Operator Description Default A | B Matrix Union type default SECOND combiner A |= B In-place Matrix Union type default SECOND combiner A + B Matrix Element-Wise Union type default PLUS combiner A += B In-place Matrix Element-Wise Union type default PLUS combiner A - B Matrix Element-Wise Union type default MINUS combiner A -= B In-place Matrix Element-Wise Union type default MINUS combiner Expand source code
def eadd( self, other, add_op=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Element-wise addition with other matrix Element-wise addition takes the set union of the patterns of A and B and applies a binary operator for all entries that appear in the set intersection of the patterns of A and B. The default operators is the `PLUS` binary operator of the output type. The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation. >>> from .gviz import draw_graph >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = list(range(len(I))) >>> A = Matrix.from_lists(I, J, V, 7, 7) >>> draw_graph(A, filename='docs/imgs/Matrix_eadd_A') <graphviz.dot.Digraph object at ...> 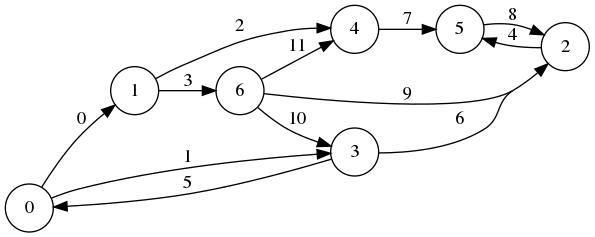 >>> B = Matrix.from_lists( ... [0, 1, 4, 6], ... [1, 3, 5, 5], ... [9, 1, 4, 7], 7, 7) >>> draw_graph(B, filename='docs/imgs/Matrix_eadd_B') <graphviz.dot.Digraph object at ...> 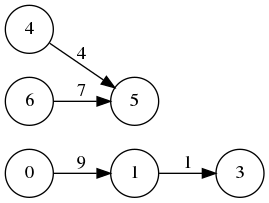 >>> draw_graph(A.eadd(B), filename='docs/imgs/Matrix_eadd_C') <graphviz.dot.Digraph object at ...> >>> print(A.eadd(B)) 0 1 2 3 4 5 6 0| 9 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 11 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 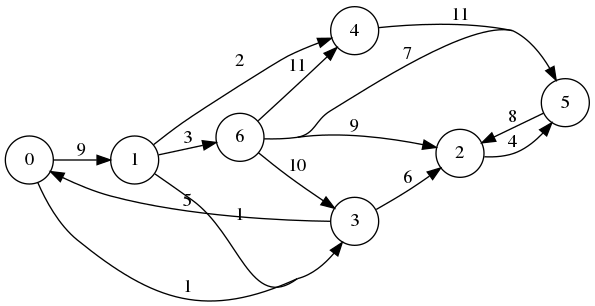 This can also be accomplished with the `+` operators: >>> print(A + B) 0 1 2 3 4 5 6 0| 9 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 11 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 The combining operator used can be provided either as a context manager or passed to `mxv` as the `add_op` argument. >>> with types.INT64.MIN: ... print(A + B) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 `eadd` is also called when a monoid is used by name as an attribute of an object: >>> print(A.min_monoid(B)) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 >>> print(A.eadd(B, A.type.min_plus)) 0 1 2 3 4 5 6 0| 0 1 | 0 1| 1 2 3| 1 2| 4 | 2 3| 5 6 | 3 4| 4 | 4 5| 8 | 5 6| 9 10 11 7 | 6 0 1 2 3 4 5 6 The following operators default to use `eadd`: Operator | Description | Default --- | --- | --- A \\| B | Matrix Union | type default SECOND combiner A \\|= B | In-place Matrix Union | type default SECOND combiner A + B | Matrix Element-Wise Union | type default PLUS combiner A += B | In-place Matrix Element-Wise Union | type default PLUS combiner A - B | Matrix Element-Wise Union | type default MINUS combiner A -= B | In-place Matrix Element-Wise Union | type default MINUS combiner """ func = lib.GrB_Matrix_eWiseAdd_BinaryOp if add_op is None: add_op = current_binop.get(NULL) elif isinstance(add_op, Monoid): func = lib.GrB_Matrix_eWiseAdd_Monoid elif isinstance(add_op, Semiring): func = lib.GrB_Matrix_eWiseAdd_Semiring mask, accum, desc = self._get_args(mask, accum, desc) if out is None: typ = cast or types.promote(self.type, other.type) _out = ffi.new("GrB_Matrix*") _check(self, lib.GrB_Matrix_new(_out, typ._gb_type, self.nrows, self.ncols)) out = Matrix(_out, typ) if add_op is NULL: add_op = out.type._default_addop() _check( self, func( out._matrix[0], mask, accum, add_op.get_op(), self._matrix[0], other._matrix[0], desc, ), ) return out
def emult(self, other, mult_op=None, cast=None, out=None, mask=None, accum=None, desc=None)
-
Element-wise multiplication with other matrix.
Element-wise multiplication applies a binary operator element-wise on two matrices A and B, for all entries that appear in the set intersection of the patterns of A and B. Other operators other than addition can be used.
The pattern of the result of the element-wise multiplication is exactly this set intersection. Entries in A but not B, or visa versa, do not appear in the result.
The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation.
>>> from .gviz import draw_graph >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = list(range(len(I))) >>> A = Matrix.from_lists(I, J, V, 7, 7) >>> draw_graph(A, filename='docs/imgs/Matrix_emult_A') <graphviz.dot.Digraph object at ...>
>>> B = Matrix.from_lists( ... [0, 1, 1, 6, 6], ... [1, 4, 6, 3, 5], ... [9, 1, 4, 7, 11], 7, 7) >>> draw_graph(B, filename='docs/imgs/Matrix_emult_B') <graphviz.dot.Digraph object at ...>
>>> draw_graph(A.emult(B), filename='docs/imgs/Matrix_emult_C') <graphviz.dot.Digraph object at ...> >>> print(A.emult(B)) 0 1 2 3 4 5 6 0| 0 | 0 1| 2 12| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 70 | 6 0 1 2 3 4 5 6
This can also be accomplished with the
+
operators:>>> print(A * B) 0 1 2 3 4 5 6 0| 0 | 0 1| 2 12| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 70 | 6 0 1 2 3 4 5 6
The combining operator used can be provided either as a context manager or passed to
mxv
as theadd_op
argument.>>> with types.INT64.MIN: ... print(A * B) 0 1 2 3 4 5 6 0| 0 | 0 1| 1 3| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 7 | 6 0 1 2 3 4 5 6
emult
is also called when a binary operator is accessed by name as an attribute of an object:>>> print(A.min(B)) 0 1 2 3 4 5 6 0| 0 | 0 1| 1 3| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 7 | 6 0 1 2 3 4 5 6
The following operators default to using
emult
:Operator Description Default A & B Matrix Intersection type default SECOND combiner A &= B In-place Matrix Intersection type default SECOND combiner A * B Matrix Element-Wise Intersection type default TIMES combiner A *= B In-place Matrix Element-Wise Intersection type default TIMES combiner A / B Matrix Element-Wise Intersection type default DIV combiner A /= B In-place Matrix Element-Wise Intersection type default DIV combiner Expand source code
def emult( self, other, mult_op=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Element-wise multiplication with other matrix. Element-wise multiplication applies a binary operator element-wise on two matrices A and B, for all entries that appear in the set intersection of the patterns of A and B. Other operators other than addition can be used. The pattern of the result of the element-wise multiplication is exactly this set intersection. Entries in A but not B, or visa versa, do not appear in the result. The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation. >>> from .gviz import draw_graph >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = list(range(len(I))) >>> A = Matrix.from_lists(I, J, V, 7, 7) >>> draw_graph(A, filename='docs/imgs/Matrix_emult_A') <graphviz.dot.Digraph object at ...> 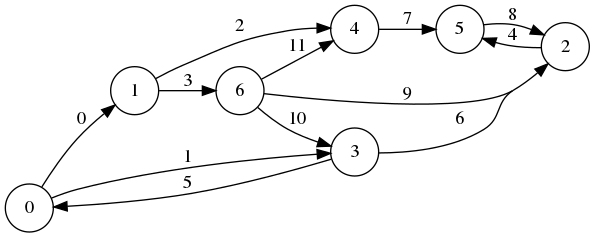 >>> B = Matrix.from_lists( ... [0, 1, 1, 6, 6], ... [1, 4, 6, 3, 5], ... [9, 1, 4, 7, 11], 7, 7) >>> draw_graph(B, filename='docs/imgs/Matrix_emult_B') <graphviz.dot.Digraph object at ...> 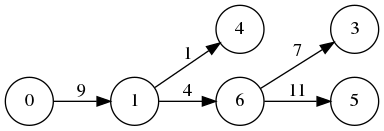 >>> draw_graph(A.emult(B), filename='docs/imgs/Matrix_emult_C') <graphviz.dot.Digraph object at ...> >>> print(A.emult(B)) 0 1 2 3 4 5 6 0| 0 | 0 1| 2 12| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 70 | 6 0 1 2 3 4 5 6 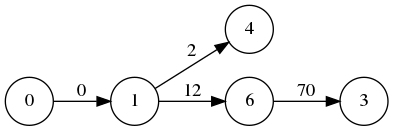 This can also be accomplished with the `+` operators: >>> print(A * B) 0 1 2 3 4 5 6 0| 0 | 0 1| 2 12| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 70 | 6 0 1 2 3 4 5 6 The combining operator used can be provided either as a context manager or passed to `mxv` as the `add_op` argument. >>> with types.INT64.MIN: ... print(A * B) 0 1 2 3 4 5 6 0| 0 | 0 1| 1 3| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 7 | 6 0 1 2 3 4 5 6 `emult` is also called when a binary operator is accessed by name as an attribute of an object: >>> print(A.min(B)) 0 1 2 3 4 5 6 0| 0 | 0 1| 1 3| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 7 | 6 0 1 2 3 4 5 6 The following operators default to using `emult`: Operator | Description | Default --- | --- | --- A & B | Matrix Intersection | type default SECOND combiner A &= B | In-place Matrix Intersection | type default SECOND combiner A * B | Matrix Element-Wise Intersection | type default TIMES combiner A *= B | In-place Matrix Element-Wise Intersection | type default TIMES combiner A / B | Matrix Element-Wise Intersection | type default DIV combiner A /= B | In-place Matrix Element-Wise Intersection | type default DIV combiner """ if mult_op is None: mult_op = current_binop.get(NULL) elif isinstance(mult_op, str): mult_op = _get_bin_op(mult_op, self.type) mask, accum, desc = self._get_args(mask, accum, desc) if out is None: typ = cast or types.promote(self.type, other.type) _out = ffi.new("GrB_Matrix*") _check(self, lib.GrB_Matrix_new(_out, typ._gb_type, self.nrows, self.ncols)) out = Matrix(_out, typ) if mult_op is NULL: mult_op = out.type._default_multop() mult_op = mult_op.get_op() _check( self, lib.GrB_Matrix_eWiseMult_BinaryOp( out._matrix[0], mask, accum, mult_op, self._matrix[0], other._matrix[0], desc, ), ) return out
def intersection(self, other, mult_op=None, cast=None, out=None, mask=None, accum=None, desc=None)
-
Element-wise multiplication with other matrix.
Element-wise multiplication applies a binary operator element-wise on two matrices A and B, for all entries that appear in the set intersection of the patterns of A and B. Other operators other than addition can be used.
The pattern of the result of the element-wise multiplication is exactly this set intersection. Entries in A but not B, or visa versa, do not appear in the result.
The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation.
>>> from .gviz import draw_graph >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = list(range(len(I))) >>> A = Matrix.from_lists(I, J, V, 7, 7) >>> draw_graph(A, filename='docs/imgs/Matrix_emult_A') <graphviz.dot.Digraph object at ...>
>>> B = Matrix.from_lists( ... [0, 1, 1, 6, 6], ... [1, 4, 6, 3, 5], ... [9, 1, 4, 7, 11], 7, 7) >>> draw_graph(B, filename='docs/imgs/Matrix_emult_B') <graphviz.dot.Digraph object at ...>
>>> draw_graph(A.emult(B), filename='docs/imgs/Matrix_emult_C') <graphviz.dot.Digraph object at ...> >>> print(A.emult(B)) 0 1 2 3 4 5 6 0| 0 | 0 1| 2 12| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 70 | 6 0 1 2 3 4 5 6
This can also be accomplished with the
+
operators:>>> print(A * B) 0 1 2 3 4 5 6 0| 0 | 0 1| 2 12| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 70 | 6 0 1 2 3 4 5 6
The combining operator used can be provided either as a context manager or passed to
mxv
as theadd_op
argument.>>> with types.INT64.MIN: ... print(A * B) 0 1 2 3 4 5 6 0| 0 | 0 1| 1 3| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 7 | 6 0 1 2 3 4 5 6
emult
is also called when a binary operator is accessed by name as an attribute of an object:>>> print(A.min(B)) 0 1 2 3 4 5 6 0| 0 | 0 1| 1 3| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 7 | 6 0 1 2 3 4 5 6
The following operators default to using
emult
:Operator Description Default A & B Matrix Intersection type default SECOND combiner A &= B In-place Matrix Intersection type default SECOND combiner A * B Matrix Element-Wise Intersection type default TIMES combiner A *= B In-place Matrix Element-Wise Intersection type default TIMES combiner A / B Matrix Element-Wise Intersection type default DIV combiner A /= B In-place Matrix Element-Wise Intersection type default DIV combiner Expand source code
def emult( self, other, mult_op=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Element-wise multiplication with other matrix. Element-wise multiplication applies a binary operator element-wise on two matrices A and B, for all entries that appear in the set intersection of the patterns of A and B. Other operators other than addition can be used. The pattern of the result of the element-wise multiplication is exactly this set intersection. Entries in A but not B, or visa versa, do not appear in the result. The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation. >>> from .gviz import draw_graph >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> J = [1, 3, 4, 6, 5, 0, 2, 5, 2, 2, 3, 4] >>> V = list(range(len(I))) >>> A = Matrix.from_lists(I, J, V, 7, 7) >>> draw_graph(A, filename='docs/imgs/Matrix_emult_A') <graphviz.dot.Digraph object at ...> 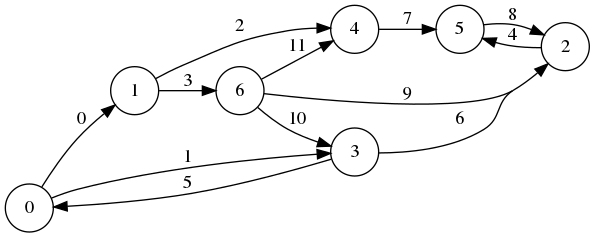 >>> B = Matrix.from_lists( ... [0, 1, 1, 6, 6], ... [1, 4, 6, 3, 5], ... [9, 1, 4, 7, 11], 7, 7) >>> draw_graph(B, filename='docs/imgs/Matrix_emult_B') <graphviz.dot.Digraph object at ...> 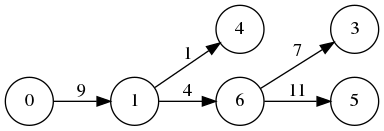 >>> draw_graph(A.emult(B), filename='docs/imgs/Matrix_emult_C') <graphviz.dot.Digraph object at ...> >>> print(A.emult(B)) 0 1 2 3 4 5 6 0| 0 | 0 1| 2 12| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 70 | 6 0 1 2 3 4 5 6 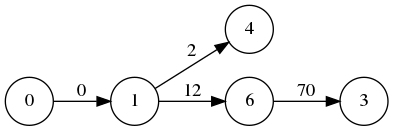 This can also be accomplished with the `+` operators: >>> print(A * B) 0 1 2 3 4 5 6 0| 0 | 0 1| 2 12| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 70 | 6 0 1 2 3 4 5 6 The combining operator used can be provided either as a context manager or passed to `mxv` as the `add_op` argument. >>> with types.INT64.MIN: ... print(A * B) 0 1 2 3 4 5 6 0| 0 | 0 1| 1 3| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 7 | 6 0 1 2 3 4 5 6 `emult` is also called when a binary operator is accessed by name as an attribute of an object: >>> print(A.min(B)) 0 1 2 3 4 5 6 0| 0 | 0 1| 1 3| 1 2| | 2 3| | 3 4| | 4 5| | 5 6| 7 | 6 0 1 2 3 4 5 6 The following operators default to using `emult`: Operator | Description | Default --- | --- | --- A & B | Matrix Intersection | type default SECOND combiner A &= B | In-place Matrix Intersection | type default SECOND combiner A * B | Matrix Element-Wise Intersection | type default TIMES combiner A *= B | In-place Matrix Element-Wise Intersection | type default TIMES combiner A / B | Matrix Element-Wise Intersection | type default DIV combiner A /= B | In-place Matrix Element-Wise Intersection | type default DIV combiner """ if mult_op is None: mult_op = current_binop.get(NULL) elif isinstance(mult_op, str): mult_op = _get_bin_op(mult_op, self.type) mask, accum, desc = self._get_args(mask, accum, desc) if out is None: typ = cast or types.promote(self.type, other.type) _out = ffi.new("GrB_Matrix*") _check(self, lib.GrB_Matrix_new(_out, typ._gb_type, self.nrows, self.ncols)) out = Matrix(_out, typ) if mult_op is NULL: mult_op = out.type._default_multop() mult_op = mult_op.get_op() _check( self, lib.GrB_Matrix_eWiseMult_BinaryOp( out._matrix[0], mask, accum, mult_op, self._matrix[0], other._matrix[0], desc, ), ) return out
def all(self, other, op)
-
Do all elements in self compare True with op to other?
>>> from . import INT64 >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> N = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> assert M.all(N, INT64.EQ) >>> assert not M.all(N, INT64.GT)
Expand source code
def all(self, other, op): """Do all elements in self compare True with op to other? >>> from . import INT64 >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> N = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> assert M.all(N, INT64.EQ) >>> assert not M.all(N, INT64.GT) """ if self.shape != other.shape: return False if self.nvals != other.nvals: return False C = self.emult(other, op, cast=types.BOOL) if C.nvals != self.nvals: return False return C.reduce_bool(types.BOOL.land_monoid)
def iseq(self, other)
-
Compare two matrices for equality returning True or False.
Not to be confused with
==
which will return a matrix of BOOL values comparing elements for equality.>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> N = M.dup() >>> M.iseq(N) True >>> del N[0, 1] >>> M.iseq(N) False
Expand source code
def iseq(self, other): """Compare two matrices for equality returning True or False. Not to be confused with `==` which will return a matrix of BOOL values comparing *elements* for equality. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> N = M.dup() >>> M.iseq(N) True >>> del N[0, 1] >>> M.iseq(N) False """ if self.type != other.type: return False return self.all(other, self.type.EQ)
def isne(self, other)
-
Compare two matrices for inequality. See
Matrix.iseq()
.Expand source code
def isne(self, other): """Compare two matrices for inequality. See `Matrix.iseq`.""" return not self.iseq(other)
def to_arrays(self)
-
Convert Matrix to tuple of three dense array objects.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.to_arrays() (array('L', [0, 1, 2]), array('L', [1, 2, 0]), array('q', [42, 314, 4224]))
Expand source code
def to_arrays(self): """Convert Matrix to tuple of three dense [array](https:/docs.python.org/3/library/array.html) objects. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 314, 4224]) >>> M.to_arrays() (array('L', [0, 1, 2]), array('L', [1, 2, 0]), array('q', [42, 314, 4224])) """ if self.type._typecode is None: raise TypeError("This matrix has no array typecode.") nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = ffi.new("GrB_Index[%s]" % nvals) J = ffi.new("GrB_Index[%s]" % nvals) X = self.type._ffi.new("%s[%s]" % (self.type._c_type, nvals)) _check(self, self.type._Matrix_extractTuples(I, J, X, _nvals, self._matrix[0])) return array("L", I), array("L", J), array(self.type._typecode, X)
def kronpow(self, exponent)
-
Do "Kronecker Power" expansion. This is useful for graph generation through expanding patterns. And it draws pretty pictures.
>>> from .gviz import draw_matrix >>> initiator = Matrix.from_lists([0, 0, 1], [0, 1, 1], [0.77, 0.88, 0.99]) >>> initiator.kronpow(0).iseq(Matrix.identity(types.FP64, 2)) True >>> initiator.kronpow(1).iseq(initiator) True >>> M = initiator.kronpow(3) >>> g = draw_matrix(M, scale=40, ... filename='docs/imgs/Matrix_kronpow')
Expand source code
def kronpow(self, exponent): """Do "Kronecker Power" expansion. This is useful for graph generation through expanding patterns. And it draws pretty pictures. >>> from .gviz import draw_matrix >>> initiator = Matrix.from_lists([0, 0, 1], [0, 1, 1], [0.77, 0.88, 0.99]) >>> initiator.kronpow(0).iseq(Matrix.identity(types.FP64, 2)) True >>> initiator.kronpow(1).iseq(initiator) True >>> M = initiator.kronpow(3) >>> g = draw_matrix(M, scale=40, ... filename='docs/imgs/Matrix_kronpow') 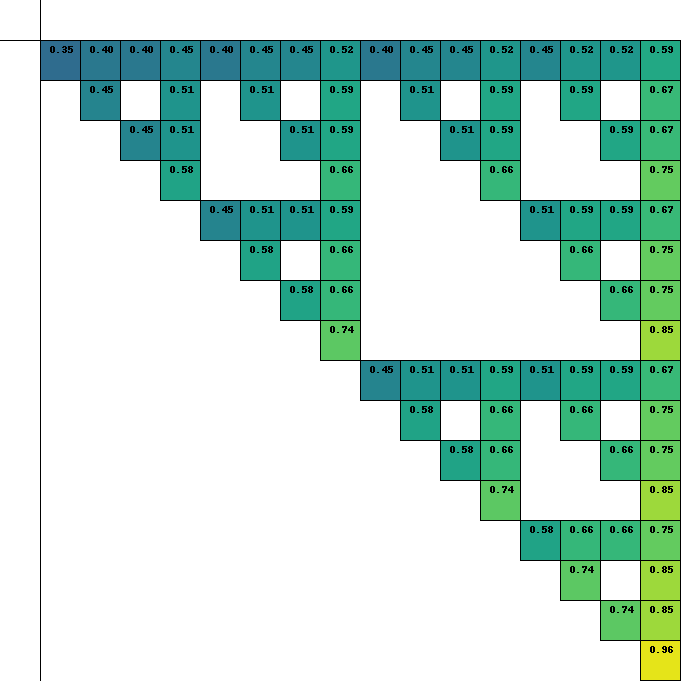 """ if exponent == 0: return self.__class__.identity(self.type, self.nrows) if exponent == 1: return self result = self.dup() for i in range(1, exponent): result = result.kronecker(result) return result
def reduce_bool(self, mon=None, mask=None, accum=None, desc=None)
-
Reduce matrix to a boolean.
>>> M = Matrix.sparse(types.INT8) >>> M.reduce_bool() False >>> M[0,1] = True >>> M.reduce_bool() True
>>> M.reduce_bool(types.BOOL.LOR_MONOID) True
Expand source code
def reduce_bool(self, mon=None, mask=None, accum=None, desc=None): """Reduce matrix to a boolean. >>> M = Matrix.sparse(types.INT8) >>> M.reduce_bool() False >>> M[0,1] = True >>> M.reduce_bool() True >>> M.reduce_bool(types.BOOL.LOR_MONOID) True """ if mon is None: mon = current_monoid.get(types.BOOL.LOR_MONOID) mon = mon.get_op() result = ffi.new("_Bool*") mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_reduce_BOOL(result, accum, mon, self._matrix[0], desc) ) return result[0]
def reduce_int(self, mon=None, mask=None, accum=None, desc=None)
-
Reduce matrix to an integer.
>>> M = Matrix.sparse(types.INT8) >>> M.reduce_int() 0 >>> M[0,1] = 42 >>> M[0,2] = 42 >>> M.reduce_int() 84 >>> M.reduce_int(types.INT8.MIN_MONOID) 42
Expand source code
def reduce_int(self, mon=None, mask=None, accum=None, desc=None): """Reduce matrix to an integer. >>> M = Matrix.sparse(types.INT8) >>> M.reduce_int() 0 >>> M[0,1] = 42 >>> M[0,2] = 42 >>> M.reduce_int() 84 >>> M.reduce_int(types.INT8.MIN_MONOID) 42 """ if mon is None: mon = current_monoid.get(types.INT64.PLUS_MONOID) mon = mon.get_op() result = ffi.new("int64_t*") mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_reduce_INT64(result, accum, mon, self._matrix[0], desc) ) return result[0]
def reduce_float(self, mon=None, mask=None, accum=None, desc=None)
-
Reduce matrix to an float.
>>> M = Matrix.sparse(types.FP32) >>> M.reduce_float() 0.0 >>> M[0,1] = 42.0 >>> M[0,2] = 42.0 >>> M.reduce_float() 84.0
Expand source code
def reduce_float(self, mon=None, mask=None, accum=None, desc=None): """Reduce matrix to an float. >>> M = Matrix.sparse(types.FP32) >>> M.reduce_float() 0.0 >>> M[0,1] = 42.0 >>> M[0,2] = 42.0 >>> M.reduce_float() 84.0 """ if mon is None: mon = current_monoid.get(self.type.PLUS_MONOID) mon = mon.get_op() mask, accum, desc = self._get_args(mask, accum, desc) result = ffi.new("double*") _check( self, lib.GrB_Matrix_reduce_FP64(result, accum, mon, self._matrix[0], desc) ) return result[0]
def reduce(self, mon=None, accum=None, desc=None)
-
Do a scalar reduce based on this object's type:
>>> M = Matrix.random(types.UINT8, 10, 3, 3, seed=42) >>> M.reduce() 133
>>> M = Matrix.random(types.FP32, 10, 3, 3, seed=42) >>> M.reduce() 2.937098979949951
>>> M = Matrix.random(types.UINT8, 10, 3, 3, seed=42) >>> M.reduce(M.type.min_monoid) 13
>>> M = Matrix.random(types.BOOL, 10, 3, 3, seed=42) >>> M.reduce() True
Expand source code
def reduce(self, mon=None, accum=None, desc=None): """Do a scalar reduce based on this object's type: >>> M = Matrix.random(types.UINT8, 10, 3, 3, seed=42) >>> M.reduce() 133 >>> M = Matrix.random(types.FP32, 10, 3, 3, seed=42) >>> M.reduce() 2.937098979949951 >>> M = Matrix.random(types.UINT8, 10, 3, 3, seed=42) >>> M.reduce(M.type.min_monoid) 13 >>> M = Matrix.random(types.BOOL, 10, 3, 3, seed=42) >>> M.reduce() True """ if mon is None: if self.type is types.BOOL: mon = current_monoid.get(getattr(self.type, "lor_monoid")) else: mon = current_monoid.get(getattr(self.type, "plus_monoid")) mon = mon.get_op() mask, accum, desc = self._get_args(None, accum, desc) result = ffi.new(self.type._c_type + "*") _check( self, self.type._Matrix_reduce(result, accum, mon, self._matrix[0], desc) ) return result[0]
def reduce_vector(self, mon=None, out=None, cast=None, mask=None, accum=None, desc=None)
-
Reduce matrix to a vector.
>>> M = Matrix.sparse(types.FP32, 3, 3) >>> print(M.reduce_vector()) 0| 1| 2| >>> M[0,1] = 42.0 >>> M[0,2] = 42.0 >>> M[2,0] = -42.0 >>> print(M.reduce_vector()) 0|84.0 1| 2|-42.0
>>> print(M.reduce_vector(types.FP32.MIN_MONOID)) 0|42.0 1| 2|-42.0
>>> v = Vector.sparse(types.FP32, M.nrows) >>> print(M.reduce_vector(out=v)) 0|84.0 1| 2|-42.0
>>> M = Matrix.sparse(types.BOOL, 3, 3) >>> print(M.reduce_vector()) 0| 1| 2|
>>> M[0,1] = True >>> M[0,2] = True >>> M[2,0] = True >>> print(M.reduce_vector()) 0| t 1| 2| t
If there is no
out
parameter, the newly created result vector can also be cast to a different type:>>> print(M.reduce_vector(cast=types.UINT8)) 0| 2 1| 2| 1
Expand source code
def reduce_vector( self, mon=None, out=None, cast=None, mask=None, accum=None, desc=None ): """Reduce matrix to a vector. >>> M = Matrix.sparse(types.FP32, 3, 3) >>> print(M.reduce_vector()) 0| 1| 2| >>> M[0,1] = 42.0 >>> M[0,2] = 42.0 >>> M[2,0] = -42.0 >>> print(M.reduce_vector()) 0|84.0 1| 2|-42.0 >>> print(M.reduce_vector(types.FP32.MIN_MONOID)) 0|42.0 1| 2|-42.0 >>> v = Vector.sparse(types.FP32, M.nrows) >>> print(M.reduce_vector(out=v)) 0|84.0 1| 2|-42.0 >>> M = Matrix.sparse(types.BOOL, 3, 3) >>> print(M.reduce_vector()) 0| 1| 2| >>> M[0,1] = True >>> M[0,2] = True >>> M[2,0] = True >>> print(M.reduce_vector()) 0| t 1| 2| t If there is no `out` parameter, the newly created result vector can also be cast to a different type: >>> print(M.reduce_vector(cast=types.UINT8)) 0| 2 1| 2| 1 """ if out is None: if cast is None: T = self.type else: T = cast out = Vector.sparse(T, self.nrows) if mon is None: if out.type is types.BOOL: mon = current_monoid.get(getattr(out.type, "lor_monoid")) else: mon = current_monoid.get(getattr(out.type, "plus_monoid")) mon = mon.get_op() mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_reduce_Monoid( out._vector[0], mask, accum, mon, self._matrix[0], desc ), ) return out
def apply(self, op, out=None, mask=None, accum=None, desc=None)
-
Apply Unary op to matrix elements.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.apply(types.INT64.ABS)) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2
>>> print(M.apply(types.INT64.ABS)) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2
Expand source code
def apply(self, op, out=None, mask=None, accum=None, desc=None): """Apply Unary op to matrix elements. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.apply(types.INT64.ABS)) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.apply(types.INT64.ABS)) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 """ if out is None: out = self.__class__.sparse(self.type, self.nrows, self.ncols) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_apply( out._matrix[0], mask, accum, op, self._matrix[0], desc ), ) return out
def apply_first(self, first, op, out=None, mask=None, accum=None, desc=None)
-
Apply a binary operator to the entries in a matrix, binding the first input to a scalar first.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.apply_first(1, types.INT64.PLUS)) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 >>> N = Matrix.sparse(M.type, M.nrows, M.ncols) >>> print(M.apply_first(1, types.INT64.PLUS, out=N)) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2
apply_first
is also used when aMatrix
is used "on the right" for math operations like+-*.
with a scalar:>>> print(1 + M) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2
Expand source code
def apply_first(self, first, op, out=None, mask=None, accum=None, desc=None): """Apply a binary operator to the entries in a matrix, binding the first input to a scalar first. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.apply_first(1, types.INT64.PLUS)) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 >>> N = Matrix.sparse(M.type, M.nrows, M.ncols) >>> print(M.apply_first(1, types.INT64.PLUS, out=N)) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 `apply_first` is also used when a `Matrix` is used "on the right" for math operations like `+-*.` with a scalar: >>> print(1 + M) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 """ if out is None: out = self.__class__.sparse(self.type, self.nrows, self.ncols) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) if isinstance(first, Scalar): f = lib.GxB_Matrix_apply_BinaryOp1st first = first._scalar[0] else: f = self.type._Matrix_apply_BinaryOp1st _check(self, f(out._matrix[0], mask, accum, op, first, self._matrix[0], desc)) return out
def apply_second(self, op, second, out=None, mask=None, accum=None, desc=None)
-
Apply a binary operator to the entries in a matrix, binding the second input to a scalar second.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.apply_second(types.INT64.PLUS, 1)) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2
apply_second
is also used when aMatrix
is used "on the left" for math operations like+-*.
with a scalar:>>> print(M + 1) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2
Expand source code
def apply_second(self, op, second, out=None, mask=None, accum=None, desc=None): """Apply a binary operator to the entries in a matrix, binding the second input to a scalar second. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.apply_second(types.INT64.PLUS, 1)) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 `apply_second` is also used when a `Matrix` is used "on the left" for math operations like `+-*.` with a scalar: >>> print(M + 1) 0 1 2 0| -41 | 0 1| 1| 1 2|150 | 2 0 1 2 """ if out is None: out = self.__class__.sparse(self.type, self.nrows, self.ncols) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) if isinstance(second, Scalar): f = lib.GxB_Matrix_apply_BinaryOp2nd second = second._scalar[0] else: f = self.type._Matrix_apply_BinaryOp2nd _check(self, f(out._matrix[0], mask, accum, op, self._matrix[0], second, desc)) return out
def select(self, op, thunk=None, out=None, mask=None, accum=None, desc=None)
-
Select elements that match the given select operation condition. Can be a string mapping to following operators:
Operator Library Operation Definition >
lib.GxB_GT_THUNK Select greater than 'thunk'. <
lib.GxB_LT_THUNK Select less than 'thunk'. >=
lib.GxB_GE_THUNK Select greater than or equal to 'thunk'. <=
lib.GxB_LE_THUNK Select less than or equal to 'thunk'. !=
lib.GxB_NE_THUNK Select not equal to 'thunk'. ==
lib.GxB_EQ_THUNK Select equal to 'thunk'. >0
lib.GxB_GT_ZERO Select greater than zero. <0
lib.GxB_LT_ZERO Select less than zero. >=0
lib.GxB_GE_ZERO Select greater than or equal to zero. <=0
lib.GxB_LE_ZERO Select less than or equal to zero. !=0
lib.GxB_NONZERO Select nonzero value. ==0
lib.GxB_EQ_ZERO Select equal to zero. max
no equivalent Select max values. min
no equivalent Select min values. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.select('>', 0)) 0 1 2 0| | 0 1| | 1 2|149 | 2 0 1 2 >>> print(M.select('>=', 0)) 0 1 2 0| | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.select('<', 0)) 0 1 2 0| -42 | 0 1| | 1 2| | 2 0 1 2 >>> N = M.dup(clear=True) >>> M.select('<', 0, out=N) is N True >>> print(N) 0 1 2 0| -42 | 0 1| | 1 2| | 2 0 1 2 >>> N = M.dup(clear=True) >>> M.select('min', out=N) is N True >>> print(N) 0 1 2 0| -42 | 0 1| | 1 2| | 2 0 1 2 >>> N = M.dup(clear=True) >>> M.select('max', out=N) is N True >>> print(N) 0 1 2 0| | 0 1| | 1 2|149 | 2 0 1 2
Expand source code
def select(self, op, thunk=None, out=None, mask=None, accum=None, desc=None): """Select elements that match the given select operation condition. Can be a string mapping to following operators: Operator | Library Operation | Definition --- | --- | --- `>` | lib.GxB_GT_THUNK | Select greater than 'thunk'. `<` | lib.GxB_LT_THUNK | Select less than 'thunk'. `>=` | lib.GxB_GE_THUNK | Select greater than or equal to 'thunk'. `<=` | lib.GxB_LE_THUNK | Select less than or equal to 'thunk'. `!=` | lib.GxB_NE_THUNK | Select not equal to 'thunk'. `==` | lib.GxB_EQ_THUNK | Select equal to 'thunk'. `>0` | lib.GxB_GT_ZERO | Select greater than zero. `<0` | lib.GxB_LT_ZERO | Select less than zero. `>=0` | lib.GxB_GE_ZERO | Select greater than or equal to zero. `<=0` | lib.GxB_LE_ZERO | Select less than or equal to zero. `!=0` | lib.GxB_NONZERO | Select nonzero value. `==0` | lib.GxB_EQ_ZERO | Select equal to zero. `max` | no equivalent | Select max values. `min` | no equivalent | Select min values. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [-42, 0, 149]) >>> print(M.select('>', 0)) 0 1 2 0| | 0 1| | 1 2|149 | 2 0 1 2 >>> print(M.select('>=', 0)) 0 1 2 0| | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.select('<', 0)) 0 1 2 0| -42 | 0 1| | 1 2| | 2 0 1 2 >>> N = M.dup(clear=True) >>> M.select('<', 0, out=N) is N True >>> print(N) 0 1 2 0| -42 | 0 1| | 1 2| | 2 0 1 2 >>> N = M.dup(clear=True) >>> M.select('min', out=N) is N True >>> print(N) 0 1 2 0| -42 | 0 1| | 1 2| | 2 0 1 2 >>> N = M.dup(clear=True) >>> M.select('max', out=N) is N True >>> print(N) 0 1 2 0| | 0 1| | 1 2|149 | 2 0 1 2 """ if out is None: out = self.__class__.sparse(self.type, self.nrows, self.ncols) if isinstance(op, str): if op == "min": op = lib.GxB_EQ_THUNK thunk = self.reduce_float(self.type.min_monoid) elif op == "max": op = lib.GxB_EQ_THUNK thunk = self.reduce_float(self.type.max_monoid) else: op = _get_select_op(op) elif isinstance(op, SelectOp): op = op.get_op() if thunk is None: thunk = NULL if isinstance(thunk, (bool, int, float, complex)): thunk = Scalar.from_value(thunk) if isinstance(thunk, Scalar): self._keep_alives[self._matrix] = thunk thunk = thunk._scalar[0] mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GxB_Matrix_select( out._matrix[0], mask, accum, op, self._matrix[0], thunk, desc ), ) return out
def tril(self, offset=None)
-
Select the lower triangular Matrix.
The diagonal
offset
can be used to select all below any diagonal rank, positive towars the upper right coner and negative toward the lower left.>>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.tril()) 0 1 2 0| 0 | 0 1| 0 0 | 1 2| 0 0 0| 2 0 1 2 >>> print(M.tril(1)) 0 1 2 0| 0 0 | 0 1| 0 0 0| 1 2| 0 0 0| 2 0 1 2 >>> print(M.tril(-1)) 0 1 2 0| | 0 1| 0 | 1 2| 0 0 | 2 0 1 2
Expand source code
def tril(self, offset=None): """Select the lower triangular Matrix. The diagonal `offset` can be used to select all below any diagonal rank, positive towars the upper right coner and negative toward the lower left. >>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.tril()) 0 1 2 0| 0 | 0 1| 0 0 | 1 2| 0 0 0| 2 0 1 2 >>> print(M.tril(1)) 0 1 2 0| 0 0 | 0 1| 0 0 0| 1 2| 0 0 0| 2 0 1 2 >>> print(M.tril(-1)) 0 1 2 0| | 0 1| 0 | 1 2| 0 0 | 2 0 1 2 """ return self.select(lib.GxB_TRIL, thunk=offset)
def triu(self, offset=None)
-
Select the upper triangular Matrix.
The diagonal
offset
can be used to select all above any diagonal rank, positive towars the upper right coner and negative toward the lower left.>>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.triu()) 0 1 2 0| 0 0 0| 0 1| 0 0| 1 2| 0| 2 0 1 2 >>> print(M.triu(1)) 0 1 2 0| 0 0| 0 1| 0| 1 2| | 2 0 1 2 >>> print(M.triu(-1)) 0 1 2 0| 0 0 0| 0 1| 0 0 0| 1 2| 0 0| 2 0 1 2
Expand source code
def triu(self, offset=None): """Select the upper triangular Matrix. The diagonal `offset` can be used to select all above any diagonal rank, positive towars the upper right coner and negative toward the lower left. >>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.triu()) 0 1 2 0| 0 0 0| 0 1| 0 0| 1 2| 0| 2 0 1 2 >>> print(M.triu(1)) 0 1 2 0| 0 0| 0 1| 0| 1 2| | 2 0 1 2 >>> print(M.triu(-1)) 0 1 2 0| 0 0 0| 0 1| 0 0 0| 1 2| 0 0| 2 0 1 2 """ return self.select(lib.GxB_TRIU, thunk=offset)
def diag(self, offset=None)
-
Select the diagonal Matrix.
The diagonal
offset
can be used to select any diagonal rank, positive towars the upper right coner and negative toward the lower left.>>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.diag()) 0 1 2 0| 0 | 0 1| 0 | 1 2| 0| 2 0 1 2 >>> print(M.diag(1)) 0 1 2 0| 0 | 0 1| 0| 1 2| | 2 0 1 2 >>> print(M.diag(-1)) 0 1 2 0| | 0 1| 0 | 1 2| 0 | 2 0 1 2
Expand source code
def diag(self, offset=None): """Select the diagonal Matrix. The diagonal `offset` can be used to select any diagonal rank, positive towars the upper right coner and negative toward the lower left. >>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.diag()) 0 1 2 0| 0 | 0 1| 0 | 1 2| 0| 2 0 1 2 >>> print(M.diag(1)) 0 1 2 0| 0 | 0 1| 0| 1 2| | 2 0 1 2 >>> print(M.diag(-1)) 0 1 2 0| | 0 1| 0 | 1 2| 0 | 2 0 1 2 """ return self.select(lib.GxB_DIAG, thunk=offset)
def vector_diag(self, k=0, desc=None)
-
GxB_Vector_diag extracts a vector v from an input matrix A, which may be rectangular. If k = 0, the main diagonal of A is extracted; k > 0 denotes diagonals above the main diagonal of A, and k < 0 denotes diagonals below the main diagonal of A. Let A have dimension m-by-n. If k is in the range 0 to n-1, then v has length min(m,n-k). If k is negative and in the range -1 to -m+1, then v has length min(m+k,n). If k is outside these ranges, v has length 0 (this is not an error). This function computes the same thing as the MATLAB statement v = diag(A,k) when A is a matrix, except that GxB_Vector_diag can also do typecasting.
>>> from pygraphblas import UINT8 >>> A = Matrix.dense(UINT8, 2, 2, fill=1) >>> print(A) 0 1 0| 1 1| 0 1| 1 1| 1 0 1 >>> print(A.vector_diag()) 0| 1 1| 1 >>> print(A.vector_diag(1)) 0| 1 >>> A.vector_diag(2) <Vector(UINT8 size: 0, nvals: 0)> >>> print(A.vector_diag(-1)) 0| 1 >>> A.vector_diag(-2) <Vector(UINT8 size: 0, nvals: 0)>
Expand source code
def vector_diag(self, k=0, desc=None): """ GxB_Vector_diag extracts a vector v from an input matrix A, which may be rectangular. If k = 0, the main diagonal of A is extracted; k > 0 denotes diagonals above the main diagonal of A, and k < 0 denotes diagonals below the main diagonal of A. Let A have dimension m-by-n. If k is in the range 0 to n-1, then v has length min(m,n-k). If k is negative and in the range -1 to -m+1, then v has length min(m+k,n). If k is outside these ranges, v has length 0 (this is not an error). This function computes the same thing as the MATLAB statement v = diag(A,k) when A is a matrix, except that GxB_Vector_diag can also do typecasting. >>> from pygraphblas import UINT8 >>> A = Matrix.dense(UINT8, 2, 2, fill=1) >>> print(A) 0 1 0| 1 1| 0 1| 1 1| 1 0 1 >>> print(A.vector_diag()) 0| 1 1| 1 >>> print(A.vector_diag(1)) 0| 1 >>> A.vector_diag(2) <Vector(UINT8 size: 0, nvals: 0)> >>> print(A.vector_diag(-1)) 0| 1 >>> A.vector_diag(-2) <Vector(UINT8 size: 0, nvals: 0)> """ n, m = self.shape if k in range(0, n): l = min(m, n - k) elif k in range(-1, -m, -1): l = min(m + k, n) else: l = 0 v = Vector.sparse(self.type, l) _, _, desc = self._get_args(desc=desc) _check(self, lib.GxB_Vector_diag(v._vector[0], self._matrix[0], k, desc)) return v
def offdiag(self, offset=None)
-
Select the off-diagonal Matrix.
The diagonal
offset
can be used to select off any diagonal rank, positive towars the upper right coner and negative toward the lower left.>>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.offdiag()) 0 1 2 0| 0 0| 0 1| 0 0| 1 2| 0 0 | 2 0 1 2 >>> print(M.offdiag(1)) 0 1 2 0| 0 0| 0 1| 0 0 | 1 2| 0 0 0| 2 0 1 2 >>> print(M.offdiag(-1)) 0 1 2 0| 0 0 0| 0 1| 0 0| 1 2| 0 0| 2 0 1 2
Expand source code
def offdiag(self, offset=None): """Select the off-diagonal Matrix. The diagonal `offset` can be used to select off any diagonal rank, positive towars the upper right coner and negative toward the lower left. >>> M = Matrix.dense(types.UINT8, 3, 3) >>> print(M.offdiag()) 0 1 2 0| 0 0| 0 1| 0 0| 1 2| 0 0 | 2 0 1 2 >>> print(M.offdiag(1)) 0 1 2 0| 0 0| 0 1| 0 0 | 1 2| 0 0 0| 2 0 1 2 >>> print(M.offdiag(-1)) 0 1 2 0| 0 0 0| 0 1| 0 0| 1 2| 0 0| 2 0 1 2 """ return self.select(lib.GxB_OFFDIAG, thunk=offset)
def nonzero(self)
-
Select the non-zero Matrix.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M.nonzero()) 0 1 2 0| 42 | 0 1| | 1 2|149 | 2 0 1 2
Expand source code
def nonzero(self): """Select the non-zero Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M.nonzero()) 0 1 2 0| 42 | 0 1| | 1 2|149 | 2 0 1 2 """ return self.select(lib.GxB_NONZERO)
def mxm(self, other, semiring=None, cast=None, out=None, mask=None, accum=None, desc=None)
-
Matrix-matrix multiply.
Multiply this matrix by
other
matrix.See Section 9.6 in the SuiteSparse User Guide for details.
mxm
can be called directly or with the@
operator:>>> m = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> n = Matrix.from_lists([0, 1, 2], [1, 2, 0], [2, 3, 4]) >>> print(m) 0 1 2 0| 1 | 0 1| 2| 1 2| 3 | 2 0 1 2 >>> print(n) 0 1 2 0| 2 | 0 1| 3| 1 2| 4 | 2 0 1 2
Matrix multiply
m
byn
:>>> o = m.mxm(n) >>> print(o) 0 1 2 0| 3| 0 1| 8 | 1 2| 6 | 2 0 1 2
Matrix matrix with the
@
operator:>>> o = m @ n >>> print(o) 0 1 2 0| 3| 0 1| 8 | 1 2| 6 | 2 0 1 2
By default,
mxm
and@
create a new result matrix of the correct type and dimensions if one is not provided. If you want to provide your own matrix to put the result in, you can pass it in theout
parameter. This is useful for accumulating results into a single matrix with minimal copying. This is also supported by the@=
syntax:>>> o = m.dup() >>> o.mxm(n, accum=types.INT64.min, out=o) is o True >>> print(o) 0 1 2 0| 1 3| 0 1| 8 2| 1 2| 3 6 | 2 0 1 2 >>> o = m.dup() >>> with Accum(types.INT64.min): ... o @= n >>> print(o) 0 1 2 0| 1 3| 0 1| 8 2| 1 2| 3 6 | 2 0 1 2
The default semiring depends on the infered result type. In the case of numbers, the default semiring is
PLUS_TIMES
. In the case of typeBOOL
, it isBOOL.LOR_LAND
.>>> from pygraphblas import INT64 >>> o = m.mxm(n, semiring=INT64.min_plus) >>> print(o) 0 1 2 0| 4| 0 1| 6 | 1 2| 5 | 2 0 1 2
An explicit semiring can be passed to the method or provided with a context manager:
>>> with INT64.min_plus: ... o = m @ n >>> print(o) 0 1 2 0| 4| 0 1| 6 | 1 2| 5 | 2 0 1 2
Or the semiring can be accessed via an attribute on the matrix:
>>> o = m.min_plus(n) >>> print(o) 0 1 2 0| 4| 0 1| 6 | 1 2| 5 | 2 0 1 2
Descriptors and accumulators can also be provided as an argument or a context manager:
>>> descriptor.T0 <Descriptor T0> >>> o = m.mxm(n, desc=descriptor.T0) >>> print(o) 0 1 2 0| 12 | 0 1| 2 | 1 2| 6| 2 0 1 2 >>> with descriptor.T0: ... o = m @ n >>> print(o) 0 1 2 0| 12 | 0 1| 2 | 1 2| 6| 2 0 1 2
The accumulator context manager requires an extra
Accum
helper class to distinguish it from binary ops used ineadd
andemult
.Output can be cast to a specific type by passing the cast parameter:
>>> o = m.mxm(n, cast=types.FP32) >>> print(o) 0 1 2 0| 3.0| 0 1|8.0 | 1 2| 6.0 | 2 0 1 2
Expand source code
def mxm( self, other, semiring=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Matrix-matrix multiply. Multiply this matrix by `other` matrix. See Section 9.6 in the [SuiteSparse User Guide](https://raw.githubusercontent.com/DrTimothyAldenDavis/GraphBLAS/stable/Doc/GraphBLAS_UserGuide.pdf) for details. `mxm` can be called directly or with the `@` operator: >>> m = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> n = Matrix.from_lists([0, 1, 2], [1, 2, 0], [2, 3, 4]) >>> print(m) 0 1 2 0| 1 | 0 1| 2| 1 2| 3 | 2 0 1 2 >>> print(n) 0 1 2 0| 2 | 0 1| 3| 1 2| 4 | 2 0 1 2 Matrix multiply `m` by `n`: >>> o = m.mxm(n) >>> print(o) 0 1 2 0| 3| 0 1| 8 | 1 2| 6 | 2 0 1 2 Matrix matrix with the `@` operator: >>> o = m @ n >>> print(o) 0 1 2 0| 3| 0 1| 8 | 1 2| 6 | 2 0 1 2 By default, `mxm` and `@` create a new result matrix of the correct type and dimensions if one is not provided. If you want to provide your own matrix to put the result in, you can pass it in the `out` parameter. This is useful for accumulating results into a single matrix with minimal copying. This is also supported by the `@=` syntax: >>> o = m.dup() >>> o.mxm(n, accum=types.INT64.min, out=o) is o True >>> print(o) 0 1 2 0| 1 3| 0 1| 8 2| 1 2| 3 6 | 2 0 1 2 >>> o = m.dup() >>> with Accum(types.INT64.min): ... o @= n >>> print(o) 0 1 2 0| 1 3| 0 1| 8 2| 1 2| 3 6 | 2 0 1 2 The default semiring depends on the infered result type. In the case of numbers, the default semiring is `PLUS_TIMES`. In the case of type `BOOL`, it is `BOOL.LOR_LAND`. >>> from pygraphblas import INT64 >>> o = m.mxm(n, semiring=INT64.min_plus) >>> print(o) 0 1 2 0| 4| 0 1| 6 | 1 2| 5 | 2 0 1 2 An explicit semiring can be passed to the method or provided with a context manager: >>> with INT64.min_plus: ... o = m @ n >>> print(o) 0 1 2 0| 4| 0 1| 6 | 1 2| 5 | 2 0 1 2 Or the semiring can be accessed via an attribute on the matrix: >>> o = m.min_plus(n) >>> print(o) 0 1 2 0| 4| 0 1| 6 | 1 2| 5 | 2 0 1 2 Descriptors and accumulators can also be provided as an argument or a context manager: >>> descriptor.T0 <Descriptor T0> >>> o = m.mxm(n, desc=descriptor.T0) >>> print(o) 0 1 2 0| 12 | 0 1| 2 | 1 2| 6| 2 0 1 2 >>> with descriptor.T0: ... o = m @ n >>> print(o) 0 1 2 0| 12 | 0 1| 2 | 1 2| 6| 2 0 1 2 The accumulator context manager requires an extra `Accum` helper class to distinguish it from binary ops used in `eadd` and `emult`. Output can be cast to a specific type by passing the cast parameter: >>> o = m.mxm(n, cast=types.FP32) >>> print(o) 0 1 2 0| 3.0| 0 1|8.0 | 1 2| 6.0 | 2 0 1 2 """ if semiring is None: semiring = current_semiring.get(NULL) if out is None: if cast is not None: typ = cast elif semiring is not NULL: typ = semiring.ztype else: typ = types.promote(self.type, other.type) out = self.__class__.sparse(typ, self.nrows, other.ncols) else: typ = out.type if semiring is NULL: semiring = out.type._default_semiring() semiring = semiring.get_op() mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_mxm( out._matrix[0], mask, accum, semiring, self._matrix[0], other._matrix[0], desc, ), ) return out
def mxv(self, other, semiring=None, cast=None, out=None, mask=None, accum=None, desc=None)
-
Matrix-vector multiply.
Multiply this matrix by
other
column vector "on the right". For row vector multiplication "on the left" seeVector.vxm()
.See Section 9.6 in the SuiteSparse User Guide for details.
mxv
can also be called directly or with the@
operator:>>> from pygraphblas import INT64 >>> m = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> v = Vector.from_lists([0, 1, 2], [2, 3, 4]) >>> o = m.mxv(v) >>> print(o) 0| 3 1| 8 2| 6 >>> o = m @ v >>> print(o) 0| 3 1| 8 2| 6
By default,
mxv
and@
create a new result matrix of the correct type and dimensions if one is not provided. If you want to provide your own matrix to put the result in, you can pass it in theout
parameter. This is useful for accumulating results into a single matrix with minimal copying.>>> o = v.dup() >>> m.mxv(v, accum=INT64.plus, out=o) is o True >>> print(o) 0| 5 1|11 2|10
The default semiring depends on the infered result type. In the case of numbers, the default semiring is
PLUS_TIMES
. In the case of typeBOOL
, it isBOOL.LOR_LAND
.An explicit semiring can be passed to the method or provided with a context manager:
>>> o = m.mxv(v, semiring=INT64.min_plus) >>> print(o) 0| 4 1| 6 2| 5
>>> with INT64.min_plus: ... o = m @ v >>> print(o) 0| 4 1| 6 2| 5
>>> o = m.min_plus(v) >>> print(o) 0| 4 1| 6 2| 5
Descriptors and accumulators can also be provided as an argument or a context manager:
>>> o = m.mxv(v, desc=descriptor.T0) >>> print(o) 0|12 1| 2 2| 6
>>> with descriptor.T0: ... o = m @ v >>> print(o) 0|12 1| 2 2| 6
>>> del o[1] >>> o = m.mxv(v, mask=o) >>> print(o) 0| 3 1| 2| 6
>>> o = m.mxv(v, cast=types.FP32) >>> print(o) 0|3.0 1|8.0 2|6.0
Expand source code
def mxv( self, other, semiring=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Matrix-vector multiply. Multiply this matrix by `other` column vector "on the right". For row vector multiplication "on the left" see `Vector.vxm`. See Section 9.6 in the [SuiteSparse User Guide](https://raw.githubusercontent.com/DrTimothyAldenDavis/GraphBLAS/stable/Doc/GraphBLAS_UserGuide.pdf) for details. `mxv` can also be called directly or with the `@` operator: >>> from pygraphblas import INT64 >>> m = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> v = Vector.from_lists([0, 1, 2], [2, 3, 4]) >>> o = m.mxv(v) >>> print(o) 0| 3 1| 8 2| 6 >>> o = m @ v >>> print(o) 0| 3 1| 8 2| 6 By default, `mxv` and `@` create a new result matrix of the correct type and dimensions if one is not provided. If you want to provide your own matrix to put the result in, you can pass it in the `out` parameter. This is useful for accumulating results into a single matrix with minimal copying. >>> o = v.dup() >>> m.mxv(v, accum=INT64.plus, out=o) is o True >>> print(o) 0| 5 1|11 2|10 The default semiring depends on the infered result type. In the case of numbers, the default semiring is `PLUS_TIMES`. In the case of type `BOOL`, it is `BOOL.LOR_LAND`. An explicit semiring can be passed to the method or provided with a context manager: >>> o = m.mxv(v, semiring=INT64.min_plus) >>> print(o) 0| 4 1| 6 2| 5 >>> with INT64.min_plus: ... o = m @ v >>> print(o) 0| 4 1| 6 2| 5 >>> o = m.min_plus(v) >>> print(o) 0| 4 1| 6 2| 5 Descriptors and accumulators can also be provided as an argument or a context manager: >>> o = m.mxv(v, desc=descriptor.T0) >>> print(o) 0|12 1| 2 2| 6 >>> with descriptor.T0: ... o = m @ v >>> print(o) 0|12 1| 2 2| 6 >>> del o[1] >>> o = m.mxv(v, mask=o) >>> print(o) 0| 3 1| 2| 6 >>> o = m.mxv(v, cast=types.FP32) >>> print(o) 0|3.0 1|8.0 2|6.0 """ if semiring is None: semiring = current_semiring.get(NULL) if out is None: new_dimension = self.ncols if T0 in (desc or ()) else self.nrows if cast is not None: typ = cast elif semiring is not NULL: typ = semiring.ztype else: typ = types.promote(self.type, other.type) out = Vector.sparse(typ, new_dimension) else: typ = out.type if semiring is NULL: semiring = out.type._default_semiring() semiring = semiring.get_op() mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_mxv( out._vector[0], mask, accum, semiring, self._matrix[0], other._vector[0], desc, ), ) return out
def kronecker(self, other, op=None, cast=None, out=None, mask=None, accum=None, desc=None)
-
>>> n = Matrix.from_lists([0, 1, 2], [1, 2, 0], [2, 3, 4]) >>> m = Matrix.dense(types.UINT64, 3, 3, fill=1) >>> print(n.kronecker(m)) 0 1 2 3 4 5 6 7 8 0| 2 2 2 | 0 1| 2 2 2 | 1 2| 2 2 2 | 2 3| 3 3 3| 3 4| 3 3 3| 4 5| 3 3 3| 5 6| 4 4 4 | 6 7| 4 4 4 | 7 8| 4 4 4 | 8 0 1 2 3 4 5 6 7 8
>>> o = Matrix.sparse(types.UINT64, 9, 9) >>> m.kronecker(n, out=o) is o True >>> print(o) 0 1 2 3 4 5 6 7 8 0| 2 2 2 | 0 1| 3 3 3| 1 2| 4 4 4 | 2 3| 2 2 2 | 3 4| 3 3 3| 4 5| 4 4 4 | 5 6| 2 2 2 | 6 7| 3 3 3| 7 8| 4 4 4 | 8 0 1 2 3 4 5 6 7 8
>>> print(m.kronecker(n, op=types.UINT64.MIN)) 0 1 2 3 4 5 6 7 8 0| 1 1 1 | 0 1| 1 1 1| 1 2| 1 1 1 | 2 3| 1 1 1 | 3 4| 1 1 1| 4 5| 1 1 1 | 5 6| 1 1 1 | 6 7| 1 1 1| 7 8| 1 1 1 | 8 0 1 2 3 4 5 6 7 8
Expand source code
def kronecker( self, other, op=None, cast=None, out=None, mask=None, accum=None, desc=None ): """[Kronecker product](https://en.wikipedia.org/wiki/Kronecker_product). >>> n = Matrix.from_lists([0, 1, 2], [1, 2, 0], [2, 3, 4]) >>> m = Matrix.dense(types.UINT64, 3, 3, fill=1) >>> print(n.kronecker(m)) 0 1 2 3 4 5 6 7 8 0| 2 2 2 | 0 1| 2 2 2 | 1 2| 2 2 2 | 2 3| 3 3 3| 3 4| 3 3 3| 4 5| 3 3 3| 5 6| 4 4 4 | 6 7| 4 4 4 | 7 8| 4 4 4 | 8 0 1 2 3 4 5 6 7 8 >>> o = Matrix.sparse(types.UINT64, 9, 9) >>> m.kronecker(n, out=o) is o True >>> print(o) 0 1 2 3 4 5 6 7 8 0| 2 2 2 | 0 1| 3 3 3| 1 2| 4 4 4 | 2 3| 2 2 2 | 3 4| 3 3 3| 4 5| 4 4 4 | 5 6| 2 2 2 | 6 7| 3 3 3| 7 8| 4 4 4 | 8 0 1 2 3 4 5 6 7 8 >>> print(m.kronecker(n, op=types.UINT64.MIN)) 0 1 2 3 4 5 6 7 8 0| 1 1 1 | 0 1| 1 1 1| 1 2| 1 1 1 | 2 3| 1 1 1 | 3 4| 1 1 1| 4 5| 1 1 1 | 5 6| 1 1 1 | 6 7| 1 1 1| 7 8| 1 1 1 | 8 0 1 2 3 4 5 6 7 8 """ mask, accum, desc = self._get_args(mask, accum, desc) typ = cast or types.promote(self.type, other.type) if out is None: out = self.__class__.sparse( typ, self.nrows * other.nrows, self.ncols * other.ncols ) if op is None: op = current_binop.get(self.type.TIMES) op = op.get_op() _check( self, lib.GrB_Matrix_kronecker_BinaryOp( out._matrix[0], mask, accum, op, self._matrix[0], other._matrix[0], desc ), ) return out
def extract_matrix(self, row_index=None, col_index=None, out=None, mask=None, accum=None, desc=None)
-
Extract a submatrix.
GrB_Matrix_extract
extracts a submatrix from another matrix. The input matrix may be transposed first, via the descriptor. The result type remains the same.row_index
andcol_index
can be slice objects that default to the equivalent of GrB_ALL. Python slice objects support the SuiteSparse extensions forGxB_RANGE
,GxB_BACKWARDS
andGxB_STRIDE
. See the User Guide for details.The size of
C
is|row_index|
-by-|col_index|
. Entries outside that sub-range are not accessed and do not take part in the computation.>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M.extract_matrix()) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2
>>> print(M.extract_matrix(0, 1)) 0 0| 42| 0 0
>>> O = Matrix.sparse(types.UINT64, 1, 1) >>> M.extract_matrix(0, 1, out=O) is O True >>> print(O) 0 0| 42| 0 0
>>> print(M.extract_matrix(slice(1,2), 2)) 0 0| 0| 0 1| | 1 0
>>> print(M.extract_matrix(0, slice(0,1))) 0 1 0| 42| 0 0 1
>>> N = Matrix.from_lists([1, 2], [2, 0], [True, True]) >>> print(M[N]) 0 1 2 0| | 0 1| 0| 1 2|149 | 2 0 1 2
Expand source code
def extract_matrix( self, row_index=None, col_index=None, out=None, mask=None, accum=None, desc=None, ): """Extract a submatrix. `GrB_Matrix_extract` extracts a submatrix from another matrix. The input matrix may be transposed first, via the descriptor. The result type remains the same. `row_index` and `col_index` can be slice objects that default to the equivalent of GrB_ALL. Python slice objects support the SuiteSparse extensions for `GxB_RANGE`, `GxB_BACKWARDS` and `GxB_STRIDE`. See the User Guide for details. The size of `C` is `|row_index|`-by-`|col_index|`. Entries outside that sub-range are not accessed and do not take part in the computation. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M.extract_matrix()) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.extract_matrix(0, 1)) 0 0| 42| 0 0 >>> O = Matrix.sparse(types.UINT64, 1, 1) >>> M.extract_matrix(0, 1, out=O) is O True >>> print(O) 0 0| 42| 0 0 >>> print(M.extract_matrix(slice(1,2), 2)) 0 0| 0| 0 1| | 1 0 >>> print(M.extract_matrix(0, slice(0,1))) 0 1 0| 42| 0 0 1 >>> N = Matrix.from_lists([1, 2], [2, 0], [True, True]) >>> print(M[N]) 0 1 2 0| | 0 1| 0| 1 2|149 | 2 0 1 2 """ ta = T0 in (desc or ()) mask, accum, desc = self._get_args(mask, accum, desc) result_nrows = self.ncols if ta else self.nrows result_ncols = self.nrows if ta else self.ncols if isinstance(row_index, int): I, ni, isize = _build_range(slice(row_index, row_index), result_nrows - 1) else: I, ni, isize = _build_range(row_index, result_nrows - 1) if isinstance(col_index, int): J, nj, jsize = _build_range(slice(col_index, col_index), result_ncols - 1) else: J, nj, jsize = _build_range(col_index, result_ncols - 1) if isize is None: isize = result_nrows if jsize is None: jsize = result_ncols if out is None: out = self.__class__.sparse(self.type, isize, jsize) _check( self, lib.GrB_Matrix_extract( out._matrix[0], mask, accum, self._matrix[0], I, ni, J, nj, desc ), ) return out
def extract_col(self, col_index, row_slice=None, out=None, mask=None, accum=None, desc=None)
-
Extract a column Vector.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.extract_col(0)) 0| 1| 2|149
>>> v = Vector.sparse(types.UINT64, M.ncols) >>> M.extract_col(0, out=v) is v True >>> print(v) 0| 1| 2|149
Expand source code
def extract_col( self, col_index, row_slice=None, out=None, mask=None, accum=None, desc=None ): """Extract a column Vector. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.extract_col(0)) 0| 1| 2|149 >>> v = Vector.sparse(types.UINT64, M.ncols) >>> M.extract_col(0, out=v) is v True >>> print(v) 0| 1| 2|149 """ stop_val = self.ncols if T0 in (desc or ()) else self.nrows if out is None: out = Vector.sparse(self.type, stop_val) mask, accum, desc = self._get_args(mask, accum, desc) I, ni, size = _build_range(row_slice, stop_val) _check( self, lib.GrB_Col_extract( out._vector[0], mask, accum, self._matrix[0], I, ni, col_index, desc ), ) return out
def extract_row(self, row_index, col_slice=None, out=None, mask=None, accum=None, desc=None)
-
Extract a row Vector.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.extract_row(0)) 0| 1|42 2|
Expand source code
def extract_row( self, row_index, col_slice=None, out=None, mask=None, accum=None, desc=None ): """Extract a row Vector. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> print(M) 0 1 2 0| 42 | 0 1| 0| 1 2|149 | 2 0 1 2 >>> print(M.extract_row(0)) 0| 1|42 2| """ desc = desc & T0 if desc else T0 return self.extract_col( row_index, col_slice, out, desc=desc, mask=None, accum=None )
def assign_col(self, col_index, value, row_slice=None, mask=None, accum=None, desc=None)
-
Assign a vector to a column.
>>> M = Matrix.sparse(types.BOOL, 3, 3) >>> M.assign_col(1, Vector.from_lists([1, 2], [True, True], 3)) >>> print(M) 0 1 2 0| | 0 1| t | 1 2| t | 2 0 1 2
Expand source code
def assign_col( self, col_index, value, row_slice=None, mask=None, accum=None, desc=None ): """Assign a vector to a column. >>> M = Matrix.sparse(types.BOOL, 3, 3) >>> M.assign_col(1, Vector.from_lists([1, 2], [True, True], 3)) >>> print(M) 0 1 2 0| | 0 1| t | 1 2| t | 2 0 1 2 """ stop_val = self.ncols if T0 in (desc or ()) else self.nrows I, ni, size = _build_range(row_slice, stop_val) mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Col_assign( self._matrix[0], mask, accum, value._vector[0], I, ni, col_index, desc ), )
def assign_row(self, row_index, value, col_slice=None, mask=None, accum=None, desc=None)
-
Assign a vector to a row.
>>> M = Matrix.sparse(types.BOOL, 3, 3) >>> M.assign_row(1, Vector.from_lists([1, 2], [True, True], 3)) >>> print(M) 0 1 2 0| | 0 1| t t| 1 2| | 2 0 1 2
Expand source code
def assign_row( self, row_index, value, col_slice=None, mask=None, accum=None, desc=None ): """Assign a vector to a row. >>> M = Matrix.sparse(types.BOOL, 3, 3) >>> M.assign_row(1, Vector.from_lists([1, 2], [True, True], 3)) >>> print(M) 0 1 2 0| | 0 1| t t| 1 2| | 2 0 1 2 """ stop_val = self.nrows if T0 in (desc or ()) else self.ncols I, ni, size = _build_range(col_slice, stop_val) mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Row_assign( self._matrix[0], mask, accum, value._vector[0], row_index, I, ni, desc ), )
def assign_matrix(self, value, rindex=None, cindex=None, mask=None, accum=None, desc=None)
-
Assign a submatrix.
Note: The name for this method
Matrix.assign_matrix()
is deprecated, use the nameMatrix.assign_matrix()
instead.>>> M = Matrix.sparse(types.BOOL, 3, 3) >>> S = Matrix.sparse(types.BOOL, 3, 3) >>> S[1,1] = True >>> M.assign_matrix(S) >>> print(M) 0 1 2 0| | 0 1| t | 1 2| | 2 0 1 2
>>> M.clear()
Masked assignment with
M[key] = value
syntax can be done with if the index and value arguments are typeMatrix
:>>> M[S] = S >>> print(M) 0 1 2 0| | 0 1| t | 1 2| | 2 0 1 2
Expand source code
def assign_matrix( self, value, rindex=None, cindex=None, mask=None, accum=None, desc=None ): """Assign a submatrix. Note: The name for this method `Matrix.assign_matrix()` is deprecated, use the name `Matrix.assign()` instead. >>> M = Matrix.sparse(types.BOOL, 3, 3) >>> S = Matrix.sparse(types.BOOL, 3, 3) >>> S[1,1] = True >>> M.assign_matrix(S) >>> print(M) 0 1 2 0| | 0 1| t | 1 2| | 2 0 1 2 >>> M.clear() Masked assignment with `M[key] = value` syntax can be done with if the index and value arguments are type `Matrix`: >>> M[S] = S >>> print(M) 0 1 2 0| | 0 1| t | 1 2| | 2 0 1 2 """ I, ni, isize = _build_range(rindex, self.nrows - 1) J, nj, jsize = _build_range(cindex, self.ncols - 1) isize = self.nrows jsize = self.ncols mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_assign( self._matrix[0], mask, accum, value._matrix[0], I, ni, J, nj, desc ), )
def assign(self, value, rindex=None, cindex=None, mask=None, accum=None, desc=None)
-
Assign a submatrix.
Note: The name for this method
Matrix.assign_matrix()
is deprecated, use the nameMatrix.assign_matrix()
instead.>>> M = Matrix.sparse(types.BOOL, 3, 3) >>> S = Matrix.sparse(types.BOOL, 3, 3) >>> S[1,1] = True >>> M.assign_matrix(S) >>> print(M) 0 1 2 0| | 0 1| t | 1 2| | 2 0 1 2
>>> M.clear()
Masked assignment with
M[key] = value
syntax can be done with if the index and value arguments are typeMatrix
:>>> M[S] = S >>> print(M) 0 1 2 0| | 0 1| t | 1 2| | 2 0 1 2
Expand source code
def assign_matrix( self, value, rindex=None, cindex=None, mask=None, accum=None, desc=None ): """Assign a submatrix. Note: The name for this method `Matrix.assign_matrix()` is deprecated, use the name `Matrix.assign()` instead. >>> M = Matrix.sparse(types.BOOL, 3, 3) >>> S = Matrix.sparse(types.BOOL, 3, 3) >>> S[1,1] = True >>> M.assign_matrix(S) >>> print(M) 0 1 2 0| | 0 1| t | 1 2| | 2 0 1 2 >>> M.clear() Masked assignment with `M[key] = value` syntax can be done with if the index and value arguments are type `Matrix`: >>> M[S] = S >>> print(M) 0 1 2 0| | 0 1| t | 1 2| | 2 0 1 2 """ I, ni, isize = _build_range(rindex, self.nrows - 1) J, nj, jsize = _build_range(cindex, self.ncols - 1) isize = self.nrows jsize = self.ncols mask, accum, desc = self._get_args(mask, accum, desc) _check( self, lib.GrB_Matrix_assign( self._matrix[0], mask, accum, value._matrix[0], I, ni, J, nj, desc ), )
def assign_scalar(self, value, row_slice=None, col_slice=None, mask=None, accum=None, desc=None)
-
Assign a scalar
value
to the Matrix.>>> M = Matrix.sparse(types.BOOL, 3, 3)
The values of
row_slice
andcol_slice
determine what elements are assigned to the Matrix. The valueNone
maps to the GraphBLAS symbollib.GrB_ALL
, so the default behavior, with no other arguments, assigns the scalar to all elements:>>> M.assign_scalar(True) >>> print(M) 0 1 2 0| t t t| 0 1| t t t| 1 2| t t t| 2 0 1 2 >>> M.clear()
This is the same as the slice syntax with a bare colon:
>>> M[:,:] = True >>> print(M) 0 1 2 0| t t t| 0 1| t t t| 1 2| t t t| 2 0 1 2 >>> M.clear()
If
row_slice
orcol_slice
is an integer, use it as an index to one row or column:>>> M.assign_scalar(True, 1) >>> print(M) 0 1 2 0| | 0 1| t t t| 1 2| | 2 0 1 2 >>> M.clear()
An integer index and a scalar does row assignment:
>>> M[1] = True >>> print(M) 0 1 2 0| | 0 1| t t t| 1 2| | 2 0 1 2 >>> M.clear()
this is the same as the syntax:
>>> M[1,:] = True >>> print(M) 0 1 2 0| | 0 1| t t t| 1 2| | 2 0 1 2 >>> M.clear()
If
col_slice
is an integer, it does column assignment:>>> M.assign_scalar(True, None, 1) >>> print(M) 0 1 2 0| t | 0 1| t | 1 2| t | 2 0 1 2 >>> M.clear()
Which is the same as the syntax:
>>> M[:,1] = True >>> print(M) 0 1 2 0| t | 0 1| t | 1 2| t | 2 0 1 2 >>> M.clear()
Just an integer index does a row assignment:
>>> M.clear() >>> M[1] = Vector.from_lists([0,1], [True, True],3) >>> print(M) 0 1 2 0| | 0 1| t t | 1 2| | 2 0 1 2 >>> M.clear()
>>> M[0:1,0:1] = True >>> print(M) 0 1 2 0| t t | 0 1| t t | 1 2| | 2 0 1 2 >>> M.clear()
Expand source code
def assign_scalar( self, value, row_slice=None, col_slice=None, mask=None, accum=None, desc=None ): """Assign a scalar `value` to the Matrix. >>> M = Matrix.sparse(types.BOOL, 3, 3) The values of `row_slice` and `col_slice` determine what elements are assigned to the Matrix. The value `None` maps to the GraphBLAS symbol `lib.GrB_ALL`, so the default behavior, with no other arguments, assigns the scalar to all elements: >>> M.assign_scalar(True) >>> print(M) 0 1 2 0| t t t| 0 1| t t t| 1 2| t t t| 2 0 1 2 >>> M.clear() This is the same as the slice syntax with a bare colon: >>> M[:,:] = True >>> print(M) 0 1 2 0| t t t| 0 1| t t t| 1 2| t t t| 2 0 1 2 >>> M.clear() If `row_slice` or `col_slice` is an integer, use it as an index to one row or column: >>> M.assign_scalar(True, 1) >>> print(M) 0 1 2 0| | 0 1| t t t| 1 2| | 2 0 1 2 >>> M.clear() An integer index and a scalar does row assignment: >>> M[1] = True >>> print(M) 0 1 2 0| | 0 1| t t t| 1 2| | 2 0 1 2 >>> M.clear() this is the same as the syntax: >>> M[1,:] = True >>> print(M) 0 1 2 0| | 0 1| t t t| 1 2| | 2 0 1 2 >>> M.clear() If `col_slice` is an integer, it does column assignment: >>> M.assign_scalar(True, None, 1) >>> print(M) 0 1 2 0| t | 0 1| t | 1 2| t | 2 0 1 2 >>> M.clear() Which is the same as the syntax: >>> M[:,1] = True >>> print(M) 0 1 2 0| t | 0 1| t | 1 2| t | 2 0 1 2 >>> M.clear() Just an integer index does a row assignment: >>> M.clear() >>> M[1] = Vector.from_lists([0,1], [True, True],3) >>> print(M) 0 1 2 0| | 0 1| t t | 1 2| | 2 0 1 2 >>> M.clear() >>> M[0:1,0:1] = True >>> print(M) 0 1 2 0| t t | 0 1| t t | 1 2| | 2 0 1 2 >>> M.clear() """ mask, accum, desc = self._get_args(mask, accum, desc) if row_slice is not None: if isinstance(row_slice, int): I, ni, isize = _build_range(slice(row_slice, row_slice), self.nrows - 1) else: I, ni, isize = _build_range(row_slice, self.nrows - 1) else: I = lib.GrB_ALL ni = 0 if col_slice is not None: if isinstance(col_slice, int): J, nj, jsize = _build_range(slice(col_slice, col_slice), self.ncols - 1) else: J, nj, jsize = _build_range(col_slice, self.ncols - 1) else: J = lib.GrB_ALL nj = 0 scalar_type = types._gb_from_type(type(value)) _check( self, scalar_type._Matrix_assignScalar( self._matrix[0], mask, accum, value, I, ni, J, nj, desc ), )
def get(self, i, j, default=None)
-
Get the element at row
i
colj
or return the default value if the element is not present.>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.get(1, 2) 0 >>> M.get(0, 0) is None True >>> M.get(0, 0, 'foo') 'foo'
Expand source code
def get(self, i, j, default=None): """Get the element at row `i` col `j` or return the default value if the element is not present. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.get(1, 2) 0 >>> M.get(0, 0) is None True >>> M.get(0, 0, 'foo') 'foo' """ try: return self[i, j] except NoValue: return default
def wait(self)
-
Wait for this Matrix to complete before allowing another thread to change it.
Expand source code
def wait(self): """Wait for this Matrix to complete before allowing another thread to change it. """ _check(self, lib.GrB_Matrix_wait(self._matrix))
def to_markdown_table(self, title='A', width=2)
-
Return a string markdown table representation of the Matrix.
>>> M = Matrix.from_lists([0, 0, 1, 2], [1, 2, 2, 0], [42, 2, 0, 149]) >>> print(M.to_markdown_table()) A|0|1|2 ---|---|---|--- 0| |42| 2 1| | | 0 2| 149| |
Expand source code
def to_markdown_table(self, title="A", width=2): """Return a string markdown table representation of the Matrix. >>> M = Matrix.from_lists([0, 0, 1, 2], [1, 2, 2, 0], [42, 2, 0, 149]) >>> print(M.to_markdown_table()) A|0|1|2 ---|---|---|--- 0| |42| 2 1| | | 0 2| 149| | """ rows = set(self.rows) cols = set(self.cols) result = f"""\ {title}|{'|'.join(map(str, cols))} ---|{"|".join(['---'] * len(cols))} """ for i, row in enumerate(rows): result += f"{row}| " + "|".join( self.type.format_value(self.get(row, col, ""), width) for col in cols ) if i != len(rows) - 1: result += "\n" return result.rstrip()
def to_html_table(self, title='A', width=2)
-
Return a string markdown table representation of the Matrix.
>>> M = Matrix.from_lists([0, 0, 1, 2], [1, 2, 2, 0], [42, 2, 0, 149]) >>> print(M.to_html_table()) <table> <th>A</th> <th>0</th> <th>1</th> <th>2</th> <BLANKLINE> <tr> <th>0</th> <td> </td> <td>42</td> <td> 2</td> </tr> <BLANKLINE> <tr> <th>1</th> <td> </td> <td> </td> <td> 0</td> </tr> <BLANKLINE> <tr> <th>2</th> <td>149</td> <td> </td> <td> </td> </tr> </table>
Expand source code
def to_html_table(self, title="A", width=2): """Return a string markdown table representation of the Matrix. >>> M = Matrix.from_lists([0, 0, 1, 2], [1, 2, 2, 0], [42, 2, 0, 149]) >>> print(M.to_html_table()) <table> <th>A</th> <th>0</th> <th>1</th> <th>2</th> <BLANKLINE> <tr> <th>0</th> <td> </td> <td>42</td> <td> 2</td> </tr> <BLANKLINE> <tr> <th>1</th> <td> </td> <td> </td> <td> 0</td> </tr> <BLANKLINE> <tr> <th>2</th> <td>149</td> <td> </td> <td> </td> </tr> </table> """ from mako.template import Template t = Template( """\ <% rows = set(A.rows) cols = set(A.cols) %><table> <th>${title}</th> % for col in cols: <th>${col}</th> % endfor % for row in rows: ${makerow(row)} % endfor </table><%def name="makerow(row)"> <tr> <th>${row}</th> % for col in cols: <td>${A.type.format_value(A.get(row, col, ''))}</td> % endfor </tr></%def>""" ) return t.render(A=self, title=title)
def print(self, level=2, name='A', f=sys.stdout)
-
Print the matrix using
GxB_Matrix_fprint()
, by default tosys.stdout
..Level 1: Short description Level 2: Short list, short numbers Level 3: Long list, short number Level 4: Short list, long numbers Level 5: Long list, long numbers
Expand source code
def print(self, level=2, name="A", f=sys.stdout): # pragma: nocover """Print the matrix using `GxB_Matrix_fprint()`, by default to `sys.stdout`.. Level 1: Short description Level 2: Short list, short numbers Level 3: Long list, short number Level 4: Short list, long numbers Level 5: Long list, long numbers """ _check( self, lib.GxB_Matrix_fprint(self._matrix[0], bytes(name, "utf8"), level, f) )
def to_string(self, format_string='{:>%s}', width=3, prec=5, empty_char='', cell_sep='')
-
Return a string representation of the Matrix.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.to_string() ' 0 1 2\n 0| 42 | 0\n 1| 0| 1\n 2|149 | 2\n 0 1 2'
Expand source code
def to_string( self, format_string="{:>%s}", width=3, prec=5, empty_char="", cell_sep="" ): """Return a string representation of the Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.to_string() ' 0 1 2\\n 0| 42 | 0\\n 1| 0| 1\\n 2|149 | 2\\n 0 1 2' """ format_string = format_string % width header = ( format_string.format("") + " " + "".join(format_string.format(i) for i in range(self.ncols)) ) result = header + "\n" for row in range(self.nrows): result += format_string.format(row) + "|" for col in range(self.ncols): value = self.get(row, col, empty_char) result += cell_sep + self.type.format_value(value, width, prec) result += "| " + str(row) + "\n" result += header return result
def to_scipy_sparse(self, format='csr')
-
Return a scipy sparse matrix of this Matrix.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.to_scipy_sparse() <3x3 sparse matrix of type '<class 'numpy.int64'>'...
Expand source code
def to_scipy_sparse(self, format="csr"): """Return a scipy sparse matrix of this Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.to_scipy_sparse() <3x3 sparse matrix of type '<class 'numpy.int64'>'... """ from scipy import sparse rows, cols, vals = self.to_arrays() s = sparse.coo_matrix( (vals, (rows, cols)), shape=self.shape, dtype=self.type._numpy_t ) if format == "coo": return s if format not in {"bsr", "csr", "csc", "coo", "lil", "dia", "dok"}: raise TypeError(f"Invalid format: {format}") return s.asformat(format)
def to_numpy(self)
-
Return a dense numpy matrix of this Matrix.
>>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.to_numpy() array([[ 0, 42, 0], [ 0, 0, 0], [149, 0, 0]], dtype=int64)
Expand source code
def to_numpy(self): """Return a dense numpy matrix of this Matrix. >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [42, 0, 149]) >>> M.to_numpy() array([[ 0, 42, 0], [ 0, 0, 0], [149, 0, 0]], dtype=int64) """ s = self.to_scipy_sparse("coo") return s.toarray()
def out_degree(self, typ=pygraphblas.types.UINT64, out=None)
-
Return a UINT64 vector of the out-degree of this graph:
>>> M = Matrix.from_lists([0, 1, 0, 2], [1, 2, 2, 0], [42, 0, 3, 149]) >>> print(M.out_degree()) 0| 2 1| 1 2| 1
Expand source code
def out_degree(self, typ=types.UINT64, out=None): """Return a UINT64 vector of the out-degree of this graph: >>> M = Matrix.from_lists([0, 1, 0, 2], [1, 2, 2, 0], [42, 0, 3, 149]) >>> print(M.out_degree()) 0| 2 1| 1 2| 1 """ return self.cast(typ).plus_pair(Vector.iso(1, self.nrows), out=out)
def gini(self, typ=pygraphblas.types.FP64)
-
Calculate the Gini coefficient of the graph.
>>> M = Matrix.random(types.UINT8, 10, 10, 10, seed=42) >>> M.gini() 0.23333333333333334
>>> M = Matrix.random(types.UINT8, 100, 10, 10, seed=42) >>> M.gini() 0.0967741935483871
>>> M = Matrix.random(types.UINT8, 10000, 100, 100, seed=42) >>> M.gini() 0.0483808618504436
>>> M = Matrix.dense(types.UINT8, 100, 100) >>> M.gini() 0.0
Expand source code
def gini(self, typ=types.FP64): """Calculate the Gini coefficient of the graph. >>> M = Matrix.random(types.UINT8, 10, 10, 10, seed=42) >>> M.gini() 0.23333333333333334 >>> M = Matrix.random(types.UINT8, 100, 10, 10, seed=42) >>> M.gini() 0.0967741935483871 >>> M = Matrix.random(types.UINT8, 10000, 100, 100, seed=42) >>> M.gini() 0.0483808618504436 >>> M = Matrix.dense(types.UINT8, 100, 100) >>> M.gini() 0.0 """ array = self.out_degree(typ).npV array.sort() n = array.shape[0] index = np.arange(1, n + 1) return (np.sum((2 * index - n - 1) * array)) / (n * np.sum(array))
class Vector (vec, typ=None)
-
GraphBLAS Sparse Vector
This is a high-level wrapper around the low-level GrB_Vector type.
A Vector supports many possible operations according to the GraphBLAS API. Many of those operations have overloaded operators.
Operator Description Default v @ A Vector Vector Multiplication type default PLUS_TIMES semiring v @= A In-place Vector Vector Multiplication type default PLUS_TIMES semiring v | w Vector Union type default SECOND combiner v |= w In-place Vector Union type default SECOND combiner v & w Vector Intersection type default SECOND combiner v &= w In-place Vector Intersection type default SECOND combiner v + w Vector Element-Wise Union type default PLUS combiner v += w In-place Vector Element-Wise Union type default PLUS combiner v - w Vector Element-Wise Union type default MINUS combiner v -= w In-place Vector Element-Wise Union type default MINUS combiner v * w Vector Element-Wise Intersection type default TIMES combiner v *= w In-place Vector Element-Wise Intersection type default TIMES combiner v / w Vector Element-Wise Intersection type default DIV combiner v /= w In-place Vector Element-Wise Intersection type default DIV combiner v == w Compare Element-Wise Union type default EQ operator v != w Compare Element-Wise Union type default NE operator v < w Compare Element-Wise Union type default LT operator v > w Compare Element-Wise Union type default GT operator v <= w Compare Element-Wise Union type default LE operator v >= w Compare Element-Wise Union type default GE operator Note that all the above operator syntax is mearly sugar over various combinations of calling
Matrix.mxv()
,Vector.vxm()
,Vector.eadd()
, andVector.emult()
.Expand source code
class Vector: """GraphBLAS Sparse Vector This is a high-level wrapper around the low-level GrB_Vector type. A Vector supports many possible operations according to the GraphBLAS API. Many of those operations have overloaded operators. Operator | Description | Default --- | --- | --- v @ A | Vector Vector Multiplication | type default PLUS_TIMES semiring v @= A | In-place Vector Vector Multiplication | type default PLUS_TIMES semiring v \\| w | Vector Union | type default SECOND combiner v \\|= w | In-place Vector Union | type default SECOND combiner v & w | Vector Intersection | type default SECOND combiner v &= w | In-place Vector Intersection | type default SECOND combiner v + w | Vector Element-Wise Union | type default PLUS combiner v += w | In-place Vector Element-Wise Union | type default PLUS combiner v - w | Vector Element-Wise Union | type default MINUS combiner v -= w | In-place Vector Element-Wise Union | type default MINUS combiner v * w | Vector Element-Wise Intersection | type default TIMES combiner v *= w | In-place Vector Element-Wise Intersection | type default TIMES combiner v / w | Vector Element-Wise Intersection | type default DIV combiner v /= w | In-place Vector Element-Wise Intersection | type default DIV combiner v == w | Compare Element-Wise Union | type default EQ operator v != w | Compare Element-Wise Union | type default NE operator v < w | Compare Element-Wise Union | type default LT operator v > w | Compare Element-Wise Union | type default GT operator v <= w | Compare Element-Wise Union | type default LE operator v >= w | Compare Element-Wise Union | type default GE operator Note that all the above operator syntax is mearly sugar over various combinations of calling `Matrix.mxv`, `Vector.vxm`, `Vector.eadd`, and `Vector.emult`. """ __slots__ = ("_vector", "type", "_keep_alives") def _check(self, res): if res != lib.GrB_SUCCESS: error_string = ffi.new("char**") lib.GrB_Vector_error(error_string, self._vector[0]) raise _error_codes[res](ffi.string(error_string[0])) def __init__(self, vec, typ=None): if typ is None: new_type = ffi.new("GrB_Type*") self._check(lib.GxB_Vector_type(new_type, vec[0])) typ = types._gb_type_to_type(new_type[0]) self._vector = vec self.type = typ self._keep_alives = weakref.WeakKeyDictionary() def __del__(self): self._check(lib.GrB_Vector_free(self._vector)) def __len__(self): return self.nvals def __iter__(self): nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = ffi.new("GrB_Index[%s]" % nvals) X = ffi.new("%s[%s]" % (self.type._c_type, nvals)) self._check(self.type._Vector_extractTuples(I, X, _nvals, self._vector[0])) return zip(I, X) def __getattr__(self, name): """Look up operators as attributes for the given object.""" return partial(getattr(self.type, name), self) @property def indices(self): """cdata array of vector indexes. >>> v = Vector.from_1_to_n(3) >>> list(v.indices) [0, 1, 2] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = ffi.new("GrB_Index[%s]" % nvals) X = NULL self._check(self.type._Vector_extractTuples(I, X, _nvals, self._vector[0])) return I @property def I(self): """Iterator over for `Vector.indices`. >>> v = Vector.from_1_to_n(3) >>> list(v.I) [0, 1, 2] """ return iter(self.indices) @property def npI(self): """numpy array over `Vector.indices`. >>> v = Vector.from_1_to_n(3) >>> v.npI array([0, 1, 2], dtype=uint64) """ return np.frombuffer(ffi.buffer(self.indices), dtype=np.uint64) @property def vals(self): """Iterator of vector values. >>> v = Vector.from_1_to_n(3) >>> list(v.vals) [1, 2, 3] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = NULL V = ffi.new("%s[%s]" % (self.type._c_type, nvals)) self._check(self.type._Vector_extractTuples(I, V, _nvals, self._vector[0])) return V @property def V(self): """Iterator over for `Vector.vals`. >>> v = Vector.from_1_to_n(3) >>> list(v.V) [1, 2, 3] """ return iter(self.vals) @property def npV(self): """numpy array over `Vector.vals`. >>> v = Vector.from_1_to_n(3) >>> v.npV array([1, 2, 3]) """ return np.frombuffer(ffi.buffer(self.vals), dtype=self.type._numpy_t) def all(self, other, op): """Do all elements in self compare True with op to other? >>> from . import INT64 >>> M = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> N = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> O = Vector.from_lists([0, 1], [1, 2]) >>> P = Vector.from_lists([0, 1], [1, 2], size=3) >>> Q = Vector.from_lists([0, 1, 3], [1, 2, 3]) >>> assert M.all(N, INT64.eq) >>> assert not M.all(N, INT64.gt) >>> assert not M.all(O, INT64.eq) >>> assert not M.all(P, INT64.eq) >>> assert not M.all(Q, INT64.eq) """ if self.size != other.size: return False if self.nvals != other.nvals: return False C = self.emult(other, op, cast=types.BOOL) if C.nvals != self.nvals: # pragma: nocover return False return C.reduce_bool(types.BOOL.land_monoid) def iseq(self, other, eq_op=None): """Compare two vectors for equality. Note to be confused with the `==` operator which does element-wise comparison and returns a `Vector`. >>> v = Vector.from_lists([0,1], [1, 1]) >>> w = Vector.from_lists([0,1], [1, 1]) >>> x = Vector.from_lists([0,1], [1.0, 1.0]) >>> v.iseq(w) True >>> v.iseq(w, eq_op=types.UINT64.GE) True >>> v.iseq(x) False """ if self.type != other.type: return False if eq_op is None: eq_op = self.type.EQ return self.all(other, eq_op) def isne(self, other): """Compare two vectors for inequality. Note to be confused with the `==` operator which does element-wise comparison and returns a `Vector`. >>> v = Vector.from_lists([0,1], [1, 1]) >>> w = Vector.from_lists([0,1], [1, 1]) >>> v.isne(w) False """ return not self.iseq(other) @classmethod def sparse(cls, typ, size=None, fill=None, mask=None): """Create an empty Vector from the given type. If `size` is not specified it defaults to `pygraphblas.GxB_INDEX_MAX`. >>> v = Vector.sparse(types.INT64, 3) >>> v <Vector(INT64 size: 3, nvals: 0)> >>> v.size 3 >>> v = Vector.sparse(types.INT64) >>> v <Vector(INT64, nvals: 0)> >>> v.size == lib.GxB_INDEX_MAX True >>> v[42] = True >>> w = Vector.sparse(types.INT64, fill=42, mask=v) >>> list(w) [(42, 42)] If no `fill` is provided, the `type.default_zero` is used: >>> w = Vector.sparse(types.INT64, mask=v) >>> list(w) [(42, 0)] """ if size is None: size = GxB_INDEX_MAX new_vec = ffi.new("GrB_Vector*") _check(lib.GrB_Vector_new(new_vec, typ._gb_type, size)) m = cls(new_vec, typ) if mask is not None: if fill is None: fill = m.type.default_zero m.assign_scalar(fill, mask=mask) return m @classmethod def random( cls, typ, nvals, size=lib.GxB_INDEX_MAX, make_pattern=False, seed=None, ): # pragma: nocover """ """ V = Vector.sparse(typ, size) if seed is not None: random.seed(seed) if V.size == 0: nvals = 0 if typ is types.BOOL: f = partial(random.randint, 0, 1) if typ is types.UINT8: f = partial(random.randint, 0, (2 ** 8) - 1) if typ is types.UINT16: f = partial(random.randint, 0, (2 ** 16) - 1) if typ is types.UINT32: f = partial(random.randint, 0, (2 ** 32) - 1) if typ is types.UINT64: f = partial(random.randint, 0, (2 ** 64) - 1) if typ is types.INT8: f = partial(random.randint, (-(2 ** 7)) + 1, (2 ** 7) - 1) if typ is types.INT16: f = partial(random.randint, (-(2 ** 15)) + 1, (2 ** 15) - 1) if typ is types.INT32: f = partial(random.randint, (-(2 ** 31)) + 1, (2 ** 31) - 1) if typ is types.INT64: f = partial(random.randint, (-(2 ** 63)) + 1, (2 ** 63) - 1) if typ in (types.FP32, types.FP64): f = random.random if typ in (types.FC32, types.FC64): f = lambda: complex(random.random(), random.random()) for i in range(nvals): i = random.randint(0, V.size - 1) V[i] = f() return V @classmethod def from_lists(cls, I, V, size=None, typ=None): """Create a new vector from the given lists of indices and values. If size is not provided, it is computed from the max values of the provides size indices. If the second argument is a scalar value, an "iso" vector is created where all values equal that scalar. >>> v = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> w = Vector.from_lists([0, 1, 2], True) >>> assert not v.iseq(w) >>> assert v.pattern().iseq(w) """ if isinstance(V, (bool, int, float)): V = [V] * len(I) assert len(I) == len(V) assert len(I) > 0 # must be non empty if not size: size = max(I) + 1 # TODO option to use ffi and GrB_Vector_build if typ is None: typ = types._gb_from_type(type(V[0])) m = cls.sparse(typ, size) for i, v in zip(I, V): m[i] = v return m @classmethod def from_list(cls, I): """Create a new dense vector from the given lists of values.""" size = len(I) assert size > 0 # TODO use ffi and GrB_Vector_build m = cls.sparse(types._gb_from_type(type(I[0])), size) for i, v in enumerate(I): m[i] = v return m @classmethod def from_1_to_n(cls, n): """Generate a vector from 1 to n. >>> v = Vector.from_1_to_n(3) >>> print(v) 0| 1 1| 2 2| 3 """ v = cls.sparse(types.INT64, n) for i in range(n): v[i] = i + 1 return v def dup(self): """Create an duplicate Vector from the given argument. >>> v = Vector.from_1_to_n(3) >>> w = v.dup() >>> w is not v True >>> w.iseq(v) True >>> print(w) 0| 1 1| 2 2| 3 """ new_vec = ffi.new("GrB_Vector*") self._check(lib.GrB_Vector_dup(new_vec, self._vector[0])) return self.__class__(new_vec, self.type) @property def hyper_switch(self): # pragma: nocover """Get the hyper_switch threshold. (See SuiteSparse User Guide)""" switch = ffi.new("double*") self._check( lib.GxB_Vector_Option_get(self._vector[0], lib.GxB_HYPER_SWITCH, switch) ) return switch[0] @hyper_switch.setter def hyper_switch(self, switch): # pragma: nocover """Set the hyper_switch threshold. (See SuiteSparse User Guide)""" switch = ffi.cast("double", switch) self._check( lib.GxB_Vector_Option_set(self._vector[0], lib.GxB_HYPER_SWITCH, switch) ) @property def sparsity(self): # pragma: nocover """Get Vector sparsity control. (See SuiteSparse User Guide)""" sparsity = ffi.new("int*") self._check( lib.GxB_Vector_Option_get( self._vector[0], lib.GxB_SPARSITY_CONTROL, sparsity ) ) return sparsity[0] @sparsity.setter def sparsity(self, sparsity): # pragma: nocover """Set Vector sparsity control. (See SuiteSparse User Guide)""" sparsity = ffi.cast("int", sparsity) self._check( lib.GxB_Vector_Option_set( self._vector[0], lib.GxB_SPARSITY_CONTROL, sparsity ) ) @property def sparsity_status(self): # pragma: nocover """Get Vector sparsity status. (See SuiteSparse User Guide)""" status = ffi.new("int*") self._check( lib.GxB_Vector_Option_get(self._vector[0], lib.GxB_SPARSITY_STATUS, status) ) return status[0] @classmethod def dense(cls, typ, size=None, fill=None): """Return a dense vector of `typ` and `size`. If `fill` is provided, use that value otherwise use `self.type.default_zero` >>> print(Vector.dense(types.FP32, 3)) 0|0.0 1|0.0 2|0.0 >>> print(Vector.dense(types.FP32, 3, fill=42.0)) 0|42.0 1|42.0 2|42.0 """ v = cls.sparse(typ, size) if fill is None: fill = v.type.default_zero v[:] = fill return v @classmethod def iso(cls, value, size=GxB_INDEX_MAX): """Build an "iso" vector from a scalar value. This is similar to `Vector.dense` but infers the type of the new Matrix from the provided vbalue. >>> v = Vector.iso(3) >>> assert v[42] == 3 """ typ = types._gb_from_type(type(value)) return cls.dense(typ, size, value) def to_lists(self): """Extract the indices and values of the Vector as 2 lists. >>> Vector.from_1_to_n(3).to_lists() [[0, 1, 2], [1, 2, 3]] """ I = ffi.new("GrB_Index[]", self.nvals) V = self.type._ffi.new(self.type._c_type + "[]", self.nvals) n = ffi.new("GrB_Index*") n[0] = self.nvals self._check(self.type._Vector_extractTuples(I, V, n, self._vector[0])) return [list(I), list(map(self.type._to_value, V))] def to_arrays(self): """Return as python `array` objects. >>> Vector.from_1_to_n(3).to_arrays() (array('L', [0, 1, 2]), array('q', [1, 2, 3])) """ if self.type._typecode is None: raise TypeError("This matrix has no array typecode.") nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = ffi.new("GrB_Index[%s]" % nvals) X = self.type._ffi.new("%s[%s]" % (self.type._c_type, nvals)) self._check(self.type._Vector_extractTuples(I, X, _nvals, self._vector[0])) return array("L", I), array(self.type._typecode, X) @property def size(self): """Return the size of the vector. >>> Vector.from_1_to_n(3).size 3 """ n = ffi.new("GrB_Index*") self._check(lib.GrB_Vector_size(n, self._vector[0])) return n[0] @property def nvals(self): """Return the number of values in the vector. >>> v = Vector.from_1_to_n(3) >>> v.nvals 3 >>> v.clear() >>> v.nvals 0 """ n = ffi.new("GrB_Index*") self._check(lib.GrB_Vector_nvals(n, self._vector[0])) return n[0] @property def memory_usage(self): """Returns the memory usage of the Vector. >>> v = Vector.from_lists([0, 1, 2], [1, 2, 0]) >>> assert v.memory_usage > 0 """ n = ffi.new("size_t*") self._check(lib.GxB_Vector_memoryUsage(n, self._vector[0])) return n[0] @property def gb_type(self): """Return the GraphBLAS low-level type object of the Vector.""" return self.type._gb_type def _full(self): B = self.__class__.sparse(self.type, self.size) self._check( self.type._Vector_assignScalar( B._vector[0], NULL, NULL, self.type.default_one, lib.GrB_ALL, 0, NULL ) ) return self.eadd(B, self.type.FIRST) def _compare(self, other, op, strop): C = self.__class__.sparse(types.BOOL, self.size) if isinstance(other, (bool, int, float, complex)): if op(other, 0): B = self.__class__.dup(self) B[:] = other self.emult(B, strop, out=C) return C else: self.select(strop, other).apply(types.BOOL.ONE, out=C) return C elif isinstance(other, Vector): A = self._full() B = other._full() A.emult(B, strop, out=C) return C else: raise NotImplementedError def __gt__(self, other): return self._compare(other, operator.gt, ">") def __lt__(self, other): return self._compare(other, operator.lt, "<") def __ge__(self, other): return self._compare(other, operator.ge, ">=") def __le__(self, other): return self._compare(other, operator.le, "<=") def __eq__(self, other): return self._compare(other, operator.eq, "==") def __ne__(self, other): return self._compare(other, operator.ne, "!=") def eadd( self, other, add_op=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Element-wise addition with other vector. Element-wise addition applies a binary operator element-wise on two vectors `v` and `w`, for all entries that appear in the set union of the patterns of `A` and `B`. The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation. >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> V = list(range(len(I))) >>> v = Vector.from_lists(I, V, 7) >>> w = Vector.from_lists( ... [0, 1, 4, 6], ... [9, 1, 4, 7], 7) >>> print(v.eadd(w)) 0|10 1| 4 2| 4 3| 6 4|11 5| 8 6|18 This can also be accomplished with the `+` operators: >>> print(v + w) 0|10 1| 4 2| 4 3| 6 4|11 5| 8 6|18 The combining operator used can be provided either as a context manager or passed to `mxv` as the `add_op` argument. >>> with types.INT64.MIN: ... print(v + w) 0| 1 1| 1 2| 4 3| 6 4| 4 5| 8 6| 7 You can provide a monoid for the operation: >>> print(v.eadd(w, v.type.min_monoid)) 0| 1 1| 1 2| 4 3| 6 4| 4 5| 8 6| 7 Or you can use a semiring: >>> print(v.eadd(w, v.type.min_plus)) 0| 1 1| 1 2| 4 3| 6 4| 4 5| 8 6| 7 The following operators default to use `eadd`: Operator | Description | Default --- | --- | --- v \\| w | Vector Union | type default SECOND combiner v \\|= w | In-place Vector Union | type default SECOND combiner v + w | Vector Element-Wise Union | type default PLUS combiner v += w | In-place Vector Element-Wise Union | type default PLUS combiner v - w | Vector Element-Wise Union | type default MINUS combiner v -= w | In-place Vector Element-Wise Union | type default MINUS combiner """ func = lib.GrB_Vector_eWiseAdd_BinaryOp if add_op is None: add_op = current_binop.get(NULL) elif isinstance(add_op, Monoid): func = lib.GrB_Vector_eWiseAdd_Monoid elif isinstance(add_op, Semiring): func = lib.GrB_Vector_eWiseAdd_Semiring mask, accum, desc = self._get_args(mask, accum, desc) if out is None: typ = cast or types.promote(self.type, other.type) _out = ffi.new("GrB_Vector*") self._check(lib.GrB_Vector_new(_out, typ._gb_type, self.size)) out = self.__class__(_out, typ) if add_op is NULL: add_op = out.type._default_addop() add_op = add_op.get_op() self._check( func( out._vector[0], mask, accum, add_op, self._vector[0], other._vector[0], desc, ) ) return out def emult( self, other, mult_op=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Element-wise multiplication with other vector. Element-wise multiplication applies a binary operator element-wise on two vectors A and B, for all entries that appear in the set intersection of the patterns of A and B. The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation. >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> V = list(range(len(I))) >>> v = Vector.from_lists(I, V, 7) >>> w = Vector.from_lists( ... [0, 1, 4, 6], ... [9, 1, 4, 7], 7) >>> print(v.emult(w)) 0| 9 1| 3 2| 3| 4|28 5| 6|77 This can also be accomplished with the `+` operators: >>> print(v * w) 0| 9 1| 3 2| 3| 4|28 5| 6|77 The combining operator used can be provided either as a context manager or passed to `mxv` as the `add_op` argument. >>> with types.INT64.MAX: ... print(v * w) 0| 9 1| 3 2| 3| 4|28 5| 6|77 """ if mult_op is None: mult_op = current_binop.get(NULL) elif isinstance(mult_op, str): mult_op = _get_bin_op(mult_op, self.type) mask, accum, desc = self._get_args(mask, accum, desc) if out is None: typ = cast or types.promote(self.type, other.type) _out = ffi.new("GrB_Vector*") self._check(lib.GrB_Vector_new(_out, typ._gb_type, self.size)) out = self.__class__(_out, typ) if mult_op is NULL: mult_op = out.type._default_multop() mult_op = mult_op.get_op() self._check( lib.GrB_Vector_eWiseMult_BinaryOp( out._vector[0], mask, accum, mult_op, self._vector[0], other._vector[0], desc, ) ) return out def vxm( self, other, semiring=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Vector-Matrix multiply. Multiply this row vector by `other` matrix "on the left". For column matrix/vector multiplication "on the right" see `Matrix.mxv`. `vxm` can also be called directly or with the `@` operator: >>> from . import Matrix, INT64 >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> v = Vector.from_lists([0, 1, 2], [2, 3, 4]) >>> o = v.vxm(M) >>> print(o) 0|12 1| 2 2| 6 >>> o = v @ M >>> print(o) 0|12 1| 2 2| 6 By default, `mxv` and `@` create a new result matrix of the correct type and dimensions if one is not provided. If you want to provide your own matrix to put the result in, you can pass it in the `out` parameter. This is useful for accumulating results into a single matrix with minimal copying. This is also supported by the `@=` syntax: >>> o = v.dup() >>> v.vxm(M, accum=INT64.plus, out=o) is o True >>> print(o) 0|14 1| 5 2|10 >>> o = v.dup() >>> with Accum(INT64.min): ... o @= M >>> print(o) 0| 2 1| 2 2| 4 The default semiring depends on the infered result type. In the case of numbers, the default semiring is `PLUS_TIMES`. In the case of type `BOOL`, it is `BOOL.lor_land`. >>> o = v.vxm(M, semiring=INT64.min_plus) >>> print(o) 0| 7 1| 3 2| 5 An explicit semiring can be passed to the method or provided with a context manager: >>> with INT64.min_plus: ... o = v @ M >>> print(o) 0| 7 1| 3 2| 5 Or the semiring can be accessed via an attribute on the vector: >>> o = v.min_plus(M) >>> print(o) 0| 7 1| 3 2| 5 Descriptors and accumulators can also be provided as an argument or a context manager: >>> o = v.vxm(M, desc=descriptor.T0) >>> print(o) 0|12 1| 2 2| 6 >>> with descriptor.T0: ... o = v @ M >>> print(o) 0|12 1| 2 2| 6 >>> del o[1] >>> o = v.vxm(M, mask=o) >>> print(o) 0|12 1| 2| 6 """ if semiring is None: semiring = current_semiring.get(NULL) if out is None: new_dimension = other.nrows if T1 in (desc or ()) else other.ncols if semiring is not NULL: typ = semiring.ztype else: typ = cast or types.promote(self.type, other.type) out = Vector.sparse(typ, new_dimension) else: typ = out.type if semiring is NULL: semiring = out.type._default_semiring() semiring = semiring.get_op() mask, accum, desc = self._get_args(mask, accum, desc) self._check( lib.GrB_vxm( out._vector[0], mask, accum, semiring, self._vector[0], other._matrix[0], desc, ) ) return out def __matmul__(self, other): return self.vxm(other) def __imatmul__(self, other): return self.vxm(other, out=self) def __and__(self, other): return self.emult(other) def __iand__(self, other): return self.emult(other, out=self) def __or__(self, other): return self.eadd(other) def __ior__(self, other): return self.eadd(other, out=self) def __add__(self, other): if not isinstance(other, Vector): return self.apply_second(self.type.PLUS, other) return self.eadd(other) def __radd__(self, other): if not isinstance(other, Vector): return self.apply_first(other, self.type.PLUS) return other.eadd(self) # pragma: nocover def __iadd__(self, other): if not isinstance(other, Vector): return self.apply_second(self.type.PLUS, other, out=self) return self.eadd(other, out=self) def __sub__(self, other): if not isinstance(other, Vector): return self.apply_second(self.type.MINUS, other) return self.eadd(other, self.type.MINUS) def __rsub__(self, other): if not isinstance(other, Vector): return self.apply_first(other, self.type.MINUS) return other.eadd(self, self.type.MINUS) # pragma: nocover def __isub__(self, other): if not isinstance(other, Vector): return self.apply_second(self.type.MINUS, other) return other.eadd(self, self.type.MINUS, out=self) def __mul__(self, other): if not isinstance(other, Vector): return self.apply_second(self.type.TIMES, other) return self.emult(other, self.type.TIMES) def __rmul__(self, other): if not isinstance(other, Vector): return self.apply_first(other, self.type.TIMES) return other.emult(self, add_op=self.type.TIMES) # pragma: nocover def __imul__(self, other): if not isinstance(other, Vector): return self.apply_second(self.type.TIMES, other, out=self) return other.emult(self, self.type.TIMES, out=self) def __truediv__(self, other): if not isinstance(other, Vector): return self.apply_second(self.type.DIV, other) return self.emult(other, self.type.DIV) def __rtruediv__(self, other): if not isinstance(other, Vector): return self.apply_first(other, self.type.DIV) return other.emult(self, self.type.DIV) # pragma: nocover def __itruediv__(self, other): if not isinstance(other, Vector): return self.apply_second(self.type.DIV, other, out=self) return other.emult(self, self.type.DIV, out=self) def __invert__(self): return self.apply(self.type.MINV) def __neg__(self): return self.apply(self.type.AINV) def __abs__(self): return self.apply(self.type.ABS) def clear(self): """Clear this vector removing all entries.""" self._check(lib.GrB_Vector_clear(self._vector[0])) def resize(self, size=lib.GxB_INDEX_MAX): """Resize the vector. If the dimensions decrease, entries that fall outside the resized vector are deleted. >>> v = Vector.dense(types.UINT8, 2) >>> v.resize(3) >>> print(v) 0| 0 1| 0 2| """ self._check(lib.GrB_Vector_resize(self._vector[0], size)) def _get_args(self, mask=None, accum=None, desc=None): if accum is None: accum = current_accum.get(NULL) if accum is not NULL: accum = accum.get_op() if desc is None: desc = current_desc.get(NULL) if desc is not NULL: desc = desc.get_desc() if mask is None: mask = NULL # else: # if desc is None: # desc = S if isinstance(mask, Vector): mask = mask._vector[0] return mask, accum, desc def reduce(self, mon=None, accum=None, desc=None): """Do a scalar reduce based on this object's type: >>> V = Vector.random(types.UINT8, 10, 3, seed=42) >>> V.reduce() 114 >>> V = Vector.random(types.FP32, 10, 3, seed=42) >>> V.reduce() 0.9517456293106079 >>> V = Vector.random(types.UINT8, 10, 3, seed=42) >>> V.reduce(V.type.min_monoid) 13 >>> V = Vector.random(types.BOOL, 10, 3, seed=42) >>> V.reduce() False """ if mon is None: if self.type is types.BOOL: mon = current_monoid.get(getattr(self.type, "lor_monoid")) else: mon = current_monoid.get(getattr(self.type, "plus_monoid")) mon = mon.get_op() mask, accum, desc = self._get_args(None, accum, desc) result = ffi.new(self.type._c_type + "*") self._check(self.type._Vector_reduce(result, accum, mon, self._vector[0], desc)) return result[0] def reduce_bool(self, mon=None, mask=None, accum=None, desc=None): """Reduce vector to a boolean. >>> v = Vector.from_lists([0, 1], [True, False]) >>> v.reduce_bool() True >>> v[0] = False >>> v.reduce_bool() False >>> v[1] = True >>> v.reduce_bool(types.BOOL.LAND_MONOID) False """ if mon is None: mon = current_monoid.get(types.BOOL.LOR_MONOID) mon = mon.get_op() mask, accum, desc = self._get_args(mask, accum, desc) result = ffi.new("_Bool*") self._check( lib.GrB_Vector_reduce_BOOL(result, accum, mon, self._vector[0], desc) ) return result[0] def reduce_int(self, mon=None, mask=None, accum=None, desc=None): """Reduce vector to a integer. >>> v = Vector.from_lists([0, 1], [1, 1]) >>> v.reduce_int() 2 >>> v[0] = 0 >>> v.reduce_int() 1 >>> v[1] = 2 >>> v.reduce_int(types.INT64.MIN_MONOID) 0 """ if mon is None: mon = current_monoid.get(types.INT64.PLUS_MONOID) mon = mon.get_op() mask, accum, desc = self._get_args(mask, accum, desc) result = ffi.new("int64_t*") self._check( lib.GrB_Vector_reduce_INT64(result, accum, mon, self._vector[0], desc) ) return result[0] def reduce_float(self, mon=None, mask=None, accum=None, desc=None): """Reduce vector to a float. >>> v = Vector.from_lists([0, 1], [1.2, 1.1]) >>> v.reduce_float() 2.3 >>> v[0] = 0 >>> v.reduce_float() 1.1 >>> v[1] = 2.2 >>> v.reduce_float(types.FP64.MIN_MONOID) 0.0 """ if mon is None: mon = current_monoid.get(types.FP64.PLUS_MONOID) mon = mon.get_op() mask, accum, desc = self._get_args(mask, accum, desc) result = ffi.new("double*") self._check( lib.GrB_Vector_reduce_FP64(result, accum, mon, self._vector[0], desc) ) return result[0] def max(self): """Return the max of the vector. >>> M = Vector.from_lists([0, 1, 2], [False, False, False]) >>> M.max() False >>> M = Vector.from_lists([0, 1, 2], [False, False, True]) >>> M.max() True >>> M = Vector.from_lists([0, 1, 2], [-42, 0, 149]) >>> M.max() 149 >>> M = Vector.from_lists([0, 1, 2], [-42.0, 0.0, 149.0]) >>> M.max() 149.0 >>> M = Vector.from_lists([0], [1j]) >>> M.max() Traceback (most recent call last): ... TypeError: Un-maxable type """ if self.type == types.BOOL: return self.reduce_bool(self.type.LOR_MONOID) if self.type in types._int_types: return self.reduce_int(self.type.MAX_MONOID) if self.type in types._float_types: return self.reduce_float(self.type.MAX_MONOID) raise TypeError("Un-maxable type") def min(self): """Return the min of the vector. >>> M = Vector.from_lists([0, 1, 2], [True, True, True]) >>> M.min() True >>> M = Vector.from_lists([0, 1, 2], [False, True, True]) >>> M.min() False >>> M = Vector.from_lists([0, 1, 2], [-42, 0, 149]) >>> M.min() -42 >>> M = Vector.from_lists([0, 1, 2], [-42.0, 0.0, 149.0]) >>> M.min() -42.0 >>> M = Vector.from_lists([0], [1j]) >>> M.min() Traceback (most recent call last): ... TypeError: Un-minable type """ if self.type == types.BOOL: return self.reduce_bool(self.type.LAND_MONOID) if self.type in types._int_types: return self.reduce_int(self.type.MIN_MONOID) if self.type in types._float_types: return self.reduce_float(self.type.MIN_MONOID) raise TypeError("Un-minable type") def apply(self, op, out=None, mask=None, accum=None, desc=None): """Apply Unary op to vector elements. >>> from . import UINT64 >>> v = Vector.from_lists([0,1], [1, 1]) >>> print(v.apply(UINT64.ainv)) 0|-1 1|-1 Unary operators can also be accessed by atribute name on vectors they are applied to: >>> print(v.ainv()) 0|-1 1|-1 """ if out is None: out = Vector.sparse(self.type, self.size) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) self._check( lib.GrB_Vector_apply(out._vector[0], mask, accum, op, self._vector[0], desc) ) return out def apply_first(self, first, op, out=None, mask=None, accum=None, desc=None): """Apply a binary operator to the entries in a vector, binding the first input to a scalar first. >>> v = Vector.from_lists([0,1], [1, 1]) >>> print(v.apply_first(3, types.UINT64.PLUS)) 0| 4 1| 4 >>> w = Vector.sparse(v.type, v.size) >>> v.apply_first(3, types.UINT64.PLUS, out=w) is w True """ if out is None: out = self.__class__.sparse(self.type, self.size) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) if isinstance(first, Scalar): f = lib.GxB_Vector_apply_BinaryOp1st first = first._scalar[0] else: f = self.type._Vector_apply_BinaryOp1st self._check(f(out._vector[0], mask, accum, op, first, self._vector[0], desc)) return out def apply_second(self, op, second, out=None, mask=None, accum=None, desc=None): """Apply a binary operator to the entries in a vector, binding the second input to a scalar second. >>> v = Vector.from_lists([0,1], [1, 1]) >>> print(v.apply_second(types.UINT64.PLUS, 3)) 0| 4 1| 4 >>> w = Vector.sparse(v.type, v.size) >>> v.apply_second(types.UINT64.PLUS, 3, out=w) is w True >>> u = Vector.from_lists([0,1], [1.1, 2.2]) >>> u.apply_second(u.type.TIMES, 3.3, out=u) is u True >>> u = Vector.from_lists([0,1], [1.1, 2.2]) >>> print(u * 3) 0|3.3 1|6.6 >>> x = Vector.from_lists([0,1], [1.1, 2.2]) >>> x *= 3.0 >>> print(x) 0|3.3 1|6.6 """ if out is None: out = self.__class__.sparse(self.type, self.size) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) if isinstance(second, Scalar): f = lib.GxB_Vector_apply_BinaryOp2nd second = second._scalar[0] else: f = self.type._Vector_apply_BinaryOp2nd self._check(f(out._vector[0], mask, accum, op, self._vector[0], second, desc)) return out def select(self, op, thunk=None, out=None, mask=None, accum=None, desc=None): """Select elements that match the given select operation condition. See `Matrix.select` for possible operators. >>> v = Vector.from_lists([0,1], [1, 0]) >>> print(v.select('>', 0)) 0| 1 1| >>> w = Vector.sparse(types.UINT8, 2) >>> v.select('>', 0, out=w) is w True `min` and `max` selectors can be shortcuts for selecting all elements that equal the min or max reduction of all elements. >>> print(v.select('min')) 0| 1| 0 >>> print(v.select('max')) 0| 1 1| """ if out is None: out = Vector.sparse(self.type, self.size) if isinstance(op, str): if op == "min": op = lib.GxB_EQ_THUNK thunk = self.min() elif op == "max": op = lib.GxB_EQ_THUNK thunk = self.max() else: op = _get_select_op(op) if thunk is None: thunk = NULL if isinstance(thunk, (bool, int, float, complex)): thunk = Scalar.from_value(thunk) if isinstance(thunk, Scalar): self._keep_alives[self._vector] = thunk thunk = thunk._scalar[0] mask, accum, desc = self._get_args(mask, accum, desc) self._check( lib.GxB_Vector_select( out._vector[0], mask, accum, op, self._vector[0], thunk, desc ) ) return out def pattern(self, typ=types.BOOL): """Return the pattern of the vector, this is a boolean Vector where every present value in this vector is set to True. """ result = Vector.sparse(typ, self.size) self.apply(types.BOOL.ONE, out=result) return result @property def S(self): """Return the vector "structure". This is the same as calling `Vector.pattern()` with no arguments. >>> v = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> assert v.S == v.pattern() """ return self.pattern() def nonzero(self): """Select vector of nonzero entries.""" return self.select(lib.GxB_NONZERO) def __setitem__(self, index, value): if isinstance(index, int): val = self.type._from_value(value) self._check(self.type._Vector_setElement(self._vector[0], val, index)) return if isinstance(index, slice): if isinstance(value, Vector): self.assign(value, index) return if isinstance(value, (bool, int, float, complex)): self.assign_scalar(value, index) return if isinstance(index, Vector): mask = index._vector[0] index = slice(None, None, None) if isinstance(value, Vector): self.assign(value, index, mask=mask) return self.assign_scalar(value, index, mask=mask) return raise TypeError("Unknown index") def assign(self, value, index=None, mask=None, accum=None, desc=None): """Assign vector to vector. >>> v = Vector.sparse(types.INT8, 3) >>> w = Vector.from_1_to_n(3) >>> v[:] = w >>> print(v) 0| 1 1| 2 2| 3 If the index is another vector it is used as an assignment mask: >>> v.clear() >>> m = Vector.sparse(types.BOOL, 3) >>> m[1] = True >>> v[m] = w >>> print(v) 0| 1| 2 2| >>> v.clear() >>> m = Vector.sparse(types.BOOL, 3) >>> m[1] = True >>> v[m] = 3 >>> print(v) 0| 1| 3 2| """ mask, accum, desc = self._get_args(mask, accum, desc) I, ni, size = _build_range(index, self.size - 1) self._check( lib.GrB_Vector_assign( self._vector[0], mask, accum, value._vector[0], I, ni, desc ) ) def assign_scalar(self, value, index=None, mask=None, accum=None, desc=None): """Assign scalar to vector. >>> v = Vector.sparse(types.INT8, 3) >>> v[:] = 2 >>> print(v) 0| 2 1| 2 2| 2 If the index is another vector it is used as an assignment mask: >>> v.clear() >>> m = Vector.sparse(types.BOOL, 3) >>> m[1] = True >>> v[m] = 3 >>> print(v) 0| 1| 3 2| """ mask, accum, desc = self._get_args(mask, accum, desc) scalar_type = types._gb_from_type(type(value)) I, ni, size = _build_range(index, self.size - 1) self._check( scalar_type._Vector_assignScalar( self._vector[0], mask, accum, value, I, ni, desc ) ) def __getitem__(self, index): if isinstance(index, int): return self.extract_element(index) else: return self.extract(index) def __delitem__(self, index): if not isinstance(index, int): raise TypeError( "__delitem__ currently only supports single element removal" ) self._check(lib.GrB_Vector_removeElement(self._vector[0], index)) def extract_element(self, index): """Extract element from vector.""" result = self.type._ffi.new(self.type._ptr) self._check( self.type._Vector_extractElement( result, self._vector[0], ffi.cast("GrB_Index", index) ) ) return self.type._to_value(result[0]) def extract(self, index, mask=None, accum=None, desc=None): """Extract subvector from vector.""" mask, accum, desc = self._get_args(mask, accum, desc) if isinstance(index, Vector): mask = index._vector[0] index = slice(None, None, None) I, ni, size = _build_range(index, self.size - 1) if size is None: size = self.size result = Vector.sparse(self.type, size) self._check( lib.GrB_Vector_extract( result._vector[0], mask, accum, self._vector[0], I, ni, desc ) ) return result def __contains__(self, index): try: v = self[index] return True except NoValue: return False def get(self, i, default=None): """Get element at `i` or return `default` if not present. >>> M = Vector.from_lists([1, 2], [42, 149]) >>> M.get(1) 42 >>> M.get(0) is None True >>> M.get(0, 'foo') 'foo' """ try: return self[i] except NoValue: return default def wait(self): """Wait for vector to complete.""" self._check(lib.GrB_Vector_wait(self._vector)) def to_string(self, format_string="{:>%s}", width=2, prec=3, empty_char=""): """Return string representation of vector.""" format_string = format_string % width result = "" for row in range(self.size): value = self.get(row, empty_char) result += str(row) + "|" result += format_string.format( self.type.format_value(value, width, prec) ).rstrip() if row < self.size - 1: result += "\n" return result def __str__(self): return self.to_string() def __repr__(self): tname = self.type.__name__ if self.size == lib.GxB_INDEX_MAX: return f"<Vector({tname}, nvals: {self.nvals})>" return f"<Vector({tname} size: {self.size}, nvals: {self.nvals})>" def print(self, level=2, name="A", f=sys.stdout): # pragma: nocover """Print the matrix using `GxB_Matrix_fprint()`, by default to `sys.stdout`.. Level 1: Short description Level 2: Short list, short numbers Level 3: Long list, short number Level 4: Short list, long numbers Level 5: Long list, long numbers """ self._check( lib.GxB_Vector_fprint(self._vector[0], bytes(name, "utf8"), level, f) )
Static methods
def sparse(typ, size=None, fill=None, mask=None)
-
Create an empty Vector from the given type. If
size
is not specified it defaults toGxB_INDEX_MAX
.>>> v = Vector.sparse(types.INT64, 3) >>> v <Vector(INT64 size: 3, nvals: 0)> >>> v.size 3 >>> v = Vector.sparse(types.INT64) >>> v <Vector(INT64, nvals: 0)> >>> v.size == lib.GxB_INDEX_MAX True
>>> v[42] = True >>> w = Vector.sparse(types.INT64, fill=42, mask=v) >>> list(w) [(42, 42)]
If no
fill
is provided, thetype.default_zero
is used:>>> w = Vector.sparse(types.INT64, mask=v) >>> list(w) [(42, 0)]
Expand source code
@classmethod def sparse(cls, typ, size=None, fill=None, mask=None): """Create an empty Vector from the given type. If `size` is not specified it defaults to `pygraphblas.GxB_INDEX_MAX`. >>> v = Vector.sparse(types.INT64, 3) >>> v <Vector(INT64 size: 3, nvals: 0)> >>> v.size 3 >>> v = Vector.sparse(types.INT64) >>> v <Vector(INT64, nvals: 0)> >>> v.size == lib.GxB_INDEX_MAX True >>> v[42] = True >>> w = Vector.sparse(types.INT64, fill=42, mask=v) >>> list(w) [(42, 42)] If no `fill` is provided, the `type.default_zero` is used: >>> w = Vector.sparse(types.INT64, mask=v) >>> list(w) [(42, 0)] """ if size is None: size = GxB_INDEX_MAX new_vec = ffi.new("GrB_Vector*") _check(lib.GrB_Vector_new(new_vec, typ._gb_type, size)) m = cls(new_vec, typ) if mask is not None: if fill is None: fill = m.type.default_zero m.assign_scalar(fill, mask=mask) return m
def random(typ, nvals, size=1152921504606846976, make_pattern=False, seed=None)
-
Expand source code
@classmethod def random( cls, typ, nvals, size=lib.GxB_INDEX_MAX, make_pattern=False, seed=None, ): # pragma: nocover """ """ V = Vector.sparse(typ, size) if seed is not None: random.seed(seed) if V.size == 0: nvals = 0 if typ is types.BOOL: f = partial(random.randint, 0, 1) if typ is types.UINT8: f = partial(random.randint, 0, (2 ** 8) - 1) if typ is types.UINT16: f = partial(random.randint, 0, (2 ** 16) - 1) if typ is types.UINT32: f = partial(random.randint, 0, (2 ** 32) - 1) if typ is types.UINT64: f = partial(random.randint, 0, (2 ** 64) - 1) if typ is types.INT8: f = partial(random.randint, (-(2 ** 7)) + 1, (2 ** 7) - 1) if typ is types.INT16: f = partial(random.randint, (-(2 ** 15)) + 1, (2 ** 15) - 1) if typ is types.INT32: f = partial(random.randint, (-(2 ** 31)) + 1, (2 ** 31) - 1) if typ is types.INT64: f = partial(random.randint, (-(2 ** 63)) + 1, (2 ** 63) - 1) if typ in (types.FP32, types.FP64): f = random.random if typ in (types.FC32, types.FC64): f = lambda: complex(random.random(), random.random()) for i in range(nvals): i = random.randint(0, V.size - 1) V[i] = f() return V
def from_lists(I, V, size=None, typ=None)
-
Create a new vector from the given lists of indices and values. If size is not provided, it is computed from the max values of the provides size indices.
If the second argument is a scalar value, an "iso" vector is created where all values equal that scalar.
>>> v = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> w = Vector.from_lists([0, 1, 2], True) >>> assert not v.iseq(w) >>> assert v.pattern().iseq(w)
Expand source code
@classmethod def from_lists(cls, I, V, size=None, typ=None): """Create a new vector from the given lists of indices and values. If size is not provided, it is computed from the max values of the provides size indices. If the second argument is a scalar value, an "iso" vector is created where all values equal that scalar. >>> v = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> w = Vector.from_lists([0, 1, 2], True) >>> assert not v.iseq(w) >>> assert v.pattern().iseq(w) """ if isinstance(V, (bool, int, float)): V = [V] * len(I) assert len(I) == len(V) assert len(I) > 0 # must be non empty if not size: size = max(I) + 1 # TODO option to use ffi and GrB_Vector_build if typ is None: typ = types._gb_from_type(type(V[0])) m = cls.sparse(typ, size) for i, v in zip(I, V): m[i] = v return m
def from_list(I)
-
Create a new dense vector from the given lists of values.
Expand source code
@classmethod def from_list(cls, I): """Create a new dense vector from the given lists of values.""" size = len(I) assert size > 0 # TODO use ffi and GrB_Vector_build m = cls.sparse(types._gb_from_type(type(I[0])), size) for i, v in enumerate(I): m[i] = v return m
def from_1_to_n(n)
-
Generate a vector from 1 to n.
>>> v = Vector.from_1_to_n(3) >>> print(v) 0| 1 1| 2 2| 3
Expand source code
@classmethod def from_1_to_n(cls, n): """Generate a vector from 1 to n. >>> v = Vector.from_1_to_n(3) >>> print(v) 0| 1 1| 2 2| 3 """ v = cls.sparse(types.INT64, n) for i in range(n): v[i] = i + 1 return v
def dense(typ, size=None, fill=None)
-
Return a dense vector of
typ
andsize
. Iffill
is provided, use that value otherwise useself.type.default_zero
>>> print(Vector.dense(types.FP32, 3)) 0|0.0 1|0.0 2|0.0 >>> print(Vector.dense(types.FP32, 3, fill=42.0)) 0|42.0 1|42.0 2|42.0
Expand source code
@classmethod def dense(cls, typ, size=None, fill=None): """Return a dense vector of `typ` and `size`. If `fill` is provided, use that value otherwise use `self.type.default_zero` >>> print(Vector.dense(types.FP32, 3)) 0|0.0 1|0.0 2|0.0 >>> print(Vector.dense(types.FP32, 3, fill=42.0)) 0|42.0 1|42.0 2|42.0 """ v = cls.sparse(typ, size) if fill is None: fill = v.type.default_zero v[:] = fill return v
def iso(value, size=1152921504606846976)
-
Build an "iso" vector from a scalar value.
This is similar to
Vector.dense()
but infers the type of the new Matrix from the provided vbalue.>>> v = Vector.iso(3) >>> assert v[42] == 3
Expand source code
@classmethod def iso(cls, value, size=GxB_INDEX_MAX): """Build an "iso" vector from a scalar value. This is similar to `Vector.dense` but infers the type of the new Matrix from the provided vbalue. >>> v = Vector.iso(3) >>> assert v[42] == 3 """ typ = types._gb_from_type(type(value)) return cls.dense(typ, size, value)
Instance Attributes
var indices
-
cdata array of vector indexes.
>>> v = Vector.from_1_to_n(3) >>> list(v.indices) [0, 1, 2]
Expand source code
@property def indices(self): """cdata array of vector indexes. >>> v = Vector.from_1_to_n(3) >>> list(v.indices) [0, 1, 2] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = ffi.new("GrB_Index[%s]" % nvals) X = NULL self._check(self.type._Vector_extractTuples(I, X, _nvals, self._vector[0])) return I
var I
-
Iterator over for
Vector.indices
.>>> v = Vector.from_1_to_n(3) >>> list(v.I) [0, 1, 2]
Expand source code
@property def I(self): """Iterator over for `Vector.indices`. >>> v = Vector.from_1_to_n(3) >>> list(v.I) [0, 1, 2] """ return iter(self.indices)
var npI
-
numpy array over
Vector.indices
.>>> v = Vector.from_1_to_n(3) >>> v.npI array([0, 1, 2], dtype=uint64)
Expand source code
@property def npI(self): """numpy array over `Vector.indices`. >>> v = Vector.from_1_to_n(3) >>> v.npI array([0, 1, 2], dtype=uint64) """ return np.frombuffer(ffi.buffer(self.indices), dtype=np.uint64)
var vals
-
Iterator of vector values.
>>> v = Vector.from_1_to_n(3) >>> list(v.vals) [1, 2, 3]
Expand source code
@property def vals(self): """Iterator of vector values. >>> v = Vector.from_1_to_n(3) >>> list(v.vals) [1, 2, 3] """ nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = NULL V = ffi.new("%s[%s]" % (self.type._c_type, nvals)) self._check(self.type._Vector_extractTuples(I, V, _nvals, self._vector[0])) return V
var V
-
Iterator over for
Vector.vals
.>>> v = Vector.from_1_to_n(3) >>> list(v.V) [1, 2, 3]
Expand source code
@property def V(self): """Iterator over for `Vector.vals`. >>> v = Vector.from_1_to_n(3) >>> list(v.V) [1, 2, 3] """ return iter(self.vals)
var npV
-
numpy array over
Vector.vals
.>>> v = Vector.from_1_to_n(3) >>> v.npV array([1, 2, 3])
Expand source code
@property def npV(self): """numpy array over `Vector.vals`. >>> v = Vector.from_1_to_n(3) >>> v.npV array([1, 2, 3]) """ return np.frombuffer(ffi.buffer(self.vals), dtype=self.type._numpy_t)
var hyper_switch
-
Get the hyper_switch threshold. (See SuiteSparse User Guide)
Expand source code
@property def hyper_switch(self): # pragma: nocover """Get the hyper_switch threshold. (See SuiteSparse User Guide)""" switch = ffi.new("double*") self._check( lib.GxB_Vector_Option_get(self._vector[0], lib.GxB_HYPER_SWITCH, switch) ) return switch[0]
var sparsity
-
Get Vector sparsity control. (See SuiteSparse User Guide)
Expand source code
@property def sparsity(self): # pragma: nocover """Get Vector sparsity control. (See SuiteSparse User Guide)""" sparsity = ffi.new("int*") self._check( lib.GxB_Vector_Option_get( self._vector[0], lib.GxB_SPARSITY_CONTROL, sparsity ) ) return sparsity[0]
var sparsity_status
-
Get Vector sparsity status. (See SuiteSparse User Guide)
Expand source code
@property def sparsity_status(self): # pragma: nocover """Get Vector sparsity status. (See SuiteSparse User Guide)""" status = ffi.new("int*") self._check( lib.GxB_Vector_Option_get(self._vector[0], lib.GxB_SPARSITY_STATUS, status) ) return status[0]
var size
-
Return the size of the vector.
>>> Vector.from_1_to_n(3).size 3
Expand source code
@property def size(self): """Return the size of the vector. >>> Vector.from_1_to_n(3).size 3 """ n = ffi.new("GrB_Index*") self._check(lib.GrB_Vector_size(n, self._vector[0])) return n[0]
var nvals
-
Return the number of values in the vector.
>>> v = Vector.from_1_to_n(3) >>> v.nvals 3 >>> v.clear() >>> v.nvals 0
Expand source code
@property def nvals(self): """Return the number of values in the vector. >>> v = Vector.from_1_to_n(3) >>> v.nvals 3 >>> v.clear() >>> v.nvals 0 """ n = ffi.new("GrB_Index*") self._check(lib.GrB_Vector_nvals(n, self._vector[0])) return n[0]
var memory_usage
-
Returns the memory usage of the Vector.
>>> v = Vector.from_lists([0, 1, 2], [1, 2, 0]) >>> assert v.memory_usage > 0
Expand source code
@property def memory_usage(self): """Returns the memory usage of the Vector. >>> v = Vector.from_lists([0, 1, 2], [1, 2, 0]) >>> assert v.memory_usage > 0 """ n = ffi.new("size_t*") self._check(lib.GxB_Vector_memoryUsage(n, self._vector[0])) return n[0]
var gb_type
-
Return the GraphBLAS low-level type object of the Vector.
Expand source code
@property def gb_type(self): """Return the GraphBLAS low-level type object of the Vector.""" return self.type._gb_type
var S
-
Return the vector "structure". This is the same as calling
Vector.pattern()
with no arguments.>>> v = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> assert v.S == v.pattern()
Expand source code
@property def S(self): """Return the vector "structure". This is the same as calling `Vector.pattern()` with no arguments. >>> v = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> assert v.S == v.pattern() """ return self.pattern()
var type
-
Return an attribute of instance, which is of type owner.
Methods
def all(self, other, op)
-
Do all elements in self compare True with op to other?
>>> from . import INT64 >>> M = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> N = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> O = Vector.from_lists([0, 1], [1, 2]) >>> P = Vector.from_lists([0, 1], [1, 2], size=3) >>> Q = Vector.from_lists([0, 1, 3], [1, 2, 3]) >>> assert M.all(N, INT64.eq) >>> assert not M.all(N, INT64.gt) >>> assert not M.all(O, INT64.eq) >>> assert not M.all(P, INT64.eq) >>> assert not M.all(Q, INT64.eq)
Expand source code
def all(self, other, op): """Do all elements in self compare True with op to other? >>> from . import INT64 >>> M = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> N = Vector.from_lists([0, 1, 2], [1, 2, 3]) >>> O = Vector.from_lists([0, 1], [1, 2]) >>> P = Vector.from_lists([0, 1], [1, 2], size=3) >>> Q = Vector.from_lists([0, 1, 3], [1, 2, 3]) >>> assert M.all(N, INT64.eq) >>> assert not M.all(N, INT64.gt) >>> assert not M.all(O, INT64.eq) >>> assert not M.all(P, INT64.eq) >>> assert not M.all(Q, INT64.eq) """ if self.size != other.size: return False if self.nvals != other.nvals: return False C = self.emult(other, op, cast=types.BOOL) if C.nvals != self.nvals: # pragma: nocover return False return C.reduce_bool(types.BOOL.land_monoid)
def iseq(self, other, eq_op=None)
-
Compare two vectors for equality.
Note to be confused with the
==
operator which does element-wise comparison and returns aVector
.>>> v = Vector.from_lists([0,1], [1, 1]) >>> w = Vector.from_lists([0,1], [1, 1]) >>> x = Vector.from_lists([0,1], [1.0, 1.0]) >>> v.iseq(w) True
>>> v.iseq(w, eq_op=types.UINT64.GE) True
>>> v.iseq(x) False
Expand source code
def iseq(self, other, eq_op=None): """Compare two vectors for equality. Note to be confused with the `==` operator which does element-wise comparison and returns a `Vector`. >>> v = Vector.from_lists([0,1], [1, 1]) >>> w = Vector.from_lists([0,1], [1, 1]) >>> x = Vector.from_lists([0,1], [1.0, 1.0]) >>> v.iseq(w) True >>> v.iseq(w, eq_op=types.UINT64.GE) True >>> v.iseq(x) False """ if self.type != other.type: return False if eq_op is None: eq_op = self.type.EQ return self.all(other, eq_op)
def isne(self, other)
-
Compare two vectors for inequality. Note to be confused with the
==
operator which does element-wise comparison and returns aVector
.>>> v = Vector.from_lists([0,1], [1, 1]) >>> w = Vector.from_lists([0,1], [1, 1]) >>> v.isne(w) False
Expand source code
def isne(self, other): """Compare two vectors for inequality. Note to be confused with the `==` operator which does element-wise comparison and returns a `Vector`. >>> v = Vector.from_lists([0,1], [1, 1]) >>> w = Vector.from_lists([0,1], [1, 1]) >>> v.isne(w) False """ return not self.iseq(other)
def dup(self)
-
Create an duplicate Vector from the given argument.
>>> v = Vector.from_1_to_n(3) >>> w = v.dup() >>> w is not v True >>> w.iseq(v) True >>> print(w) 0| 1 1| 2 2| 3
Expand source code
def dup(self): """Create an duplicate Vector from the given argument. >>> v = Vector.from_1_to_n(3) >>> w = v.dup() >>> w is not v True >>> w.iseq(v) True >>> print(w) 0| 1 1| 2 2| 3 """ new_vec = ffi.new("GrB_Vector*") self._check(lib.GrB_Vector_dup(new_vec, self._vector[0])) return self.__class__(new_vec, self.type)
def to_lists(self)
-
Extract the indices and values of the Vector as 2 lists.
>>> Vector.from_1_to_n(3).to_lists() [[0, 1, 2], [1, 2, 3]]
Expand source code
def to_lists(self): """Extract the indices and values of the Vector as 2 lists. >>> Vector.from_1_to_n(3).to_lists() [[0, 1, 2], [1, 2, 3]] """ I = ffi.new("GrB_Index[]", self.nvals) V = self.type._ffi.new(self.type._c_type + "[]", self.nvals) n = ffi.new("GrB_Index*") n[0] = self.nvals self._check(self.type._Vector_extractTuples(I, V, n, self._vector[0])) return [list(I), list(map(self.type._to_value, V))]
def to_arrays(self)
-
Return as python
array
objects.>>> Vector.from_1_to_n(3).to_arrays() (array('L', [0, 1, 2]), array('q', [1, 2, 3]))
Expand source code
def to_arrays(self): """Return as python `array` objects. >>> Vector.from_1_to_n(3).to_arrays() (array('L', [0, 1, 2]), array('q', [1, 2, 3])) """ if self.type._typecode is None: raise TypeError("This matrix has no array typecode.") nvals = self.nvals _nvals = ffi.new("GrB_Index[1]", [nvals]) I = ffi.new("GrB_Index[%s]" % nvals) X = self.type._ffi.new("%s[%s]" % (self.type._c_type, nvals)) self._check(self.type._Vector_extractTuples(I, X, _nvals, self._vector[0])) return array("L", I), array(self.type._typecode, X)
def eadd(self, other, add_op=None, cast=None, out=None, mask=None, accum=None, desc=None)
-
Element-wise addition with other vector.
Element-wise addition applies a binary operator element-wise on two vectors
v
andw
, for all entries that appear in the set union of the patterns ofA
andB
.The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation.
>>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> V = list(range(len(I))) >>> v = Vector.from_lists(I, V, 7)
>>> w = Vector.from_lists( ... [0, 1, 4, 6], ... [9, 1, 4, 7], 7)
>>> print(v.eadd(w)) 0|10 1| 4 2| 4 3| 6 4|11 5| 8 6|18
This can also be accomplished with the
+
operators:>>> print(v + w) 0|10 1| 4 2| 4 3| 6 4|11 5| 8 6|18
The combining operator used can be provided either as a context manager or passed to
mxv
as theadd_op
argument.>>> with types.INT64.MIN: ... print(v + w) 0| 1 1| 1 2| 4 3| 6 4| 4 5| 8 6| 7
You can provide a monoid for the operation:
>>> print(v.eadd(w, v.type.min_monoid)) 0| 1 1| 1 2| 4 3| 6 4| 4 5| 8 6| 7
Or you can use a semiring:
>>> print(v.eadd(w, v.type.min_plus)) 0| 1 1| 1 2| 4 3| 6 4| 4 5| 8 6| 7
The following operators default to use
eadd
:Operator Description Default v | w Vector Union type default SECOND combiner v |= w In-place Vector Union type default SECOND combiner v + w Vector Element-Wise Union type default PLUS combiner v += w In-place Vector Element-Wise Union type default PLUS combiner v - w Vector Element-Wise Union type default MINUS combiner v -= w In-place Vector Element-Wise Union type default MINUS combiner Expand source code
def eadd( self, other, add_op=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Element-wise addition with other vector. Element-wise addition applies a binary operator element-wise on two vectors `v` and `w`, for all entries that appear in the set union of the patterns of `A` and `B`. The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation. >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> V = list(range(len(I))) >>> v = Vector.from_lists(I, V, 7) >>> w = Vector.from_lists( ... [0, 1, 4, 6], ... [9, 1, 4, 7], 7) >>> print(v.eadd(w)) 0|10 1| 4 2| 4 3| 6 4|11 5| 8 6|18 This can also be accomplished with the `+` operators: >>> print(v + w) 0|10 1| 4 2| 4 3| 6 4|11 5| 8 6|18 The combining operator used can be provided either as a context manager or passed to `mxv` as the `add_op` argument. >>> with types.INT64.MIN: ... print(v + w) 0| 1 1| 1 2| 4 3| 6 4| 4 5| 8 6| 7 You can provide a monoid for the operation: >>> print(v.eadd(w, v.type.min_monoid)) 0| 1 1| 1 2| 4 3| 6 4| 4 5| 8 6| 7 Or you can use a semiring: >>> print(v.eadd(w, v.type.min_plus)) 0| 1 1| 1 2| 4 3| 6 4| 4 5| 8 6| 7 The following operators default to use `eadd`: Operator | Description | Default --- | --- | --- v \\| w | Vector Union | type default SECOND combiner v \\|= w | In-place Vector Union | type default SECOND combiner v + w | Vector Element-Wise Union | type default PLUS combiner v += w | In-place Vector Element-Wise Union | type default PLUS combiner v - w | Vector Element-Wise Union | type default MINUS combiner v -= w | In-place Vector Element-Wise Union | type default MINUS combiner """ func = lib.GrB_Vector_eWiseAdd_BinaryOp if add_op is None: add_op = current_binop.get(NULL) elif isinstance(add_op, Monoid): func = lib.GrB_Vector_eWiseAdd_Monoid elif isinstance(add_op, Semiring): func = lib.GrB_Vector_eWiseAdd_Semiring mask, accum, desc = self._get_args(mask, accum, desc) if out is None: typ = cast or types.promote(self.type, other.type) _out = ffi.new("GrB_Vector*") self._check(lib.GrB_Vector_new(_out, typ._gb_type, self.size)) out = self.__class__(_out, typ) if add_op is NULL: add_op = out.type._default_addop() add_op = add_op.get_op() self._check( func( out._vector[0], mask, accum, add_op, self._vector[0], other._vector[0], desc, ) ) return out
def emult(self, other, mult_op=None, cast=None, out=None, mask=None, accum=None, desc=None)
-
Element-wise multiplication with other vector.
Element-wise multiplication applies a binary operator element-wise on two vectors A and B, for all entries that appear in the set intersection of the patterns of A and B.
The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation.
>>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> V = list(range(len(I))) >>> v = Vector.from_lists(I, V, 7)
>>> w = Vector.from_lists( ... [0, 1, 4, 6], ... [9, 1, 4, 7], 7)
>>> print(v.emult(w)) 0| 9 1| 3 2| 3| 4|28 5| 6|77
This can also be accomplished with the
+
operators:>>> print(v * w) 0| 9 1| 3 2| 3| 4|28 5| 6|77
The combining operator used can be provided either as a context manager or passed to
mxv
as theadd_op
argument.>>> with types.INT64.MAX: ... print(v * w) 0| 9 1| 3 2| 3| 4|28 5| 6|77
Expand source code
def emult( self, other, mult_op=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Element-wise multiplication with other vector. Element-wise multiplication applies a binary operator element-wise on two vectors A and B, for all entries that appear in the set intersection of the patterns of A and B. The only difference between element-wise multiplication and addition is the pattern of the result, and what happens to entries outside the intersection. With multiplication the pattern of T is the intersection; with addition it is the set union. Entries outside the set intersection are dropped for multiplication, and kept for addition; in both cases the operator is only applied to those (and only those) entries in the intersection. Any binary operator can be used interchangeably for either operation. >>> I = [0, 0, 1, 1, 2, 3, 3, 4, 5, 6, 6, 6] >>> V = list(range(len(I))) >>> v = Vector.from_lists(I, V, 7) >>> w = Vector.from_lists( ... [0, 1, 4, 6], ... [9, 1, 4, 7], 7) >>> print(v.emult(w)) 0| 9 1| 3 2| 3| 4|28 5| 6|77 This can also be accomplished with the `+` operators: >>> print(v * w) 0| 9 1| 3 2| 3| 4|28 5| 6|77 The combining operator used can be provided either as a context manager or passed to `mxv` as the `add_op` argument. >>> with types.INT64.MAX: ... print(v * w) 0| 9 1| 3 2| 3| 4|28 5| 6|77 """ if mult_op is None: mult_op = current_binop.get(NULL) elif isinstance(mult_op, str): mult_op = _get_bin_op(mult_op, self.type) mask, accum, desc = self._get_args(mask, accum, desc) if out is None: typ = cast or types.promote(self.type, other.type) _out = ffi.new("GrB_Vector*") self._check(lib.GrB_Vector_new(_out, typ._gb_type, self.size)) out = self.__class__(_out, typ) if mult_op is NULL: mult_op = out.type._default_multop() mult_op = mult_op.get_op() self._check( lib.GrB_Vector_eWiseMult_BinaryOp( out._vector[0], mask, accum, mult_op, self._vector[0], other._vector[0], desc, ) ) return out
def vxm(self, other, semiring=None, cast=None, out=None, mask=None, accum=None, desc=None)
-
Vector-Matrix multiply.
Multiply this row vector by
other
matrix "on the left". For column matrix/vector multiplication "on the right" seeMatrix.mxv()
.vxm
can also be called directly or with the@
operator:>>> from . import Matrix, INT64 >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> v = Vector.from_lists([0, 1, 2], [2, 3, 4]) >>> o = v.vxm(M) >>> print(o) 0|12 1| 2 2| 6 >>> o = v @ M >>> print(o) 0|12 1| 2 2| 6
By default,
mxv
and@
create a new result matrix of the correct type and dimensions if one is not provided. If you want to provide your own matrix to put the result in, you can pass it in theout
parameter. This is useful for accumulating results into a single matrix with minimal copying. This is also supported by the@=
syntax:>>> o = v.dup() >>> v.vxm(M, accum=INT64.plus, out=o) is o True >>> print(o) 0|14 1| 5 2|10 >>> o = v.dup() >>> with Accum(INT64.min): ... o @= M >>> print(o) 0| 2 1| 2 2| 4
The default semiring depends on the infered result type. In the case of numbers, the default semiring is
PLUS_TIMES
. In the case of typeBOOL
, it isBOOL.lor_land
.>>> o = v.vxm(M, semiring=INT64.min_plus) >>> print(o) 0| 7 1| 3 2| 5
An explicit semiring can be passed to the method or provided with a context manager:
>>> with INT64.min_plus: ... o = v @ M >>> print(o) 0| 7 1| 3 2| 5
Or the semiring can be accessed via an attribute on the vector:
>>> o = v.min_plus(M) >>> print(o) 0| 7 1| 3 2| 5
Descriptors and accumulators can also be provided as an argument or a context manager:
>>> o = v.vxm(M, desc=descriptor.T0) >>> print(o) 0|12 1| 2 2| 6 >>> with descriptor.T0: ... o = v @ M >>> print(o) 0|12 1| 2 2| 6 >>> del o[1] >>> o = v.vxm(M, mask=o) >>> print(o) 0|12 1| 2| 6
Expand source code
def vxm( self, other, semiring=None, cast=None, out=None, mask=None, accum=None, desc=None, ): """Vector-Matrix multiply. Multiply this row vector by `other` matrix "on the left". For column matrix/vector multiplication "on the right" see `Matrix.mxv`. `vxm` can also be called directly or with the `@` operator: >>> from . import Matrix, INT64 >>> M = Matrix.from_lists([0, 1, 2], [1, 2, 0], [1, 2, 3]) >>> v = Vector.from_lists([0, 1, 2], [2, 3, 4]) >>> o = v.vxm(M) >>> print(o) 0|12 1| 2 2| 6 >>> o = v @ M >>> print(o) 0|12 1| 2 2| 6 By default, `mxv` and `@` create a new result matrix of the correct type and dimensions if one is not provided. If you want to provide your own matrix to put the result in, you can pass it in the `out` parameter. This is useful for accumulating results into a single matrix with minimal copying. This is also supported by the `@=` syntax: >>> o = v.dup() >>> v.vxm(M, accum=INT64.plus, out=o) is o True >>> print(o) 0|14 1| 5 2|10 >>> o = v.dup() >>> with Accum(INT64.min): ... o @= M >>> print(o) 0| 2 1| 2 2| 4 The default semiring depends on the infered result type. In the case of numbers, the default semiring is `PLUS_TIMES`. In the case of type `BOOL`, it is `BOOL.lor_land`. >>> o = v.vxm(M, semiring=INT64.min_plus) >>> print(o) 0| 7 1| 3 2| 5 An explicit semiring can be passed to the method or provided with a context manager: >>> with INT64.min_plus: ... o = v @ M >>> print(o) 0| 7 1| 3 2| 5 Or the semiring can be accessed via an attribute on the vector: >>> o = v.min_plus(M) >>> print(o) 0| 7 1| 3 2| 5 Descriptors and accumulators can also be provided as an argument or a context manager: >>> o = v.vxm(M, desc=descriptor.T0) >>> print(o) 0|12 1| 2 2| 6 >>> with descriptor.T0: ... o = v @ M >>> print(o) 0|12 1| 2 2| 6 >>> del o[1] >>> o = v.vxm(M, mask=o) >>> print(o) 0|12 1| 2| 6 """ if semiring is None: semiring = current_semiring.get(NULL) if out is None: new_dimension = other.nrows if T1 in (desc or ()) else other.ncols if semiring is not NULL: typ = semiring.ztype else: typ = cast or types.promote(self.type, other.type) out = Vector.sparse(typ, new_dimension) else: typ = out.type if semiring is NULL: semiring = out.type._default_semiring() semiring = semiring.get_op() mask, accum, desc = self._get_args(mask, accum, desc) self._check( lib.GrB_vxm( out._vector[0], mask, accum, semiring, self._vector[0], other._matrix[0], desc, ) ) return out
def clear(self)
-
Clear this vector removing all entries.
Expand source code
def clear(self): """Clear this vector removing all entries.""" self._check(lib.GrB_Vector_clear(self._vector[0]))
def resize(self, size=1152921504606846976)
-
Resize the vector. If the dimensions decrease, entries that fall outside the resized vector are deleted.
>>> v = Vector.dense(types.UINT8, 2) >>> v.resize(3) >>> print(v) 0| 0 1| 0 2|
Expand source code
def resize(self, size=lib.GxB_INDEX_MAX): """Resize the vector. If the dimensions decrease, entries that fall outside the resized vector are deleted. >>> v = Vector.dense(types.UINT8, 2) >>> v.resize(3) >>> print(v) 0| 0 1| 0 2| """ self._check(lib.GrB_Vector_resize(self._vector[0], size))
def reduce(self, mon=None, accum=None, desc=None)
-
Do a scalar reduce based on this object's type:
>>> V = Vector.random(types.UINT8, 10, 3, seed=42) >>> V.reduce() 114
>>> V = Vector.random(types.FP32, 10, 3, seed=42) >>> V.reduce() 0.9517456293106079
>>> V = Vector.random(types.UINT8, 10, 3, seed=42) >>> V.reduce(V.type.min_monoid) 13
>>> V = Vector.random(types.BOOL, 10, 3, seed=42) >>> V.reduce() False
Expand source code
def reduce(self, mon=None, accum=None, desc=None): """Do a scalar reduce based on this object's type: >>> V = Vector.random(types.UINT8, 10, 3, seed=42) >>> V.reduce() 114 >>> V = Vector.random(types.FP32, 10, 3, seed=42) >>> V.reduce() 0.9517456293106079 >>> V = Vector.random(types.UINT8, 10, 3, seed=42) >>> V.reduce(V.type.min_monoid) 13 >>> V = Vector.random(types.BOOL, 10, 3, seed=42) >>> V.reduce() False """ if mon is None: if self.type is types.BOOL: mon = current_monoid.get(getattr(self.type, "lor_monoid")) else: mon = current_monoid.get(getattr(self.type, "plus_monoid")) mon = mon.get_op() mask, accum, desc = self._get_args(None, accum, desc) result = ffi.new(self.type._c_type + "*") self._check(self.type._Vector_reduce(result, accum, mon, self._vector[0], desc)) return result[0]
def reduce_bool(self, mon=None, mask=None, accum=None, desc=None)
-
Reduce vector to a boolean.
>>> v = Vector.from_lists([0, 1], [True, False]) >>> v.reduce_bool() True >>> v[0] = False >>> v.reduce_bool() False >>> v[1] = True >>> v.reduce_bool(types.BOOL.LAND_MONOID) False
Expand source code
def reduce_bool(self, mon=None, mask=None, accum=None, desc=None): """Reduce vector to a boolean. >>> v = Vector.from_lists([0, 1], [True, False]) >>> v.reduce_bool() True >>> v[0] = False >>> v.reduce_bool() False >>> v[1] = True >>> v.reduce_bool(types.BOOL.LAND_MONOID) False """ if mon is None: mon = current_monoid.get(types.BOOL.LOR_MONOID) mon = mon.get_op() mask, accum, desc = self._get_args(mask, accum, desc) result = ffi.new("_Bool*") self._check( lib.GrB_Vector_reduce_BOOL(result, accum, mon, self._vector[0], desc) ) return result[0]
def reduce_int(self, mon=None, mask=None, accum=None, desc=None)
-
Reduce vector to a integer.
>>> v = Vector.from_lists([0, 1], [1, 1]) >>> v.reduce_int() 2 >>> v[0] = 0 >>> v.reduce_int() 1 >>> v[1] = 2 >>> v.reduce_int(types.INT64.MIN_MONOID) 0
Expand source code
def reduce_int(self, mon=None, mask=None, accum=None, desc=None): """Reduce vector to a integer. >>> v = Vector.from_lists([0, 1], [1, 1]) >>> v.reduce_int() 2 >>> v[0] = 0 >>> v.reduce_int() 1 >>> v[1] = 2 >>> v.reduce_int(types.INT64.MIN_MONOID) 0 """ if mon is None: mon = current_monoid.get(types.INT64.PLUS_MONOID) mon = mon.get_op() mask, accum, desc = self._get_args(mask, accum, desc) result = ffi.new("int64_t*") self._check( lib.GrB_Vector_reduce_INT64(result, accum, mon, self._vector[0], desc) ) return result[0]
def reduce_float(self, mon=None, mask=None, accum=None, desc=None)
-
Reduce vector to a float.
>>> v = Vector.from_lists([0, 1], [1.2, 1.1]) >>> v.reduce_float() 2.3 >>> v[0] = 0 >>> v.reduce_float() 1.1 >>> v[1] = 2.2 >>> v.reduce_float(types.FP64.MIN_MONOID) 0.0
Expand source code
def reduce_float(self, mon=None, mask=None, accum=None, desc=None): """Reduce vector to a float. >>> v = Vector.from_lists([0, 1], [1.2, 1.1]) >>> v.reduce_float() 2.3 >>> v[0] = 0 >>> v.reduce_float() 1.1 >>> v[1] = 2.2 >>> v.reduce_float(types.FP64.MIN_MONOID) 0.0 """ if mon is None: mon = current_monoid.get(types.FP64.PLUS_MONOID) mon = mon.get_op() mask, accum, desc = self._get_args(mask, accum, desc) result = ffi.new("double*") self._check( lib.GrB_Vector_reduce_FP64(result, accum, mon, self._vector[0], desc) ) return result[0]
def max(self)
-
Return the max of the vector.
>>> M = Vector.from_lists([0, 1, 2], [False, False, False]) >>> M.max() False >>> M = Vector.from_lists([0, 1, 2], [False, False, True]) >>> M.max() True >>> M = Vector.from_lists([0, 1, 2], [-42, 0, 149]) >>> M.max() 149 >>> M = Vector.from_lists([0, 1, 2], [-42.0, 0.0, 149.0]) >>> M.max() 149.0 >>> M = Vector.from_lists([0], [1j]) >>> M.max() Traceback (most recent call last): ... TypeError: Un-maxable type
Expand source code
def max(self): """Return the max of the vector. >>> M = Vector.from_lists([0, 1, 2], [False, False, False]) >>> M.max() False >>> M = Vector.from_lists([0, 1, 2], [False, False, True]) >>> M.max() True >>> M = Vector.from_lists([0, 1, 2], [-42, 0, 149]) >>> M.max() 149 >>> M = Vector.from_lists([0, 1, 2], [-42.0, 0.0, 149.0]) >>> M.max() 149.0 >>> M = Vector.from_lists([0], [1j]) >>> M.max() Traceback (most recent call last): ... TypeError: Un-maxable type """ if self.type == types.BOOL: return self.reduce_bool(self.type.LOR_MONOID) if self.type in types._int_types: return self.reduce_int(self.type.MAX_MONOID) if self.type in types._float_types: return self.reduce_float(self.type.MAX_MONOID) raise TypeError("Un-maxable type")
def min(self)
-
Return the min of the vector.
>>> M = Vector.from_lists([0, 1, 2], [True, True, True]) >>> M.min() True >>> M = Vector.from_lists([0, 1, 2], [False, True, True]) >>> M.min() False >>> M = Vector.from_lists([0, 1, 2], [-42, 0, 149]) >>> M.min() -42 >>> M = Vector.from_lists([0, 1, 2], [-42.0, 0.0, 149.0]) >>> M.min() -42.0 >>> M = Vector.from_lists([0], [1j]) >>> M.min() Traceback (most recent call last): ... TypeError: Un-minable type
Expand source code
def min(self): """Return the min of the vector. >>> M = Vector.from_lists([0, 1, 2], [True, True, True]) >>> M.min() True >>> M = Vector.from_lists([0, 1, 2], [False, True, True]) >>> M.min() False >>> M = Vector.from_lists([0, 1, 2], [-42, 0, 149]) >>> M.min() -42 >>> M = Vector.from_lists([0, 1, 2], [-42.0, 0.0, 149.0]) >>> M.min() -42.0 >>> M = Vector.from_lists([0], [1j]) >>> M.min() Traceback (most recent call last): ... TypeError: Un-minable type """ if self.type == types.BOOL: return self.reduce_bool(self.type.LAND_MONOID) if self.type in types._int_types: return self.reduce_int(self.type.MIN_MONOID) if self.type in types._float_types: return self.reduce_float(self.type.MIN_MONOID) raise TypeError("Un-minable type")
def apply(self, op, out=None, mask=None, accum=None, desc=None)
-
Apply Unary op to vector elements.
>>> from . import UINT64 >>> v = Vector.from_lists([0,1], [1, 1]) >>> print(v.apply(UINT64.ainv)) 0|-1 1|-1
Unary operators can also be accessed by atribute name on vectors they are applied to:
>>> print(v.ainv()) 0|-1 1|-1
Expand source code
def apply(self, op, out=None, mask=None, accum=None, desc=None): """Apply Unary op to vector elements. >>> from . import UINT64 >>> v = Vector.from_lists([0,1], [1, 1]) >>> print(v.apply(UINT64.ainv)) 0|-1 1|-1 Unary operators can also be accessed by atribute name on vectors they are applied to: >>> print(v.ainv()) 0|-1 1|-1 """ if out is None: out = Vector.sparse(self.type, self.size) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) self._check( lib.GrB_Vector_apply(out._vector[0], mask, accum, op, self._vector[0], desc) ) return out
def apply_first(self, first, op, out=None, mask=None, accum=None, desc=None)
-
Apply a binary operator to the entries in a vector, binding the first input to a scalar first.
>>> v = Vector.from_lists([0,1], [1, 1]) >>> print(v.apply_first(3, types.UINT64.PLUS)) 0| 4 1| 4 >>> w = Vector.sparse(v.type, v.size) >>> v.apply_first(3, types.UINT64.PLUS, out=w) is w True
Expand source code
def apply_first(self, first, op, out=None, mask=None, accum=None, desc=None): """Apply a binary operator to the entries in a vector, binding the first input to a scalar first. >>> v = Vector.from_lists([0,1], [1, 1]) >>> print(v.apply_first(3, types.UINT64.PLUS)) 0| 4 1| 4 >>> w = Vector.sparse(v.type, v.size) >>> v.apply_first(3, types.UINT64.PLUS, out=w) is w True """ if out is None: out = self.__class__.sparse(self.type, self.size) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) if isinstance(first, Scalar): f = lib.GxB_Vector_apply_BinaryOp1st first = first._scalar[0] else: f = self.type._Vector_apply_BinaryOp1st self._check(f(out._vector[0], mask, accum, op, first, self._vector[0], desc)) return out
def apply_second(self, op, second, out=None, mask=None, accum=None, desc=None)
-
Apply a binary operator to the entries in a vector, binding the second input to a scalar second.
>>> v = Vector.from_lists([0,1], [1, 1]) >>> print(v.apply_second(types.UINT64.PLUS, 3)) 0| 4 1| 4 >>> w = Vector.sparse(v.type, v.size) >>> v.apply_second(types.UINT64.PLUS, 3, out=w) is w True >>> u = Vector.from_lists([0,1], [1.1, 2.2]) >>> u.apply_second(u.type.TIMES, 3.3, out=u) is u True >>> u = Vector.from_lists([0,1], [1.1, 2.2]) >>> print(u * 3) 0|3.3 1|6.6 >>> x = Vector.from_lists([0,1], [1.1, 2.2]) >>> x *= 3.0 >>> print(x) 0|3.3 1|6.6
Expand source code
def apply_second(self, op, second, out=None, mask=None, accum=None, desc=None): """Apply a binary operator to the entries in a vector, binding the second input to a scalar second. >>> v = Vector.from_lists([0,1], [1, 1]) >>> print(v.apply_second(types.UINT64.PLUS, 3)) 0| 4 1| 4 >>> w = Vector.sparse(v.type, v.size) >>> v.apply_second(types.UINT64.PLUS, 3, out=w) is w True >>> u = Vector.from_lists([0,1], [1.1, 2.2]) >>> u.apply_second(u.type.TIMES, 3.3, out=u) is u True >>> u = Vector.from_lists([0,1], [1.1, 2.2]) >>> print(u * 3) 0|3.3 1|6.6 >>> x = Vector.from_lists([0,1], [1.1, 2.2]) >>> x *= 3.0 >>> print(x) 0|3.3 1|6.6 """ if out is None: out = self.__class__.sparse(self.type, self.size) op = op.get_op() mask, accum, desc = self._get_args(mask, accum, desc) if isinstance(second, Scalar): f = lib.GxB_Vector_apply_BinaryOp2nd second = second._scalar[0] else: f = self.type._Vector_apply_BinaryOp2nd self._check(f(out._vector[0], mask, accum, op, self._vector[0], second, desc)) return out
def select(self, op, thunk=None, out=None, mask=None, accum=None, desc=None)
-
Select elements that match the given select operation condition. See
Matrix.select()
for possible operators.>>> v = Vector.from_lists([0,1], [1, 0]) >>> print(v.select('>', 0)) 0| 1 1|
>>> w = Vector.sparse(types.UINT8, 2) >>> v.select('>', 0, out=w) is w True
min
andmax
selectors can be shortcuts for selecting all elements that equal the min or max reduction of all elements.>>> print(v.select('min')) 0| 1| 0 >>> print(v.select('max')) 0| 1 1|
Expand source code
def select(self, op, thunk=None, out=None, mask=None, accum=None, desc=None): """Select elements that match the given select operation condition. See `Matrix.select` for possible operators. >>> v = Vector.from_lists([0,1], [1, 0]) >>> print(v.select('>', 0)) 0| 1 1| >>> w = Vector.sparse(types.UINT8, 2) >>> v.select('>', 0, out=w) is w True `min` and `max` selectors can be shortcuts for selecting all elements that equal the min or max reduction of all elements. >>> print(v.select('min')) 0| 1| 0 >>> print(v.select('max')) 0| 1 1| """ if out is None: out = Vector.sparse(self.type, self.size) if isinstance(op, str): if op == "min": op = lib.GxB_EQ_THUNK thunk = self.min() elif op == "max": op = lib.GxB_EQ_THUNK thunk = self.max() else: op = _get_select_op(op) if thunk is None: thunk = NULL if isinstance(thunk, (bool, int, float, complex)): thunk = Scalar.from_value(thunk) if isinstance(thunk, Scalar): self._keep_alives[self._vector] = thunk thunk = thunk._scalar[0] mask, accum, desc = self._get_args(mask, accum, desc) self._check( lib.GxB_Vector_select( out._vector[0], mask, accum, op, self._vector[0], thunk, desc ) ) return out
def pattern(self, typ=pygraphblas.types.BOOL)
-
Return the pattern of the vector, this is a boolean Vector where every present value in this vector is set to True.
Expand source code
def pattern(self, typ=types.BOOL): """Return the pattern of the vector, this is a boolean Vector where every present value in this vector is set to True. """ result = Vector.sparse(typ, self.size) self.apply(types.BOOL.ONE, out=result) return result
def nonzero(self)
-
Select vector of nonzero entries.
Expand source code
def nonzero(self): """Select vector of nonzero entries.""" return self.select(lib.GxB_NONZERO)
def assign(self, value, index=None, mask=None, accum=None, desc=None)
-
Assign vector to vector.
>>> v = Vector.sparse(types.INT8, 3) >>> w = Vector.from_1_to_n(3) >>> v[:] = w >>> print(v) 0| 1 1| 2 2| 3
If the index is another vector it is used as an assignment mask:
>>> v.clear() >>> m = Vector.sparse(types.BOOL, 3) >>> m[1] = True >>> v[m] = w >>> print(v) 0| 1| 2 2| >>> v.clear() >>> m = Vector.sparse(types.BOOL, 3) >>> m[1] = True >>> v[m] = 3 >>> print(v) 0| 1| 3 2|
Expand source code
def assign(self, value, index=None, mask=None, accum=None, desc=None): """Assign vector to vector. >>> v = Vector.sparse(types.INT8, 3) >>> w = Vector.from_1_to_n(3) >>> v[:] = w >>> print(v) 0| 1 1| 2 2| 3 If the index is another vector it is used as an assignment mask: >>> v.clear() >>> m = Vector.sparse(types.BOOL, 3) >>> m[1] = True >>> v[m] = w >>> print(v) 0| 1| 2 2| >>> v.clear() >>> m = Vector.sparse(types.BOOL, 3) >>> m[1] = True >>> v[m] = 3 >>> print(v) 0| 1| 3 2| """ mask, accum, desc = self._get_args(mask, accum, desc) I, ni, size = _build_range(index, self.size - 1) self._check( lib.GrB_Vector_assign( self._vector[0], mask, accum, value._vector[0], I, ni, desc ) )
def assign_scalar(self, value, index=None, mask=None, accum=None, desc=None)
-
Assign scalar to vector.
>>> v = Vector.sparse(types.INT8, 3) >>> v[:] = 2 >>> print(v) 0| 2 1| 2 2| 2
If the index is another vector it is used as an assignment mask:
>>> v.clear() >>> m = Vector.sparse(types.BOOL, 3) >>> m[1] = True >>> v[m] = 3 >>> print(v) 0| 1| 3 2|
Expand source code
def assign_scalar(self, value, index=None, mask=None, accum=None, desc=None): """Assign scalar to vector. >>> v = Vector.sparse(types.INT8, 3) >>> v[:] = 2 >>> print(v) 0| 2 1| 2 2| 2 If the index is another vector it is used as an assignment mask: >>> v.clear() >>> m = Vector.sparse(types.BOOL, 3) >>> m[1] = True >>> v[m] = 3 >>> print(v) 0| 1| 3 2| """ mask, accum, desc = self._get_args(mask, accum, desc) scalar_type = types._gb_from_type(type(value)) I, ni, size = _build_range(index, self.size - 1) self._check( scalar_type._Vector_assignScalar( self._vector[0], mask, accum, value, I, ni, desc ) )
def extract_element(self, index)
-
Extract element from vector.
Expand source code
def extract_element(self, index): """Extract element from vector.""" result = self.type._ffi.new(self.type._ptr) self._check( self.type._Vector_extractElement( result, self._vector[0], ffi.cast("GrB_Index", index) ) ) return self.type._to_value(result[0])
def extract(self, index, mask=None, accum=None, desc=None)
-
Extract subvector from vector.
Expand source code
def extract(self, index, mask=None, accum=None, desc=None): """Extract subvector from vector.""" mask, accum, desc = self._get_args(mask, accum, desc) if isinstance(index, Vector): mask = index._vector[0] index = slice(None, None, None) I, ni, size = _build_range(index, self.size - 1) if size is None: size = self.size result = Vector.sparse(self.type, size) self._check( lib.GrB_Vector_extract( result._vector[0], mask, accum, self._vector[0], I, ni, desc ) ) return result
def get(self, i, default=None)
-
Get element at
i
or returndefault
if not present.>>> M = Vector.from_lists([1, 2], [42, 149]) >>> M.get(1) 42 >>> M.get(0) is None True >>> M.get(0, 'foo') 'foo'
Expand source code
def get(self, i, default=None): """Get element at `i` or return `default` if not present. >>> M = Vector.from_lists([1, 2], [42, 149]) >>> M.get(1) 42 >>> M.get(0) is None True >>> M.get(0, 'foo') 'foo' """ try: return self[i] except NoValue: return default
def wait(self)
-
Wait for vector to complete.
Expand source code
def wait(self): """Wait for vector to complete.""" self._check(lib.GrB_Vector_wait(self._vector))
def to_string(self, format_string='{:>%s}', width=2, prec=3, empty_char='')
-
Return string representation of vector.
Expand source code
def to_string(self, format_string="{:>%s}", width=2, prec=3, empty_char=""): """Return string representation of vector.""" format_string = format_string % width result = "" for row in range(self.size): value = self.get(row, empty_char) result += str(row) + "|" result += format_string.format( self.type.format_value(value, width, prec) ).rstrip() if row < self.size - 1: result += "\n" return result
def print(self, level=2, name='A', f=sys.stdout)
-
Print the matrix using
GxB_Matrix_fprint()
, by default tosys.stdout
..Level 1: Short description Level 2: Short list, short numbers Level 3: Long list, short number Level 4: Short list, long numbers Level 5: Long list, long numbers
Expand source code
def print(self, level=2, name="A", f=sys.stdout): # pragma: nocover """Print the matrix using `GxB_Matrix_fprint()`, by default to `sys.stdout`.. Level 1: Short description Level 2: Short list, short numbers Level 3: Long list, short number Level 4: Short list, long numbers Level 5: Long list, long numbers """ self._check( lib.GxB_Vector_fprint(self._vector[0], bytes(name, "utf8"), level, f) )
class Scalar (s, typ)
-
GraphBLAS Scalar
Used for now mostly for the
Matrix.select()
.Expand source code
class Scalar: """GraphBLAS Scalar Used for now mostly for the `pygraphblas.Matrix.select`. """ __slots__ = ("_scalar", "type") def __init__(self, s, typ): self._scalar = s self.type = typ def __del__(self): _check(lib.GxB_Scalar_free(self._scalar)) def __len__(self): return self.nvals def dup(self): """Create an duplicate Scalar from the given argument.""" new_sca = ffi.new("GxB_Scalar*") _check(lib.GxB_Scalar_dup(new_sca, self._scalar[0])) return self.__class__(new_sca, self.type) @classmethod def from_type(cls, typ): """Create an empty Scalar from the given type and size.""" new_sca = ffi.new("GxB_Scalar*") _check(lib.GxB_Scalar_new(new_sca, typ._gb_type)) return cls(new_sca, typ) @classmethod def from_value(cls, value): """Create an empty Scalar from the given type and size.""" new_sca = ffi.new("GxB_Scalar*") typ = _gb_from_type(type(value)) _check(lib.GxB_Scalar_new(new_sca, typ._gb_type)) s = cls(new_sca, typ) s[0] = value return s @property def gb_type(self): """Return the GraphBLAS low-level type object of the Scalar.""" return self.type._gb_type def clear(self): """Clear the scalar.""" _check(lib.GxB_Scalar_clear(self._scalar[0])) def __getitem__(self, index): result = ffi.new(self.type._c_type + "*") _check( self.type._Scalar_extractElement(result, self._scalar[0]), raise_no_val=True ) return result[0] def __setitem__(self, index, value): _check( self.type._Scalar_setElement( self._scalar[0], ffi.cast(self.type._c_type, value) ) ) def wait(self): _check(lib.GxB_Scalar_wait(self._scalar)) @property def nvals(self): """Return the number of values in the scalar (0 or 1).""" n = ffi.new("GrB_Index*") _check(lib.GxB_Scalar_nvals(n, self._scalar[0])) return n[0] def __bool__(self): return bool(self.nvals)
Static methods
def from_type(typ)
-
Create an empty Scalar from the given type and size.
Expand source code
@classmethod def from_type(cls, typ): """Create an empty Scalar from the given type and size.""" new_sca = ffi.new("GxB_Scalar*") _check(lib.GxB_Scalar_new(new_sca, typ._gb_type)) return cls(new_sca, typ)
def from_value(value)
-
Create an empty Scalar from the given type and size.
Expand source code
@classmethod def from_value(cls, value): """Create an empty Scalar from the given type and size.""" new_sca = ffi.new("GxB_Scalar*") typ = _gb_from_type(type(value)) _check(lib.GxB_Scalar_new(new_sca, typ._gb_type)) s = cls(new_sca, typ) s[0] = value return s
Instance Attributes
var gb_type
-
Return the GraphBLAS low-level type object of the Scalar.
Expand source code
@property def gb_type(self): """Return the GraphBLAS low-level type object of the Scalar.""" return self.type._gb_type
var nvals
-
Return the number of values in the scalar (0 or 1).
Expand source code
@property def nvals(self): """Return the number of values in the scalar (0 or 1).""" n = ffi.new("GrB_Index*") _check(lib.GxB_Scalar_nvals(n, self._scalar[0])) return n[0]
var type
-
Return an attribute of instance, which is of type owner.
Methods
def dup(self)
-
Create an duplicate Scalar from the given argument.
Expand source code
def dup(self): """Create an duplicate Scalar from the given argument.""" new_sca = ffi.new("GxB_Scalar*") _check(lib.GxB_Scalar_dup(new_sca, self._scalar[0])) return self.__class__(new_sca, self.type)
def clear(self)
-
Clear the scalar.
Expand source code
def clear(self): """Clear the scalar.""" _check(lib.GxB_Scalar_clear(self._scalar[0]))
def wait(self)
-
Expand source code
def wait(self): _check(lib.GxB_Scalar_wait(self._scalar))
class Accum (binaryop)
-
Helper context manager to specify accumulator binary operator in overloaded operator contexts like
@
. This disambiguates for methods likeMatrix.eadd()
andMatrix.emult()
that can specify both a binary operators and a binary accumulator.See those methods and
Matrix.mxm()
for examples.Expand source code
class Accum: """Helper context manager to specify accumulator binary operator in overloaded operator contexts like `@`. This disambiguates for methods like `Matrix.eadd` and `Matrix.emult` that can specify both a binary operators *and* a binary accumulator. See those methods and `Matrix.mxm` for examples. """ __slots__ = ("binaryop", "token") def __init__(self, binaryop): self.binaryop = binaryop def __enter__(self): self.token = current_accum.set(self.binaryop) return self def __exit__(self, *errors): current_accum.reset(self.token) return False
Instance Attributes
var binaryop
-
Return an attribute of instance, which is of type owner.
var token
-
Return an attribute of instance, which is of type owner.
class BOOL
-
GraphBLAS Boolean Type.
Expand source code
class BOOL(Type): """GraphBLAS Boolean Type.""" _gb_type = lib.GrB_BOOL _c_type = "_Bool" default_one = True default_zero = False _typecode = "B" _numba_t = numba.boolean _numpy_t = numpy.bool_ @classmethod def _default_addop(self): # pragma: nocover return self.LOR @classmethod def _default_multop(self): # pragma: nocover return self.LAND @classmethod def _default_semiring(self): # pragma: nocover return self.LOR_LAND @classmethod def format_value(cls, val, width=2, prec=None): f = "{:>%s}" % width if not isinstance(val, bool): return f.format(val) return f.format("t") if val is True else f.format("f") @classmethod def _to_value(cls, cdata): return bool(cdata)
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ]
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype bool size: 1
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: ytype bool size: 1
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var EQ_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var EQ_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var EQ_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var EQ_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var EQ_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ]
var EQ_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype bool size: 1
var EQ_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: ytype bool size: 1
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LAND_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LAND_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LAND_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LAND_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LAND_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ]
var LAND_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype bool size: 1
var LAND_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: ytype bool size: 1
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LOR_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LOR_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LOR_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LOR_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ]
var LOR_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype bool size: 1
var LOR_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: ytype bool size: 1
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LXNOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ]
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LXOR_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LXOR_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LXOR_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LXOR_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var LXOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ]
var LXOR_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype bool size: 1
var LXOR_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: ytype bool size: 1
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype bool size: 1
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype bool size: 1
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: ytype bool size: 1
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1
var abs
var ainv
var any
var any_eq
var any_first
var any_ge
var any_gt
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_monoid
var any_pair
var any_second
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_first
var eq_ge
var eq_gt
var eq_land
var eq_le
var eq_lor
var eq_lt
var eq_lxor
var eq_monoid
var eq_pair
var eq_second
var first
var ge
var gt
var identity
var land
var land_eq
var land_first
var land_ge
var land_gt
var land_land
var land_le
var land_lor
var land_lt
var land_lxor
var land_monoid
var land_pair
var land_second
var le
var lnot
var lor
var lor_eq
var lor_first
var lor_ge
var lor_gt
var lor_land
var lor_le
var lor_lor
var lor_lt
var lor_lxor
var lor_monoid
var lor_pair
var lor_second
var lt
var lxnor_monoid
var lxor
var lxor_eq
var lxor_first
var lxor_ge
var lxor_gt
var lxor_land
var lxor_le
var lxor_lor
var lxor_lt
var lxor_lxor
var lxor_monoid
var lxor_pair
var lxor_second
var max
var min
var minus
var minv
var ne
var one
var pair
var plus
var pow
var rdiv
var rminus
var second
var times
Static methods
def format_value(val, width=2, prec=None)
-
Return the value as a formatted string for display.
Expand source code
@classmethod def format_value(cls, val, width=2, prec=None): f = "{:>%s}" % width if not isinstance(val, bool): return f.format(val) return f.format("t") if val is True else f.format("f")
class FP64
-
GraphBLAS 64 bit float.
Expand source code
class FP64(Type): """GraphBLAS 64 bit float.""" default_one = 1.0 default_zero = 0.0 _gb_type = lib.GrB_FP64 _c_type = "double" _typecode = "d" _numba_t = numba.float64 _numpy_t = numpy.float64 @classmethod def format_value(cls, val, width=2, prec=2): return f"{val:>{width}.{prec}}"
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var ACOS
-
GraphBLAS UnaryOp: (built-in) z=acos(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var ACOSH
-
GraphBLAS UnaryOp: (built-in) z=acosh(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ]
var ANY_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype double size: 8
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: ytype double size: 8
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ASIN
-
GraphBLAS UnaryOp: (built-in) z=asin(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var ASINH
-
GraphBLAS UnaryOp: (built-in) z=asinh(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var ATAN
-
GraphBLAS UnaryOp: (built-in) z=atan(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var ATAN2
-
GraphBLAS BinaryOp: (built-in) z=atan2(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ATANH
-
GraphBLAS UnaryOp: (built-in) z=atanh(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var CEIL
-
GraphBLAS UnaryOp: (built-in) z=ceil(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var CMPLX
-
GraphBLAS BinaryOp: (built-in) z=cmplx(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var COPYSIGN
-
GraphBLAS BinaryOp: (built-in) z=copysign(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var COS
-
GraphBLAS UnaryOp: (built-in) z=cos(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var COSH
-
GraphBLAS UnaryOp: (built-in) z=cosh(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var EQ_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ERF
-
GraphBLAS UnaryOp: (built-in) z=erf(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var ERFC
-
GraphBLAS UnaryOp: (built-in) z=erfc(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var EXP
-
GraphBLAS UnaryOp: (built-in) z=exp(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var EXP2
-
GraphBLAS UnaryOp: (built-in) z=exp2(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var EXPM1
-
GraphBLAS UnaryOp: (built-in) z=expm1(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var FLOOR
-
GraphBLAS UnaryOp: (built-in) z=floor(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var FMOD
-
GraphBLAS BinaryOp: (built-in) z=fmod(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var FREXPE
-
GraphBLAS UnaryOp: (built-in) z=frexpe(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var FREXPX
-
GraphBLAS UnaryOp: (built-in) z=frexpx(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var HYPOT
-
GraphBLAS BinaryOp: (built-in) z=hypot(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var ISFINITE
-
GraphBLAS UnaryOp: (built-in) z=isfinite(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8
var ISINF
-
GraphBLAS UnaryOp: (built-in) z=isinf(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8
var ISNAN
-
GraphBLAS UnaryOp: (built-in) z=isnan(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LAND_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LDEXP
-
GraphBLAS BinaryOp: (built-in) z=ldexp(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LGAMMA
-
GraphBLAS UnaryOp: (built-in) z=lgamma(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var LOG
-
GraphBLAS UnaryOp: (built-in) z=log(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var LOG1P
-
GraphBLAS UnaryOp: (built-in) z=log1p(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var LOG2
-
GraphBLAS UnaryOp: (built-in) z=log2(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var LXOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var MAX_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ]
var MAX_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype double size: 8
var MAX_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: ytype double size: 8
var MAX_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var MIN_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var MIN_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ]
var MIN_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype double size: 8
var MIN_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: ytype double size: 8
var MIN_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype double size: 8
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype double size: 8
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var PLUS_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype double size: 8
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: ytype double size: 8
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var REMAINDER
-
GraphBLAS BinaryOp: (built-in) z=remainder(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var ROUND
-
GraphBLAS UnaryOp: (built-in) z=round(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: ytype double size: 8
var SIGNUM
-
GraphBLAS UnaryOp: (built-in) z=signum(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var SIN
-
GraphBLAS UnaryOp: (built-in) z=sin(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var SINH
-
GraphBLAS UnaryOp: (built-in) z=sinh(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var SQRT
-
GraphBLAS UnaryOp: (built-in) z=sqrt(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var TAN
-
GraphBLAS UnaryOp: (built-in) z=tan(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var TANH
-
GraphBLAS UnaryOp: (built-in) z=tanh(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var TGAMMA
-
GraphBLAS UnaryOp: (built-in) z=tgamma(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var TIMES_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype double size: 8
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: ytype double size: 8
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8 GraphBLAS type: ytype double size: 8
var TRUNC
-
GraphBLAS UnaryOp: (built-in) z=trunc(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double size: 8
var abs
var acos
var acosh
var ainv
var any
var any_div
var any_eq
var any_first
var any_ge
var any_gt
var any_iseq
var any_isge
var any_isgt
var any_isle
var any_islt
var any_isne
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_max
var any_min
var any_minus
var any_monoid
var any_ne
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_times
var asin
var asinh
var atan
var atan2
var atanh
var ceil
var cmplx
var copysign
var cos
var cosh
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_ge
var eq_gt
var eq_le
var eq_lt
var eq_ne
var erf
var erfc
var exp
var exp2
var expm1
var first
var floor
var fmod
var frexpe
var frexpx
var ge
var gt
var hypot
var identity
var isfinite
var isinf
var isnan
var land
var land_eq
var land_ge
var land_gt
var land_le
var land_lt
var land_ne
var ldexp
var le
var lgamma
var lnot
var log
var log1p
var log2
var lor
var lor_eq
var lor_ge
var lor_gt
var lor_le
var lor_lt
var lor_ne
var lt
var lxor
var lxor_eq
var lxor_ge
var lxor_gt
var lxor_le
var lxor_lt
var lxor_ne
var max
var max_div
var max_first
var max_iseq
var max_isge
var max_isgt
var max_isle
var max_islt
var max_isne
var max_land
var max_lor
var max_lxor
var max_max
var max_min
var max_minus
var max_monoid
var max_pair
var max_plus
var max_rdiv
var max_rminus
var max_second
var max_times
var min
var min_div
var min_first
var min_iseq
var min_isge
var min_isgt
var min_isle
var min_islt
var min_isne
var min_land
var min_lor
var min_lxor
var min_max
var min_min
var min_minus
var min_monoid
var min_pair
var min_plus
var min_rdiv
var min_rminus
var min_second
var min_times
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_iseq
var plus_isge
var plus_isgt
var plus_isle
var plus_islt
var plus_isne
var plus_land
var plus_lor
var plus_lxor
var plus_max
var plus_min
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_times
var pow
var rdiv
var remainder
var rminus
var round
var second
var signum
var sin
var sinh
var sqrt
var tan
var tanh
var tgamma
var times
var times_div
var times_first
var times_iseq
var times_isge
var times_isgt
var times_isle
var times_islt
var times_isne
var times_land
var times_lor
var times_lxor
var times_max
var times_min
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_times
var trunc
Static methods
def format_value(val, width=2, prec=2)
-
Return the value as a formatted string for display.
Expand source code
@classmethod def format_value(cls, val, width=2, prec=2): return f"{val:>{width}.{prec}}"
class FP32
-
GraphBLAS 32 bit float.
Expand source code
class FP32(Type): """GraphBLAS 32 bit float.""" default_one = 1.0 default_zero = 0.0 _gb_type = lib.GrB_FP32 _c_type = "float" _typecode = "f" _numba_t = numba.float32 _numpy_t = numpy.float32 @classmethod def format_value(cls, val, width=2, prec=2): return f"{val:>{width}.{prec}}"
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var ACOS
-
GraphBLAS UnaryOp: (built-in) z=acos(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var ACOSH
-
GraphBLAS UnaryOp: (built-in) z=acosh(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ]
var ANY_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype float size: 4
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: ytype float size: 4
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ASIN
-
GraphBLAS UnaryOp: (built-in) z=asin(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var ASINH
-
GraphBLAS UnaryOp: (built-in) z=asinh(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var ATAN
-
GraphBLAS UnaryOp: (built-in) z=atan(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var ATAN2
-
GraphBLAS BinaryOp: (built-in) z=atan2(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ATANH
-
GraphBLAS UnaryOp: (built-in) z=atanh(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var CEIL
-
GraphBLAS UnaryOp: (built-in) z=ceil(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var CMPLX
-
GraphBLAS BinaryOp: (built-in) z=cmplx(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var COPYSIGN
-
GraphBLAS BinaryOp: (built-in) z=copysign(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var COS
-
GraphBLAS UnaryOp: (built-in) z=cos(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var COSH
-
GraphBLAS UnaryOp: (built-in) z=cosh(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var EQ_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ERF
-
GraphBLAS UnaryOp: (built-in) z=erf(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var ERFC
-
GraphBLAS UnaryOp: (built-in) z=erfc(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var EXP
-
GraphBLAS UnaryOp: (built-in) z=exp(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var EXP2
-
GraphBLAS UnaryOp: (built-in) z=exp2(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var EXPM1
-
GraphBLAS UnaryOp: (built-in) z=expm1(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var FLOOR
-
GraphBLAS UnaryOp: (built-in) z=floor(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var FMOD
-
GraphBLAS BinaryOp: (built-in) z=fmod(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var FREXPE
-
GraphBLAS UnaryOp: (built-in) z=frexpe(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var FREXPX
-
GraphBLAS UnaryOp: (built-in) z=frexpx(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var HYPOT
-
GraphBLAS BinaryOp: (built-in) z=hypot(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var ISFINITE
-
GraphBLAS UnaryOp: (built-in) z=isfinite(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4
var ISINF
-
GraphBLAS UnaryOp: (built-in) z=isinf(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4
var ISNAN
-
GraphBLAS UnaryOp: (built-in) z=isnan(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LAND_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LDEXP
-
GraphBLAS BinaryOp: (built-in) z=ldexp(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LGAMMA
-
GraphBLAS UnaryOp: (built-in) z=lgamma(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var LOG
-
GraphBLAS UnaryOp: (built-in) z=log(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var LOG1P
-
GraphBLAS UnaryOp: (built-in) z=log1p(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var LOG2
-
GraphBLAS UnaryOp: (built-in) z=log2(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var LXOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var MAX_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ]
var MAX_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype float size: 4
var MAX_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: ytype float size: 4
var MAX_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ -Inf ] terminal: [ Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var MIN_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var MIN_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ]
var MIN_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype float size: 4
var MIN_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: ytype float size: 4
var MIN_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ Inf ] terminal: [ -Inf ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype float size: 4
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype float size: 4
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var PLUS_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype float size: 4
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: ytype float size: 4
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var REMAINDER
-
GraphBLAS BinaryOp: (built-in) z=remainder(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var ROUND
-
GraphBLAS UnaryOp: (built-in) z=round(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: ytype float size: 4
var SIGNUM
-
GraphBLAS UnaryOp: (built-in) z=signum(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var SIN
-
GraphBLAS UnaryOp: (built-in) z=sin(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var SINH
-
GraphBLAS UnaryOp: (built-in) z=sinh(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var SQRT
-
GraphBLAS UnaryOp: (built-in) z=sqrt(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var TAN
-
GraphBLAS UnaryOp: (built-in) z=tan(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var TANH
-
GraphBLAS UnaryOp: (built-in) z=tanh(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var TGAMMA
-
GraphBLAS UnaryOp: (built-in) z=tgamma(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var TIMES_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype float size: 4
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: ytype float size: 4
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4 GraphBLAS type: ytype float size: 4
var TRUNC
-
GraphBLAS UnaryOp: (built-in) z=trunc(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float size: 4
var abs
var acos
var acosh
var ainv
var any
var any_div
var any_eq
var any_first
var any_ge
var any_gt
var any_iseq
var any_isge
var any_isgt
var any_isle
var any_islt
var any_isne
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_max
var any_min
var any_minus
var any_monoid
var any_ne
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_times
var asin
var asinh
var atan
var atan2
var atanh
var ceil
var cmplx
var copysign
var cos
var cosh
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_ge
var eq_gt
var eq_le
var eq_lt
var eq_ne
var erf
var erfc
var exp
var exp2
var expm1
var first
var floor
var fmod
var frexpe
var frexpx
var ge
var gt
var hypot
var identity
var isfinite
var isinf
var isnan
var land
var land_eq
var land_ge
var land_gt
var land_le
var land_lt
var land_ne
var ldexp
var le
var lgamma
var lnot
var log
var log1p
var log2
var lor
var lor_eq
var lor_ge
var lor_gt
var lor_le
var lor_lt
var lor_ne
var lt
var lxor
var lxor_eq
var lxor_ge
var lxor_gt
var lxor_le
var lxor_lt
var lxor_ne
var max
var max_div
var max_first
var max_iseq
var max_isge
var max_isgt
var max_isle
var max_islt
var max_isne
var max_land
var max_lor
var max_lxor
var max_max
var max_min
var max_minus
var max_monoid
var max_pair
var max_plus
var max_rdiv
var max_rminus
var max_second
var max_times
var min
var min_div
var min_first
var min_iseq
var min_isge
var min_isgt
var min_isle
var min_islt
var min_isne
var min_land
var min_lor
var min_lxor
var min_max
var min_min
var min_minus
var min_monoid
var min_pair
var min_plus
var min_rdiv
var min_rminus
var min_second
var min_times
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_iseq
var plus_isge
var plus_isgt
var plus_isle
var plus_islt
var plus_isne
var plus_land
var plus_lor
var plus_lxor
var plus_max
var plus_min
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_times
var pow
var rdiv
var remainder
var rminus
var round
var second
var signum
var sin
var sinh
var sqrt
var tan
var tanh
var tgamma
var times
var times_div
var times_first
var times_iseq
var times_isge
var times_isgt
var times_isle
var times_islt
var times_isne
var times_land
var times_lor
var times_lxor
var times_max
var times_min
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_times
var trunc
Static methods
def format_value(val, width=2, prec=2)
-
Return the value as a formatted string for display.
Expand source code
@classmethod def format_value(cls, val, width=2, prec=2): return f"{val:>{width}.{prec}}"
class FC64
-
GraphBLAS 64 bit float complex.
Expand source code
class FC64(Type): """GraphBLAS 64 bit float complex.""" default_one = complex(1.0) default_zero = complex(0.0) _gb_type = lib.GxB_FC64 _c_type = "double _Complex" _numba_t = numba.complex128 _numpy_t = numpy.complex128
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double complex size: 16
var ACOS
-
GraphBLAS UnaryOp: (built-in) z=acos(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var ACOSH
-
GraphBLAS UnaryOp: (built-in) z=acosh(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] terminal: [ 0 + 0i ]
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype double complex size: 16
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var ASIN
-
GraphBLAS UnaryOp: (built-in) z=asin(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var ASINH
-
GraphBLAS UnaryOp: (built-in) z=asinh(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var ATAN
-
GraphBLAS UnaryOp: (built-in) z=atan(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var ATANH
-
GraphBLAS UnaryOp: (built-in) z=atanh(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var CARG
-
GraphBLAS UnaryOp: (built-in) z=carg(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double complex size: 16
var CEIL
-
GraphBLAS UnaryOp: (built-in) z=ceil(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var CIMAG
-
GraphBLAS UnaryOp: (built-in) z=cimag(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double complex size: 16
var CONJ
-
GraphBLAS UnaryOp: (built-in) z=conj(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var COS
-
GraphBLAS UnaryOp: (built-in) z=cos(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var COSH
-
GraphBLAS UnaryOp: (built-in) z=cosh(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var CREAL
-
GraphBLAS UnaryOp: (built-in) z=creal(x) GraphBLAS type: ztype double size: 8 GraphBLAS type: xtype double complex size: 16
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var EXP
-
GraphBLAS UnaryOp: (built-in) z=exp(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var EXP2
-
GraphBLAS UnaryOp: (built-in) z=exp2(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var EXPM1
-
GraphBLAS UnaryOp: (built-in) z=expm1(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var FLOOR
-
GraphBLAS UnaryOp: (built-in) z=floor(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var ISFINITE
-
GraphBLAS UnaryOp: (built-in) z=isfinite(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double complex size: 16
var ISINF
-
GraphBLAS UnaryOp: (built-in) z=isinf(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double complex size: 16
var ISNAN
-
GraphBLAS UnaryOp: (built-in) z=isnan(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double complex size: 16
var LOG
-
GraphBLAS UnaryOp: (built-in) z=log(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var LOG1P
-
GraphBLAS UnaryOp: (built-in) z=log1p(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var LOG2
-
GraphBLAS UnaryOp: (built-in) z=log2(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype double complex size: 16
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype double complex size: 16
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype double complex size: 16
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var ROUND
-
GraphBLAS UnaryOp: (built-in) z=round(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var SIGNUM
-
GraphBLAS UnaryOp: (built-in) z=signum(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var SIN
-
GraphBLAS UnaryOp: (built-in) z=sin(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var SINH
-
GraphBLAS UnaryOp: (built-in) z=sinh(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var SQRT
-
GraphBLAS UnaryOp: (built-in) z=sqrt(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var TAN
-
GraphBLAS UnaryOp: (built-in) z=tan(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var TANH
-
GraphBLAS UnaryOp: (built-in) z=tanh(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 1 + 0i ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype double complex size: 16
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16 GraphBLAS type: ytype double complex size: 16
var TRUNC
-
GraphBLAS UnaryOp: (built-in) z=trunc(x) GraphBLAS type: ztype double complex size: 16 GraphBLAS type: xtype double complex size: 16
var abs
var acos
var acosh
var ainv
var any
var any_div
var any_first
var any_minus
var any_monoid
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_times
var asin
var asinh
var atan
var atanh
var carg
var ceil
var cimag
var conj
var cos
var cosh
var creal
var default_one
var default_zero
var div
var eq
var exp
var exp2
var expm1
var first
var floor
var identity
var isfinite
var isinf
var isnan
var log
var log1p
var log2
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_times
var pow
var rdiv
var rminus
var round
var second
var signum
var sin
var sinh
var sqrt
var tan
var tanh
var times
var times_div
var times_first
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_times
var trunc
class FC32
-
GraphBLAS 32 bit float complex.
Expand source code
class FC32(Type): """GraphBLAS 32 bit float complex.""" default_one = complex(1.0) default_zero = complex(0.0) _gb_type = lib.GxB_FC32 _c_type = "float _Complex" _numba_t = numba.complex64 _numpy_t = numpy.complex64
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float complex size: 8
var ACOS
-
GraphBLAS UnaryOp: (built-in) z=acos(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var ACOSH
-
GraphBLAS UnaryOp: (built-in) z=acosh(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] terminal: [ 0 + 0i ]
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype float complex size: 8
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] terminal: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var ASIN
-
GraphBLAS UnaryOp: (built-in) z=asin(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var ASINH
-
GraphBLAS UnaryOp: (built-in) z=asinh(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var ATAN
-
GraphBLAS UnaryOp: (built-in) z=atan(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var ATANH
-
GraphBLAS UnaryOp: (built-in) z=atanh(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var CARG
-
GraphBLAS UnaryOp: (built-in) z=carg(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float complex size: 8
var CEIL
-
GraphBLAS UnaryOp: (built-in) z=ceil(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var CIMAG
-
GraphBLAS UnaryOp: (built-in) z=cimag(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float complex size: 8
var CONJ
-
GraphBLAS UnaryOp: (built-in) z=conj(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var COS
-
GraphBLAS UnaryOp: (built-in) z=cos(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var COSH
-
GraphBLAS UnaryOp: (built-in) z=cosh(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var CREAL
-
GraphBLAS UnaryOp: (built-in) z=creal(x) GraphBLAS type: ztype float size: 4 GraphBLAS type: xtype float complex size: 8
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var EXP
-
GraphBLAS UnaryOp: (built-in) z=exp(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var EXP2
-
GraphBLAS UnaryOp: (built-in) z=exp2(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var EXPM1
-
GraphBLAS UnaryOp: (built-in) z=expm1(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var FLOOR
-
GraphBLAS UnaryOp: (built-in) z=floor(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var ISFINITE
-
GraphBLAS UnaryOp: (built-in) z=isfinite(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float complex size: 8
var ISINF
-
GraphBLAS UnaryOp: (built-in) z=isinf(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float complex size: 8
var ISNAN
-
GraphBLAS UnaryOp: (built-in) z=isnan(x) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float complex size: 8
var LOG
-
GraphBLAS UnaryOp: (built-in) z=log(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var LOG1P
-
GraphBLAS UnaryOp: (built-in) z=log1p(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var LOG2
-
GraphBLAS UnaryOp: (built-in) z=log2(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype float complex size: 8
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype float complex size: 8
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype float complex size: 8
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 0 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var ROUND
-
GraphBLAS UnaryOp: (built-in) z=round(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var SIGNUM
-
GraphBLAS UnaryOp: (built-in) z=signum(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var SIN
-
GraphBLAS UnaryOp: (built-in) z=sin(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var SINH
-
GraphBLAS UnaryOp: (built-in) z=sinh(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var SQRT
-
GraphBLAS UnaryOp: (built-in) z=sqrt(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var TAN
-
GraphBLAS UnaryOp: (built-in) z=tan(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var TANH
-
GraphBLAS UnaryOp: (built-in) z=tanh(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 1 + 0i ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype float complex size: 8
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8 identity: [ 1 + 0i ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8 GraphBLAS type: ytype float complex size: 8
var TRUNC
-
GraphBLAS UnaryOp: (built-in) z=trunc(x) GraphBLAS type: ztype float complex size: 8 GraphBLAS type: xtype float complex size: 8
var abs
var acos
var acosh
var ainv
var any
var any_div
var any_first
var any_minus
var any_monoid
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_times
var asin
var asinh
var atan
var atanh
var carg
var ceil
var cimag
var conj
var cos
var cosh
var creal
var default_one
var default_zero
var div
var eq
var exp
var exp2
var expm1
var first
var floor
var identity
var isfinite
var isinf
var isnan
var log
var log1p
var log2
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_times
var pow
var rdiv
var rminus
var round
var second
var signum
var sin
var sinh
var sqrt
var tan
var tanh
var times
var times_div
var times_first
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_times
var trunc
class INT64
-
GraphBLAS 64 bit signed integer.
Expand source code
class INT64(Type): """GraphBLAS 64 bit signed integer.""" _gb_type = lib.GrB_INT64 _c_type = "int64_t" _typecode = "q" _numba_t = numba.int64 _numpy_t = numpy.int64
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var ANY_FIRSTI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti(x,y) GraphBLAS type: ztype int64_t size: 8
var ANY_FIRSTI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti1(x,y) GraphBLAS type: ztype int64_t size: 8
var ANY_FIRSTJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj(x,y) GraphBLAS type: ztype int64_t size: 8
var ANY_FIRSTJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj1(x,y) GraphBLAS type: ztype int64_t size: 8
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ]
var ANY_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int64_t size: 8
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ANY_SECONDI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi(x,y) GraphBLAS type: ztype int64_t size: 8
var ANY_SECONDI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi1(x,y) GraphBLAS type: ztype int64_t size: 8
var ANY_SECONDJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj(x,y) GraphBLAS type: ztype int64_t size: 8
var ANY_SECONDJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj1(x,y) GraphBLAS type: ztype int64_t size: 8
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var BAND
-
GraphBLAS BinaryOp: (built-in) z=bitand(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var BCLR
-
GraphBLAS BinaryOp: (built-in) z=bitclear(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var BGET
-
GraphBLAS BinaryOp: (built-in) z=bitget(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var BOR
-
GraphBLAS BinaryOp: (built-in) z=bitor(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var BSET
-
GraphBLAS BinaryOp: (built-in) z=bitset(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var BSHIFT
-
GraphBLAS BinaryOp: (built-in) z=bitshift(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int8_t size: 1
var BXNOR
-
GraphBLAS BinaryOp: (built-in) z=bitxnor(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var BXOR
-
GraphBLAS BinaryOp: (built-in) z=bitxor(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var EQ_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var FIRSTI
-
GraphBLAS BinaryOp: (built-in) z=firsti(x,y) GraphBLAS type: ztype int64_t size: 8
var FIRSTI1
-
GraphBLAS BinaryOp: (built-in) z=firsti1(x,y) GraphBLAS type: ztype int64_t size: 8
var FIRSTJ
-
GraphBLAS BinaryOp: (built-in) z=firstj(x,y) GraphBLAS type: ztype int64_t size: 8
var FIRSTJ1
-
GraphBLAS BinaryOp: (built-in) z=firstj1(x,y) GraphBLAS type: ztype int64_t size: 8
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LAND_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var LXOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var MAX_FIRSTI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti(x,y) GraphBLAS type: ztype int64_t size: 8
var MAX_FIRSTI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti1(x,y) GraphBLAS type: ztype int64_t size: 8
var MAX_FIRSTJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj(x,y) GraphBLAS type: ztype int64_t size: 8
var MAX_FIRSTJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj1(x,y) GraphBLAS type: ztype int64_t size: 8
var MAX_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ]
var MAX_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int64_t size: 8
var MAX_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MAX_SECONDI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi(x,y) GraphBLAS type: ztype int64_t size: 8
var MAX_SECONDI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi1(x,y) GraphBLAS type: ztype int64_t size: 8
var MAX_SECONDJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj(x,y) GraphBLAS type: ztype int64_t size: 8
var MAX_SECONDJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj1(x,y) GraphBLAS type: ztype int64_t size: 8
var MAX_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ -9223372036854775808 ] terminal: [ 9223372036854775807 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var MIN_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var MIN_FIRSTI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti(x,y) GraphBLAS type: ztype int64_t size: 8
var MIN_FIRSTI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti1(x,y) GraphBLAS type: ztype int64_t size: 8
var MIN_FIRSTJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj(x,y) GraphBLAS type: ztype int64_t size: 8
var MIN_FIRSTJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj1(x,y) GraphBLAS type: ztype int64_t size: 8
var MIN_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ]
var MIN_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int64_t size: 8
var MIN_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var MIN_SECONDI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi(x,y) GraphBLAS type: ztype int64_t size: 8
var MIN_SECONDI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi1(x,y) GraphBLAS type: ztype int64_t size: 8
var MIN_SECONDJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj(x,y) GraphBLAS type: ztype int64_t size: 8
var MIN_SECONDJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj1(x,y) GraphBLAS type: ztype int64_t size: 8
var MIN_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 9223372036854775807 ] terminal: [ -9223372036854775808 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype int64_t size: 8
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype int64_t size: 8
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var PLUS_FIRSTI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti(x,y) GraphBLAS type: ztype int64_t size: 8
var PLUS_FIRSTI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti1(x,y) GraphBLAS type: ztype int64_t size: 8
var PLUS_FIRSTJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj(x,y) GraphBLAS type: ztype int64_t size: 8
var PLUS_FIRSTJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj1(x,y) GraphBLAS type: ztype int64_t size: 8
var PLUS_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int64_t size: 8
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var PLUS_SECONDI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi(x,y) GraphBLAS type: ztype int64_t size: 8
var PLUS_SECONDI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi1(x,y) GraphBLAS type: ztype int64_t size: 8
var PLUS_SECONDJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj(x,y) GraphBLAS type: ztype int64_t size: 8
var PLUS_SECONDJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj1(x,y) GraphBLAS type: ztype int64_t size: 8
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var POSITIONI
-
GraphBLAS UnaryOp: (built-in) z=positioni(x) GraphBLAS type: ztype int64_t size: 8
var POSITIONI1
-
GraphBLAS UnaryOp: (built-in) z=positioni1(x) GraphBLAS type: ztype int64_t size: 8
var POSITIONJ
-
GraphBLAS UnaryOp: (built-in) z=positionj(x) GraphBLAS type: ztype int64_t size: 8
var POSITIONJ1
-
GraphBLAS UnaryOp: (built-in) z=positionj1(x) GraphBLAS type: ztype int64_t size: 8
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var SECONDI
-
GraphBLAS BinaryOp: (built-in) z=secondi(x,y) GraphBLAS type: ztype int64_t size: 8
var SECONDI1
-
GraphBLAS BinaryOp: (built-in) z=secondi1(x,y) GraphBLAS type: ztype int64_t size: 8
var SECONDJ
-
GraphBLAS BinaryOp: (built-in) z=secondj(x,y) GraphBLAS type: ztype int64_t size: 8
var SECONDJ1
-
GraphBLAS BinaryOp: (built-in) z=secondj1(x,y) GraphBLAS type: ztype int64_t size: 8
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8
var TIMES_FIRSTI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti(x,y) GraphBLAS type: ztype int64_t size: 8
var TIMES_FIRSTI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti1(x,y) GraphBLAS type: ztype int64_t size: 8
var TIMES_FIRSTJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj(x,y) GraphBLAS type: ztype int64_t size: 8
var TIMES_FIRSTJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj1(x,y) GraphBLAS type: ztype int64_t size: 8
var TIMES_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int64_t size: 8
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var TIMES_SECONDI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi(x,y) GraphBLAS type: ztype int64_t size: 8
var TIMES_SECONDI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi1(x,y) GraphBLAS type: ztype int64_t size: 8
var TIMES_SECONDJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj(x,y) GraphBLAS type: ztype int64_t size: 8
var TIMES_SECONDJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj1(x,y) GraphBLAS type: ztype int64_t size: 8
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int64_t size: 8 GraphBLAS type: xtype int64_t size: 8 GraphBLAS type: ytype int64_t size: 8
var abs
var ainv
var any
var any_div
var any_eq
var any_first
var any_firsti
var any_firsti1
var any_firstj
var any_firstj1
var any_ge
var any_gt
var any_iseq
var any_isge
var any_isgt
var any_isle
var any_islt
var any_isne
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_max
var any_min
var any_minus
var any_monoid
var any_ne
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_secondi
var any_secondi1
var any_secondj
var any_secondj1
var any_times
var band
var bclr
var bget
var bor
var bset
var bshift
var bxnor
var bxor
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_ge
var eq_gt
var eq_le
var eq_lt
var eq_ne
var first
var firsti
var firsti1
var firstj
var firstj1
var ge
var gt
var identity
var land
var land_eq
var land_ge
var land_gt
var land_le
var land_lt
var land_ne
var le
var lnot
var lor
var lor_eq
var lor_ge
var lor_gt
var lor_le
var lor_lt
var lor_ne
var lt
var lxor
var lxor_eq
var lxor_ge
var lxor_gt
var lxor_le
var lxor_lt
var lxor_ne
var max
var max_div
var max_first
var max_firsti
var max_firsti1
var max_firstj
var max_firstj1
var max_iseq
var max_isge
var max_isgt
var max_isle
var max_islt
var max_isne
var max_land
var max_lor
var max_lxor
var max_max
var max_min
var max_minus
var max_monoid
var max_pair
var max_plus
var max_rdiv
var max_rminus
var max_second
var max_secondi
var max_secondi1
var max_secondj
var max_secondj1
var max_times
var min
var min_div
var min_first
var min_firsti
var min_firsti1
var min_firstj
var min_firstj1
var min_iseq
var min_isge
var min_isgt
var min_isle
var min_islt
var min_isne
var min_land
var min_lor
var min_lxor
var min_max
var min_min
var min_minus
var min_monoid
var min_pair
var min_plus
var min_rdiv
var min_rminus
var min_second
var min_secondi
var min_secondi1
var min_secondj
var min_secondj1
var min_times
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_firsti
var plus_firsti1
var plus_firstj
var plus_firstj1
var plus_iseq
var plus_isge
var plus_isgt
var plus_isle
var plus_islt
var plus_isne
var plus_land
var plus_lor
var plus_lxor
var plus_max
var plus_min
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_secondi
var plus_secondi1
var plus_secondj
var plus_secondj1
var plus_times
var positioni
var positioni1
var positionj
var positionj1
var pow
var rdiv
var rminus
var second
var secondi
var secondi1
var secondj
var secondj1
var times
var times_div
var times_first
var times_firsti
var times_firsti1
var times_firstj
var times_firstj1
var times_iseq
var times_isge
var times_isgt
var times_isle
var times_islt
var times_isne
var times_land
var times_lor
var times_lxor
var times_max
var times_min
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_secondi
var times_secondi1
var times_secondj
var times_secondj1
var times_times
class INT32
-
GraphBLAS 32 bit signed integer.
Expand source code
class INT32(Type): """GraphBLAS 32 bit signed integer.""" _gb_type = lib.GrB_INT32 _c_type = "int32_t" _typecode = "l" _numba_t = numba.int32 _numpy_t = numpy.int32
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var ANY_FIRSTI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti(x,y) GraphBLAS type: ztype int32_t size: 4
var ANY_FIRSTI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti1(x,y) GraphBLAS type: ztype int32_t size: 4
var ANY_FIRSTJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj(x,y) GraphBLAS type: ztype int32_t size: 4
var ANY_FIRSTJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj1(x,y) GraphBLAS type: ztype int32_t size: 4
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ]
var ANY_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int32_t size: 4
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ANY_SECONDI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi(x,y) GraphBLAS type: ztype int32_t size: 4
var ANY_SECONDI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi1(x,y) GraphBLAS type: ztype int32_t size: 4
var ANY_SECONDJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj(x,y) GraphBLAS type: ztype int32_t size: 4
var ANY_SECONDJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj1(x,y) GraphBLAS type: ztype int32_t size: 4
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var BAND
-
GraphBLAS BinaryOp: (built-in) z=bitand(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var BCLR
-
GraphBLAS BinaryOp: (built-in) z=bitclear(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var BGET
-
GraphBLAS BinaryOp: (built-in) z=bitget(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var BOR
-
GraphBLAS BinaryOp: (built-in) z=bitor(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var BSET
-
GraphBLAS BinaryOp: (built-in) z=bitset(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var BSHIFT
-
GraphBLAS BinaryOp: (built-in) z=bitshift(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int8_t size: 1
var BXNOR
-
GraphBLAS BinaryOp: (built-in) z=bitxnor(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var BXOR
-
GraphBLAS BinaryOp: (built-in) z=bitxor(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var EQ_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var FIRSTI
-
GraphBLAS BinaryOp: (built-in) z=firsti(x,y) GraphBLAS type: ztype int32_t size: 4
var FIRSTI1
-
GraphBLAS BinaryOp: (built-in) z=firsti1(x,y) GraphBLAS type: ztype int32_t size: 4
var FIRSTJ
-
GraphBLAS BinaryOp: (built-in) z=firstj(x,y) GraphBLAS type: ztype int32_t size: 4
var FIRSTJ1
-
GraphBLAS BinaryOp: (built-in) z=firstj1(x,y) GraphBLAS type: ztype int32_t size: 4
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LAND_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var LXOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var MAX_FIRSTI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti(x,y) GraphBLAS type: ztype int32_t size: 4
var MAX_FIRSTI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti1(x,y) GraphBLAS type: ztype int32_t size: 4
var MAX_FIRSTJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj(x,y) GraphBLAS type: ztype int32_t size: 4
var MAX_FIRSTJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj1(x,y) GraphBLAS type: ztype int32_t size: 4
var MAX_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ]
var MAX_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int32_t size: 4
var MAX_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MAX_SECONDI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi(x,y) GraphBLAS type: ztype int32_t size: 4
var MAX_SECONDI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi1(x,y) GraphBLAS type: ztype int32_t size: 4
var MAX_SECONDJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj(x,y) GraphBLAS type: ztype int32_t size: 4
var MAX_SECONDJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj1(x,y) GraphBLAS type: ztype int32_t size: 4
var MAX_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ -2147483648 ] terminal: [ 2147483647 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var MIN_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var MIN_FIRSTI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti(x,y) GraphBLAS type: ztype int32_t size: 4
var MIN_FIRSTI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti1(x,y) GraphBLAS type: ztype int32_t size: 4
var MIN_FIRSTJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj(x,y) GraphBLAS type: ztype int32_t size: 4
var MIN_FIRSTJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj1(x,y) GraphBLAS type: ztype int32_t size: 4
var MIN_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ]
var MIN_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int32_t size: 4
var MIN_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var MIN_SECONDI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi(x,y) GraphBLAS type: ztype int32_t size: 4
var MIN_SECONDI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi1(x,y) GraphBLAS type: ztype int32_t size: 4
var MIN_SECONDJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj(x,y) GraphBLAS type: ztype int32_t size: 4
var MIN_SECONDJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj1(x,y) GraphBLAS type: ztype int32_t size: 4
var MIN_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 2147483647 ] terminal: [ -2147483648 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype int32_t size: 4
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype int32_t size: 4
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var PLUS_FIRSTI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti(x,y) GraphBLAS type: ztype int32_t size: 4
var PLUS_FIRSTI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti1(x,y) GraphBLAS type: ztype int32_t size: 4
var PLUS_FIRSTJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj(x,y) GraphBLAS type: ztype int32_t size: 4
var PLUS_FIRSTJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj1(x,y) GraphBLAS type: ztype int32_t size: 4
var PLUS_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int32_t size: 4
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var PLUS_SECONDI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi(x,y) GraphBLAS type: ztype int32_t size: 4
var PLUS_SECONDI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi1(x,y) GraphBLAS type: ztype int32_t size: 4
var PLUS_SECONDJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj(x,y) GraphBLAS type: ztype int32_t size: 4
var PLUS_SECONDJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj1(x,y) GraphBLAS type: ztype int32_t size: 4
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var POSITIONI
-
GraphBLAS UnaryOp: (built-in) z=positioni(x) GraphBLAS type: ztype int32_t size: 4
var POSITIONI1
-
GraphBLAS UnaryOp: (built-in) z=positioni1(x) GraphBLAS type: ztype int32_t size: 4
var POSITIONJ
-
GraphBLAS UnaryOp: (built-in) z=positionj(x) GraphBLAS type: ztype int32_t size: 4
var POSITIONJ1
-
GraphBLAS UnaryOp: (built-in) z=positionj1(x) GraphBLAS type: ztype int32_t size: 4
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var SECONDI
-
GraphBLAS BinaryOp: (built-in) z=secondi(x,y) GraphBLAS type: ztype int32_t size: 4
var SECONDI1
-
GraphBLAS BinaryOp: (built-in) z=secondi1(x,y) GraphBLAS type: ztype int32_t size: 4
var SECONDJ
-
GraphBLAS BinaryOp: (built-in) z=secondj(x,y) GraphBLAS type: ztype int32_t size: 4
var SECONDJ1
-
GraphBLAS BinaryOp: (built-in) z=secondj1(x,y) GraphBLAS type: ztype int32_t size: 4
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4
var TIMES_FIRSTI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti(x,y) GraphBLAS type: ztype int32_t size: 4
var TIMES_FIRSTI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firsti1(x,y) GraphBLAS type: ztype int32_t size: 4
var TIMES_FIRSTJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj(x,y) GraphBLAS type: ztype int32_t size: 4
var TIMES_FIRSTJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=firstj1(x,y) GraphBLAS type: ztype int32_t size: 4
var TIMES_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int32_t size: 4
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var TIMES_SECONDI
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi(x,y) GraphBLAS type: ztype int32_t size: 4
var TIMES_SECONDI1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondi1(x,y) GraphBLAS type: ztype int32_t size: 4
var TIMES_SECONDJ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj(x,y) GraphBLAS type: ztype int32_t size: 4
var TIMES_SECONDJ1
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=secondj1(x,y) GraphBLAS type: ztype int32_t size: 4
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int32_t size: 4 GraphBLAS type: xtype int32_t size: 4 GraphBLAS type: ytype int32_t size: 4
var abs
var ainv
var any
var any_div
var any_eq
var any_first
var any_firsti
var any_firsti1
var any_firstj
var any_firstj1
var any_ge
var any_gt
var any_iseq
var any_isge
var any_isgt
var any_isle
var any_islt
var any_isne
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_max
var any_min
var any_minus
var any_monoid
var any_ne
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_secondi
var any_secondi1
var any_secondj
var any_secondj1
var any_times
var band
var bclr
var bget
var bor
var bset
var bshift
var bxnor
var bxor
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_ge
var eq_gt
var eq_le
var eq_lt
var eq_ne
var first
var firsti
var firsti1
var firstj
var firstj1
var ge
var gt
var identity
var land
var land_eq
var land_ge
var land_gt
var land_le
var land_lt
var land_ne
var le
var lnot
var lor
var lor_eq
var lor_ge
var lor_gt
var lor_le
var lor_lt
var lor_ne
var lt
var lxor
var lxor_eq
var lxor_ge
var lxor_gt
var lxor_le
var lxor_lt
var lxor_ne
var max
var max_div
var max_first
var max_firsti
var max_firsti1
var max_firstj
var max_firstj1
var max_iseq
var max_isge
var max_isgt
var max_isle
var max_islt
var max_isne
var max_land
var max_lor
var max_lxor
var max_max
var max_min
var max_minus
var max_monoid
var max_pair
var max_plus
var max_rdiv
var max_rminus
var max_second
var max_secondi
var max_secondi1
var max_secondj
var max_secondj1
var max_times
var min
var min_div
var min_first
var min_firsti
var min_firsti1
var min_firstj
var min_firstj1
var min_iseq
var min_isge
var min_isgt
var min_isle
var min_islt
var min_isne
var min_land
var min_lor
var min_lxor
var min_max
var min_min
var min_minus
var min_monoid
var min_pair
var min_plus
var min_rdiv
var min_rminus
var min_second
var min_secondi
var min_secondi1
var min_secondj
var min_secondj1
var min_times
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_firsti
var plus_firsti1
var plus_firstj
var plus_firstj1
var plus_iseq
var plus_isge
var plus_isgt
var plus_isle
var plus_islt
var plus_isne
var plus_land
var plus_lor
var plus_lxor
var plus_max
var plus_min
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_secondi
var plus_secondi1
var plus_secondj
var plus_secondj1
var plus_times
var positioni
var positioni1
var positionj
var positionj1
var pow
var rdiv
var rminus
var second
var secondi
var secondi1
var secondj
var secondj1
var times
var times_div
var times_first
var times_firsti
var times_firsti1
var times_firstj
var times_firstj1
var times_iseq
var times_isge
var times_isgt
var times_isle
var times_islt
var times_isne
var times_land
var times_lor
var times_lxor
var times_max
var times_min
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_secondi
var times_secondi1
var times_secondj
var times_secondj1
var times_times
class INT16
-
GraphBLAS 16 bit signed integer.
Expand source code
class INT16(Type): """GraphBLAS 16 bit signed integer.""" _gb_type = lib.GrB_INT16 _c_type = "int16_t" _typecode = "i" _numba_t = numba.int16 _numpy_t = numpy.int16
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ]
var ANY_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int16_t size: 2
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var BAND
-
GraphBLAS BinaryOp: (built-in) z=bitand(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var BCLR
-
GraphBLAS BinaryOp: (built-in) z=bitclear(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var BGET
-
GraphBLAS BinaryOp: (built-in) z=bitget(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var BOR
-
GraphBLAS BinaryOp: (built-in) z=bitor(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var BSET
-
GraphBLAS BinaryOp: (built-in) z=bitset(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var BSHIFT
-
GraphBLAS BinaryOp: (built-in) z=bitshift(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int8_t size: 1
var BXNOR
-
GraphBLAS BinaryOp: (built-in) z=bitxnor(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var BXOR
-
GraphBLAS BinaryOp: (built-in) z=bitxor(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var EQ_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LAND_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var LXOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var MAX_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ]
var MAX_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int16_t size: 2
var MAX_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MAX_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ -32768 ] terminal: [ 32767 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var MIN_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var MIN_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ]
var MIN_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int16_t size: 2
var MIN_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var MIN_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 32767 ] terminal: [ -32768 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype int16_t size: 2
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype int16_t size: 2
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var PLUS_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int16_t size: 2
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2
var TIMES_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int16_t size: 2
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int16_t size: 2 GraphBLAS type: xtype int16_t size: 2 GraphBLAS type: ytype int16_t size: 2
var abs
var ainv
var any
var any_div
var any_eq
var any_first
var any_ge
var any_gt
var any_iseq
var any_isge
var any_isgt
var any_isle
var any_islt
var any_isne
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_max
var any_min
var any_minus
var any_monoid
var any_ne
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_times
var band
var bclr
var bget
var bor
var bset
var bshift
var bxnor
var bxor
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_ge
var eq_gt
var eq_le
var eq_lt
var eq_ne
var first
var ge
var gt
var identity
var land
var land_eq
var land_ge
var land_gt
var land_le
var land_lt
var land_ne
var le
var lnot
var lor
var lor_eq
var lor_ge
var lor_gt
var lor_le
var lor_lt
var lor_ne
var lt
var lxor
var lxor_eq
var lxor_ge
var lxor_gt
var lxor_le
var lxor_lt
var lxor_ne
var max
var max_div
var max_first
var max_iseq
var max_isge
var max_isgt
var max_isle
var max_islt
var max_isne
var max_land
var max_lor
var max_lxor
var max_max
var max_min
var max_minus
var max_monoid
var max_pair
var max_plus
var max_rdiv
var max_rminus
var max_second
var max_times
var min
var min_div
var min_first
var min_iseq
var min_isge
var min_isgt
var min_isle
var min_islt
var min_isne
var min_land
var min_lor
var min_lxor
var min_max
var min_min
var min_minus
var min_monoid
var min_pair
var min_plus
var min_rdiv
var min_rminus
var min_second
var min_times
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_iseq
var plus_isge
var plus_isgt
var plus_isle
var plus_islt
var plus_isne
var plus_land
var plus_lor
var plus_lxor
var plus_max
var plus_min
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_times
var pow
var rdiv
var rminus
var second
var times
var times_div
var times_first
var times_iseq
var times_isge
var times_isgt
var times_isle
var times_islt
var times_isne
var times_land
var times_lor
var times_lxor
var times_max
var times_min
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_times
class INT8
-
GraphBLAS 8 bit signed integer.
Expand source code
class INT8(Type): """GraphBLAS 8 bit signed integer.""" _gb_type = lib.GrB_INT8 _c_type = "int8_t" _typecode = "b" _numba_t = numba.int8 _numpy_t = numpy.int8
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ]
var ANY_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int8_t size: 1
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var BAND
-
GraphBLAS BinaryOp: (built-in) z=bitand(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var BCLR
-
GraphBLAS BinaryOp: (built-in) z=bitclear(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var BGET
-
GraphBLAS BinaryOp: (built-in) z=bitget(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var BOR
-
GraphBLAS BinaryOp: (built-in) z=bitor(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var BSET
-
GraphBLAS BinaryOp: (built-in) z=bitset(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var BSHIFT
-
GraphBLAS BinaryOp: (built-in) z=bitshift(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var BXNOR
-
GraphBLAS BinaryOp: (built-in) z=bitxnor(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var BXOR
-
GraphBLAS BinaryOp: (built-in) z=bitxor(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var EQ_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LAND_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var LXOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var MAX_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ]
var MAX_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int8_t size: 1
var MAX_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MAX_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ -128 ] terminal: [ 127 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var MIN_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var MIN_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ]
var MIN_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int8_t size: 1
var MIN_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var MIN_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 127 ] terminal: [ -128 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype int8_t size: 1
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype int8_t size: 1
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var PLUS_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int8_t size: 1
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1
var TIMES_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype int8_t size: 1
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype int8_t size: 1 GraphBLAS type: xtype int8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var abs
var ainv
var any
var any_div
var any_eq
var any_first
var any_ge
var any_gt
var any_iseq
var any_isge
var any_isgt
var any_isle
var any_islt
var any_isne
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_max
var any_min
var any_minus
var any_monoid
var any_ne
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_times
var band
var bclr
var bget
var bor
var bset
var bshift
var bxnor
var bxor
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_ge
var eq_gt
var eq_le
var eq_lt
var eq_ne
var first
var ge
var gt
var identity
var land
var land_eq
var land_ge
var land_gt
var land_le
var land_lt
var land_ne
var le
var lnot
var lor
var lor_eq
var lor_ge
var lor_gt
var lor_le
var lor_lt
var lor_ne
var lt
var lxor
var lxor_eq
var lxor_ge
var lxor_gt
var lxor_le
var lxor_lt
var lxor_ne
var max
var max_div
var max_first
var max_iseq
var max_isge
var max_isgt
var max_isle
var max_islt
var max_isne
var max_land
var max_lor
var max_lxor
var max_max
var max_min
var max_minus
var max_monoid
var max_pair
var max_plus
var max_rdiv
var max_rminus
var max_second
var max_times
var min
var min_div
var min_first
var min_iseq
var min_isge
var min_isgt
var min_isle
var min_islt
var min_isne
var min_land
var min_lor
var min_lxor
var min_max
var min_min
var min_minus
var min_monoid
var min_pair
var min_plus
var min_rdiv
var min_rminus
var min_second
var min_times
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_iseq
var plus_isge
var plus_isgt
var plus_isle
var plus_islt
var plus_isne
var plus_land
var plus_lor
var plus_lxor
var plus_max
var plus_min
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_times
var pow
var rdiv
var rminus
var second
var times
var times_div
var times_first
var times_iseq
var times_isge
var times_isgt
var times_isle
var times_islt
var times_isne
var times_land
var times_lor
var times_lxor
var times_max
var times_min
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_times
class UINT64
-
GraphBLAS 64 bit unsigned integer.
Expand source code
class UINT64(Type): """GraphBLAS 64 bit unsigned integer.""" _gb_type = lib.GrB_UINT64 _c_type = "uint64_t" _typecode = "Q" _numba_t = numba.uint64 _numpy_t = numpy.uint64
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ]
var ANY_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint64_t size: 8
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BAND
-
GraphBLAS BinaryOp: (built-in) z=bitand(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BAND_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BAND_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BAND_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BAND_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BAND_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ]
var BCLR
-
GraphBLAS BinaryOp: (built-in) z=bitclear(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BGET
-
GraphBLAS BinaryOp: (built-in) z=bitget(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BOR
-
GraphBLAS BinaryOp: (built-in) z=bitor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ]
var BSET
-
GraphBLAS BinaryOp: (built-in) z=bitset(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BSHIFT
-
GraphBLAS BinaryOp: (built-in) z=bitshift(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype int8_t size: 1
var BXNOR
-
GraphBLAS BinaryOp: (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BXNOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BXNOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BXNOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BXNOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BXNOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ]
var BXOR
-
GraphBLAS BinaryOp: (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BXOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BXOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BXOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BXOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var BXOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ]
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var EQ_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LAND_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var LXOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var MAX_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ]
var MAX_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint64_t size: 8
var MAX_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MAX_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] terminal: [ 18446744073709551615 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var MIN_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var MIN_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ]
var MIN_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint64_t size: 8
var MIN_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var MIN_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 18446744073709551615 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype uint64_t size: 8
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype uint64_t size: 8
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var PLUS_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint64_t size: 8
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8
var TIMES_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint64_t size: 8
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint64_t size: 8 GraphBLAS type: xtype uint64_t size: 8 GraphBLAS type: ytype uint64_t size: 8
var abs
var ainv
var any
var any_div
var any_eq
var any_first
var any_ge
var any_gt
var any_iseq
var any_isge
var any_isgt
var any_isle
var any_islt
var any_isne
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_max
var any_min
var any_minus
var any_monoid
var any_ne
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_times
var band
var band_band
var band_bor
var band_bxnor
var band_bxor
var band_monoid
var bclr
var bget
var bor
var bor_band
var bor_bor
var bor_bxnor
var bor_bxor
var bor_monoid
var bset
var bshift
var bxnor
var bxnor_band
var bxnor_bor
var bxnor_bxnor
var bxnor_bxor
var bxnor_monoid
var bxor
var bxor_band
var bxor_bor
var bxor_bxnor
var bxor_bxor
var bxor_monoid
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_ge
var eq_gt
var eq_le
var eq_lt
var eq_ne
var first
var ge
var gt
var identity
var land
var land_eq
var land_ge
var land_gt
var land_le
var land_lt
var land_ne
var le
var lnot
var lor
var lor_eq
var lor_ge
var lor_gt
var lor_le
var lor_lt
var lor_ne
var lt
var lxor
var lxor_eq
var lxor_ge
var lxor_gt
var lxor_le
var lxor_lt
var lxor_ne
var max
var max_div
var max_first
var max_iseq
var max_isge
var max_isgt
var max_isle
var max_islt
var max_isne
var max_land
var max_lor
var max_lxor
var max_max
var max_min
var max_minus
var max_monoid
var max_pair
var max_plus
var max_rdiv
var max_rminus
var max_second
var max_times
var min
var min_div
var min_first
var min_iseq
var min_isge
var min_isgt
var min_isle
var min_islt
var min_isne
var min_land
var min_lor
var min_lxor
var min_max
var min_min
var min_minus
var min_monoid
var min_pair
var min_plus
var min_rdiv
var min_rminus
var min_second
var min_times
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_iseq
var plus_isge
var plus_isgt
var plus_isle
var plus_islt
var plus_isne
var plus_land
var plus_lor
var plus_lxor
var plus_max
var plus_min
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_times
var pow
var rdiv
var rminus
var second
var times
var times_div
var times_first
var times_iseq
var times_isge
var times_isgt
var times_isle
var times_islt
var times_isne
var times_land
var times_lor
var times_lxor
var times_max
var times_min
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_times
class UINT32
-
GraphBLAS 32 bit unsigned integer.
Expand source code
class UINT32(Type): """GraphBLAS 32 bit unsigned integer.""" _gb_type = lib.GrB_UINT32 _c_type = "uint32_t" _typecode = "L" _numba_t = numba.uint32 _numpy_t = numpy.uint32
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ]
var ANY_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint32_t size: 4
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BAND
-
GraphBLAS BinaryOp: (built-in) z=bitand(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BAND_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BAND_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BAND_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BAND_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BAND_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ]
var BCLR
-
GraphBLAS BinaryOp: (built-in) z=bitclear(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BGET
-
GraphBLAS BinaryOp: (built-in) z=bitget(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BOR
-
GraphBLAS BinaryOp: (built-in) z=bitor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ]
var BSET
-
GraphBLAS BinaryOp: (built-in) z=bitset(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BSHIFT
-
GraphBLAS BinaryOp: (built-in) z=bitshift(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype int8_t size: 1
var BXNOR
-
GraphBLAS BinaryOp: (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BXNOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BXNOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BXNOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BXNOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BXNOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ]
var BXOR
-
GraphBLAS BinaryOp: (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BXOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BXOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BXOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BXOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var BXOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ]
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var EQ_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LAND_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var LXOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var MAX_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ]
var MAX_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint32_t size: 4
var MAX_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MAX_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] terminal: [ 4294967295 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var MIN_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var MIN_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ]
var MIN_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint32_t size: 4
var MIN_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var MIN_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 4294967295 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype uint32_t size: 4
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype uint32_t size: 4
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var PLUS_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint32_t size: 4
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4
var TIMES_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint32_t size: 4
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint32_t size: 4 GraphBLAS type: xtype uint32_t size: 4 GraphBLAS type: ytype uint32_t size: 4
var abs
var ainv
var any
var any_div
var any_eq
var any_first
var any_ge
var any_gt
var any_iseq
var any_isge
var any_isgt
var any_isle
var any_islt
var any_isne
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_max
var any_min
var any_minus
var any_monoid
var any_ne
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_times
var band
var band_band
var band_bor
var band_bxnor
var band_bxor
var band_monoid
var bclr
var bget
var bor
var bor_band
var bor_bor
var bor_bxnor
var bor_bxor
var bor_monoid
var bset
var bshift
var bxnor
var bxnor_band
var bxnor_bor
var bxnor_bxnor
var bxnor_bxor
var bxnor_monoid
var bxor
var bxor_band
var bxor_bor
var bxor_bxnor
var bxor_bxor
var bxor_monoid
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_ge
var eq_gt
var eq_le
var eq_lt
var eq_ne
var first
var ge
var gt
var identity
var land
var land_eq
var land_ge
var land_gt
var land_le
var land_lt
var land_ne
var le
var lnot
var lor
var lor_eq
var lor_ge
var lor_gt
var lor_le
var lor_lt
var lor_ne
var lt
var lxor
var lxor_eq
var lxor_ge
var lxor_gt
var lxor_le
var lxor_lt
var lxor_ne
var max
var max_div
var max_first
var max_iseq
var max_isge
var max_isgt
var max_isle
var max_islt
var max_isne
var max_land
var max_lor
var max_lxor
var max_max
var max_min
var max_minus
var max_monoid
var max_pair
var max_plus
var max_rdiv
var max_rminus
var max_second
var max_times
var min
var min_div
var min_first
var min_iseq
var min_isge
var min_isgt
var min_isle
var min_islt
var min_isne
var min_land
var min_lor
var min_lxor
var min_max
var min_min
var min_minus
var min_monoid
var min_pair
var min_plus
var min_rdiv
var min_rminus
var min_second
var min_times
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_iseq
var plus_isge
var plus_isgt
var plus_isle
var plus_islt
var plus_isne
var plus_land
var plus_lor
var plus_lxor
var plus_max
var plus_min
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_times
var pow
var rdiv
var rminus
var second
var times
var times_div
var times_first
var times_iseq
var times_isge
var times_isgt
var times_isle
var times_islt
var times_isne
var times_land
var times_lor
var times_lxor
var times_max
var times_min
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_times
class UINT16
-
GraphBLAS 16 bit unsigned integer.
Expand source code
class UINT16(Type): """GraphBLAS 16 bit unsigned integer.""" _gb_type = lib.GrB_UINT16 _c_type = "uint16_t" _typecode = "I" _numba_t = numba.uint16 _numpy_t = numpy.uint16
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ]
var ANY_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint16_t size: 2
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BAND
-
GraphBLAS BinaryOp: (built-in) z=bitand(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BAND_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BAND_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BAND_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BAND_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BAND_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ]
var BCLR
-
GraphBLAS BinaryOp: (built-in) z=bitclear(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BGET
-
GraphBLAS BinaryOp: (built-in) z=bitget(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BOR
-
GraphBLAS BinaryOp: (built-in) z=bitor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ]
var BSET
-
GraphBLAS BinaryOp: (built-in) z=bitset(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BSHIFT
-
GraphBLAS BinaryOp: (built-in) z=bitshift(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype int8_t size: 1
var BXNOR
-
GraphBLAS BinaryOp: (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BXNOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BXNOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BXNOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BXNOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BXNOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ]
var BXOR
-
GraphBLAS BinaryOp: (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BXOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BXOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BXOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BXOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var BXOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ]
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var EQ_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LAND_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var LXOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var MAX_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ]
var MAX_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint16_t size: 2
var MAX_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MAX_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] terminal: [ 65535 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var MIN_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var MIN_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ]
var MIN_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint16_t size: 2
var MIN_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var MIN_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 65535 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype uint16_t size: 2
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype uint16_t size: 2
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var PLUS_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint16_t size: 2
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2
var TIMES_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint16_t size: 2
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint16_t size: 2 GraphBLAS type: xtype uint16_t size: 2 GraphBLAS type: ytype uint16_t size: 2
var abs
var ainv
var any
var any_div
var any_eq
var any_first
var any_ge
var any_gt
var any_iseq
var any_isge
var any_isgt
var any_isle
var any_islt
var any_isne
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_max
var any_min
var any_minus
var any_monoid
var any_ne
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_times
var band
var band_band
var band_bor
var band_bxnor
var band_bxor
var band_monoid
var bclr
var bget
var bor
var bor_band
var bor_bor
var bor_bxnor
var bor_bxor
var bor_monoid
var bset
var bshift
var bxnor
var bxnor_band
var bxnor_bor
var bxnor_bxnor
var bxnor_bxor
var bxnor_monoid
var bxor
var bxor_band
var bxor_bor
var bxor_bxnor
var bxor_bxor
var bxor_monoid
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_ge
var eq_gt
var eq_le
var eq_lt
var eq_ne
var first
var ge
var gt
var identity
var land
var land_eq
var land_ge
var land_gt
var land_le
var land_lt
var land_ne
var le
var lnot
var lor
var lor_eq
var lor_ge
var lor_gt
var lor_le
var lor_lt
var lor_ne
var lt
var lxor
var lxor_eq
var lxor_ge
var lxor_gt
var lxor_le
var lxor_lt
var lxor_ne
var max
var max_div
var max_first
var max_iseq
var max_isge
var max_isgt
var max_isle
var max_islt
var max_isne
var max_land
var max_lor
var max_lxor
var max_max
var max_min
var max_minus
var max_monoid
var max_pair
var max_plus
var max_rdiv
var max_rminus
var max_second
var max_times
var min
var min_div
var min_first
var min_iseq
var min_isge
var min_isgt
var min_isle
var min_islt
var min_isne
var min_land
var min_lor
var min_lxor
var min_max
var min_min
var min_minus
var min_monoid
var min_pair
var min_plus
var min_rdiv
var min_rminus
var min_second
var min_times
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_iseq
var plus_isge
var plus_isgt
var plus_isle
var plus_islt
var plus_isne
var plus_land
var plus_lor
var plus_lxor
var plus_max
var plus_min
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_times
var pow
var rdiv
var rminus
var second
var times
var times_div
var times_first
var times_iseq
var times_isge
var times_isgt
var times_isle
var times_islt
var times_isne
var times_land
var times_lor
var times_lxor
var times_max
var times_min
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_times
class UINT8
-
GraphBLAS 8 bit unsigned integer.
Expand source code
class UINT8(Type): """GraphBLAS 8 bit unsigned integer.""" _gb_type = lib.GrB_UINT8 _c_type = "uint8_t" _typecode = "B" _numba_t = numba.uint8 _numpy_t = numpy.uint8
Class Attributes
var ABS
-
GraphBLAS UnaryOp: (built-in) z=abs(x) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var AINV
-
GraphBLAS UnaryOp: (built-in) z=ainv(x) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var ANY
-
GraphBLAS BinaryOp: (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var ANY_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ]
var ANY_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint8_t size: 1
var ANY_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ANY_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=any(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BAND
-
GraphBLAS BinaryOp: (built-in) z=bitand(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BAND_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BAND_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BAND_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BAND_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BAND_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitand(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ]
var BCLR
-
GraphBLAS BinaryOp: (built-in) z=bitclear(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BGET
-
GraphBLAS BinaryOp: (built-in) z=bitget(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BOR
-
GraphBLAS BinaryOp: (built-in) z=bitor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ]
var BSET
-
GraphBLAS BinaryOp: (built-in) z=bitset(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BSHIFT
-
GraphBLAS BinaryOp: (built-in) z=bitshift(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype int8_t size: 1
var BXNOR
-
GraphBLAS BinaryOp: (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BXNOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BXNOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BXNOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BXNOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BXNOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ]
var BXOR
-
GraphBLAS BinaryOp: (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BXOR_BAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitand(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BXOR_BOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BXOR_BXNOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxnor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BXOR_BXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var BXOR_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=bitxor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ]
var DIV
-
GraphBLAS BinaryOp: (built-in) z=div(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var EQ
-
GraphBLAS BinaryOp: (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var EQ_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var EQ_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var EQ_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var EQ_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var EQ_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var EQ_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var FIRST
-
GraphBLAS BinaryOp: (built-in) z=first(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var GE
-
GraphBLAS BinaryOp: (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var GT
-
GraphBLAS BinaryOp: (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var IDENTITY
-
GraphBLAS UnaryOp: (built-in) z=identity(x) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var LAND
-
GraphBLAS BinaryOp: (built-in) z=and(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LAND_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LAND_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LAND_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LAND_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LAND_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LAND_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=and(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LE
-
GraphBLAS BinaryOp: (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LNOT
-
GraphBLAS UnaryOp: (built-in) z=not(x) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var LOR
-
GraphBLAS BinaryOp: (built-in) z=or(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=or(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] terminal: [ 1 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LT
-
GraphBLAS BinaryOp: (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LXOR
-
GraphBLAS BinaryOp: (built-in) z=xor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LXOR_EQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=eq(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LXOR_GE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ge(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LXOR_GT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=gt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LXOR_LE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=le(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LXOR_LT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=lt(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var LXOR_NE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=xor(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype bool size: 1 GraphBLAS type: ytype bool size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX
-
GraphBLAS BinaryOp: (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var MAX_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ]
var MAX_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint8_t size: 1
var MAX_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MAX_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] terminal: [ 255 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN
-
GraphBLAS BinaryOp: (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MINUS
-
GraphBLAS BinaryOp: (built-in) z=minus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MINV
-
GraphBLAS UnaryOp: (built-in) z=minv(x) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var MIN_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var MIN_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ]
var MIN_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint8_t size: 1
var MIN_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var MIN_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 255 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var NE
-
GraphBLAS BinaryOp: (built-in) z=ne(x,y) GraphBLAS type: ztype bool size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var ONE
-
GraphBLAS UnaryOp: (built-in) z=one(x) GraphBLAS type: ztype uint8_t size: 1
var PAIR
-
GraphBLAS BinaryOp: (built-in) z=pair(x,y) GraphBLAS type: ztype uint8_t size: 1
var PLUS
-
GraphBLAS BinaryOp: (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var PLUS_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ]
var PLUS_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint8_t size: 1
var PLUS_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var PLUS_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var POW
-
GraphBLAS BinaryOp: (built-in) z=pow(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var RDIV
-
GraphBLAS BinaryOp: (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var RMINUS
-
GraphBLAS BinaryOp: (built-in) z=rminus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var SECOND
-
GraphBLAS BinaryOp: (built-in) z=second(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES
-
GraphBLAS BinaryOp: (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_DIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=div(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_FIRST
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=first(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1
var TIMES_ISEQ
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=iseq(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_ISGE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isge(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_ISGT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isgt(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_ISLE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isle(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_ISLT
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=islt(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_ISNE
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=isne(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_LAND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=and(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_LOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=or(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_LXOR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=xor(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_MAX
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=max(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_MIN
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=min(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_MINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=minus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_MONOID
-
GraphBLAS Monoid: (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ]
var TIMES_PAIR
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=pair(x,y) GraphBLAS type: ztype uint8_t size: 1
var TIMES_PLUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=plus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_RDIV
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rdiv(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_RMINUS
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=rminus(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_SECOND
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=second(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var TIMES_TIMES
-
GraphBLAS Semiring: (built-in) GraphBLAS Monoid: semiring->add (built-in) GraphBLAS BinaryOp: monoid->op (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1 identity: [ 1 ] terminal: [ 0 ] GraphBLAS BinaryOp: semiring->multiply (built-in) z=times(x,y) GraphBLAS type: ztype uint8_t size: 1 GraphBLAS type: xtype uint8_t size: 1 GraphBLAS type: ytype uint8_t size: 1
var abs
var ainv
var any
var any_div
var any_eq
var any_first
var any_ge
var any_gt
var any_iseq
var any_isge
var any_isgt
var any_isle
var any_islt
var any_isne
var any_land
var any_le
var any_lor
var any_lt
var any_lxor
var any_max
var any_min
var any_minus
var any_monoid
var any_ne
var any_pair
var any_plus
var any_rdiv
var any_rminus
var any_second
var any_times
var band
var band_band
var band_bor
var band_bxnor
var band_bxor
var band_monoid
var bclr
var bget
var bor
var bor_band
var bor_bor
var bor_bxnor
var bor_bxor
var bor_monoid
var bset
var bshift
var bxnor
var bxnor_band
var bxnor_bor
var bxnor_bxnor
var bxnor_bxor
var bxnor_monoid
var bxor
var bxor_band
var bxor_bor
var bxor_bxnor
var bxor_bxor
var bxor_monoid
var default_one
var default_zero
var div
var eq
var eq_eq
var eq_ge
var eq_gt
var eq_le
var eq_lt
var eq_ne
var first
var ge
var gt
var identity
var land
var land_eq
var land_ge
var land_gt
var land_le
var land_lt
var land_ne
var le
var lnot
var lor
var lor_eq
var lor_ge
var lor_gt
var lor_le
var lor_lt
var lor_ne
var lt
var lxor
var lxor_eq
var lxor_ge
var lxor_gt
var lxor_le
var lxor_lt
var lxor_ne
var max
var max_div
var max_first
var max_iseq
var max_isge
var max_isgt
var max_isle
var max_islt
var max_isne
var max_land
var max_lor
var max_lxor
var max_max
var max_min
var max_minus
var max_monoid
var max_pair
var max_plus
var max_rdiv
var max_rminus
var max_second
var max_times
var min
var min_div
var min_first
var min_iseq
var min_isge
var min_isgt
var min_isle
var min_islt
var min_isne
var min_land
var min_lor
var min_lxor
var min_max
var min_min
var min_minus
var min_monoid
var min_pair
var min_plus
var min_rdiv
var min_rminus
var min_second
var min_times
var minus
var minv
var ne
var one
var pair
var plus
var plus_div
var plus_first
var plus_iseq
var plus_isge
var plus_isgt
var plus_isle
var plus_islt
var plus_isne
var plus_land
var plus_lor
var plus_lxor
var plus_max
var plus_min
var plus_minus
var plus_monoid
var plus_pair
var plus_plus
var plus_rdiv
var plus_rminus
var plus_second
var plus_times
var pow
var rdiv
var rminus
var second
var times
var times_div
var times_first
var times_iseq
var times_isge
var times_isgt
var times_isle
var times_islt
var times_isne
var times_land
var times_lor
var times_lxor
var times_max
var times_min
var times_minus
var times_monoid
var times_pair
var times_plus
var times_rdiv
var times_rminus
var times_second
var times_times
Sub-modules
pygraphblas.descriptor
-
Contains all automatically generated Descriptors from CFFI.
pygraphblas.gviz
-
Helper functions for drawing graphs and matrices with graphviz in doctests and Jupyter notebooks …
pygraphblas.selectop
-
Contains all automatically generated SelectOps from CFFI …
Global variables
var GxB_INDEX_MAX
-
Maximum key size for SuiteSparse, defaults to
2**60
. var GxB_IMPLEMENTATION
-
Tuple containing GxB_IMPLEMENTATION (MAJOR, MINOR, SUB)
var GxB_SPEC
-
Tuple containing GxB_SPEC (MAJOR, MINOR, SUB)
Functions
def binary_op(arg_type, nopython=True)
-
Decorator to jit-compile Python function into a GrB_BinaryOp object.
>>> from random import uniform >>> from pygraphblas import Matrix, binary_op, types, gviz >>> @binary_op(types.FP64) ... def uniform(x, y): ... return uniform(x, y) >>> A = Matrix.dense(types.FP64, 3, 3, fill=0) >>> B = A.dup() >>> with uniform: ... A += 1
Calling
A += 1
with theuniform
binary operator is the same as callingapply_second
with anout
parameter:>>> B.apply_second(uniform, 1, out=B) is B True >>> ga = gviz.draw_matrix(A, scale=40, ... filename='docs/imgs/binary_op_A') >>> gb = gviz.draw_matrix(B, scale=40, ... filename='docs/imgs/binary_op_B')
Expand source code
def binary_op(arg_type, nopython=True): """Decorator to jit-compile Python function into a GrB_BinaryOp object. >>> from random import uniform >>> from pygraphblas import Matrix, binary_op, types, gviz >>> @binary_op(types.FP64) ... def uniform(x, y): ... return uniform(x, y) >>> A = Matrix.dense(types.FP64, 3, 3, fill=0) >>> B = A.dup() >>> with uniform: ... A += 1 Calling `A += 1` with the `uniform` binary operator is the same as calling `apply_second` with an `out` parameter: >>> B.apply_second(uniform, 1, out=B) is B True >>> ga = gviz.draw_matrix(A, scale=40, ... filename='docs/imgs/binary_op_A') >>> gb = gviz.draw_matrix(B, scale=40, ... filename='docs/imgs/binary_op_B') 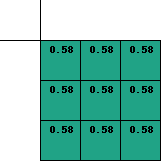 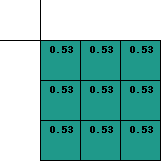 """ def inner(func): func_name = func.__name__ sig = numba.void( numba.types.CPointer(arg_type._numba_t), numba.types.CPointer(arg_type._numba_t), numba.types.CPointer(arg_type._numba_t), ) jitfunc = numba.jit(func, nopython=nopython) @numba.cfunc(sig, nopython=True) def wrapper(z, x, y): # pragma: no cover result = jitfunc(x[0], y[0]) z[0] = result out = ffi.new("GrB_BinaryOp*") lib.GrB_BinaryOp_new( out, ffi.cast("GxB_binary_function", wrapper.address), arg_type._gb_type, arg_type._gb_type, arg_type._gb_type, ) return BinaryOp(func_name, arg_type.__name__, out[0]) return inner
def unary_op(arg_type)
-
Decorator to jit-compile Python function into a GrB_BinaryOp object.
>>> from random import random >>> from pygraphblas import Matrix, binary_op, types, gviz >>> @unary_op(types.FP64) ... def random(x): ... return x * random() >>> A = Matrix.dense(types.FP64, 3, 3, fill=1) >>> A.apply(random, out=A) is A True
>>> ga = gviz.draw_matrix(A, scale=40, ... filename='docs/imgs/unary_op_A')
Expand source code
def unary_op(arg_type): """Decorator to jit-compile Python function into a GrB_BinaryOp object. >>> from random import random >>> from pygraphblas import Matrix, binary_op, types, gviz >>> @unary_op(types.FP64) ... def random(x): ... return x * random() >>> A = Matrix.dense(types.FP64, 3, 3, fill=1) >>> A.apply(random, out=A) is A True >>> ga = gviz.draw_matrix(A, scale=40, ... filename='docs/imgs/unary_op_A') 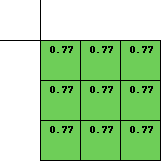 """ def inner(func): func_name = func.__name__ sig = numba.void( numba.types.CPointer(arg_type._numba_t), numba.types.CPointer(arg_type._numba_t), ) jitfunc = numba.jit(func, nopython=True) @numba.cfunc(sig, nopython=True) def wrapper(z, x): # pragma: no cover result = jitfunc(x[0]) z[0] = result out = core_ffi.new("GrB_UnaryOp*") lib.GrB_UnaryOp_new( out, core_ffi.cast("GxB_unary_function", wrapper.address), arg_type._gb_type, arg_type._gb_type, ) return UnaryOp(func_name, arg_type.__name__, out[0]) return inner
def select_op(arg_type, thunk_type=None)
-
Decorator to jit-compile Python function into a GrB_BinaryOp object.
>>> from random import random >>> from pygraphblas import Matrix, select_op, types, gviz, descriptor >>> @select_op(types.FP64, types.FP64) ... def random_gt(i, j, x, v): ... if random() > v: ... return True ... return False >>> A = Matrix.dense(types.FP64, 3, 3, fill=1) >>> A.select(random_gt, 0.5, out=A, desc=descriptor.R) is A True >>> ga = gviz.draw_matrix(A, scale=40, ... filename='docs/imgs/select_op_A')
>>> @select_op(types.FP64) ... def coin_flip(i, j, x, v): ... if random() > 0.5: ... return True ... return False >>> A = Matrix.dense(types.FP64, 3, 3, fill=1) >>> A.select(coin_flip) <Matrix(FP64, shape: (3, 3), nvals: ...)>
Expand source code
def select_op(arg_type, thunk_type=None): """Decorator to jit-compile Python function into a GrB_BinaryOp object. >>> from random import random >>> from pygraphblas import Matrix, select_op, types, gviz, descriptor >>> @select_op(types.FP64, types.FP64) ... def random_gt(i, j, x, v): ... if random() > v: ... return True ... return False >>> A = Matrix.dense(types.FP64, 3, 3, fill=1) >>> A.select(random_gt, 0.5, out=A, desc=descriptor.R) is A True >>> ga = gviz.draw_matrix(A, scale=40, ... filename='docs/imgs/select_op_A') 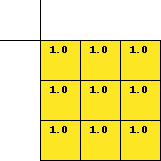 >>> @select_op(types.FP64) ... def coin_flip(i, j, x, v): ... if random() > 0.5: ... return True ... return False >>> A = Matrix.dense(types.FP64, 3, 3, fill=1) >>> A.select(coin_flip) <Matrix(FP64, shape: (3, 3), nvals: ...)> """ if thunk_type is not None: thunk_type = thunk_type._gb_type else: thunk_type = core_ffi.NULL def inner(func): func_name = func.__name__ sig = numba.boolean( numba.types.uint64, numba.types.uint64, numba.types.CPointer(arg_type._numba_t), numba.types.CPointer(arg_type._numba_t), ) jitfunc = numba.jit(func, nopython=True) @numba.cfunc(sig, nopython=True) def wrapper(i, j, x, t): # pragma: no cover return jitfunc(i, j, x[0], t[0]) out = core_ffi.new("GxB_SelectOp*") lib.GxB_SelectOp_new( out, core_ffi.cast("GxB_select_function", wrapper.address), arg_type._gb_type, thunk_type, ) return SelectOp(func_name, out[0]) return inner
def options_set(nthreads=None, chunk=None, burble=None, hyper_switch=None, bitmap_switch=None, format=None)
-
Set global library options.
This options are passed directly to SuiteSparse so see the SuiteSparse User Guide for details.
-
nthreads
: Globals number of threads to use. -
chunk
: Chunk size for dividing parallel work. -
burble
: Switch to enable "burble" debug output. SuiteSparse must be compiled with burble turned on. -
hyper_switch
: Controls the hypersparsity of the internal data structure for a matrix. The parameter is typically in the range 0 to 1. -
bitmap_switch
: Controls when to switch to bitmap format. -
format
: Default global matrix data format.
Expand source code
def options_set( nthreads=None, chunk=None, burble=None, hyper_switch=None, bitmap_switch=None, format=None, ): """Set global library options. This options are passed directly to SuiteSparse so see the SuiteSparse User Guide for details. - `nthreads`: Globals number of threads to use. - `chunk`: Chunk size for dividing parallel work. - `burble`: Switch to enable "burble" debug output. SuiteSparse must be compiled with burble turned on. - `hyper_switch`: Controls the hypersparsity of the internal data structure for a matrix. The parameter is typically in the range 0 to 1. - `bitmap_switch`: Controls when to switch to bitmap format. - `format`: Default global matrix data format. """ if nthreads is not None: nthreads = ffi.cast("int", nthreads) _check(lib.GxB_Global_Option_set(lib.GxB_GLOBAL_NTHREADS, nthreads)) if chunk is not None: chunk = ffi.cast("double", chunk) _check(lib.GxB_Global_Option_set(lib.GxB_GLOBAL_CHUNK, chunk)) if burble is not None: burble = ffi.cast("int", burble) _check(lib.GxB_Global_Option_set(lib.GxB_BURBLE, burble)) if hyper_switch is not None: hyper_switch = ffi.cast("double", hyper_switch) _check(lib.GxB_Global_Option_set(lib.GxB_HYPER_SWITCH, hyper_switch)) if bitmap_switch is not None: bitmap_switch = ffi.new("double[8]", bitmap_switch) _check(lib.GxB_Global_Option_set(lib.GxB_BITMAP_SWITCH, bitmap_switch)) if format is not None: format = ffi.cast("GxB_Format_Value*", format) _check(lib.GxB_Global_Option_set(lib.GxB_FORMAT, format))
-
def options_get()
-
Get global library options. See SuiteSparse User Guide.
>>> pprint(options_get()) {'bitmap_switch': [...], 'burble': ..., 'chunk': ..., 'format': ..., 'hyper_switch': ..., 'nthreads': ...}
Expand source code
def options_get(): """Get global library options. See SuiteSparse User Guide. >>> pprint(options_get()) {'bitmap_switch': [...], 'burble': ..., 'chunk': ..., 'format': ..., 'hyper_switch': ..., 'nthreads': ...} """ nthreads = ffi.new("int*") _check(lib.GxB_Global_Option_get(lib.GxB_GLOBAL_NTHREADS, nthreads)) chunk = ffi.new("double*") _check(lib.GxB_Global_Option_get(lib.GxB_GLOBAL_CHUNK, chunk)) burble = ffi.new("int*") _check(lib.GxB_Global_Option_get(lib.GxB_BURBLE, burble)) hyper_switch = ffi.new("double*") _check(lib.GxB_Global_Option_get(lib.GxB_HYPER_SWITCH, hyper_switch)) bitmap_switch = ffi.new("double[8]") _check(lib.GxB_Global_Option_get(lib.GxB_BITMAP_SWITCH, bitmap_switch)) format = ffi.new("GxB_Format_Value*") _check(lib.GxB_Global_Option_get(lib.GxB_FORMAT, format)) return dict( nthreads=nthreads[0], chunk=chunk[0], burble=burble[0], hyper_switch=hyper_switch[0], bitmap_switch=list(bitmap_switch), format=format[0], )